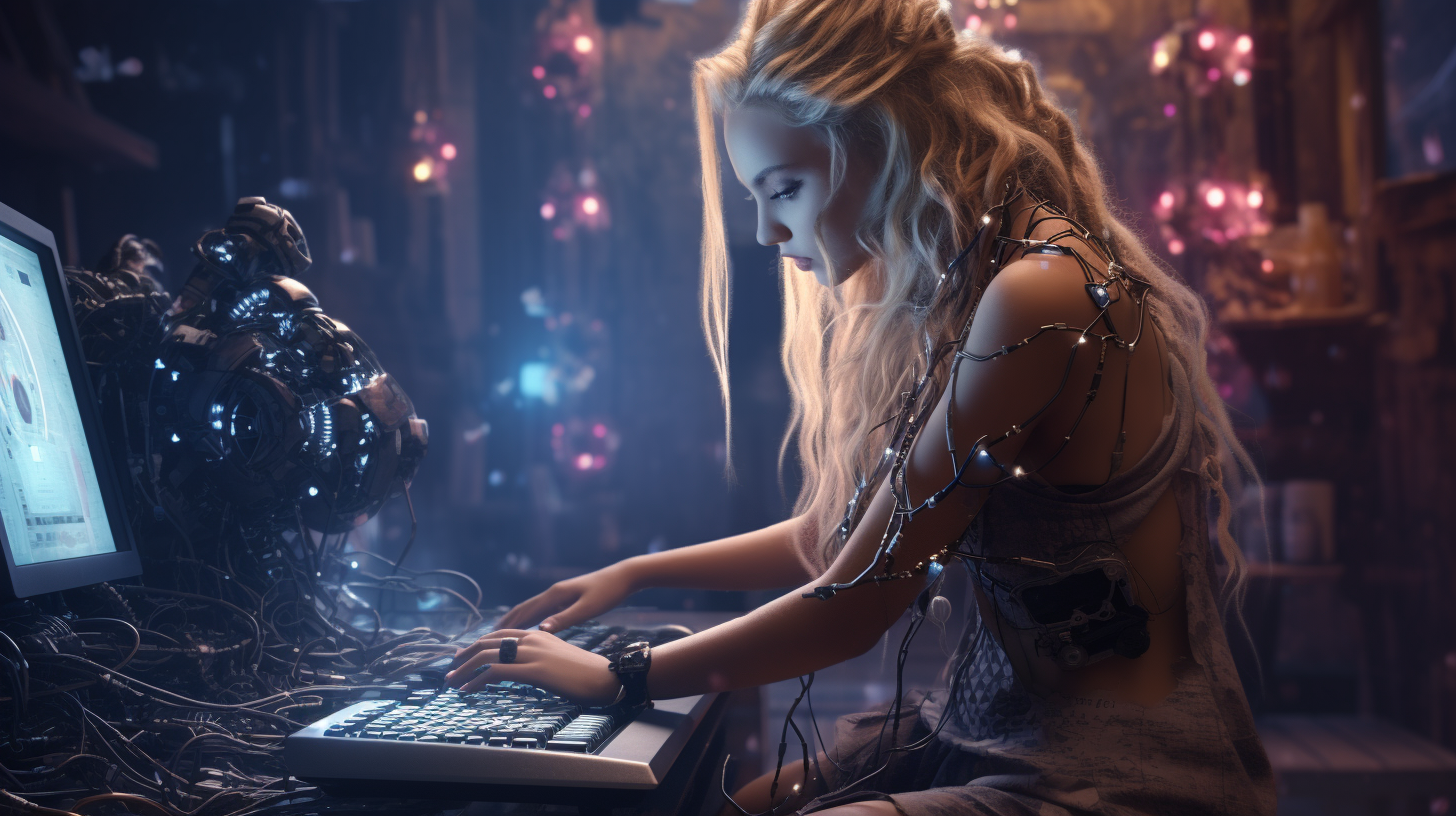
Python and Political Science: Data Analysis
Python has emerged as a powerful tool in the context of political science research, enabling scholars and analysts to tackle complex datasets with ease and efficiency. From analyzing electoral data to assessing public opinion through social media, Python provides a versatile platform for various applications.
One of the most compelling applications of Python in political science is in the context of sentiment analysis. Researchers can gauge public sentiment around political events or figures by mining text data from social media platforms. Using libraries such as nltk
or TextBlob
, analysts can process and analyze large volumes of text to uncover trends in public opinion.
import pandas as pd from textblob import TextBlob # Sample data containing tweets about a political figure data = {'tweets': ["I love the new policies!", "What a disaster this administration is!", "Feeling optimistic about the future.", "This is the worst government ever!"]} df = pd.DataFrame(data) # Function to calculate sentiment def analyze_sentiment(tweet): analysis = TextBlob(tweet) return analysis.sentiment.polarity # Applying sentiment analysis df['sentiment'] = df['tweets'].apply(analyze_sentiment) print(df)
Another significant area where Python shines is in network analysis. Political scientists often study the interactions between various entities, such as voters, political parties, or interest groups. Python libraries like NetworkX
allow researchers to create, manipulate, and study the structure of complex networks.
