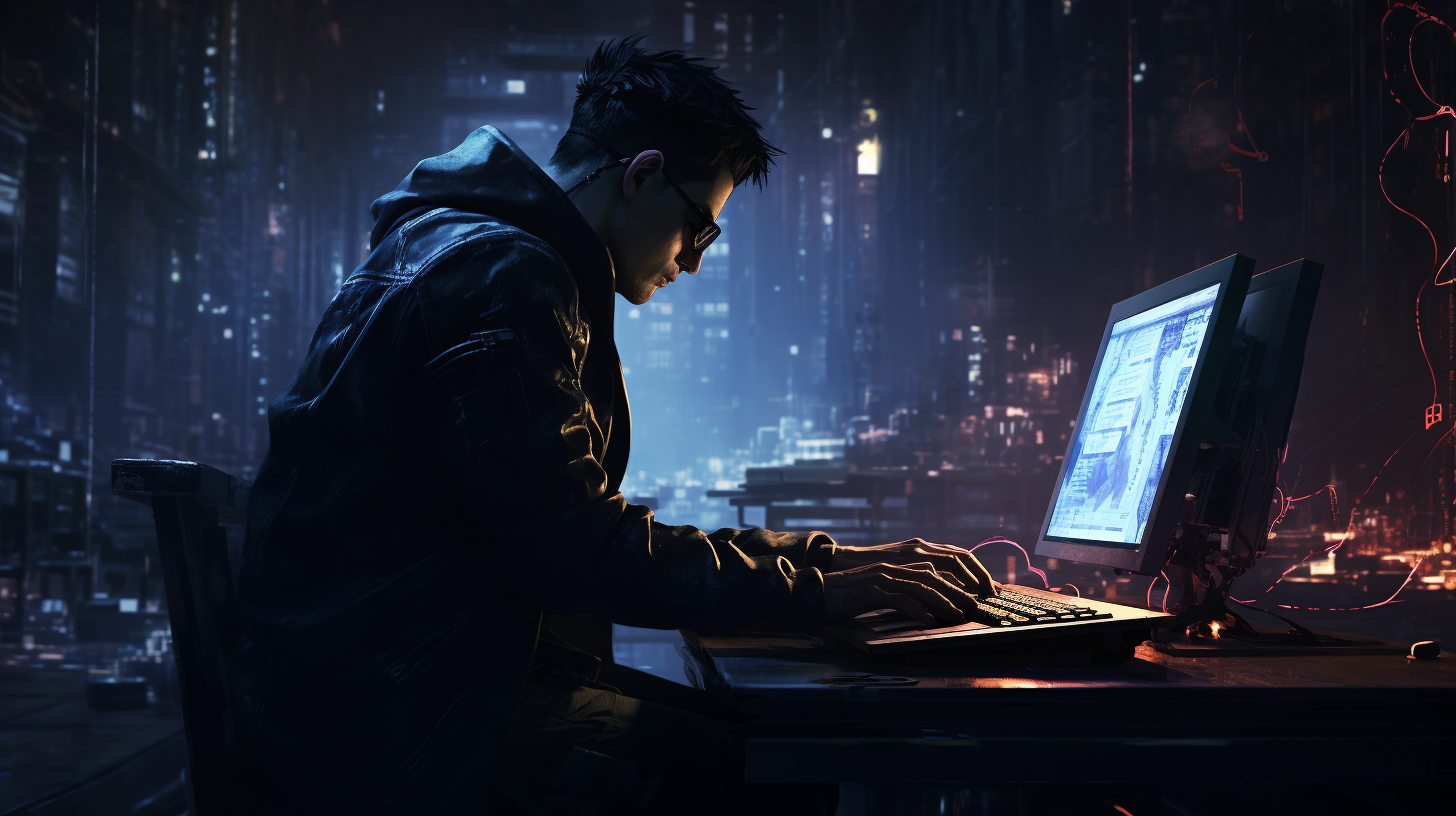
Java and RabbitMQ: Message Queuing
Message queuing is a fundamental concept in distributed systems that facilitates communication between different parts of an application through the use of messages. In Java, adopting a message queuing paradigm allows for the decoupling of application components, enhancing scalability and reliability. By using a message broker, such as RabbitMQ, Java applications can send and receive messages asynchronously, ensuring that the components interact smoothly without direct dependencies.
