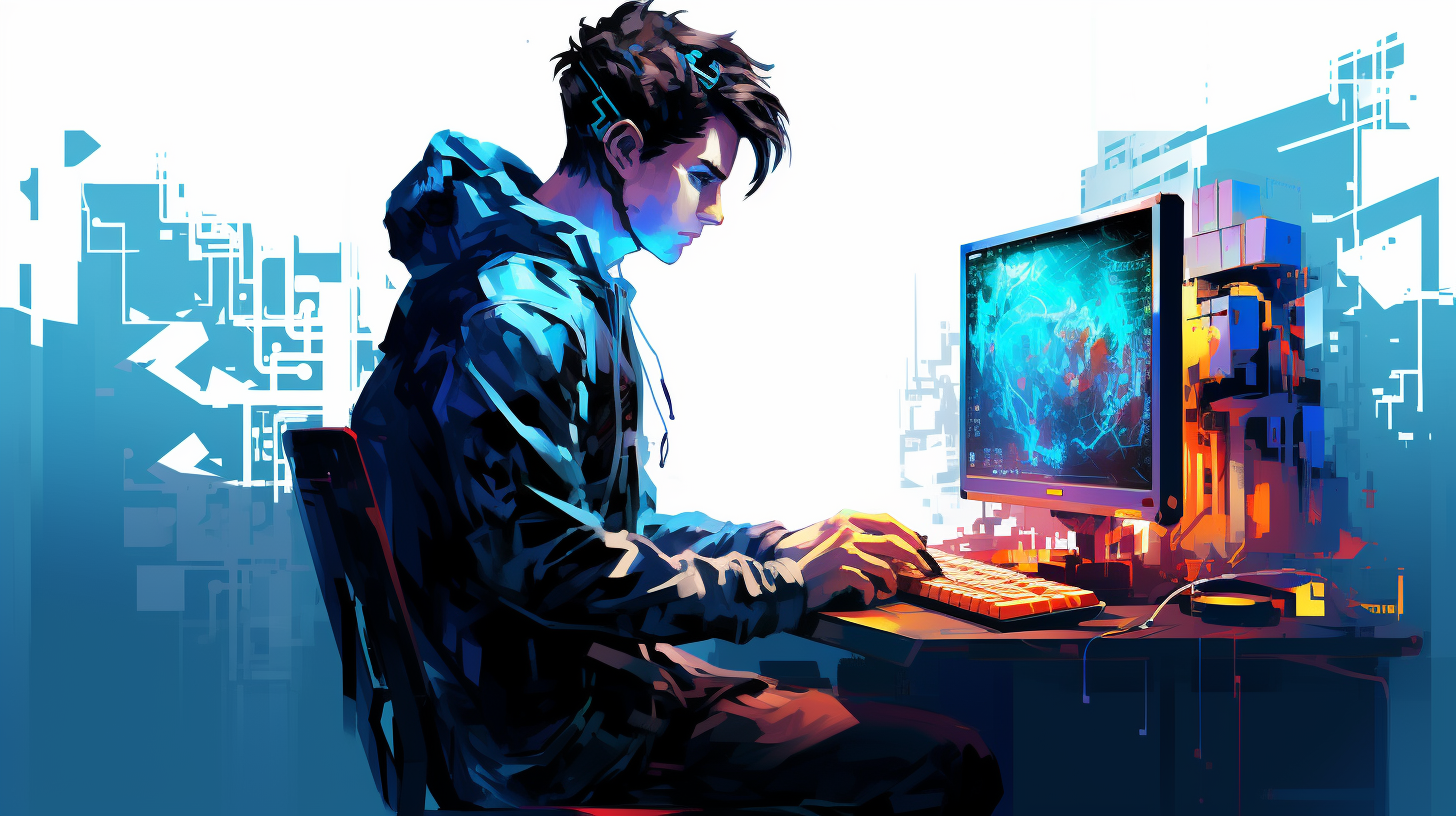
Bash Arithmetic Operations
Bash arithmetic is a powerful feature that allows you to perform mathematical calculations directly within your scripts. Understanding the basics of arithmetic in Bash can significantly enhance your ability to manipulate data and automate tasks. In Bash, arithmetic operations can be handled mainly through integer calculations, as Bash natively supports integer arithmetic. This means that if you need to work with decimals or floating-point numbers, you may need to rely on external tools like bc
or awk
.
To start performing arithmetic in Bash, one can utilize various arithmetic operators. The common operators include:
-
+
-
-
-
*
-
/
-
%
These operators can be used in different contexts, such as within command substitution or within arithmetic evaluation constructs. Here’s a simple example of using arithmetic in a script:
#!/bin/bash # Define two variables a=10 b=5 # Perform arithmetic operations sum=$((a + b)) difference=$((a - b)) product=$((a * b)) quotient=$((a / b)) modulus=$((a % b)) # Output the results echo "Sum: $sum" echo "Difference: $difference" echo "Product: $product" echo "Quotient: $quotient" echo "Modulus: $modulus"
When you run this script, it will perform the calculations and print the results. Bash evaluates the arithmetic expressions using the $(( ))
syntax which allows for clean and efficient mathematical operations.