import networkx as nx import matplotlib.pyplot as plt # Creating a simple network of political connections G = nx.Graph() G.add_edges_from([("Party A", "Voter 1"), ("Party A", "Voter 2"), ("Party B", "Voter 3"), ("Party C", "Voter 1"), ("Voter 2", "Voter 3")]) # Drawing the network graph nx.draw(G, with_labels=True, node_color="skyblue", node_size=2000, font_size=10) plt.show()
In the context of voter behavior analysis, Python can be used to model and predict electoral outcomes based on historical data. Libraries such as scikit-learn
provide robust tools for implementing various machine learning algorithms, allowing researchers to explore factors that influence voting patterns.
from sklearn.model_selection import train_test_split from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score # Hypothetical voter data data = pd.DataFrame({ 'age': [18, 22, 35, 40, 50, 60], 'income': [20000, 30000, 50000, 60000, 80000, 90000], 'voted': [0, 1, 1, 1, 0, 0] }) # Features and target variable X = data[['age', 'income']] y = data['voted'] # Splitting the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Logistic Regression model model = LogisticRegression() model.fit(X_train, y_train) # Making predictions predictions = model.predict(X_test) print('Accuracy:', accuracy_score(y_test, predictions))
These examples illustrate the vast potential of Python in political science research, facilitating the analysis of complex data and providing insights that were previously difficult or impossible to obtain. As the field continues to evolve, Python stands as a key ally in understanding the intricate dynamics of political behavior and structure.
Data Collection Techniques for Political Analysis
In response to a rising demand for political science research, data collection serves as the foundation upon which analyses and insights are built. Python, with its extensive libraries and frameworks, provides a multitude of techniques for gathering data across various platforms, making it an indispensable tool for researchers in this field.
One of the prevalent methods for collecting data involves web scraping, where Python can be used to extract information from websites that may not provide APIs. Libraries such as BeautifulSoup and Scrapy are instrumental in parsing HTML and navigating web pages efficiently. For example, ponder the scenario where a researcher wants to gather information on political candidates from a news website. The following code snippet demonstrates how to use BeautifulSoup to scrape candidate names and their corresponding parties:
from bs4 import BeautifulSoup import requests # URL of the page to scrape url = 'http://example.com/political_candidates' response = requests.get(url) # Parsing the HTML content soup = BeautifulSoup(response.content, 'html.parser') # Finding candidate names and parties candidates = soup.find_all('div', class_='candidate-info') data = [] for candidate in candidates: name = candidate.find('h2').text party = candidate.find('span', class_='party').text data.append({'name': name, 'party': party}) print(data)
Moreover, social media platforms have become a rich source of political data. Python can interface with APIs such as Twitter’s, allowing researchers to retrieve tweets containing specific hashtags or keywords. The Tweepy library simplifies the process of interacting with the Twitter API. Below is an example that shows how to collect tweets regarding a political event:
import tweepy # Authenticating with the Twitter API consumer_key = 'your_consumer_key' consumer_secret = 'your_consumer_secret' access_token = 'your_access_token' access_token_secret = 'your_access_token_secret' auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) api = tweepy.API(auth) # Searching for tweets about a political event tweets = api.search(q='Election2024', count=100) # Extracting tweet texts tweet_data = [{'user': tweet.user.screen_name, 'text': tweet.text} for tweet in tweets] print(tweet_data)
Additionally, survey data is another critical source of information for political analysis. Python can facilitate the collection and analysis of survey responses through libraries such as pandas and NumPy. Researchers can design surveys, collect responses, and analyze the results seamlessly. A simple example of how to process survey data in Python is shown below:
import pandas as pd # Sample survey data data = { 'respondent_id': [1, 2, 3, 4, 5], 'age': [25, 30, 22, 40, 35], 'vote_preference': ['A', 'B', 'A', 'A', 'C'] } df = pd.DataFrame(data) # Analyzing vote preferences vote_counts = df['vote_preference'].value_counts() print(vote_counts)
These diverse data collection techniques demonstrate the adaptability of Python to meet the research needs of political scientists. By using web scraping, API interactions, and survey analysis, researchers can gather comprehensive datasets that form the basis for robust political analysis. This capability not only enhances the depth of research but also allows for the examination of emerging political trends in real-time.
Statistical Methods and Libraries for Political Data
Statistical analysis plays an important role in political science research, allowing scholars to draw meaningful conclusions from data and test hypotheses about political behavior and trends. Python’s rich ecosystem of libraries provides a robust framework for implementing various statistical methods and models, making it an invaluable asset for political analysts.