At its core, message queuing operates on the principle of sending a message from a producer to a consumer through a queue. The producer publishes messages to the queue, while the consumer retrieves messages from it. This separation allows producers and consumers to operate independently, which especially important in scenarios where application load fluctuates or where components may need to be updated or scaled independently.
The advantages of using message queues include:
- Producers and consumers do not need to interact in real-time, which can significantly enhance the throughput of the system.
- With multiple consumers processing messages from the same queue, the workload can be distributed effectively, preventing any one component from becoming a bottleneck.
- Messages can be persisted in the queue until they are processed, ensuring that no information is lost even if a consumer fails or is temporarily unavailable.
In Java, the integration of message queuing can be accomplished using several libraries and frameworks, with RabbitMQ being a popular choice due to its robust messaging capabilities. RabbitMQ implements the Advanced Message Queuing Protocol (AMQP), which defines a standard way for client applications to communicate with the broker. To effectively utilize RabbitMQ in Java applications, it’s essential to understand the fundamentals of message queuing in this context.
When working with message queues, you’ll typically deal with the following components:
- These are the buffers that hold messages until they’re processed by consumers.
- Exchanges route messages to one or more queues based on defined rules.
- These establish the relationship between exchanges and queues, determining how messages flow through the system.
Here’s a simple example demonstrating how to send a message to a queue in Java using RabbitMQ:
import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.Connection; import com.rabbitmq.client.Channel; public class Send { private final static String QUEUE_NAME = "hello"; public static void main(String[] argv) throws Exception { ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); try (Connection connection = factory.newConnection(); Channel channel = connection.createChannel()) { channel.queueDeclare(QUEUE_NAME, false, false, false, null); String message = "Hello World!"; channel.basicPublish("", QUEUE_NAME, null, message.getBytes("UTF-8")); System.out.println(" [x] Sent '" + message + "'"); } } }
In this code snippet, we establish a connection to the RabbitMQ server, declare a queue named “hello,” and then send a simple text message. The use of a try-with-resources statement ensures that the connection and channel are closed automatically after use, promoting resource management best practices.
Understanding message queuing in Java is not solely about the mechanics of sending and receiving messages; it also involves grasping the architecture of the system you are building. By effectively designing your message flow and using the features of RabbitMQ, you can create robust, fault-tolerant applications that respond dynamically to varying workloads.
Integrating RabbitMQ with Java Applications
Integrating RabbitMQ into your Java applications requires a few simpler steps, enabling you to harness the full power of message queuing. First, make sure to include the RabbitMQ Java client library in your project. If you’re using Maven, you can add the following dependency to your `pom.xml`:
com.rabbitmq amqp-client 5.15.0
With the library included, you can begin to implement the core components of RabbitMQ in your Java application. Below, we’ll walk through a more comprehensive example of both a producer and a consumer to show how these elements interact within a message queue system.
The producer is responsible for sending messages to a queue. Here is an example demonstrating a producer that sends messages in a loop:
import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.Connection; import com.rabbitmq.client.Channel; public class Send { private final static String QUEUE_NAME = "hello"; public static void main(String[] argv) throws Exception { ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); try (Connection connection = factory.newConnection(); Channel channel = connection.createChannel()) { channel.queueDeclare(QUEUE_NAME, false, false, false, null); for (int i = 0; i < 10; i++) { String message = "Hello World " + i + "!"; channel.basicPublish("", QUEUE_NAME, null, message.getBytes("UTF-8")); System.out.println(" [x] Sent '" + message + "'"); Thread.sleep(1000); // Delay to simulate work } } } }
This producer sends ten messages, pausing for one second between sends. The `queueDeclare` method ensures the queue exists before sending messages, preventing any runtime exceptions. Each message is uniquely identified with an incrementing number, enhancing traceability during testing.
