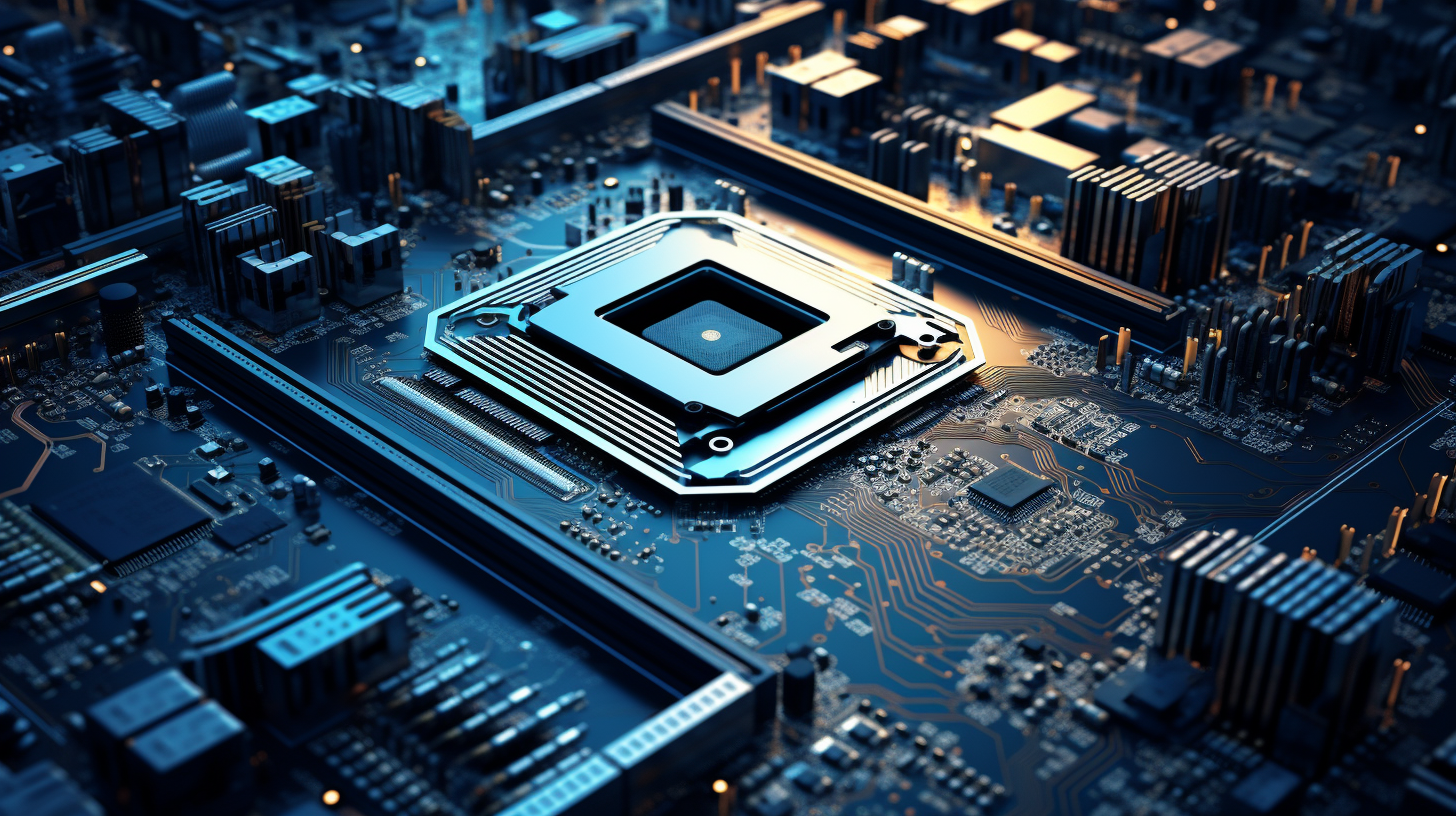
Writing Conditional Statements in JavaScript
Conditional statements are a cornerstone of programming in JavaScript, allowing developers to execute code based on certain conditions. Understanding how these statements function is important for controlling the flow of a program. At its core, a conditional statement evaluates a boolean expression, which can either be true or false.
