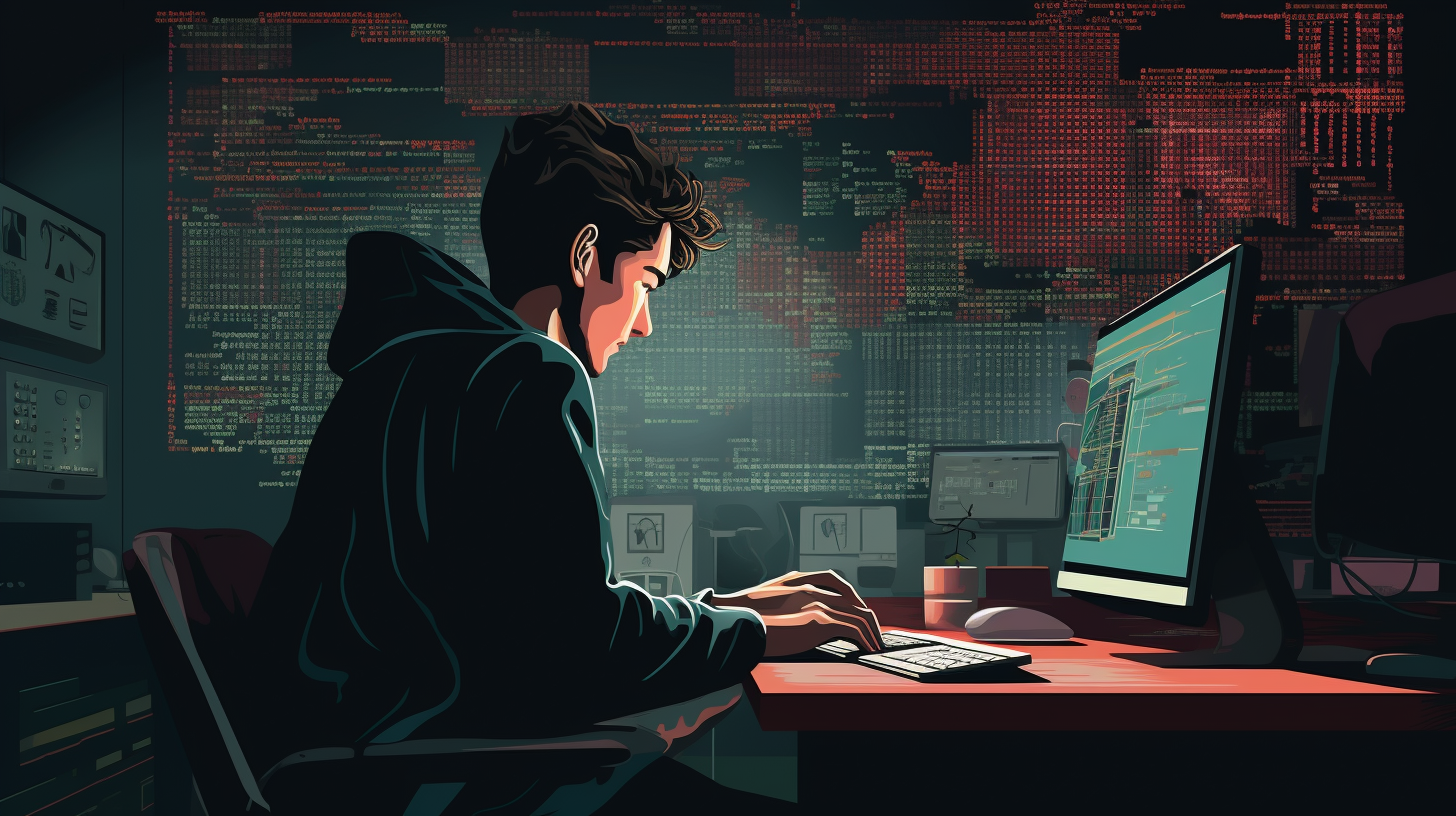
Implementing Data Caching in SQL
Data caching in SQL is a powerful technique designed to improve database performance by temporarily storing frequently accessed data in a faster, more accessible location. The primary goal of caching is to reduce the time it takes to access data repeatedly and minimize the load on the database server. By understanding how data caching works, you can leverage its benefits, making your SQL applications more efficient and responsive.
