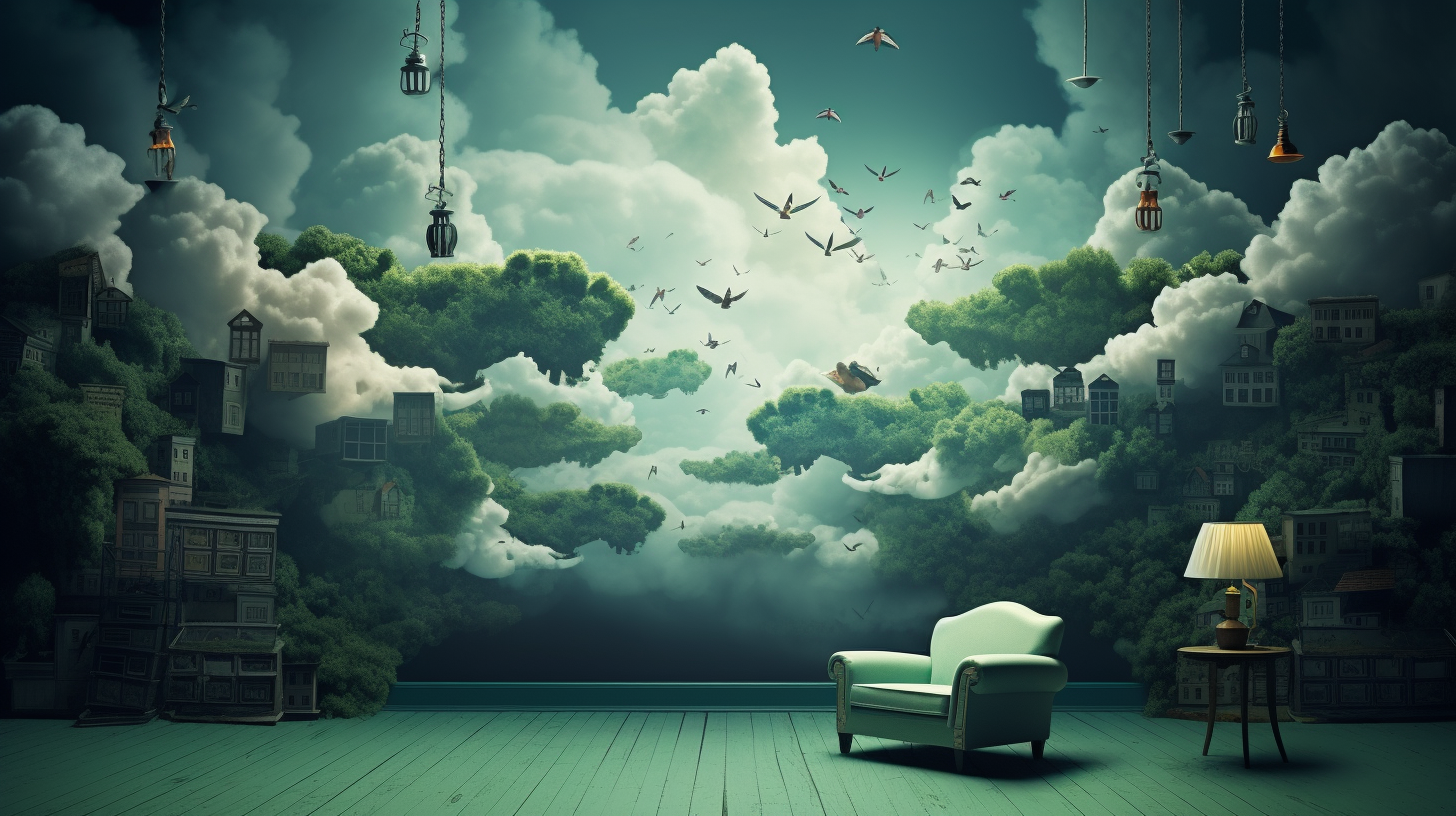
Command Line Arguments in Bash
In Bash, command line arguments are a powerful way to pass data to a script at runtime. When you execute a script, you can provide various inputs directly from the command line, which can then be accessed and manipulated within the script. This feature enables your scripts to be more dynamic and versatile, allowing them to respond to user inputs effectively.
Command line arguments are indexed and can be accessed using certain predefined variables. The first argument after the script name is referred to as $1, the second as $2, and so on, up to a maximum of $9 for the first nine arguments. The variable $0 holds the name of the script itself. This indexing allows for simpler access to the provided parameters, rendering it effortless to handle user input.
