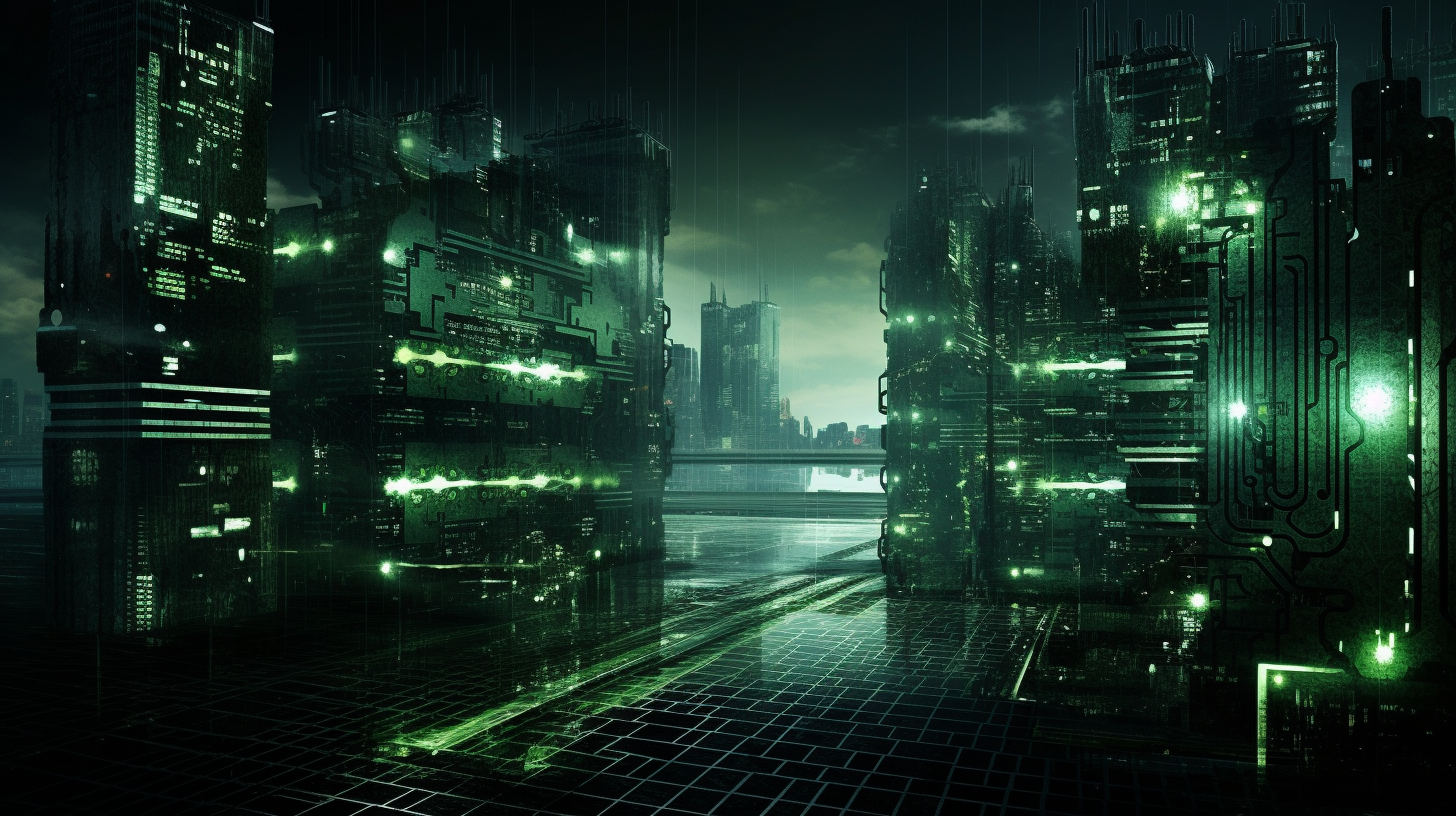
SQL Clustering Techniques for Scalability
SQL clustering techniques are essential for managing large datasets and improving query performance. At their core, clustering methods group related data together, which allows for more efficient data retrieval and manipulation. Understanding these techniques very important for database administrators and developers aiming to optimize the performance and scalability of their SQL databases.