One of the foundational libraries for statistical analysis in Python is statsmodels. This library offers classes and functions for estimating many different statistical models, including linear regression, time series analysis, and hypothesis testing. For instance, when analyzing the relationship between voter turnout and demographic factors, researchers can utilize linear regression to model this relationship effectively.
import pandas as pd import statsmodels.api as sm # Sample data: Voter turnout based on age and income data = { 'age': [18, 22, 35, 40, 50, 60], 'income': [20000, 30000, 50000, 60000, 80000, 90000], 'turnout': [0.1, 0.3, 0.5, 0.6, 0.7, 0.8] } df = pd.DataFrame(data) # Defining the independent variables and adding a constant for the intercept X = df[['age', 'income']] X = sm.add_constant(X) # Defining the dependent variable y = df['turnout'] # Fitting the linear regression model model = sm.OLS(y, X).fit() # Printing the summary of the regression print(model.summary())
Another important library is scipy, which provides a wide array of statistical functions. It can be particularly useful for conducting hypothesis tests. For example, political scientists might want to compare the means of two groups to ascertain if there are significant differences in voting behavior based on party affiliation. An independent t-test can be performed using scipy as demonstrated below:
from scipy import stats # Sample data: Voting behavior of two different parties group_a = [1, 0, 1, 1, 0, 1] group_b = [0, 0, 1, 0, 0, 1] # Performing an independent t-test t_stat, p_value = stats.ttest_ind(group_a, group_b) print('T-statistic:', t_stat) print('P-value:', p_value)
For more complex analyses, such as logistic regression used for predicting binary outcomes (e.g., whether an individual voted or not), scikit-learn becomes essential. This library provides accessible implementations of machine learning algorithms, allowing researchers to explore patterns in voting behavior based on multiple predictors.
from sklearn.linear_model import LogisticRegression from sklearn.model_selection import train_test_split from sklearn.metrics import classification_report # Hypothetical voter data data = pd.DataFrame({ 'age': [18, 22, 35, 40, 50, 60], 'income': [20000, 30000, 50000, 60000, 80000, 90000], 'voted': [0, 1, 1, 1, 0, 0] }) # Features and target variable X = data[['age', 'income']] y = data['voted'] # Splitting the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Logistic Regression model model = LogisticRegression() model.fit(X_train, y_train) # Making predictions predictions = model.predict(X_test) # Generating classification report print(classification_report(y_test, predictions))
These libraries and methods illustrate the power of Python in conducting statistical analysis within political science. By employing a mix of regression models, hypothesis testing, and machine learning techniques, researchers can derive insights that inform understanding of electoral behavior, public opinion, and other critical political phenomena. The capabilities provided by Python not only streamline the analysis process but also enhance the rigor and reproducibility of research findings.
Visualizing Political Trends and Patterns Using Python
Visualizing data is an essential component of political science research, as it allows scholars to present complex results in a clear and meaningful way. Python, with its robust visualization libraries, offers an array of tools to create compelling graphics that can illuminate trends, patterns, and anomalies within political datasets.
One of the most widely used libraries for creating plots in Python is Matplotlib. This library provides a foundation for producing high-quality graphics in various formats. For instance, researchers can visualize voter turnout across different demographic groups by generating bar charts or line graphs. Below is an example illustrating how to use Matplotlib to create a simple bar chart comparing voter turnout by age group:
import matplotlib.pyplot as plt # Sample data: Voter turnout by age group age_groups = ['18-24', '25-34', '35-44', '45-54', '55-64', '65+'] voter_turnout = [0.45, 0.58, 0.60, 0.67, 0.70, 0.75] # Creating a bar chart plt.bar(age_groups, voter_turnout, color='skyblue') plt.title('Voter Turnout by Age Group') plt.xlabel('Age Group') plt.ylabel('Voter Turnout (%)') plt.ylim(0, 1) # Set limit for y-axis plt.show()
Beyond Matplotlib, Seaborn enhances the visualization experience by providing a higher-level interface for drawing attractive statistical graphics. It’s particularly useful for visualizing complex datasets and allows for easy integration with pandas DataFrames. For example, researchers can create heatmaps to visualize correlations among various political variables:
import seaborn as sns import numpy as np # Sample data: Correlation matrix data = np.random.rand(10, 12) heatmap_data = pd.DataFrame(data, columns=[f'Var {i}' for i in range(1, 13)]) # Creating a heatmap plt.figure(figsize=(10, 8)) sns.heatmap(heatmap_data.corr(), annot=True, cmap='coolwarm', fmt=".2f") plt.title('Correlation Heatmap of Political Variables') plt.show()
Furthermore, for more interactive visualizations, Plotly can be utilized. This library allows users to create dynamic graphs that can be embedded in web applications. For instance, researchers can utilize Plotly to plot voter preferences across different political parties in an interactive pie chart:
import plotly.express as px # Sample data: Voter preferences data = {'Party': ['A', 'B', 'C', 'D'], 'Votes': [300, 150, 100, 50]} df = pd.DataFrame(data) # Creating an interactive pie chart fig = px.pie(df, values='Votes', names='Party', title='Voter Preferences by Political Party') fig.show()
Visualizing data not only aids in understanding complex relationships but also serves as a powerful tool for communicating findings to broader audiences. The ability to present political data in engaging and insightful ways enhances the impact of research and fosters informed discussions around political phenomena.