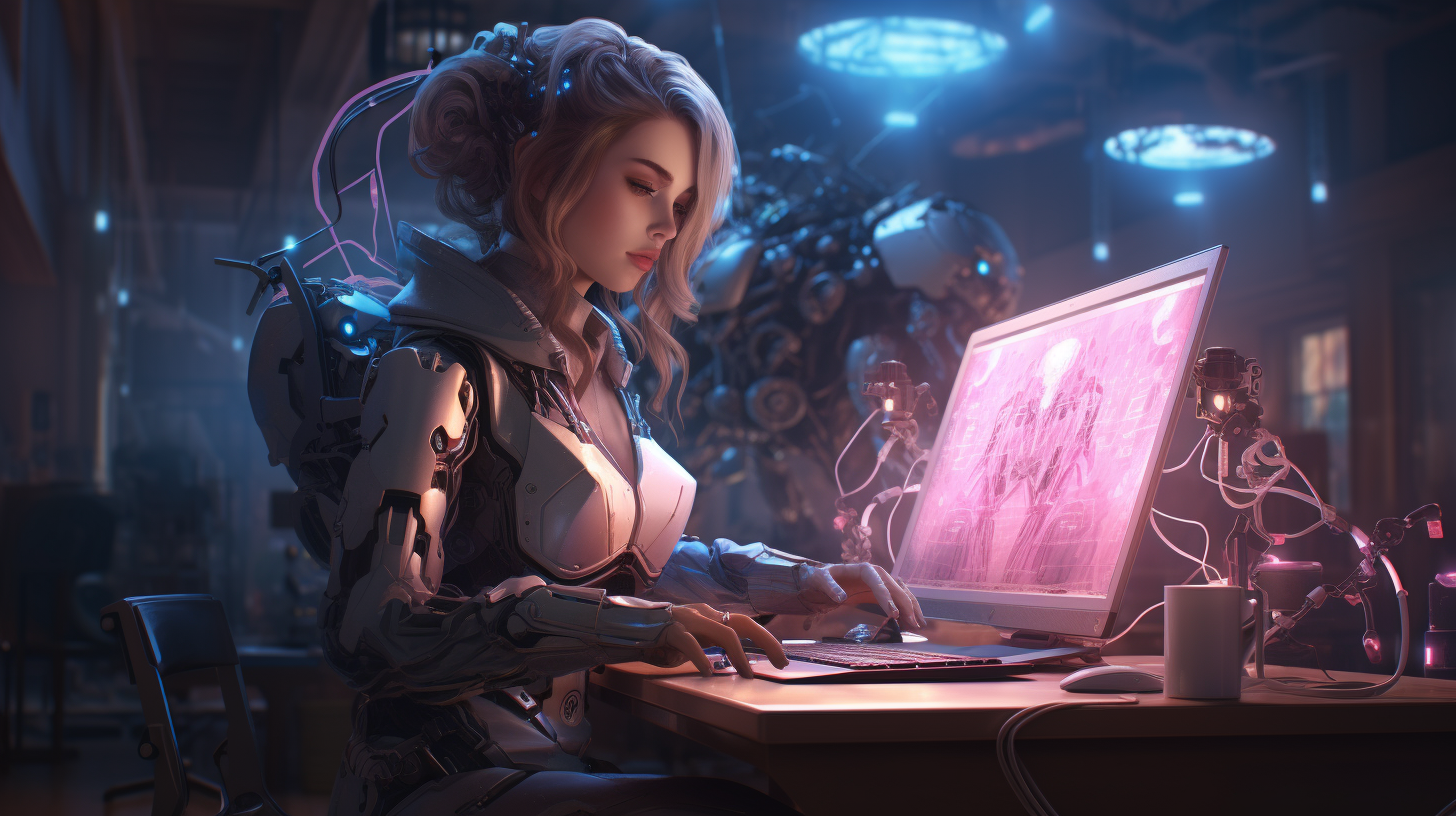
Classes in Swift
In Swift, a class is a foundational building block that encapsulates data and behavior. Unlike structures, classes are reference types, meaning instances of classes are shared across various parts of your program. This characteristic of reference types allows for a more dynamic interaction between objects, as modifying a class instance in one part of your code will reflect in all references to that instance.
Classes can have properties, which are variables that define the state of the class, and methods, which are functions that define the behavior. The ability to create complex types by bundling state and behavior together makes classes an essential part of object-oriented programming in Swift.