Clustering is primarily concerned with how data is organized on disk and how it can be accessed efficiently by SQL queries. The goal is to minimize the amount of I/O operations required to retrieve data, thereby increasing performance. When data is physically organized to reflect the logical relationships inherent in the data model, it can be retrieved more quickly.
Two primary concepts underpin SQL clustering techniques:
- A clustered index determines the physical order of data in a table. Each table can have only one clustered index because the data rows themselves can only be sorted in one order. This index is particularly useful for range queries, where retrieving a sequence of rows can be optimized.
- These indexes do not alter the physical order of the data but create a separate structure that points to the data rows. A table can have multiple non-clustered indexes, which can be leveraged for different types of queries.
To illustrate the idea of clustered indexes, ponder the following SQL example that creates a clustered index on a table called Employees
:
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, LastName VARCHAR(50), FirstName VARCHAR(50), HireDate DATE ); CREATE CLUSTERED INDEX IDX_HireDate ON Employees(HireDate);
In this example, the HireDate
column is used to define the clustered index, which organizes the Employees
table’s rows based on the hire date. This allows for more efficient queries that retrieve employees hired within a specific date range.
Another important aspect of clustering is the use of partitioning to manage large tables. Partitioning involves dividing a table into smaller, more manageable pieces, known as partitions. Each partition can be treated as a separate table, allowing for more efficient data management and query execution.
For instance, if you have a large sales table, you can partition it based on the year:
CREATE TABLE Sales ( SaleID INT PRIMARY KEY, SaleDate DATE, Amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(SaleDate)) ( PARTITION p2022 VALUES LESS THAN (2023), PARTITION p2023 VALUES LESS THAN (2024) );
This example distributes sales data across two partitions based on the year, making it easier to manage and query specific time frames.
Understanding these clustering techniques allows database professionals to design systems that can handle increasing loads while minimizing the performance hit typically associated with large datasets. By effectively using clustered and non-clustered indexes, as well as partitioning data, organizations can achieve scalable and performant SQL databases.
Types of Clustering Methods in SQL
When delving deeper into the types of clustering methods available in SQL, it is essential to distinguish not just between clustered and non-clustered indexes, but also to explore the various indexing strategies that can enhance performance and scalability. Each of these strategies has unique characteristics and ideal use cases, making it critical for database administrators to select the right method based on specific workload requirements.
Clustered Indexes are the most fundamental type of indexing in SQL. As mentioned previously, they dictate the physical order of data in a table. This means that the data rows are stored on disk in the same order as the clustered index, which can speed up retrieval times for range queries significantly. However, maintaining a clustered index does come with its challenges, particularly when it comes to insertions, updates, and deletions, as these operations may require the reordering of existing data to maintain the physical order.
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100), Price DECIMAL(10, 2) ); CREATE CLUSTERED INDEX IDX_ProductName ON Products(ProductName);
In the example above, the Products table creates a clustered index on ProductName. This organization can drastically improve performance for queries that search for products by name, but it may slow down performance for insert operations due to potential page splits.
Non-Clustered Indexes, on the other hand, maintain a structure separate from the data itself. This allows for multiple non-clustered indexes on a single table, making them highly versatile. However, the trade-off is that accessing data via a non-clustered index may require an additional lookup to fetch the actual data rows, which can add overhead.
CREATE NONCLUSTERED INDEX IDX_Price ON Products(Price);
This SQL command creates a non-clustered index on the Price column of the Products table, enabling fast lookups for queries that filter based on product pricing without altering the physical storage of the data.
Bitmap Indexes are another type of indexing strategy that can be particularly useful in scenarios with low cardinality, where the number of unique values in a column is relatively small compared to the total number of rows. Bitmap indexes store a bitmap for each distinct value, making them efficient for read-heavy scenarios, especially in data warehousing.
CREATE BITMAP INDEX IDX_Category ON Products(ProductCategory);
While support for bitmap indexes can vary by SQL implementation, many systems, like Oracle, fully utilize this indexing strategy to speed up analytics queries against large datasets.
Additionally, Full-Text Indexes provide capabilities for searching large text columns effectively. They enable natural language queries, supporting advanced search functionalities such as stemming, thesaurus support, and ranking of results based on relevance.