At its core, caching operates based on the principle of locality, which suggests that data that has been accessed recently is likely to be accessed again in the near future. This principle is exploited in various caching mechanisms, allowing for rapid retrieval of data without the overhead of making repeated database queries.
One common approach to caching in SQL involves the use of an in-memory data store. This can significantly speed up query performance, as data is retrieved from RAM rather than disk storage. Popular in-memory data stores like Redis and Memcached provide developers with tools to implement sophisticated caching strategies.
When discussing caching, differentiating between the types of data is essential. The data you choose to cache should be based on access patterns, size, and volatility. For instance, static data that rarely changes, such as configuration settings or reference tables, is an excellent candidate for caching. On the other hand, highly dynamic data, like user transactions or real-time analytics, may not benefit as much from caching due to its ever-changing nature.
Here’s a basic SQL example illustrating how to implement caching for a frequently accessed query result:
SELECT * FROM users WHERE last_login > NOW() - INTERVAL '7 days';
By caching the results of this query, subsequent requests for recently logged-in users can be served directly from the cache instead of hitting the database each time, leading to substantial performance improvements.
Understanding the lifecycle of cached data is also crucial. Data can be cached on various levels: at the application level, within the database, or through middleware. Each level has its trade-offs in terms of complexity, performance, and consistency. The choice of caching strategy must align with the application’s requirements and the expected workload to ensure optimal performance.
Finally, it is essential to recognize that caching is not a silver bullet. While it can dramatically enhance performance, it introduces complexity, such as cache invalidation and consistency challenges that must be managed effectively. By building a strong foundation in data caching principles, you position yourself to implement more advanced caching strategies and respond adeptly to the challenges that arise in SQL application development.
Types of Caching Mechanisms
The types of caching mechanisms available to SQL developers can be categorized primarily into three main approaches: in-memory caching, disk caching, and application-level caching. Each mechanism has its unique advantages, performance characteristics, and use cases. Understanding these differences especially important in selecting the right caching strategy for your specific SQL application needs.
In-Memory Caching: This mechanism stores data in the system’s RAM, providing the fastest possible access times. In-memory caches like Redis and Memcached are designed to handle high-throughput requests and deliver low-latency responses. Given their speed, they are perfect for scenarios where quick data retrieval is critical, such as serving frequent read queries or storing the results of complex calculations that do not change often.
-- Example of fetching cached data from Redis GET recently_logged_in_users
In this example, the query result from Redis can be retrieved instantly, minimizing database load and speeding up response times. However, the challenge lies in managing cache expiration and ensuring that stale data doesn’t mislead the application.
Disk Caching: Unlike in-memory caching, which relies on RAM, disk caching writes cached data to a local disk or a distributed file system. This method is slower than in-memory caching but allows for larger data storage. It’s beneficial in scenarios where the data volume exceeds memory capabilities or when data persistence is required beyond application runtime.
-- Example of disk caching using a SQL view CREATE VIEW cached_recent_logins AS SELECT * FROM users WHERE last_login > NOW() - INTERVAL '7 days';
By using a SQL view, the results of a heavy query can be stored and accessed quickly, though it may not deliver the same performance as an in-memory solution. The trade-off is often between speed and storage capacity.
Application-Level Caching: This approach involves caching data at the application layer, which can be achieved through programming techniques or frameworks. In application-level caching, developers can store frequently used data objects or query results in memory, allowing for immediate access without needing to query the database each time.
-- Example of application-level caching in a hypothetical function function getRecentLogins() { if (cache.exists('recent_logins')) { return cache.get('recent_logins'); } else { $result = executeQuery("SELECT * FROM users WHERE last_login > NOW() - INTERVAL '7 days'"); cache.set('recent_logins', $result, 3600); // Cache for 1 hour return $result; } }
This method allows for fine-grained control over caching logic, but it can also increase application complexity, especially concerning cache invalidation and management strategies.
Moreover, a hybrid approach, combining various caching techniques, can yield optimal performance. For instance, using in-memory caching for the most frequently accessed data while maintaining a disk cache for less frequently used information can balance speed and resource usage effectively.