Defining a class in Swift is simpler. You use the class
keyword followed by the class name. Inside the class, you can define properties and methods. Here’s a simple example of a class definition:
class Vehicle { var currentSpeed: Double = 0.0 var description: String { return "Traveling at (currentSpeed) miles per hour" } func accelerate() { currentSpeed += 10.0 } }
In this example, the Vehicle
class has one property, currentSpeed
, which keeps track of the vehicle’s speed. It also has a computed property description
that provides a string representation of the vehicle’s current state. The accelerate
method increases the vehicle’s speed by 10 miles per hour.
When you create an instance of a class, you are creating a reference to that object. Here’s how you would instantiate the Vehicle
class and use its methods:
let myVehicle = Vehicle() myVehicle.accelerate() print(myVehicle.description) // Prints: Traveling at 10.0 miles per hour
This dynamic aspect of class instances is powerful, especially when managing state across different parts of an application. Swift classes also support advanced features like inheritance, so that you can create new classes based on existing ones, which fosters code reuse and organization.
In essence, understanding Swift classes paves the way for writing clean, efficient, and maintainable code. By using properties and methods within classes, developers can model real-world entities and behaviors effectively, forming the backbone of object-oriented design in Swift.
Defining Properties and Methods
Defining properties and methods within a class provides the structure needed to represent complex data types and encapsulate behavior. In Swift, properties can take on various forms, including stored properties, computed properties, and type properties. Each form serves a distinct purpose in shaping the internal state of a class.
A stored property is a constant or variable that’s stored as part of an instance of a class. Stored properties can be initialized with a default value or assigned a value during initialization. For instance, you can expand the Vehicle class to include a stored property for the number of wheels:
class Vehicle { var currentSpeed: Double = 0.0 var numberOfWheels: Int var description: String { return "Traveling at (currentSpeed) miles per hour on (numberOfWheels) wheels." } init(numberOfWheels: Int) { self.numberOfWheels = numberOfWheels } func accelerate() { currentSpeed += 10.0 } }
In this updated Vehicle class, the numberOfWheels property is defined as a stored property, initialized via a custom initializer. This process highlights how properties can be integrated into the overall design of a class, providing a richer context for the object’s state.
Computed properties, on the other hand, do not store a value. Instead, they provide a getter and an optional setter to indirectly manage the underlying data. The description property in the previous example is a computed property that creates a dynamic string based on the current speed and number of wheels. Here’s how you can extend the Vehicle class to include a computed property for fuel efficiency:
class Vehicle { var currentSpeed: Double = 0.0 var numberOfWheels: Int var fuelEfficiency: Double { return currentSpeed > 0 ? 50.0 / currentSpeed : 0.0 } var description: String { return "Traveling at (currentSpeed) miles per hour on (numberOfWheels) wheels with a fuel efficiency of (fuelEfficiency) mpg." } init(numberOfWheels: Int) { self.numberOfWheels = numberOfWheels } func accelerate() { currentSpeed += 10.0 } }
In this code, the fuelEfficiency computed property calculates the vehicle’s fuel efficiency based on its current speed. If the speed is zero, the fuel efficiency returns zero to avoid division by zero.
Methods are the actions that instances of classes can perform, encapsulating behavior that can manipulate the class’s properties or execute certain functionality. Methods are defined within the class body just like properties. In addition to the basic methods, you can also create methods that take parameters and return values, adding further flexibility to your class design.
Let’s illustrate this with a method that allows the Vehicle to brake:
class Vehicle { var currentSpeed: Double = 0.0 var numberOfWheels: Int var fuelEfficiency: Double { return currentSpeed > 0 ? 50.0 / currentSpeed : 0.0 } var description: String { return "Traveling at (currentSpeed) miles per hour on (numberOfWheels) wheels with a fuel efficiency of (fuelEfficiency) mpg." } init(numberOfWheels: Int) { self.numberOfWheels = numberOfWheels } func accelerate() { currentSpeed += 10.0 } func brake(deceleration: Double) { currentSpeed = max(0, currentSpeed - deceleration) } }
The brake method reduces the current speed by a specified deceleration amount, ensuring that the speed does not drop below zero. This demonstrates how methods can control and modify the properties of a class, thereby influencing its state and behavior.
Classes in Swift are extremely versatile, allowing for the encapsulation of both state (in properties) and behavior (in methods). Understanding how to define and utilize properties and methods effectively is important for any Swift developer aiming to create modular and maintainable code.
Inheritance and Method Overriding
Inheritance is a powerful feature of classes in Swift, which will allow you to create a new class based on an existing class. This new class, known as a subclass, inherits all the properties and methods of its superclass, allowing you to extend or modify its behavior without having to rewrite existing code. This concept not only promotes code reuse but also helps in organizing complex code structures.