It is essential to note that Bash does not handle floating-point arithmetic natively. If you require precision with decimal points, using either bc
or awk
becomes necessary. For instance, to calculate the division of two floating-point numbers, you might use:
#!/bin/bash # Define two floating-point numbers x=5.5 y=2.0 # Use bc for floating point division result=$(echo "scale=2; $x / $y" | bc) # Output the result echo "Result: $result"
The use of scale=2
defines the number of decimal places to be displayed, ensuring that the output is formatted to your specifications. This is the essence of arithmetic in Bash—understanding when to leverage its built-in capabilities and when to reach for external tools to achieve your desired precision.
Using Arithmetic Operators
Bash offers a versatile set of arithmetic operators that allow you to manipulate numbers and variables efficiently. When using these operators, it’s crucial to recognize how to properly format your expressions and understand the precedence of operations to avoid unexpected results.
The five primary arithmetic operators in Bash work as follows:
- Adds two numbers together.
- Subtracts the second number from the first.
- Multiplies two numbers.
- Divides the first number by the second, returning an integer value in integer division.
- Returns the remainder of the division of the first number by the second.
To illustrate the use of these operators in practice, think the following Bash script:
#!/bin/bash # Assign values to variables num1=12 num2=8 # Perform arithmetic operations add_result=$((num1 + num2)) sub_result=$((num1 - num2)) mul_result=$((num1 * num2)) div_result=$((num1 / num2)) mod_result=$((num1 % num2)) # Output the results echo "Addition: $add_result" echo "Subtraction: $sub_result" echo "Multiplication: $mul_result" echo "Division: $div_result" echo "Modulus: $mod_result"
When executed, this script will output the results of the arithmetic operations, clearly demonstrating the use of each operator.
Aside from basic arithmetic, Bash also allows for more complex expressions by combining operations. However, it’s vital to use parentheses to control the order of operations. For example:
#!/bin/bash # Define variables x=10 y=5 z=2 # Complex arithmetic expression result=$((x + y * z)) # Output the result echo "Result of 10 + 5 * 2: $result" # Outputs: 20
In the above example, the multiplication operation takes precedence over addition, leading to a result of 20. To change the order of operations, parentheses can be employed:
#!/bin/bash # Define variables x=10 y=5 z=2 # Adjusted complex arithmetic expression result=$(( (x + y) * z )) # Output the result echo "Result of (10 + 5) * 2: $result" # Outputs: 30
This adjustment alters the calculation substantially, showcasing the importance of understanding operator precedence in Bash arithmetic.
Using arithmetic operators in Bash is simpler, yet understanding their behavior, particularly in complex expressions, is essential for accurate calculations. With practice, you’ll find that these operators are invaluable tools in creating dynamic and responsive scripts.
Integer vs Floating Point Arithmetic
When diving deeper into arithmetic in Bash, one must confront the limitations inherent in its design, particularly when dealing with numerical types. While Bash shines in integer arithmetic, it lacks native support for floating-point operations, necessitating a strategy for handling decimal values. Understanding the implications of this very important, especially when transitioning from integer to floating-point arithmetic.
In Bash, all arithmetic operations performed using the built-in arithmetic evaluation features, such as $(( )) or let, are confined to integers. This means that calculations involving fractions or decimal points are inherently rounded down to the nearest whole number. For example, when you attempt to divide two integers, Bash will truncate the result:
#!/bin/bash # Define two integer variables a=7 b=2 # Perform division result=$((a / b)) # Output the result echo "Integer division of 7 by 2: $result" # Outputs: 3
In this case, even though mathematically, 7 divided by 2 equals 3.5, Bash will output only 3. This behavior is a defining characteristic of integer arithmetic and should be taken into account when designing scripts that involve calculations where precision is imperative.
To manage floating-point numbers, external tools must be employed. The most common utilities in this realm are bc
(an arbitrary precision calculator language) and awk
, both of which can handle decimal values effectively. Here’s how you can leverage bc
for floating-point arithmetic:
#!/bin/bash # Define two floating-point numbers x=7.0 y=2.0 # Use bc for floating-point division result=$(echo "scale=2; $x / $y" | bc) # Output the result echo "Floating-point division of 7.0 by 2.0: $result" # Outputs: 3.50
In this example, we use scale=2
to specify that we want the output to display two decimal places. This allows for more precise results, highlighting the flexibility provided by external tools for floating-point arithmetic.