CREATE FULLTEXT INDEX ON Products(ProductDescription);
This example demonstrates the creation of a full-text index on the ProductDescription column, thereby allowing for more sophisticated search capabilities than traditional LIKE queries.
Lastly, Spatial Indexes cater to geospatial data, enabling efficient querying of location-based information. These are particularly useful in applications that require geographical data processing, such as mapping services or location-based queries.
CREATE SPATIAL INDEX IDX_Location ON Locations(GeoData);
Understanding the various types of clustering methods in SQL equips database administrators with the tools necessary to optimize performance under varying workloads. Each indexing strategy has its strengths and weaknesses, and knowing when to apply them will ultimately lead to more efficient and scalable database solutions.
Implementing SQL Clustering for Performance Optimization
When implementing SQL clustering for performance optimization, it is essential to adhere to a structured approach that revolves around understanding the workload, data access patterns, and overall database architecture. The implementation process typically involves a series of steps aimed at maximizing data retrieval efficiency while minimizing overhead associated with data modifications. Below are several critical practices for implementing effective SQL clustering.
Identifying the Right Columns for Indexing
The first step in implementing clustering techniques is to identify which columns should be indexed. Focus on columns frequently used in WHERE clauses, JOIN conditions, and ORDER BY statements. Analyzing query patterns through execution plans can provide insights into which columns are predominantly accessed, guiding the selection of appropriate indexes.
SELECT * FROM sys.dm_db_index_usage_stats WHERE database_id = DB_ID('YourDatabaseName');
This query retrieves index usage statistics, helping you identify which indexes are frequently accessed and should be optimized or created.
Choosing Between Clustered and Non-Clustered Indexes
Once you’ve identified the critical columns, you need to decide whether to implement clustered or non-clustered indexes. If your data retrieval frequently requires sorting or is range-based, a clustered index is appropriate. For example, if users frequently query records based on creation dates, think creating a clustered index on the date column.
CREATE CLUSTERED INDEX IDX_CreationDate ON YourTableName(CreationDate);
Conversely, if you have multiple query patterns against the same table requiring different access paths, leverage non-clustered indexes. For instance, if you are often filtering by both ‘Category’ and ‘Price’, creating separate non-clustered indexes can be beneficial.
CREATE NONCLUSTERED INDEX IDX_Category ON YourTableName(Category); CREATE NONCLUSTERED INDEX IDX_Price ON YourTableName(Price);
Using Partitioning to enhance Performance
Partitioning large tables can significantly improve query performance by allowing SQL Server to read only the relevant partitions rather than scanning the entire table. When defining partitions, think how data is accessed over time. For example, if your data is time-based, partitioning by year or month can lead to more efficient queries.
CREATE TABLE Sales ( SaleID INT PRIMARY KEY, SaleDate DATE, Amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(SaleDate)) ( PARTITION p2021 VALUES LESS THAN (2022), PARTITION p2022 VALUES LESS THAN (2023), PARTITION p2023 VALUES LESS THAN (2024) );
With this partition setup, queries targeting specific years will only scan the relevant partition, dramatically reducing I/O operations.
Monitoring and Maintaining Indexes
Effective clustering is not a set-it-and-forget-it process. Regular monitoring of index usage especially important. SQL Server provides dynamic management views (DMVs) that can be utilized for this monitoring. Keeping an eye on index fragmentation is also essential, as excessive fragmentation can lead to degraded performance over time.
SELECT OBJECT_NAME(i.object_id) AS TableName, i.name AS IndexName, ps.avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats (DB_ID(), NULL, NULL, NULL, 'LIMITED') AS ps JOIN sys.indexes AS i ON ps.object_id = i.object_id AND ps.index_id = i.index_id WHERE ps.avg_fragmentation_in_percent > 30;
By regularly assessing fragmentation and re-indexing as needed, you can maintain optimal performance levels.
Testing and Validating Performance Gains
Finally, once the changes have been implemented, rigorous testing is necessary. Use query performance metrics before and after implementing clustering techniques to gauge improvements. SQL Server Profiler and Execution Plans can help you evaluate how well the indexes are serving your queries.