JavaScript offers a variety of conditional statements, the most common being if, else, and switch. These constructs enable developers to direct the program’s execution path based on dynamic conditions. For instance, consider a simple scenario where you want to check a user’s age to determine access to specific content:
let age = 18; if (age >= 21) { console.log("You can enter the bar."); } else { console.log("You are not old enough to enter the bar."); }
In the example above, the if
statement checks whether the age
variable is greater than or equal to 21. If the condition is met, the first message is logged; otherwise, the second message is displayed. This simple mechanism forms the basis for more complex decision-making processes in your applications.
Conditional statements can also be nested to handle multiple layers of logic. This allows for a refined control structure, letting you manage various paths of execution based on a range of conditions. Here’s how you might handle age with more complexity:
let age = 16; if (age >= 21) { console.log("You can enter the bar."); } else if (age >= 18) { console.log("You can enter the club."); } else { console.log("You are not old enough for any venues."); }
In this example, additional else if
statements allow the program to evaluate multiple conditions sequentially, providing different outputs based on the user’s age. This flexibility is what makes conditional statements an essential tool in your JavaScript toolbox.
As you dive deeper into the JavaScript landscape, mastering these conditional statements will empower you to make your programs responsive and tailored to user inputs or application states. Whether you are developing a simple application or a complex solution, understanding how to harness the power of conditionals will significantly enhance your coding capabilities.
The If Statement: Basic Syntax and Usage
The if statement in JavaScript is the foundation for creating conditional logic. Its primary purpose is to execute a block of code only if a specified condition evaluates to true. The basic syntax is straightforward:
if (condition) { // code to execute if the condition is true }
In this snippet, condition is a boolean expression that the JavaScript engine evaluates. If the condition resolves to true, the code within the curly braces is executed. If it resolves to false, the code block is skipped. Exploring this structure further, we can look at an example that checks whether a user is logged in:
let isLoggedIn = true; if (isLoggedIn) { console.log("Welcome back, user!"); }
In this case, because isLoggedIn is set to true, the message “Welcome back, user!” is printed to the console. If you were to set isLoggedIn to false, that message would not appear, demonstrating how the if statement controls which code is executed based on the condition’s truthiness.
Furthermore, the code block within the if statement can contain multiple lines of code. This allows for complex operations to be performed when the condition is satisfied. Think the following example where we check for both user permissions and their login status:
let isAdmin = true; let isLoggedIn = true; if (isAdmin && isLoggedIn) { console.log("Access granted: Admin panel available."); // Additional admin-related code here }
Here, we use the logical AND operator (&&) to check both conditions simultaneously. The code inside the block will only execute if both isAdmin and isLoggedIn are true. This kind of logical combination enables more sophisticated decision-making within your applications.
It’s also worth noting how JavaScript handles various data types in conditional statements. JavaScript uses type coercion, meaning it will convert values to booleans when evaluating conditions. For instance, the following values are considered falsy in JavaScript:
- false
- 0
- “” (empty string)
- null
- undefined
- NaN
Any other value is considered truthy, including non-empty strings, non-zero numbers, and objects. This behavior can lead to some interesting shorthand notations in your code. For example:
let userName = "Alice"; if (userName) { console.log("Hello, " + userName + "!"); }
Here, even if userName were an empty string, the greeting would not be printed. Understanding these subtleties of the if statement and how JavaScript evaluates conditions will help you write more efficient and bug-free code.
The if statement is a fundamental tool in your programming arsenal, allowing you to control how your code behaves in various scenarios based on dynamic conditions. By mastering its syntax and behavior, you can create robust applications that respond intelligently to user inputs and application states.
Using Else and Else If for Multiple Conditions
When dealing with multiple conditions in JavaScript, the else and else if statements become invaluable. They allow for a more intricate decision-making structure by providing alternative paths based on different evaluations. That is particularly beneficial in scenarios where a single condition is insufficient to capture all possible outcomes.