Similarly, awk
can also be utilized for floating-point calculations, often with easier syntax for certain tasks:
#!/bin/bash # Define two floating-point numbers x=7.0 y=2.0 # Use awk for floating-point division result=$(awk "BEGIN {print $x/$y}") # Output the result echo "Floating-point division of 7.0 by 2.0 using awk: $result" # Outputs: 3.5
Ultimately, while Bash’s integer arithmetic capabilities are robust, the transition to floating-point calculations necessitates a shift in strategy. By understanding the native limitations and using external tools like bc
and awk
, you can effectively handle more complex mathematical operations while retaining precision in your Bash scripts. This dual approach ensures that you can harness the strengths of Bash without being hindered by its shortcomings in mathematical representation.
Arithmetic Evaluation with `(( ))`
Bash provides a powerful and concise way to evaluate arithmetic expressions using the $(( )) syntax. This method allows you to perform calculations directly within your scripts while providing readability and efficiency. The $(( )) construct evaluates the enclosed arithmetic expression and returns the result, all while being simpler to implement. To illustrate the use of arithmetic evaluation with $(( )), consider the following script: #!/bin/bash # Assign values to variables num1=15 num2=4 # Perform arithmetic evaluation result_add=$((num1 + num2)) result_sub=$((num1 - num2)) result_mul=$((num1 * num2)) result_div=$((num1 / num2)) result_mod=$((num1 % num2)) # Output the results echo "Addition: $result_add" echo "Subtraction: $result_sub" echo "Multiplication: $result_mul" echo "Division: $result_div" echo "Modulus: $result_mod"
When this script is executed, it demonstrates how efficiently Bash handles arithmetic evaluations, producing outputs that showcase the results of the different operations.
One of the significant advantages of using $(( )) is its ability to handle expressions involving multiple operations and parentheses, allowing for more complex calculations. For example:
#!/bin/bash # Define variables a=10 b=3 c=5 # Complex arithmetic expression result=$((a * b + c - b / 3)) # Output the result echo "Result of (10 * 3 + 5 - 3 / 3): $result" # Outputs: 30
In this example, the script accurately handles the order of operations, calculating the result of the expression correctly. Parentheses can be strategically placed to ensure that the desired precedence of operations is maintained, which especially important in scenarios involving more complicated formulas.
Another noteworthy feature of $(( )) is its support for bitwise operations, which can be advantageous for specific applications. Bash supports several bitwise operators, such as AND (&), OR (|), XOR (^), and NOT (~). Here’s how you can use them:
#!/bin/bash # Define two variables x=5 # In binary: 0101 y=3 # In binary: 0011 # Perform bitwise operations and_result=$((x & y)) # Bitwise AND or_result=$((x | y)) # Bitwise OR xor_result=$((x ^ y)) # Bitwise XOR not_result=$((~x)) # Bitwise NOT # Output the results echo "Bitwise AND: $and_result" echo "Bitwise OR: $or_result" echo "Bitwise XOR: $xor_result" echo "Bitwise NOT: $not_result"
This snippet demonstrates how bitwise operations can also be integrated into arithmetic evaluations, showcasing the flexibility of the $(( )) construct.
Lastly, it’s important to be aware of potential pitfalls when working with arithmetic evaluation in Bash. For instance, if you attempt to divide by zero, Bash will return an error. Therefore, adding checks to ensure that the denominator is not zero before performing division is a best practice:
#!/bin/bash # Define variables numerator=10 denominator=0 # Check for division by zero if [[ $denominator -ne 0 ]]; then result=$((numerator / denominator)) echo "Result: $result" else echo "Error: Division by zero is not allowed." fi
Incorporating these checks will make your scripts more robust and prevent runtime errors, enhancing the overall reliability of your calculations. The $(( )) evaluation construct in Bash is more than just a way to perform arithmetic; it is a foundational tool that, when understood and utilized correctly, can significantly streamline your scripting capabilities.
Command Substitution in Arithmetic
In Bash, command substitution provides a powerful way to incorporate the output of commands directly into arithmetic expressions. This feature allows you to execute a command and use its output as part of an arithmetic calculation, making your scripts dynamic and responsive to varying data inputs. The syntax for command substitution can be achieved using backticks (“ ` “) or the more state-of-the-art `$()` notation, with the latter being preferred for its clarity and versatility.
Let’s explore how command substitution can be effectively utilized in arithmetic operations by examining a few practical examples.
#!/bin/bash # Get the current date and time in seconds since epoch current_time=$(date +%s) # Define a variable for a past event (e.g., Unix epoch) past_event=0 # Calculate the difference in seconds time_difference=$((current_time - past_event)) # Output the result echo "Time since Unix epoch: $time_difference seconds"
In this example, we utilize `date +%s` to get the current time in seconds since the Unix epoch. By using command substitution, we can directly incorporate this value into our arithmetic calculation to find the time elapsed since a specified past event.
