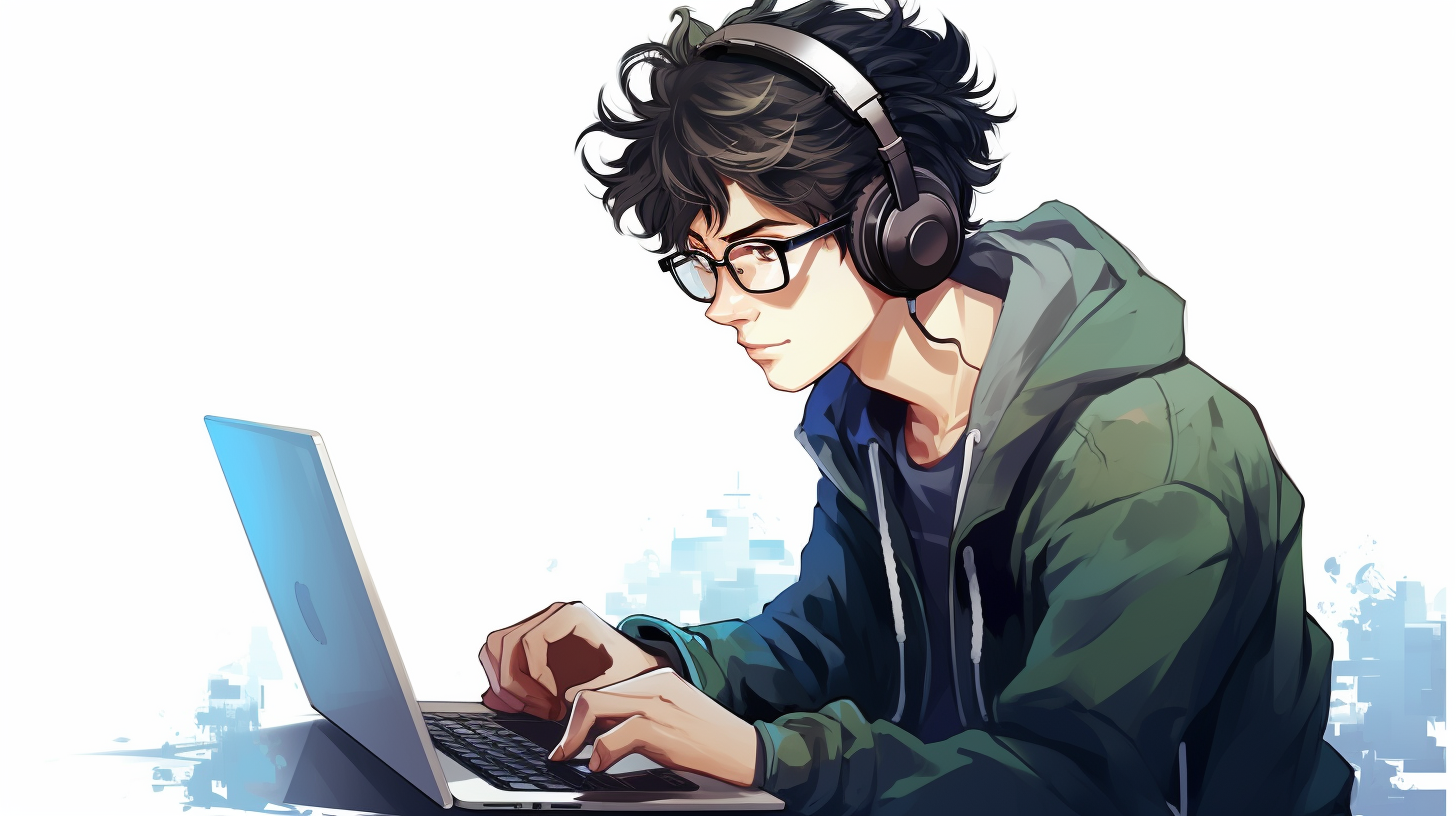
Advanced Python: Decorators, Context Managers, and Metaclasses
In Python, decorators are a powerful and expressive tool that allows you to modify the behavior of functions or methods. A decorator is essentially a callable (like a function) that takes another function as an argument and extends or alters its functionality without permanently modifying it. This feature can lead to cleaner and more readable code, enhancing the overall design of your applications.
To understand decorators, it is helpful to break down their mechanics. At its core, a decorator wraps a function, so that you can execute code before and after the wrapped function runs. This wrapping process can be visualized as follows:
def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper
In the example above, my_decorator
is a simple decorator that prints messages before and after the execution of the function it wraps. To use this decorator, you can apply it to a function using the @ syntax:
@my_decorator def say_hello(): print("Hello!") say_hello()
When you call say_hello()
, the output will be:
Something is happening before the function is called. Hello! Something is happening after the function is called.
This illustrates how decorators can encapsulate behavior and enhance the functionality of your functions without altering their core logic. They are particularly useful for cross-cutting concerns such as logging, authentication, and performance monitoring. For instance, a logging decorator might look like this:
def log_function_call(func): def wrapper(*args, **kwargs): print(f"Calling function {func.__name__} with arguments {args} and {kwargs}") return func(*args, **kwargs) return wrapper @log_function_call def add(a, b): return a + b add(2, 3)
In this case, every time the add
function is called, it logs its execution details, enabling easier debugging and monitoring.
Nested decorators can also be employed, where you can stack multiple decorators together to enrich a function with multiple behaviors. Consider the following:
@my_decorator @log_function_call def multiply(a, b): return a * b multiply(3, 4)
Here, the multiply
function will first log the call and then execute the wrapped behavior defined in my_decorator
.
