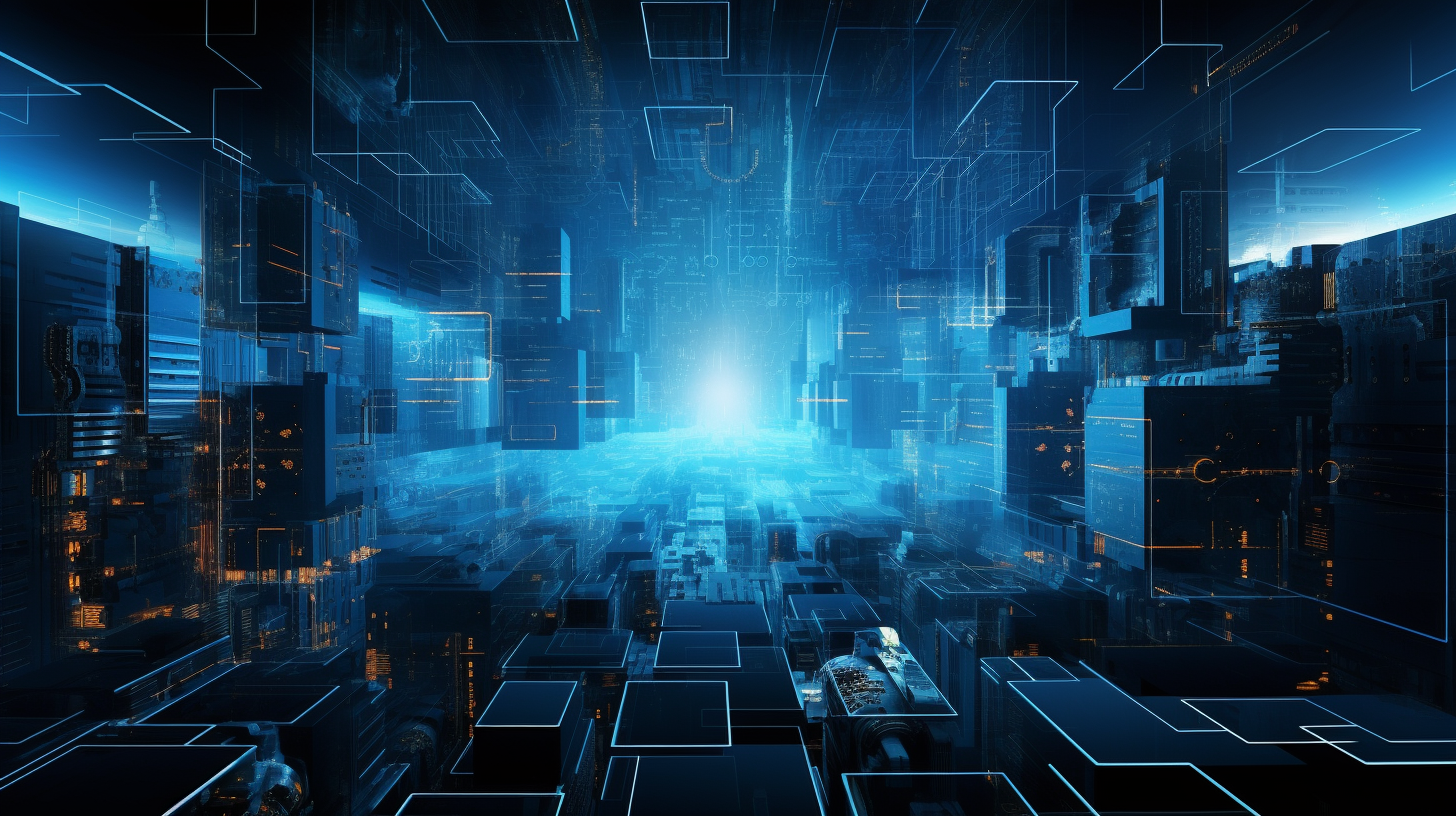
Integrating Bash with Docker
The Docker Command-Line Interface (CLI) is a powerful tool that allows developers to interact with the Docker engine and manage containers, images, networks, and volumes effectively. Understanding the CLI is essential for integrating Bash scripts into your Docker workflow.
At its core, the Docker CLI provides a set of commands that can be executed in the terminal. Each command typically follows the pattern:
docker [OPTIONS] COMMAND [ARG...]
where OPTIONS are optional flags that modify the command’s behavior, COMMAND is the specific action to execute, and ARG… represents any additional arguments required by the command.
Key commands you should know include:
- This command creates and starts a new container from a specified image. You can pass various options to customize the container’s environment.
- Lists all running containers. The -a flag can be added to see all containers, including those that are stopped.
- Displays all images stored on your local machine.
- This command allows you to run a command inside a running container, which can be particularly useful for debugging or interacting with the application.
- Removes one or more stopped containers, helping you manage your resources efficiently.
- Deletes specified images from your local repository, clearing up disk space.