The basic structure for using else and else if is as follows:
if (condition1) { // code to execute if condition1 is true } else if (condition2) { // code to execute if condition2 is true } else { // code to execute if both conditions are false }
In this format, the code evaluates condition1 first. If it’s true, the associated block executes, and the conditional statement completes. If not, JavaScript evaluates condition2. If that condition is true, the corresponding block runs. If neither condition is true, the code in the else block executes as a fallback.
Let’s illustrate this with a practical example that checks a student’s score and assigns a grade based on that score:
let score = 75; if (score >= 90) { console.log("Grade: A"); } else if (score >= 80) { console.log("Grade: B"); } else if (score >= 70) { console.log("Grade: C"); } else if (score >= 60) { console.log("Grade: D"); } else { console.log("Grade: F"); }
In this code snippet, the program checks the score variable against multiple conditions to determine the appropriate grade. The evaluation proceeds from the highest grade to the lowest, ensuring that only one grade is assigned based on the score value. If the score is 75, the output will be “Grade: C” since it meets the condition for that range but does not meet the higher conditions.
Using else if statements can lead to more readable and maintainable code than nesting multiple if statements. However, it is essential to organize your conditions logically to ensure that the most likely cases are checked first. This practice not only enhances performance by reducing the number of evaluations needed but also makes your code clearer.
Moreover, when using else if statements, it’s crucial to avoid overly complicated conditions that can confuse both you and anyone else reading your code later. Keeping conditions simple and simpler will lead to better understanding and easier debugging. For instance, if your conditions start to look unwieldy, it might be a sign that a switch statement or a lookup object could be more appropriate.
In summary, the else and else if constructs in JavaScript offer a powerful means of handling multiple conditions efficiently. By mastering their use, you can create cleaner, more effective control flows that respond aptly to a variety of scenarios, greatly improving the user experience and functionality of your applications.
Switch Statements: An Alternative to If-Else Chains
As your JavaScript applications grow in complexity, so does the need for efficient and organized control flow. That’s where the switch statement comes into play, serving as a cleaner alternative to the lengthy if-else chains we often encounter. The switch statement is particularly useful when dealing with multiple possible values of a single variable, so that you can branch your code based on these values without the clunky syntax that can come with chaining multiple if-else statements.
The basic syntax of the switch statement is as follows:
switch (expression) { case value1: // code to execute if expression matches value1 break; case value2: // code to execute if expression matches value2 break; // More cases... default: // code to execute if no case matches }
Here’s how it works: the switch statement evaluates the expression once and compares its value to each case. If a match is found, the corresponding block of code executes until a break statement is encountered. The break statement is important, as it prevents the execution from falling through to subsequent cases. If no cases match, the code in the default block runs, which is similar to the final else in an if-else chain.
To illustrate the switch statement in action, consider a scenario where we want to display different messages based on the day of the week:
let day = 3; // 1 for Sunday, 2 for Monday, etc. switch (day) { case 1: console.log("It's Sunday!"); break; case 2: console.log("It's Monday!"); break; case 3: console.log("It's Tuesday!"); break; case 4: console.log("It's Wednesday!"); break; case 5: console.log("It's Thursday!"); break; case 6: console.log("It's Friday!"); break; case 7: console.log("It's Saturday!"); break; default: console.log("Invalid day!"); }
In this example, if the value of day is 3, the output will be “It’s Tuesday!” The switch statement provides a simpler way to handle the various conditions based on the value of day, eliminating the need for a lengthy series of if-else statements.
One important aspect to note is that switch statements use strict comparison (===) when evaluating cases. This means that both the value and type must match. For example, if you were to switch on a string value and provide a number in your cases, no matches would be found:
let fruit = "apple"; switch (fruit) { case "banana": console.log("It's a banana!"); break; case "apple": console.log("It's an apple!"); break; default: console.log("Unknown fruit!"); }
In this case, because fruit is a string, matching it against string cases will yield the correct output: “It’s an apple!”