Mastering Context Managers
Context managers are a core feature of Python that provide a convenient way to manage resources, ensuring that they are properly acquired and released. They are particularly useful for managing file operations and network connections, where you want to ensure that resources are cleaned up after use, regardless of whether an error occurred during the operation. The most common way to utilize a context manager is through the with
statement, which simplifies exception handling and resource management within a block of code.
The essence of a context manager is that it defines two essential methods: __enter__()
and __exit__()
. When the with
block is entered, __enter__()
is executed, and when the block is exited, __exit__()
is executed. This guarantees that resources are properly cleaned up after use, even if an exception is raised in the block.
Let’s think a simple example where we open a file using a context manager:
with open('example.txt', 'w') as file: file.write('Hello, World!')
In this example, the open
function returns a file object that acts as a context manager. The file is automatically closed after the block is exited, even if an error occurs while writing to it.
To create custom context managers, you can define a class that implements the __enter__()
and __exit__()
methods. Here’s a simple implementation of a context manager that measures the execution time of a code block:
import time class Timer: def __enter__(self): self.start = time.time() return self def __exit__(self, exc_type, exc_value, traceback): self.end = time.time() self.interval = self.end - self.start print(f'Time taken: {self.interval:.2f} seconds') with Timer() as timer: sum(range(1000000))
When this code runs, it will output the time taken to execute the code block within the context manager, giving you valuable insights into performance.
Python also provides a more concise way to create context managers using the contextlib
module, specifically the contextlib.contextmanager
decorator. This allows you to create a generator function that can yield control back to the block of code within the with
statement. Here’s how you can implement a simple context manager using this approach:
from contextlib import contextmanager @contextmanager def sample_context(): print('Entering the context') yield print('Exiting the context') with sample_context(): print('Inside the context')
In this example, when you enter the context, it prints a message indicating entry, and upon exiting, it prints another message. The yield
statement is where the code block within the with
statement runs.
Exploring Metaclasses
Metaclasses in Python are one of the most powerful features that often remain in the shadows, overshadowed by more common elements like classes and decorators. At a high level, a metaclass is a class of a class that defines how a class behaves. Just like classes define the behavior of the objects created from them, metaclasses specify the behavior of classes themselves. This allows for customization and control over class creation and modification, making it a valuable tool for advanced Python developers.
To understand metaclasses, you first need to grasp how classes are formed. When you define a class, Python uses a metaclass to create it. By default, this metaclass is type. However, you can define your own metaclass by inheriting from type and overriding its methods. The primary methods you might override include __new__ and __init__.
The __new__ method is responsible for creating the class, while __init__ is for initializing it. Here’s a simple example of a custom metaclass that modifies class creation:
class UppercaseMeta(type): def __new__(cls, name, bases, attrs): uppercase_attrs = {key.upper(): value for key, value in attrs.items()} return super().__new__(cls, name, bases, uppercase_attrs) class MyClass(metaclass=UppercaseMeta): greeting = 'Hello, World!' print(MyClass.GREETING) # Outputs: Hello, World!
In this example, UppercaseMeta transforms all attribute names of the class MyClass to uppercase when the class is created. This demonstrates how metaclasses can dynamically alter the structure of a class, providing an extra layer of flexibility.
Metaclasses can also be leveraged for enforcing coding standards or automating the addition of methods and properties. Consider the following example, where we use a metaclass to automatically add a __str__ method to any class defined with it:
class AutoStrMeta(type): def __new__(cls, name, bases, attrs): attrs['__str__'] = lambda self: f'{self.__class__.__name__} instance' return super().__new__(cls, name, bases, attrs) class MyClass(metaclass=AutoStrMeta): pass obj = MyClass() print(obj) # Outputs: MyClass instance
In this case, every class created using AutoStrMeta will have a default string representation that indicates its class name. This illustrates how metaclasses can streamline class design by enforcing certain patterns without requiring repetitive code.
Beyond these examples, metaclasses can be used for various advanced techniques such as class registration, validation, and even modifying method behavior at the class level. While they can add complexity to your code, when used judiciously, they can significantly enhance your ability to create adaptable and reusable components.
Practical Applications and Use Cases
Practical applications of decorators, context managers, and metaclasses are abundant in Python, and understanding these use cases can greatly improve your programming skills. Let’s delve into some scenarios where these concepts shine.
Starting with decorators, they are often employed in scenarios such as logging, access control, and caching. Think a case where you want to implement a caching mechanism for a function this is computationally expensive. This can be achieved elegantly with a decorator:
def cache(func): memo = {} def wrapper(*args): if args in memo: return memo[args] result = func(*args) memo[args] = result return result return wrapper @cache def fibonacci(n): if n < 2: return n return fibonacci(n-1) + fibonacci(n-2) print(fibonacci(10)) # Outputs: 55
In this example, the cache decorator stores the results of the fibonacci function, so subsequent calls with the same arguments return the cached result, significantly improving performance.
Moving to context managers, they come into play in resource management tasks like handling database connections, file operations, or network sockets. Here’s a practical example using a context manager to handle database connections:
import sqlite3 from contextlib import contextmanager @contextmanager def get_db_connection(db_name): conn = sqlite3.connect(db_name) try: yield conn finally: conn.close() with get_db_connection('example.db') as connection: cursor = connection.cursor() cursor.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)') cursor.execute('INSERT INTO users (name) VALUES (?)', ('Alice',)) connection.commit()
In this scenario, the get_db_connection context manager ensures that the database connection is closed properly after the operations are completed, even if an exception occurs. This pattern keeps your code clean and helps prevent resource leaks.
Metaclasses, while less common in daily work, find their place in scenarios where class behavior needs to be altered dynamically. One practical use case is for creating singletons, ensuring that only one instance of a class is created:
class SingletonMeta(type):
_instances = {}