Command substitution can also be useful in situations where you need to calculate values based on the output of other commands. Ponder the following script that utilizes command substitution to capture the number of files in a directory and perform calculations based on that number:
#!/bin/bash # Count the number of files in the current directory file_count=$(ls -1 | wc -l) # Define a variable for the maximum allowed files max_files=100 # Calculate how many more files can be added remaining_files=$((max_files - file_count)) # Output the result echo "You can add $remaining_files more files."
Here, `ls -1 | wc -l` counts the number of files in the current directory. This value is then used in arithmetic to determine how many files can still be added, demonstrating the flexibility of command substitution in arithmetic evaluations.
Another important aspect is ensuring that the output of the command is suitable for arithmetic operations. For example, if you accidentally try to perform arithmetic on non-numeric output, you will encounter an error. Hence, it’s prudent to validate the output before using it in calculations.
#!/bin/bash # Get the disk usage of the root directory in human-readable format disk_usage=$(df / | tail -1 | awk '{print $5}' | sed 's/%//') # Check if the disk usage is numeric before performing arithmetic if [[ $disk_usage =~ ^[0-9]+$ ]]; then remaining_space=$((100 - disk_usage)) echo "Remaining disk space: $remaining_space%" else echo "Error: Disk usage is not a valid number." fi
In this script, we extract the disk usage percentage using a combination of `df`, `tail`, `awk`, and `sed`. Before performing the arithmetic operation, we use a regular expression to validate that the output is indeed a number, thus preventing any runtime errors.
Command substitution is an invaluable tool in Bash scripting, allowing for the integration of dynamic command output into arithmetic calculations. By using this feature, you can create more complex and responsive scripts that adapt to the state of the system or the results of previous commands. Understanding how to effectively implement command substitution will enhance your scripting prowess and enable you to tackle more sophisticated programming challenges within the Bash environment.
Best Practices for Arithmetic in Bash
When it comes to arithmetic in Bash, adhering to best practices can greatly affect the reliability, maintainability, and clarity of your scripts. Here are some key strategies to keep in mind while performing arithmetic operations in Bash:
1. Use $(( )) for Arithmetic Operations: The $(( )) syntax is the preferred method for arithmetic evaluation in Bash. It isn’t only concise but also supports a variety of arithmetic expressions, including addition, subtraction, multiplication, and more. For example:
#!/bin/bash # Simple arithmetic using $(( )) a=10 b=2 result=$((a * b)) echo "The product of $a and $b is: $result"
2. Validate Input Data: When your calculations depend on user input or command outputs, always validate the data type before performing any arithmetic. This helps prevent runtime errors and unexpected behaviors. For instance:
#!/bin/bash # Read user input read -p "Enter a number: " num # Validate that input is a number if [[ "$num" =~ ^[0-9]+$ ]]; then echo "You entered a valid number: $num" else echo "Error: Please enter a valid number." fi
3. Avoid Division by Zero: Always check for division by zero before performing division operations. Bash does not handle this gracefully and will produce an error. Implement checks to improve script robustness:
#!/bin/bash numerator=10 denominator=0 # Check for division by zero if [[ $denominator -ne 0 ]]; then result=$((numerator / denominator)) echo "Result: $result" else echo "Error: Division by zero is not allowed." fi
4. Use External Tools for Floating Point Arithmetic: Since Bash does not natively support floating-point arithmetic, leverage tools like bc
or awk
when needed. Here’s a typical example using bc
:
#!/bin/bash x=5.5 y=2.0 # Use bc for floating point division result=$(echo "scale=2; $x / $y" | bc) echo "Result of division: $result"
5. Use Meaningful Variable Names: Choose variable names that convey the purpose of the variable clearly. This practice improves readability and maintainability of your scripts. For example, instead of using x
or y
, use total_cost
or item_price
.
#!/bin/bash # Define meaningful variable names item_price=50 tax_rate=0.07 total_cost=$(echo "scale=2; $item_price * (1 + $tax_rate)" | bc) echo "Total cost including tax: $total_cost"
6. Comment Your Code: Don’t underestimate the power of comments. Adding clear comments on what each section of your code does can be invaluable, especially as the complexity of arithmetic operations increases.
#!/bin/bash # Calculate area of a circle radius=5 pi=3.14 area=$(echo "scale=2; $pi * $radius * $radius" | bc) echo "Area of the circle: $area"
7. Test Extensively: Always test your scripts with a variety of inputs, including edge cases. That’s especially important for arithmetic operations, as small errors can lead to significant issues in your calculations and outputs.
By adopting these best practices, you will ensure that your arithmetic operations in Bash are not only functional but also efficient and reliable. Well-structured and validated scripts can save you time and trouble down the road, allowing you to focus on solving the larger problems at hand.