Another significant advantage of the switch statement is its ability to handle complex scenarios with multiple conditions easily. For example, if you need to evaluate a numerical variable and execute different code based on various ranges, using switch can lead to clearer and more maintainable code compared to a series of if-else statements.
However, it is worth mentioning that while switch statements can simplify your code, overusing them for simple comparisons might not always be ideal. For instance, if you only have a couple of conditions to check, using an if-else construct might still be more readable. As with all programming constructs, understanding the context and requirements of your application will guide you to choose the best tool for the job.
The switch statement is a powerful feature in JavaScript that can streamline your code when evaluating a single variable against multiple potential values. By employing switch statements, you can write cleaner, more organized code that enhances readability and maintainability, especially in scenarios involving numerous discrete conditions.
Best Practices for Writing Clear Conditional Logic
When it comes to writing clear conditional logic in JavaScript, best practices are key to maintaining readability and reducing the likelihood of bugs. As you craft your conditionals, think the following strategies to make your code more effective and easier to manage.
1. Keep It Simple
One of the most important principles in programming is to keep your code as simple as possible. Avoid overly complex conditions that can confuse both you and others who may read your code later. If you find yourself nesting multiple if statements or using complicated logical expressions, it may be time to refactor your code. For instance, ponder breaking down complex conditions into smaller, well-named functions that return boolean values.
function isAdult(age) { return age >= 18; } if (isAdult(userAge)) { console.log("User is an adult."); } else { console.log("User is not an adult."); }
This approach can make your logic clearer and allow you to reuse the function in different parts of your code.
2. Use Descriptive Variable Names
Descriptive variable names can provide context to your conditions, making them easier to understand. Instead of using generic names like a
or b
, use names that convey meaning, such as isUserLoggedIn
or hasPermissionToEdit
. This practice enhances the readability of your conditionals and reduces the cognitive load when someone else (or you in the future) reads the code.
let isUserLoggedIn = true; let hasPermissionToEdit = false; if (isUserLoggedIn && hasPermissionToEdit) { console.log("User has access to edit."); } else { console.log("User does not have access to edit."); }
3. Avoid Deep Nesting
Deeply nested conditionals can quickly become unwieldy and hard to read. To avoid this, ponder using early returns in functions, or refactor your logic into separate functions. This reduces the depth of nesting and makes the flow of the code more apparent.
function processUser(user) { if (!user) { return "No user found."; } if (!user.isLoggedIn) { return "User is not logged in."; } // Process user data return "User is logged in."; }
By returning early, you streamline your code and highlight the main logic flow more effectively.
4. Group Related Conditions
When you are checking multiple conditions, grouping related ones together can make your intent clearer. Use logical operators to consolidate conditions that are closely related. This can prevent repetitive code and increase maintainability.
let isAdmin = true; let isEditor = false; if (isAdmin || isEditor) { console.log("User has editing privileges."); } else { console.log("User does not have editing privileges."); }
In this example, combining the conditions into a single if statement clarifies that either role grants the same access.
5. Using Ternary Operators for Simple Conditions
For simple conditional logic that fits into a single line, think using the ternary operator. This can make your code more concise while still being readable. However, it’s essential to use this sparingly and only when the logic remains simpler.
let isOnline = true; let statusMessage = isOnline ? "User is online." : "User is offline."; console.log(statusMessage);
While the ternary operator can condense your code, overusing it for complex conditions defeats the purpose of clarity.
6. Comment Your Logic
When the logic behind your conditionals may not be immediately clear, use comments to explain your reasoning. This practice not only aids others in understanding your code but can also serve as a reminder for yourself when you revisit your code later.
let userAge = 20; // Check if user is eligible for adult content if (userAge >= 18) { console.log("User can access adult content."); } else { console.log("User cannot access adult content."); }
By incorporating comments, you make your intentions explicit, which is invaluable in collaborative environments or when maintaining legacy code.
By following these best practices, you can write clear, maintainable, and effective conditional logic in JavaScript. This not only enhances the quality of your code but also facilitates collaboration and understanding among developers and stakeholders alike.