Choosing the right caching mechanism requires careful consideration of the application’s specific needs, data access patterns, and overall architecture. By understanding these types of caching mechanisms, developers can make informed decisions that enhance application performance and user experience.
Implementing Caching Strategies
When it comes to implementing caching strategies in SQL, methodical planning and execution are essential. The effectiveness of a caching strategy hinges not only on the choice of caching mechanism but also on its configuration, management, and alignment with the specific requirements of your application. To create a robust caching strategy, ponder the following key components:
1. Cache Design
Begin by defining what data you will cache and how it will be accessed. Identify the most frequently accessed queries and the data sets that are less frequently modified. For example, if your application often queries user profiles and these profiles do not change often, caching this data can lead to significant performance gains.
SELECT * FROM user_profiles WHERE active = true;
By caching the result of the above query, you can provide faster access to active user profiles, reducing the load on your SQL database.
2. Cache Population Strategy
Decide how cache data will be populated. You can pre-load the cache during application startup or use lazy loading, where the cache is populated on-demand based on requests. The latter method can help avoid unnecessary memory usage but may introduce latency during the first access. Here’s a simple example of lazy loading in pseudo code:
function getActiveUserProfiles() { if (cache.exists('active_user_profiles')) { return cache.get('active_user_profiles'); } else { $result = executeQuery("SELECT * FROM user_profiles WHERE active = true"); cache.set('active_user_profiles', $result, 3600); // Cache for 1 hour return $result; } }
3. Cache Expiration
Determine a strategy for cache expiration to ensure that stale data does not mislead your application. Implementing time-based expiration is a common practice, where cached data is invalidated after a specified duration. Additionally, ponder event-based expiration, which can be employed to clear the cache whenever the underlying data changes, ensuring that users are always served the most current information.
CREATE TRIGGER invalidate_cache AFTER UPDATE ON user_profiles FOR EACH ROW BEGIN DELETE FROM cache WHERE key = 'active_user_profiles'; END;
This SQL trigger will automatically invalidate the cached user profiles whenever an update occurs, thus maintaining cache consistency.
4. Cache Hit and Miss Strategy
Establish a monitoring mechanism to track cache hits and misses. This will help you understand the effectiveness of your caching strategy and guide future optimizations. You can log cache usage data to analyze patterns, which may inform decisions about which queries to cache or adjust cache expiration settings.
INSERT INTO cache_logs (cache_key, hit_miss, timestamp) VALUES ('active_user_profiles', 'hit', NOW());
Tracking this information provides valuable insights into how often cached data is accessed and how much it’s relied upon compared to direct database queries.
5. Testing and Optimization
Once your caching strategy is implemented, conduct thorough testing to evaluate its performance. Measure the response times for cached and non-cached queries, and use profiling tools to analyze the load on your database. Based on these insights, you may need to refine your caching strategy, adjusting what gets cached, its expiration policies, or even the caching layer used.
Each aspect of your caching strategy should be tailored to fit your application’s unique requirements and usage patterns. By thoughtfully implementing these strategies, you can significantly enhance performance, improve user experience, and reduce the strain on your SQL database.
Best Practices for Data Caching
When it comes to implementing best practices for data caching in SQL, a systematic approach can significantly enhance your caching strategy’s effectiveness. Here are some critical considerations to keep in mind:
1. Cache the Right Data
Not all data is suitable for caching. Focus on caching data that’s frequently accessed and less likely to change. For instance, ponder data that’s read-heavy but not updated often, such as reference data or user preferences. Caching such data can lead to substantial performance improvements without the downsides of stale data affecting the application.
SELECT * FROM product_catalog WHERE category = 'electronics';
By caching the results of this query, you can enhance access speed for product information that users frequently request while reducing load on the underlying database.
2. Implement Cache Hierarchy
A hierarchical caching strategy can improve efficiency. Utilize multiple layers of caching, such as an in-memory cache for high-frequency queries, a disk-based cache for moderately accessed data, and a database query cache for less frequent calls. This approach ensures that the most accessed data is served quickest while maintaining a broader cache for other data.
CREATE VIEW cached_electronics AS SELECT * FROM product_catalog WHERE category = 'electronics';
This SQL view can serve as a secondary cache, providing a fallback for less frequently accessed queries.