SET STATISTICS IO ON; SET STATISTICS TIME ON; SELECT * FROM YourTableName WHERE YourCondition;
By focusing on these strategies, database professionals can effectively implement SQL clustering techniques that not only enhance performance but also ensure that systems remain responsive as data scales. This proactive approach to clustering will yield significant dividends in the long run, accommodating growth without sacrificing speed or efficiency.
Challenges and Best Practices for SQL Clustering
SQL clustering, while powerful for improving database performance, does come with its own set of challenges. Recognizing these challenges and adopting best practices is essential for maximizing the benefits of clustering techniques and ensuring sustained performance as data volumes grow. Below are key challenges and corresponding best practices that database administrators should ponder.
Challenges with SQL Clustering
One of the primary challenges with SQL clustering is the potential for increased maintenance overhead. Clustered indexes, by their nature, dictate the physical order of data, which means that operations such as inserts, updates, and deletes can lead to page splits and fragmentation. This can degrade performance over time, necessitating regular maintenance tasks like index rebuilding or reorganizing.
Another issue arises from the complexity of query optimization. As the number of indexes grows, the SQL Server query optimizer must work harder to determine the most efficient execution plan, which can sometimes lead to suboptimal performance if the statistics are outdated or the query patterns change.
Additionally, there is the risk of over-indexing. While it may seem beneficial to create a high number of indexes to cover various queries, excessive indexing can lead to diminished performance during data modification operations, due to the need to maintain multiple indexes simultaneously.
Best Practices for SQL Clustering
To mitigate these challenges, several best practices can be implemented:
1. Regular Index Maintenance
Establish a routine for monitoring and maintaining indexes. Use tools and scripts to check for fragmentation and update statistics regularly. SQL Server provides the sys.dm_db_index_physical_stats
function to help assess the health of your indexes.
SELECT OBJECT_NAME(i.object_id) AS TableName, i.name AS IndexName, ps.avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats (DB_ID(), NULL, NULL, NULL, 'LIMITED') AS ps JOIN sys.indexes AS i ON ps.object_id = i.object_id AND ps.index_id = i.index_id WHERE ps.avg_fragmentation_in_percent > 30;
This query identifies indexes that may need attention due to high fragmentation, allowing for timely reorganization or rebuilding.
2. Analyze Query Patterns
Use execution plans and query statistics to understand which queries are executed most frequently and how indexes are being utilized. This analysis will help you make data-driven decisions about which indexes to keep, modify, or remove.
SET STATISTICS IO ON; SET STATISTICS TIME ON; SELECT * FROM YourTableName WHERE YourCondition;
By examining the output of this command, you can identify how much I/O and time are consumed by your queries, informing your indexing strategy.
3. Balance Clustered and Non-Clustered Indexes
Carefully evaluate when to use clustered versus non-clustered indexes. While clustered indexes are great for range queries, non-clustered indexes can provide flexibility without altering the physical data storage. Striking a balance here can help improve performance without incurring excessive maintenance costs.
CREATE NONCLUSTERED INDEX IDX_ColumnName ON YourTableName(ColumnName);
Creating targeted non-clustered indexes for frequently queried columns can significantly enhance performance, particularly in read-heavy databases.
4. Utilize Partitioning Wisely
When working with large datasets, partitioning can be an important strategy. However, the partitioning strategy should align with how data is queried. For example, if queries often target specific time frames, partitioning by date can reduce the amount of data scanned, improving performance.
CREATE TABLE Sales ( SaleID INT PRIMARY KEY, SaleDate DATE, Amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(SaleDate)) ( PARTITION p2021 VALUES LESS THAN (2022), PARTITION p2022 VALUES LESS THAN (2023), PARTITION p2023 VALUES LESS THAN (2024) );
This partitioning strategy ensures that only relevant partitions are accessed during queries, thus optimizing performance.
5. Document and Review Indexes Regularly
Maintain comprehensive documentation of all indexes, including their purpose, usage statistics, and any related queries. Regularly review this documentation to adapt to changing workload patterns, ensuring that your indexing strategy evolves alongside your application requirements.
By embracing these best practices and addressing the inherent challenges of SQL clustering, database administrators can create highly efficient, scalable systems capable of handling large datasets and complex queries with ease. This proactive approach not only enhances performance but also ensures that the database environment remains robust and responsive as data needs evolve.