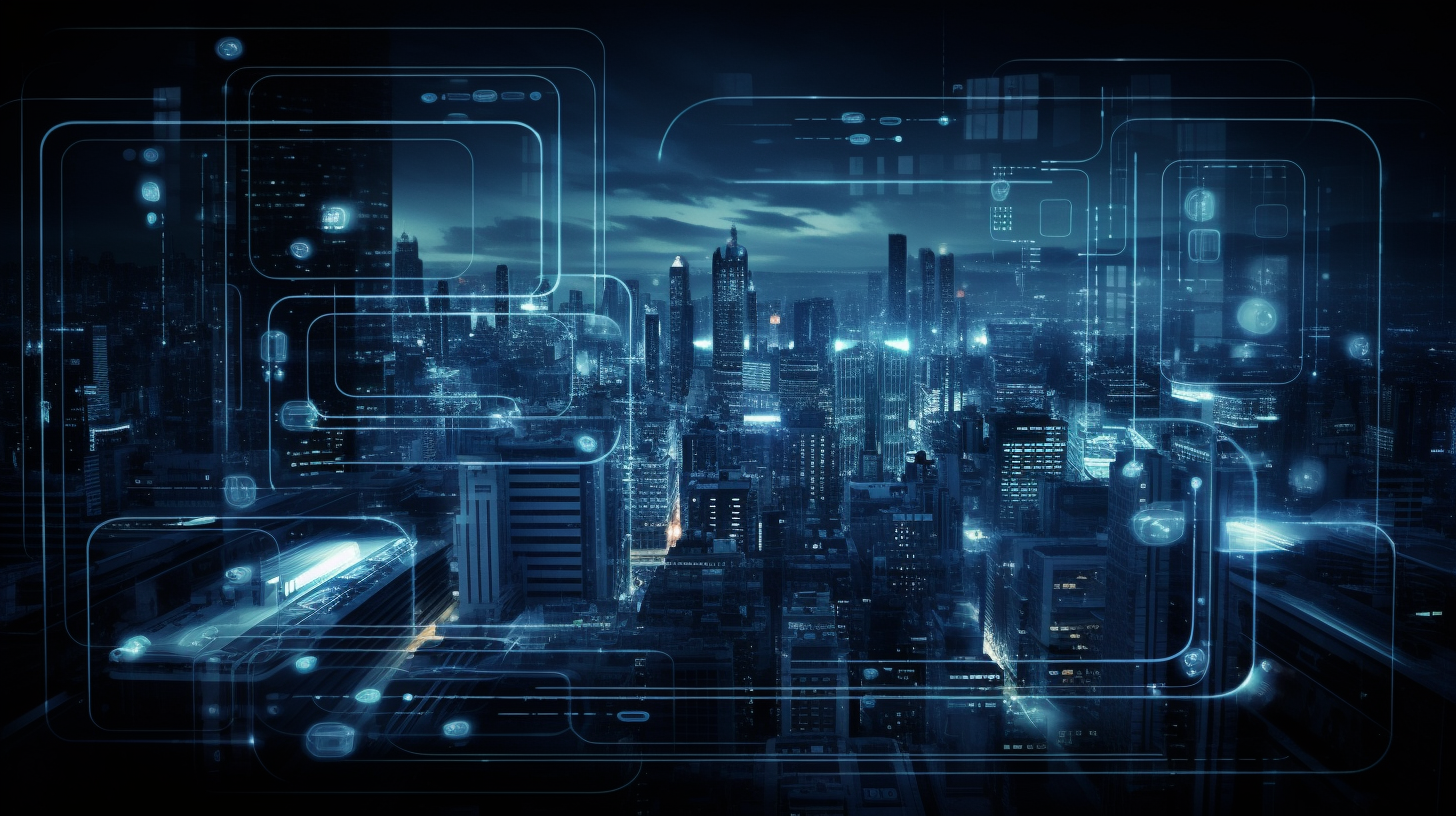
Bash Loops Explained
Bash loops are a fundamental part of scripting, so that you can execute a block of code multiple times until a certain condition is met or for a specified number of iterations. This characteristic makes them incredibly powerful for automating tasks that require repetition.
At the core of Bash loops is the ability to iterate over lists, ranges, or even files. This means you can manipulate data efficiently without the need for cumbersome manual input. Understanding how loops work allows you to harness the true power of Bash scripting, allowing for the automation of complex processes with minimal effort.