3. Use Cache Invalidation Wisely
Cache invalidation is one of the biggest challenges in caching. To maintain consistency, employ strategies that proactively manage cache updates. Time-based expiration is common but might not be sufficient for highly dynamic data. Consider implementing event-driven cache invalidation whenever underlying data changes. This ensures that your cache reflects the most up-to-date information.
CREATE TRIGGER update_product_cache AFTER UPDATE ON product_catalog FOR EACH ROW BEGIN DELETE FROM cache WHERE key = 'product_catalog'; END;
This trigger will clear any cached entries related to this product catalog whenever an update occurs, thus preventing stale reads.
4. Monitor Cache Performance
Regularly monitor cache performance metrics such as hit rates, miss rates, and response times. This data provides insights into the effectiveness of your caching strategy. High miss rates could indicate that the wrong data is being cached or that your cache size is insufficient. Use logging to track these metrics effectively.
INSERT INTO cache_metrics (cache_key, hits, misses, timestamp) VALUES ('product_catalog', 100, 10, NOW());
Tracking these metrics allows you to adjust caching policies dynamically based on real usage patterns.
5. Keep It Simple
A complex caching strategy can lead to increased maintenance overhead and potential bugs. Aim for simplicity in your caching layer. Start with basic caching strategies and evolve them as necessary. Optimize based on observed performance rather than theoretical improvements, and always validate changes through rigorous testing.
6. Plan for Cache Bloat
As your application scales, so will your cache. Monitor your cache size and implement a purging strategy that removes old or unused cache entries. This prevents cache bloat and ensures that your cache remains efficient and relevant.
DELETE FROM cache WHERE last_accessed < NOW() - INTERVAL '1 week';
This SQL command can help clean up stale entries from your cache, ensuring that it only retains active data.
By adhering to these best practices, you can create a more robust and efficient caching strategy in your SQL applications. This approach not only improves application performance but also enhances the overall user experience by providing faster data access with minimal latency.
Monitoring and Managing Cached Data
Monitoring and managing cached data effectively is important for maintaining the performance and reliability of your SQL applications. As your application grows, so does the complexity of the caching layer, necessitating a structured approach to ensure that your cache remains efficient, consistent, and relevant. Here are key strategies to think when monitoring and managing cached data.
1. Implement Cache Monitoring Tools
Employing specialized monitoring tools can provide deep insights into your cache’s performance. Many caching solutions come with built-in monitoring capabilities that track metrics such as cache hit rates, eviction rates, and latency. For example, Redis offers the Redis Monitor command, which can help you observe cache performance in real-time:
MONITOR
This command outputs all commands processed by the server, enabling you to analyze usage patterns and identify potential bottlenecks. It is essential to regularly review these metrics to inform adjustments in your caching strategy.
2. Cache Hit and Miss Analysis
Understanding the ratio of cache hits to misses is vital for evaluating the effectiveness of your caching strategy. A high cache hit rate indicates that the caching layer is functioning as intended, while a high miss rate might suggest that data is not being cached effectively. You can log cache hits and misses using a simple SQL procedure:
INSERT INTO cache_usage (cache_key, hit, timestamp) VALUES ('active_user_profiles', 1, NOW());
This logging allows you to analyze trends over time and adjust which queries or data you choose to cache based on their performance.
3. Refreshing Cached Data
Stale data can lead to incorrect application behavior. Implementing a strategy for refreshing cached data is essential. Time-based expiration is one approach, but event-driven invalidation can be more efficient in many cases. For instance, using database triggers can automatically invalidate cached data when it changes:
CREATE TRIGGER invalidate_cache AFTER UPDATE ON user_profiles FOR EACH ROW BEGIN DELETE FROM cache WHERE key = 'active_user_profiles'; END;
This trigger ensures that any update to the user profiles table will result in the corresponding cache entry being invalidated, thus maintaining data integrity.
4. Analyze Cache Performance Regularly
Perform periodic reviews of your cache usage and performance metrics. This analysis allows you to adapt your caching strategies based on changing access patterns. You may find that certain data is accessed more frequently than anticipated or that other data does not warrant caching at all. Analyzing cache performance can involve joining cache metrics with application logs:
SELECT cache_key, COUNT(*) AS access_count FROM cache_usage GROUP BY cache_key ORDER BY access_count DESC;
This query provides insight into which cached items are the most accessed, helping you prioritize cache optimizations.