On the consumer side, the implementation is equally simpler. The consumer will listen for messages on the specified queue and process them as they arrive. Here’s how you can set up a simple consumer:
import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.Connection; import com.rabbitmq.client.Channel; import com.rabbitmq.client.DeliverCallback; public class Recv { private final static String QUEUE_NAME = "hello"; public static void main(String[] argv) throws Exception { ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); try (Connection connection = factory.newConnection(); Channel channel = connection.createChannel()) { channel.queueDeclare(QUEUE_NAME, false, false, false, null); System.out.println(" [*] Waiting for messages. To exit press CTRL+C"); DeliverCallback deliverCallback = (consumerTag, delivery) -> { String message = new String(delivery.getBody(), "UTF-8"); System.out.println(" [x] Received '" + message + "'"); }; channel.basicConsume(QUEUE_NAME, true, deliverCallback, consumerTag -> { }); } } }
This consumer listens for messages on the “hello” queue. When a message is received, it prints the message to the console. The `basicConsume` method allows the consumer to continuously listen for incoming messages, effectively decoupling the message production from the processing.
By integrating RabbitMQ into your Java applications in this manner, you enable a robust messaging infrastructure that can handle varying loads and asynchronous processing challenges. The combination of producers and consumers working with queues serves as the backbone for scalable and resilient applications, so that you can focus on building functional features without worrying about the intricacies of communication mechanisms.
Best Practices for Effective Message Handling
When implementing message handling in Java applications using RabbitMQ, it’s essential to adhere to best practices that ensure efficiency, reliability, and maintainability. These practices not only improve performance but also reduce the likelihood of errors or message loss in production environments.
1. Acknowledge Messages Appropriately
In RabbitMQ, messages should be acknowledged once they have been successfully processed. This prevents message loss in case of consumer failure. Use manual acknowledgments to gain finer control over message processing. The following snippet shows how to set manual acknowledgments:
channel.basicConsume(QUEUE_NAME, false, deliverCallback, consumerTag -> { });
By setting the autoAck parameter to false, you can ensure that messages are acknowledged only after successful processing:
DeliverCallback deliverCallback = (consumerTag, delivery) -> { String message = new String(delivery.getBody(), "UTF-8"); try { // Process the message System.out.println(" [x] Received '" + message + "'"); // Acknowledge the message channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false); } catch (Exception e) { // Handle processing failure channel.basicNack(delivery.getEnvelope().getDeliveryTag(), false, true); } };
2. Implement Retry Logic
Messages can sometimes fail processing due to transient issues. Implementing retry logic to reprocess messages before moving them to a dead-letter queue (DLQ) is a common practice. By using a simple counter, you can limit the number of retries:
int retryCount = 0; while (retryCount < MAX_RETRIES) { try { // Process the message break; // Exit loop if processing is successful } catch (Exception e) { retryCount++; // Optionally add a delay Thread.sleep(RETRY_DELAY); } } if (retryCount == MAX_RETRIES) { // Move to a dead-letter queue channel.basicPublish("dead_letter_exchange", "dead_letter_routing_key", null, message.getBytes()); }
3. Monitor and Log
Effective logging and monitoring of message processing can provide insights into the health and performance of your system. Implement logging at various points in your consumer code to track message processing times, errors, and acknowledgments. Use tools like Prometheus or Grafana to visualize metrics over time.
4. Use Connection Pools
Creating a new connection to RabbitMQ for every message can be resource-intensive. Implement connection pooling to reuse connections efficiently. Libraries such as HikariCP can manage connection pools, allowing for better resource utilization:
// Configure HikariCP for RabbitMQ connections HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:your_database"); config.setUsername("user"); config.setPassword("password"); HikariDataSource dataSource = new HikariDataSource(config);
5. Design for Scalability
When designing your message processing architecture, always ponder scalability. Utilize multiple consumers to handle increased message loads. RabbitMQ supports multiple instances of consumers for the same queue, distributing the workload effectively. Additionally, ponder sharding your queues if your application expects a high volume of messages.