In Bash, there are several types of loops, each suited for specific scenarios. The most commonly used loops are for loops, while loops, and until loops. Each type offers distinct advantages and can be used based on the requirements of your script.
For instance, the for loop is typically employed when the number of iterations is known in advance. A classic use case would be looping through a list of files in a directory:
for file in *.txt; do echo "Processing $file" done
In this example, the loop iterates over all text files in the current directory, echoing each filename. This demonstrates the ease with which you can handle a series of items without explicitly managing the iteration index.
On the other hand, while and until loops allow you to execute code based on conditions, rather than a fixed number of iterations. A while loop continues as long as a given condition evaluates to true:
count=1 while [ $count -le 5 ]; do echo "Count is $count" ((count++)) done
This snippet demonstrates a simple counter, incrementing by one until it reaches five. In contrast, an until loop works oppositely—it continues until a specified condition becomes true:
count=1 until [ $count -gt 5 ]; do echo "Count is $count" ((count++)) done
Both loops serve different purposes, but the flexibility they offer makes them essential tools in a Bash developer’s toolkit.
Types of Loops in Bash
Bash provides several types of loops, each tailored for different situations, allowing programmers to choose the most appropriate structure based on the task at hand. These loops not only enhance code readability but also improve efficiency by simplifying repetitive tasks.
For Loop
The for loop is ideal when you know in advance how many times you want to iterate. It allows you to loop over a list of items, such as files or numbers, making it an effective choice for batch processing. The basic syntax follows a simple structure:
for item in list; do commands done
Here’s an example that demonstrates the for loop iterating over a set of numbers:
for number in {1..5}; do echo "Number is $number" done
This code snippet prints the numbers from 1 to 5, showcasing how quickly you can iterate through a range of values.
While Loop
The while loop is more versatile, allowing execution as long as a specified condition remains true. This type of loop is particularly useful when the number of iterations is not predetermined. The general syntax is:
while [ condition ]; do commands done
Ponder this example that prompts for user input until a valid response is received:
input="" while [ "$input" != "yes" ]; do read -p "Please type 'yes' to continue: " input done
In this case, the loop will continue to prompt the user until they enter “yes”, demonstrating the adaptability of while loops for user-driven scenarios.
Until Loop
Complementing the while loop is the until loop, which executes commands until a specified condition becomes true. The syntax mirrors that of the while loop:
until [ condition ]; do commands done
An example of an until loop could be tracking a process until it completes:
process_running=true until [ "$process_running" = false ]; do echo "Waiting for the process to complete..." # Simulate checking the process status sleep 1 process_running=false # Replace this with actual process check done
This example highlights how until loops can be used effectively for monitoring tasks that may take an indeterminate time to finish.
Syntax and Structure of Loop Constructs
The syntax and structure of loop constructs in Bash are designed to be both simpler and powerful, allowing for a wide range of applications. While the specifics can vary based on the type of loop, there are fundamental components common to all loop structures that every Bash programmer should grasp.
At the heart of every loop is the keyword that initiates the iteration. For a for loop, that’s the for
keyword; for a while loop, it’s while
; and for an until loop, it’s until
. Each loop begins with the respective keyword followed by a conditional expression or list of items, then proceeds to the do
keyword, which signifies the start of the commands that will be executed within the loop.
Here’s a detailed look at the syntax of each loop type:
# For Loop Syntax for item in list; do # Commands to execute for each item done # While Loop Syntax while [ condition ]; do # Commands to execute as long as the condition is true done # Until Loop Syntax until [ condition ]; do # Commands to execute until the condition is true done
For the for loop, the list
can be an explicit list of values, a range, or even the output of a command. This flexibility allows you to execute similar commands on each item in the list efficiently. For example:
# Looping over a list of filenames for file in *.sh; do echo "Found shell script: $file" done
When using a while loop, the condition
typically involves a test expression that evaluates to true or false. This condition is checked before each iteration, and the loop continues as long as the condition remains true. This is useful for scenarios where the number of iterations is not predetermined, as shown below:
# Loop until a variable reaches a certain value count=0 while [ $count -lt 10 ]; do echo "Count is $count" ((count++)) done
In contrast, an until loop continues execution until the specified condition evaluates to true. This means the loop will run as long as the condition remains false, which can sometimes be more intuitive depending on the logic you’re implementing:
# Loop until a process is finished process_finished=false until [ "$process_finished" = true ]; do echo "The process is still running..." sleep 2 # Simulate checking for process completion process_finished=true # This would typically be a condition check done
Each loop structure concludes with the done
keyword, which indicates the end of the loop block. Properly formatting your loops with these essential keywords not only enhances readability but also ensures that your script behaves as intended.