When working with Bash scripts, you’ll often invoke these commands dynamically. For instance, to run a container while passing environment variables, you might use a script like the following:
#!/bin/bash # Define variables for the image and container name IMAGE_NAME="my_app_image" CONTAINER_NAME="my_app_container" # Run the Docker container with environment variables docker run -d --name $CONTAINER_NAME -e "ENV_VAR=value" $IMAGE_NAME
In the example above, we define the IMAGE_NAME and CONTAINER_NAME variables, making it easier to update the script later. The -d flag runs the container in detached mode, letting it run in the background.
Another essential aspect of the Docker CLI is managing container logs. You can view logs for a specific container with the following command:
docker logs my_app_container
This command is invaluable for troubleshooting issues within your containers.
Understanding how to utilize the Docker CLI effectively not only enhances your productivity but also allows seamless integration with Bash scripts, making automation and orchestration of your containerized applications more manageable.
Creating Docker Containers with Bash Scripts
Creating Docker containers using Bash scripts provides a powerful means to automate your development and deployment processes. This approach allows for consistency, reproducibility, and a reduction in manual errors, particularly in complex environments where multiple containers are used.
To begin, you’ll want to establish a basic structure for your Bash script that defines the necessary parameters for your Docker containers. This includes specifying the Docker image to use, any required environment variables, port mappings, and volume mounts. Below is an example of a Bash script that creates a Docker container with these considerations:
#!/bin/bash # Variables for the Docker image and container IMAGE_NAME="my_app_image" CONTAINER_NAME="my_app_container" HOST_PORT=8080 CONTAINER_PORT=80 VOLUME_PATH="/path/on/host:/path/in/container" # Check if the container is already running if [ "$(docker ps -q -f name=${CONTAINER_NAME})" ]; then echo "Container ${CONTAINER_NAME} is already running." else # Run the Docker container docker run -d --name ${CONTAINER_NAME} -p ${HOST_PORT}:${CONTAINER_PORT} -v ${VOLUME_PATH} ${IMAGE_NAME} echo "Container ${CONTAINER_NAME} has been started." fi
In this script, we start by defining the necessary variables for our Docker configuration. We then check if a container with the specified name is already running to prevent redundant instances. If it’s not running, we invoke the docker run
command with the appropriate flags:
- Runs the container in detached mode.
- Maps a port on the host to a port in the container.
- Mounts a volume to persist data across container restarts.
Additionally, you may want to incorporate error handling into your scripts to ensure that the creation and management of your containers are resilient. Here’s an updated example that includes error handling:
#!/bin/bash # Variables for the Docker image and container IMAGE_NAME="my_app_image" CONTAINER_NAME="my_app_container" # Function to check if Docker is running function check_docker { if ! systemctl is-active --quiet docker; then echo "Docker is not running. Please start Docker and try again." exit 1 fi } # Check if Docker is running check_docker # Create and start the container if [ "$(docker ps -q -f name=${CONTAINER_NAME})" ]; then echo "Container ${CONTAINER_NAME} is already running." else docker run -d --name ${CONTAINER_NAME} ${IMAGE_NAME} || { echo "Failed to start container ${CONTAINER_NAME}." exit 1 } echo "Container ${CONTAINER_NAME} has been successfully started." }
This version of the script includes a function to check if the Docker service is active. If Docker is not running, it outputs an error message and exits the script. Additionally, we capture the exit status of the docker run
command to ensure that any issues starting the container are reported back to the user.
By structuring your Bash scripts in this way, you create a robust framework for managing your Docker containers efficiently. This not only streamlines your workflow but also promotes best practices in container management.
Automating Docker Workflows using Bash
Automating Docker workflows using Bash scripts can substantially enhance your development processes by which will allow you to run complex commands, handle multiple containers, and integrate Docker operations into larger scripts. By using Bash’s scripting capabilities, you can create powerful automation solutions that minimize manual intervention and reduce the likelihood of human error.
One of the most effective ways to automate your Docker workflows is by creating scripts that can build, run, and manage your containers seamlessly. For example, you can write a script to build a Docker image from a Dockerfile and immediately run a container based on that image. Consider the following Bash script:
#!/bin/bash # Variables for the image and container IMAGE_NAME="my_app_image" CONTAINER_NAME="my_app_container" # Build the Docker image docker build -t ${IMAGE_NAME} . || { echo "Failed to build the Docker image." exit 1 } # Run the Docker container docker run -d --name ${CONTAINER_NAME} ${IMAGE_NAME} || { echo "Failed to start the container." exit 1 } echo "Docker image ${IMAGE_NAME} built and container ${CONTAINER_NAME} started successfully."
In this script, we start by defining the image and container names. The docker build
command is executed to create the image from the current directory’s Dockerfile. If the build fails, an error message is printed, and the script exits. When the image has been successfully built, we run a container with the docker run
command, again capturing any failure for reporting.