For instance, consider a simple script that takes two numbers from the command line and adds them together:
#!/bin/bash # A simple script to add two numbers result=$(( $1 + $2 )) echo "The sum of $1 and $2 is: $result"
When you run this script, you could invoke it as follows:
./add_numbers.sh 5 10
This would output:
The sum of 5 and 10 is: 15
In addition to the positional parameters, you also have access to the total number of arguments passed to your script through the special variable $#
. This can be invaluable when you need to validate the number of inputs before proceeding with further operations.
For example:
#!/bin/bash # A script to check the number of arguments if [ $# -ne 2 ]; then echo "Please provide exactly two arguments." exit 1 fi echo "You provided the correct number of arguments: $1 and $2"
In this case, before the script performs any calculations, it ensures that exactly two arguments are provided. If not, it prompts the user with a message and exits gracefully. This type of input validation is an important aspect of working with command line arguments in Bash.
Accessing Command Line Arguments
Accessing command line arguments in Bash is not just about fetching inputs; it is about making those inputs workable and applicable within your scripts. The positional parameters ($1, $2, …, $9) serve as gateways to user-supplied data, allowing scripts to interact dynamically based on user input. This means that as you build more complex scripts, the ability to manipulate these parameters effectively can greatly enhance functionality and user experience.
One particularly useful feature in Bash is the ability to handle an arbitrary number of arguments using the special variable $@
or $*
. While both contain all the positional parameters, they behave differently when quoted. $@
treats each argument as a separate entity, while $*
treats all arguments as a single string. This distinction can be critical depending on how you intend to process the arguments.
#!/bin/bash # A script to demonstrate the difference between $@ and $* echo "Using $@:" for arg in "$@"; do echo "$arg" done echo "Using $*:" for arg in "$*"; do echo "$arg" done
When executed with multiple arguments, such as:
./my_script.sh arg1 arg2 arg3
The output will illustrate how $@
separates each argument, while $*
concatenates them into a single line. This distinction can influence how you parse or process inputs, especially when arguments may include spaces or need to be handled as distinct items.
Another common task is to loop through all provided arguments, which can be easily achieved with a simple loop structure:
#!/bin/bash # Looping through all command line arguments echo "You passed the following arguments:" for arg in "$@"; do echo "$arg" done
In this script, every argument provided at runtime is printed line by line, showcasing how to iterate over the arguments flexibly. This technique is particularly useful in scenarios where the number of arguments is not fixed or known beforehand.
Moreover, you can also access specific arguments in a more dynamic way using array-like indexing. For example, to access the last argument without knowing its position, you can use ${!#}
, which refers to the last positional parameter:
#!/bin/bash # Accessing the last argument echo "The last argument is: ${!#}"
Navigating command line arguments in Bash is a multifaceted skill that allows scripts to adapt and respond based on user input. Using positional parameters, special variables, and looping constructs transforms static scripts into robust, interactive tools capable of handling a wide range of use cases.
Using Special Parameters
In addition to positional parameters and their inherent functionality, Bash provides a set of special parameters that can enhance your script’s ability to handle command line arguments in a more sophisticated manner. These special parameters include variables like `$#`, `$@`, `$*`, and others, each serving a distinct purpose that can significantly influence how your scripts behave with user-provided inputs.
One of the most critical special parameters is `$#`, which holds the count of the total number of arguments passed to your script. That is particularly useful for validation purposes, ensuring that the correct number of inputs is provided before proceeding with any logic. For instance, a script designed to require three arguments can leverage `$#` to enforce this requirement:
#!/bin/bash # A script that requires exactly three arguments if [ $# -ne 3 ]; then echo "Error: You must provide exactly three arguments." exit 1 fi echo "You've successfully provided three arguments: $1, $2, and $3"
When run with fewer or more than three arguments, the script will gracefully notify the user of the requirement, allowing for a more controlled execution flow.
Another essential special parameter is `$@`, which contains all the positional parameters passed to the script. When used within double quotes, it expands to each parameter as a separate quoted string, making it perfect for scenarios where arguments might contain spaces. This feature can be utilized in scripts that need to process or pass along these arguments to other commands or functions:
#!/bin/bash # A script that echoes all arguments separately echo "Arguments received:" for arg in "$@"; do echo "Argument: $arg" done
On the other hand, `$*` behaves similarly but treats all parameters as a single word when quoted. This can lead to different outcomes based on how you intend to handle the arguments, especially when the arguments include spaces:
#!/bin/bash # A script to demonstrate usage of $* echo "All arguments as a single string:" echo "$*"
Understanding when to use `$@` versus `$*` can prevent subtle bugs in your scripts and ensure that arguments are processed correctly.