6. Use JSON or Protobuf for Structured Messages
When sending messages, using structured formats like JSON or Protocol Buffers (Protobuf) can enhance readability and maintainability. Ensure that both producers and consumers agree on the message format to avoid serialization issues. Here’s an example of sending a JSON message:
import org.json.JSONObject; JSONObject jsonMessage = new JSONObject(); jsonMessage.put("message", "Hello World!"); channel.basicPublish("", QUEUE_NAME, null, jsonMessage.toString().getBytes("UTF-8"));
By adhering to these best practices, your Java applications will be better equipped to handle message queuing effectively with RabbitMQ. This approach not only optimizes performance but also ensures that your applications are robust and resilient in the face of challenges.
Troubleshooting Common RabbitMQ Issues in Java
Troubleshooting issues with RabbitMQ in Java applications requires a systematic approach, as various factors can contribute to problems in message processing, delivery, or performance. Here are some common issues you might encounter and practical strategies for resolving them.
Connection Issues
One of the first hurdles in working with RabbitMQ is establishing a stable connection to the broker. If your application fails to connect, check the following:
- Ensure that the RabbitMQ server URL is correct. Verify that the hostname and port are accessible from the machine running your Java application.
- Confirm that any firewalls allow traffic on the RabbitMQ port (default is 5672).
- Check that the RabbitMQ server is running. You can use the RabbitMQ management plugin or command-line tools to verify its status.
Here’s a snippet that includes error handling for connection problems:
ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); try (Connection connection = factory.newConnection()) { // Connection successful } catch (IOException | TimeoutException e) { System.err.println("Failed to connect to RabbitMQ: " + e.getMessage()); }
Message Acknowledgment Issues
Failing to acknowledge messages can lead to messages being re-queued, causing duplicate processing. Ensure the acknowledgment logic is correctly implemented. If you encounter unexpected message redelivery, review your acknowledgment strategy:
- If set to true, messages are acknowledged immediately upon receipt, which can lead to unprocessed messages being lost if an exception occurs. Set it to false to acknowledge only after successful processing.
- Use manual acknowledgments to have greater control over when a message is acknowledged:
channel.basicConsume(QUEUE_NAME, false, deliverCallback, consumerTag -> { }); // In the DeliverCallback DeliverCallback deliverCallback = (consumerTag, delivery) -> { try { // Process the message System.out.println(" [x] Received '" + message + "'"); channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false); } catch (Exception e) { // Handle exception channel.basicNack(delivery.getEnvelope().getDeliveryTag(), false, true); } };
Message Format and Serialization Problems
Issues may arise if producers and consumers do not agree on the message format. This can lead to serialization errors or data loss. Make sure to:
- Use a consistent format such as JSON or Protobuf across your application.
- Implement error handling to catch serialization exceptions and log them for easier debugging.
Example of handling JSON serialization:
try { String message = jsonMessage.toString(); channel.basicPublish("", QUEUE_NAME, null, message.getBytes("UTF-8")); } catch (JSONException e) { System.err.println("Failed to serialize message: " + e.getMessage()); }
Performance Bottlenecks
As your application scales, you might notice performance issues. Use the following metrics to identify bottlenecks:
- Log processing times for each message to identify slow consumers.
- Monitor the number of unprocessed messages in the queue. A growing queue indicates that consumers are unable to keep up with the production rate.
Ponder implementing multiple consumers or optimizing the message processing logic if you notice bottlenecks:
ExecutorService executor = Executors.newFixedThreadPool(NUM_CONSUMERS); for (int i = 0; i { // Consumer logic here }); }
Dead Letter Exchanges
Messages that cannot be processed after several attempts should be directed to a Dead Letter Exchange (DLX). Configure DLXs to handle these scenarios gracefully:
- Define a DLX for the queue that specifies where to route messages that are rejected or expired.
- Use a TTL (Time-To-Live) setting on your messages to automatically route old messages to the DLX.
Setting up a DLX can be done as follows:
Map args = new HashMap(); args.put("x-dead-letter-exchange", "dlx_exchange"); channel.queueDeclare(QUEUE_NAME, false, false, false, args);
By systematically addressing these common issues and implementing robust error handling, connection management, and acknowledgment strategies, you can enhance the reliability and performance of your RabbitMQ-based Java applications. This approach not only resolves immediate problems but also sets a solid foundation for scalable, resilient systems capable of handling a dynamic workload.