Another key aspect of automating Docker workflows is managing multiple containers. If your application consists of several services running in different containers, you can orchestrate their startup and configuration using Bash scripts. Here’s an example that demonstrates how to start multiple containers:
#!/bin/bash # Define an array of container names declare -a CONTAINERS=("service1" "service2" "service3") # Loop through the container names and start each one for CONTAINER in "${CONTAINERS[@]}"; do docker run -d --name ${CONTAINER} my_app_image || { echo "Failed to start container ${CONTAINER}." exit 1 } echo "Started container ${CONTAINER}." done
In this script, we define an array containing the names of the containers we want to start. The for
loop iterates over each container name, attempting to run it using the specified image. This method allows for quick and efficient deployment of multiple services, ensuring that each container is started in the correct order and that any failure is handled gracefully.
Additionally, you can schedule your scripts to run at specific intervals or in response to certain events using cron jobs. This integration can be invaluable for tasks such as automatic backups of containers or regularly polling container statuses, as shown below:
# Check the status of a running container CONTAINER_NAME="my_app_container" if [ "$(docker ps -q -f name=${CONTAINER_NAME})" ]; then echo "Container ${CONTAINER_NAME} is running." else echo "Container ${CONTAINER_NAME} is not running. Attempting to restart..." docker start ${CONTAINER_NAME} || { echo "Failed to restart ${CONTAINER_NAME}." exit 1 } echo "Container ${CONTAINER_NAME} has been restarted." }
This script checks whether a specific container is running and attempts to restart it if it isn’t. By automating such monitoring tasks, you can ensure that your applications remain available and maintain optimal performance over time.
By combining the power of Bash scripting with Docker, you can significantly streamline your development workflow. The ability to automate container management not only saves time but also enhances consistency and reliability across your applications. As you become more familiar with Bash’s capabilities and Docker’s CLI, your scripts can evolve to encompass more complex deployments and integrations, paving the way for efficient DevOps practices.
Best Practices for Bash and Docker Integration
Integrating Bash with Docker requires adherence to certain best practices that enhance the reliability, maintainability, and efficiency of your scripts. These practices help mitigate common pitfalls associated with container management and streamline your workflow.
1. Use Descriptive Variable Names: In your Bash scripts, always opt for descriptive variable names that clearly express their purpose. This makes your code more readable and easier to maintain. For example:
CONTAINER_NAME="my_app_container" IMAGE_NAME="my_app_image"
By naming your variables intuitively, you provide context for anyone who may read your script later, including your future self.
2. Handle Errors Gracefully: Implementing error handling in your scripts is important. Use conditional statements to check the success of each Docker command. You can leverage the special variable $?
to capture the exit status of commands. Here is an example:
docker run -d --name ${CONTAINER_NAME} ${IMAGE_NAME} if [ $? -ne 0 ]; then echo "Error: Failed to start ${CONTAINER_NAME}." exit 1 fi
This way, if something goes wrong, your script can report the error and exit cleanly rather than continuing with potentially undesired results.
3. Clean Up Resources: It’s essential to clean up stopped containers and unused images to prevent unnecessary resource consumption. You can incorporate commands like docker rm
and docker rmi
in your scripts:
# Remove stopped containers docker container prune -f # Remove unused images docker image prune -f
By automating resource cleanup, you ensure that your environment remains tidy and efficient, reducing the chance of conflicts or resource shortages.
4. Use Docker Compose for Complex Applications: For applications with multiple services, think using Docker Compose instead of managing each container individually with Bash scripts. Docker Compose allows you to define multi-container applications using a simple YAML file. However, if you choose to stick with Bash, ensure you script the orchestration of container dependencies appropriately.
5. Document Your Scripts: Always document your Bash scripts, especially if they interact with Docker. Use comments liberally to explain the purpose of complex commands or sections of your script. This practice not only aids in understanding but also assists others who may modify your code in the future:
# This script sets up the application container docker run -d --name ${CONTAINER_NAME} ${IMAGE_NAME}
Documentation is key to maintaining a robust environment, as it minimizes the time spent deciphering the functionality of your scripts.
6. Keep Scripts Modular: Whenever possible, make your scripts modular. Split them into functions that encapsulate specific tasks. This approach enhances code reusability and makes debugging easier. For example:
function start_container { docker run -d --name ${CONTAINER_NAME} ${IMAGE_NAME} } function stop_container { docker stop ${CONTAINER_NAME} } # Start the container start_container
With this structure, you can call functions as needed, and updating a particular function will propagate changes throughout your script.
7. Test Scripts in a Safe Environment: Before deploying your scripts in a production environment, ensure that they are thoroughly tested in a safe, isolated environment. This practice helps you identify and rectify potential issues without affecting your live applications.
By following these best practices, you can foster a more efficient and reliable integration of Bash scripting with Docker. These guidelines not only improve your current workflows but also lay a solid foundation for scaling your container management processes in the future.