In addition to understanding loop syntax, it is essential to ponder control statements within the loop. These include break and continue. The break
statement allows you to exit a loop prematurely, while continue
skips the current iteration and proceeds with the next one. Incorporating these control statements can provide additional flow control in your scripts:
# Example of break and continue for number in {1..10}; do if [ $number -eq 5 ]; then echo "Skipping number 5" continue # Skip the rest of this iteration fi if [ $number -eq 8 ]; then echo "Breaking out of the loop" break # Exit the loop completely fi echo "Number is $number" done
Using Loop Control Statements
Loop control statements in Bash provide essential mechanisms to manage the flow of execution within loops, allowing for greater flexibility and control over how iterations are handled. The primary control statements utilized in loops are break and continue, each serving distinct purposes to influence the iteration process.
The break statement is used to terminate the loop entirely, immediately exiting the loop upon execution. This is particularly useful when a specific condition is met that necessitates stopping the loop, preventing any further iterations. The syntax is simple:
break
Here’s an example demonstrating the use of break within a for loop that processes numbers:
for number in {1..10}; do if [ $number -eq 6 ]; then echo "Breaking out of the loop at number $number" break fi echo "Number is $number" done
In this snippet, the loop iterates through numbers 1 to 10 and checks if the current number is 6. When it reaches 6, the loop terminates, and no further numbers are processed.
On the other hand, the continue statement skips the current iteration of the loop and proceeds directly to the next iteration. This can be handy in scenarios where you want to bypass certain conditions without halting the entire loop. The syntax for continue is also straightforward:
continue
Think the following example where continue is used to skip even numbers:
for number in {1..10}; do if [ $((number % 2)) -eq 0 ]; then echo "Skipping even number $number" continue fi echo "Odd number is $number" done
This snippet iterates through the numbers 1 to 10, checking if each number is even. If it’s, the current iteration is skipped, and the loop moves to the next number without executing the echo statement for even numbers.
Using these control statements enhances the logic and efficiency of Bash scripts by allowing for more nuanced behavior within loops. For instance, a combination of break and continue can be employed for complex data processing tasks, where specific conditions dictate whether to process, skip, or terminate operations.
It’s also important to note that these control statements can be nested within multiple loops. When using break in a nested loop, it will only exit the innermost loop unless specified otherwise. To exit multiple levels, one might use a labeled break, although this feature is not natively supported in Bash. A common practice is to use flags or functions to manage multi-level exits effectively.
Practical Examples and Use Cases
Practical examples of Bash loops illuminate their versatility and power in real-world scripting scenarios. Here, we explore several use cases that demonstrate how loops can automate tedious tasks and enhance productivity.
One common application of loops is managing files within directories. For instance, if you need to rename a batch of files, a for loop can facilitate this process smoothly. Ponder a scenario where you want to append a prefix to all text files in a directory:
for file in *.txt; do mv "$file" "prefix_$file" done
This script iterates over each text file, renaming it by adding “prefix_” to the beginning of its filename. It’s simpler, yet it saves substantial time compared to renaming files individually.
Another practical use case involves processing data within a list. Imagine you have a list of server addresses, and you need to ping each server to check its availability. A while loop can handle this elegantly:
servers=("192.168.1.1" "192.168.1.2" "192.168.1.3") count=0 while [ $count -lt ${#servers[@]} ]; do ping -c 1 "${servers[$count]}" ((count++)) done
In this example, the while loop iterates through the array of server addresses, pinging each one to check if it’s reachable. The use of an array allows for dynamic management of server addresses without hardcoding them into the script, thus enhancing flexibility.
When dealing with user input, loops can ensure scripts run until valid input is received, as shown in this example where a while loop prompts until a valid password is entered:
password="" while [ "$password" != "securepassword" ]; do read -p "Enter password: " password done echo "Access granted!"
This script continues to prompt the user for a password until the correct one is entered, demonstrating how loops can enhance user interactivity in scripts.
Loops also shine in data processing tasks, such as parsing log files. Ponder a scenario where you want to extract error messages from a log file:
while read line; do if [[ $line == *"ERROR"* ]]; then echo "$line" fi done < logfile.txt
This while loop reads each line of a log file and checks for the presence of the string “ERROR.” If found, it prints the line, effectively filtering the log for relevant information.