Furthermore, to access specific arguments more dynamically, Bash provides the flexibility to use indirect expansion. For example, if you need to reference the nth argument without hardcoding its position, you can use notation like `${!n}` where `n` is the index. This can be particularly useful in scripts that loop through a range of arguments or need to access the last argument dynamically:
#!/bin/bash # A script to access the last argument using indirect expansion echo "The last argument is: ${!#}"
This flexibility allows for more dynamic script behavior, as users can provide a varying number of arguments with ease.
Using these special parameters effectively can greatly enhance your ability to interact with command line arguments, allowing for more robust and effortless to handle Bash scripts. By integrating checks, loops, and proper parameter handling into your script design, you can create tools that not only execute commands but also intelligently respond to user input, giving you the power to build truly dynamic shell applications.
Best Practices for Handling Command Line Arguments
When working with command line arguments in Bash, employing best practices can significantly enhance the reliability, maintainability, and user-friendliness of your scripts. By carefully managing how inputs are received and processed, you can prevent common pitfalls and create scripts that behave predictably, even in edge cases.
One of the first best practices is to validate the number of arguments before proceeding with any other logic. This ensures that your script has the necessary data to execute correctly and helps avoid runtime errors. As previously demonstrated, using the special variable $#
allows you to check the count of arguments efficiently:
#!/bin/bash # Validate number of arguments if [ $# -lt 2 ]; then echo "Error: At least two arguments are required." exit 1 fi echo "Processing arguments: $1 and $2"
In this example, if fewer than two arguments are provided, the script exits early with an error message, preventing any further processing. That’s especially useful in larger scripts where subsequent code relies on the presence of expected inputs.
Another important aspect of handling command line arguments is sanitizing the input. Depending on the data being processed, you may need to ensure that user inputs do not introduce unexpected behavior or security risks. For instance, if your script modifies system files or executes commands based on user inputs, validating that these inputs conform to expected formats is crucial:
#!/bin/bash # Example of input sanitization if [[ ! $1 =~ ^[a-zA-Z0-9_]+$ ]]; then echo "Error: Invalid input. Only alphanumeric characters and underscores are allowed." exit 1 fi echo "Input is valid: $1"
This snippet checks whether the first argument consists solely of alphanumeric characters and underscores. If it doesn’t, the script informs the user and exits. Such checks can help avoid injection vulnerabilities and other unintended consequences.
It is also a good practice to provide informative usage messages to your users. Documenting how to use your script and what arguments are expected can greatly improve the user experience. You can implement a simple help function that displays usage instructions when invoked with a specific flag, such as -h
or --help
:
#!/bin/bash # Help function for usage instructions function show_help { echo "Usage: $0 [options] arg1 arg2" echo "Options:" echo " -h, --help Show this help message and exit" echo "Example: $0 5 10" } if [[ $1 == "-h" || $1 == "--help" ]]; then show_help exit 0 fi
This method allows users to quickly access help information, facilitating a better understanding of how to interact with your script.
Moreover, consider using meaningful variable names and comments throughout your script. Clear naming conventions and well-placed comments make it easier for others (and yourself) to read and maintain the code in the future. For example:
#!/bin/bash # A script that calculates the sum of two numbers # Check if correct number of arguments is provided if [ $# -ne 2 ]; then echo "Error: Please provide exactly two numbers." exit 1 fi # Assign arguments to descriptive variable names first_number=$1 second_number=$2 # Calculate and display the sum sum=$((first_number + second_number)) echo "The sum of $first_number and $second_number is: $sum"
This approach not only enhances readability but also aids in debugging and future modifications.
Finally, be mindful of exit statuses in your scripts. Use exit codes to indicate success or failure, allowing users and other scripts to handle outcomes appropriately. A successful execution typically returns 0
, while any error conditions should return a non-zero value:
#!/bin/bash # Example script demonstrating exit statuses if [ $# -lt 1 ]; then echo "Error: At least one argument is required." exit 1 fi echo "Argument provided: $1" exit 0
By implementing these best practices—validating and sanitizing inputs, providing usage instructions, using clear variable names and comments, and managing exit statuses—you can craft Bash scripts that are robust, uncomplicated to manage, and maintainable. These practices not only mitigate potential issues but also enhance the overall functionality and user experience of your scripts.