5. Set Up Alerts for Cache Issues
Establishing alerts for specific cache-related metrics can help you respond to potential issues before they affect your application. For example, if the cache hit rate drops below a certain threshold, triggering an alert can allow for immediate investigation. Monitoring solutions like Prometheus can be integrated with your cache system to set these alerts:
ALERT cache_hit_rate_low IF cache.hit_rate < 0.75 FOR 5 minutes
This proactive approach ensures that you can maintain the health of your caching layer and make necessary adjustments in a timely manner.
6. Review Cache Configuration Settings
Regularly revisiting your cache configuration settings is equally important. The size of your cache, eviction policies, and expiration strategies should align with your application’s performance requirements and data access patterns. Depending on usage, you might switch between Least Recently Used (LRU) or Time-to-Live (TTL) policies to optimize performance:
SET maxmemory-policy volatile-lru;
This command sets Redis to evict keys based on the LRU algorithm when it reaches its memory limit, balancing performance with available resources.
By systematically monitoring and managing cached data, you can ensure that your caching layer is not only enhancing performance but also maintaining the integrity and reliability of your SQL applications.
Common Challenges and Solutions in Caching
When implementing caching in SQL, it is essential to be aware of the common challenges that may arise and how to address them effectively. Caching, while offering substantial performance benefits, introduces its own set of complexities that developers and database administrators must navigate.
One of the primary challenges in caching is the issue of cache coherence. This occurs when the data in the cache becomes stale due to changes in the underlying database. For instance, if a record is updated in the database but the corresponding cached entry is not invalidated, subsequent reads from the cache will return outdated information. To mitigate this issue, it especially important to implement robust cache invalidation strategies. Using triggers, as shown in the following SQL example, can help ensure that cache entries are updated or removed when the underlying data changes:
CREATE TRIGGER invalidate_cache AFTER UPDATE ON user_profiles FOR EACH ROW BEGIN DELETE FROM cache WHERE key = 'active_user_profiles'; END;
Another challenge comes from the complexity of managing cache size. As applications scale and data volumes grow, the cache may become bloated with outdated or less frequently accessed data. This can lead to inefficient memory usage and degraded performance. Regularly monitoring cache size and implementing eviction policies, such as Least Recently Used (LRU), can help address this problem. For example, in Redis, you can set the eviction policy with:
CONFIG SET maxmemory-policy allkeys-lru
Performance metrics are vital in identifying and resolving caching issues. A high cache miss rate can indicate that the data being requested is not present in the cache, leading to unnecessary database queries. It’s essential to track hit and miss rates to adjust your caching strategy accordingly. You can log cache usage with:
INSERT INTO cache_usage (cache_key, hit, timestamp) VALUES ('active_user_profiles', 1, NOW());
This allows you to analyze how effectively your cache is serving requests. If certain queries consistently result in cache misses, it may be time to reconsider which data is being cached.
Moreover, developers often face challenges related to the complexity of caching logic. Implementing caching at multiple levels (database, application, etc.) can lead to confusion and increased maintenance overhead. To counter this, it’s beneficial to maintain a simpler caching layer with well-defined boundaries and responsibilities. Focus on caching the most frequently accessed data first, and gradually expand your caching strategy as needed.
Lastly, testing and debugging caching mechanisms can be particularly challenging. Caches can mask underlying issues in application logic or database performance, making it difficult to identify the root cause of performance problems. Implementing thorough logging and monitoring practices can help provide visibility into cache operations and support troubleshooting efforts. For instance, logging cache hits and misses allows for a clearer understanding of cache performance, which helps in diagnosing potential issues:
SELECT cache_key, COUNT(*) AS access_count FROM cache_usage GROUP BY cache_key ORDER BY access_count DESC;
By addressing these common challenges with proactive strategies and careful monitoring, you can optimize your caching implementation and ensure that it serves as a reliable component of your SQL application architecture. Emphasizing cache coherence, size management, performance tracking, simplicity, and robust testing will empower developers to harness the full potential of caching while minimizing its pitfalls.