To illustrate inheritance, let’s create a subclass called Car that inherits from the Vehicle class. The Car class will have some additional properties specific to cars, such as model and numberOfDoors.
class Car: Vehicle { var model: String var numberOfDoors: Int init(model: String, numberOfDoors: Int, numberOfWheels: Int) { self.model = model self.numberOfDoors = numberOfDoors super.init(numberOfWheels: numberOfWheels) } override var description: String { return "A (model) traveling at (currentSpeed) miles per hour on (numberOfWheels) wheels with (numberOfDoors) doors and a fuel efficiency of (fuelEfficiency) mpg." } }
In this example, the Car class extends Vehicle by adding new properties. The initializer of Car calls the superclass’s initializer using super.init(), passing the necessary parameters. Additionally, the description property is overridden to provide a more specific description that includes the model and number of doors of the car.
When you create an instance of Car, you can see both inherited and overridden behavior in action:
let myCar = Car(model: "Toyota Camry", numberOfDoors: 4, numberOfWheels: 4) myCar.accelerate() print(myCar.description) // Prints: A Toyota Camry traveling at 10.0 miles per hour on 4 wheels with 4 doors and a fuel efficiency of 5.0 mpg.
Method overriding allows subclasses to provide specific implementations of methods that are defined in their superclass. In our Car class, we have overridden the description property to return a string that includes information specific to the car. This flexibility is important for creating specialized behavior while still using the base functionality provided by the superclass.
Moreover, Swift provides a way to ensure that methods intended for overriding are explicitly marked. By using the override keyword, you make it clear that you are intentionally replacing a method from the superclass. If you forget to use override when overriding a method, Swift will generate a compile-time error, helping you catch mistakes early in the development process.
Additionally, you can also use final to prevent further subclasses from overriding a method or property. For instance:
class Vehicle { final func displayType() { print("This is a vehicle.") } }
In this case, any attempt to override displayType in subclasses will result in a compilation error, ensuring the method’s behavior remains consistent across all instances of Vehicle and its subclasses.
Inheritance and method overriding in Swift not only facilitate code reuse but also enhance the ability to create a flexible architecture, fostering clean and maintainable code. By understanding these principles, Swift developers can build robust class hierarchies that properly encapsulate and extend the functionality of base classes, resulting in a more organized codebase.
Using Initializers and Deinitializers
class Building { var numberOfFloors: Int var hasElevator: Bool init(numberOfFloors: Int, hasElevator: Bool) { self.numberOfFloors = numberOfFloors self.hasElevator = hasElevator } deinit { print("Building with (numberOfFloors) floors is being deinitialized.") } } class Apartment: Building { var numberOfUnits: Int init(numberOfFloors: Int, hasElevator: Bool, numberOfUnits: Int) { self.numberOfUnits = numberOfUnits super.init(numberOfFloors: numberOfFloors, hasElevator: hasElevator) } deinit { print("Apartment with (numberOfUnits) units is being deinitialized.") } }
In Swift, initializers are special methods used to set up an instance of a class. They ensure that all properties of the class are initialized before the instance is used. Every class has at least one initializer, known as a designated initializer, that sets up the properties of the class. You can also define convenience initializers, which are secondary initializers that call designated initializers to streamline the process of creating an instance.
The above example illustrates an Apartment class that inherits from a Building class. Each class has its own initializer to set up its properties. The Apartment class takes additional parameters specific to its context, demonstrating the idea of subclassing and initialization in action.
Moreover, deinitializers come into play when the instance of a class is about to be deallocated. They’re defined using the `deinit` keyword, so that you can perform any necessary cleanup, such as freeing resources or saving state. In the example, both the Building and Apartment classes contain deinitializers that notify when an instance is being deallocated.
var myBuilding: Building? = Building(numberOfFloors: 5, hasElevator: true) myBuilding = nil // Outputs: Building with 5 floors is being deinitialized. var myApartment: Apartment? = Apartment(numberOfFloors: 10, hasElevator: false, numberOfUnits: 30) myApartment = nil // Outputs: Apartment with 30 units is being deinitialized.
When you set an instance of a class to nil, the deinitializer is called, allowing you to manage the lifecycle of your objects effectively. This is particularly important in scenarios involving resource management, making it clear when an object is no longer needed, thus providing a mechanism to clean up.
Notably, initializers can also accept parameters to allow for flexible instance creation. For instance, if you want to create a building with a specific number of floors and elevator status, an initializer can take those parameters and use them to set the initial state of the object. This pattern enhances code readability and maintainability, as it consolidates the setup logic into a single place.
In summary, understanding initializers and deinitializers in Swift especially important for managing the lifecycle of class instances efficiently. They ensure that objects are set up correctly when they’re created and cleaned up properly when they are no longer needed, leading to safer and more predictable memory management in your applications.