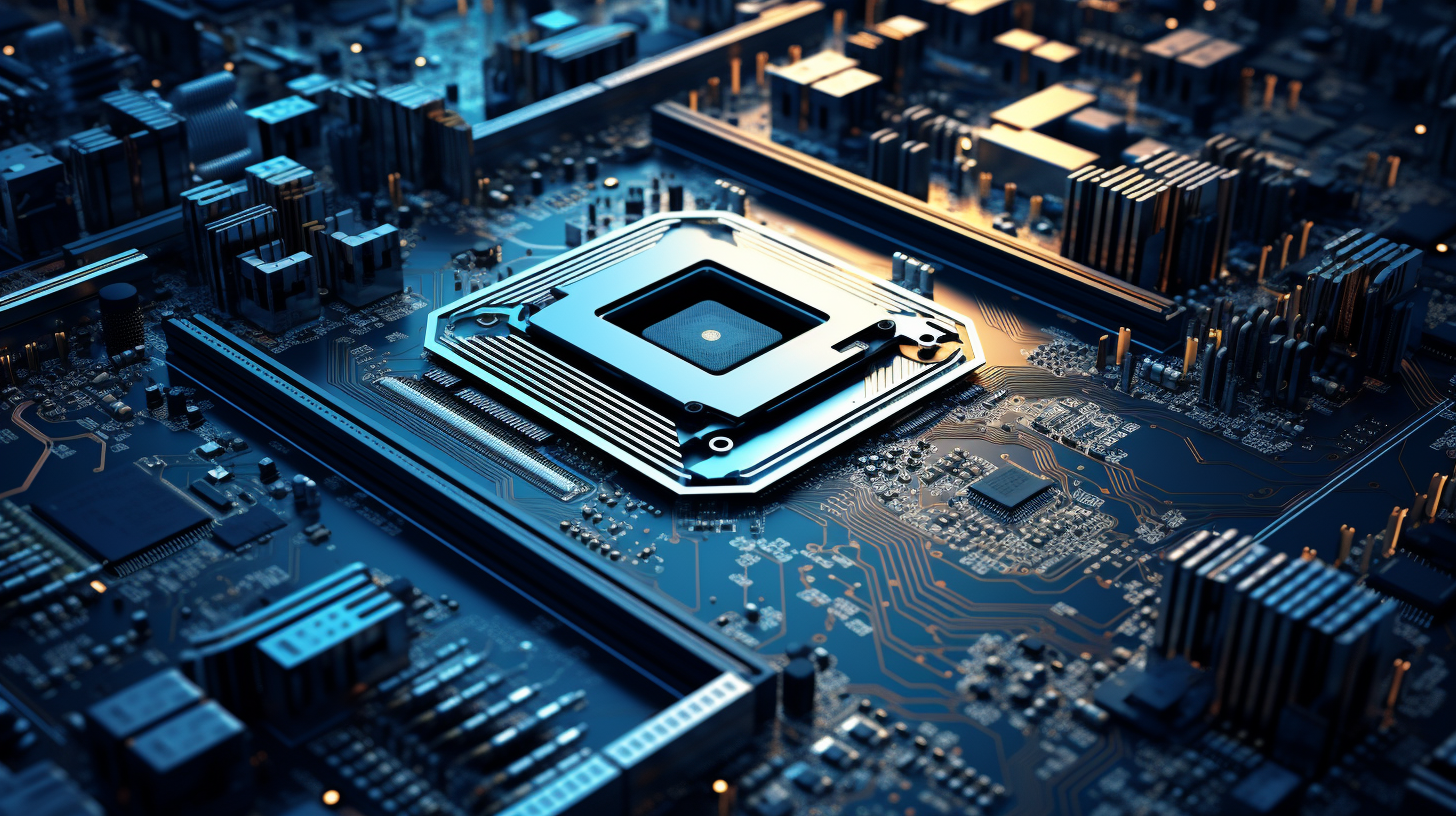
Getting Started with JavaScript
JavaScript is a versatile programming language this is primarily used for enhancing the interactivity and functionality of web pages. It enables developers to implement complex features on web applications, from dynamic content updates to interactive maps and animations. To truly grasp JavaScript, one must understand its fundamental concepts and syntax.
At its core, JavaScript operates on a simple, yet powerful syntax that includes variables, data types, functions, and control structures. Let’s explore these basics in more detail:
Variables are the foundation of storing data in JavaScript. You can declare variables using var
, let
, or const
. While var
has function scope, let
and const
have block scope, which helps in managing variable visibility.
var name = "John"; // This can be re-declared and updated let age = 30; // This can be updated, but not re-declared in the same scope const pi = 3.14; // This cannot be updated or re-declared
Next, we have data types, which can be divided into primitives (such as strings, numbers, and booleans) and objects. Understanding these types helps in manipulating data effectively. For example:
let isJavaScriptFun = true; // Boolean let numberOfStudents = 25; // Number let studentName = "Alice"; // String
Functions are another crucial aspect of JavaScript. They allow you to encapsulate code for reuse. Functions can be defined in different ways, including function declarations, function expressions, and arrow functions:
function greet(name) { return "Hello, " + name + "!"; } const greetArrow = (name) => "Hello, " + name + "!"; let greeting = greet("Bob"); // Function call
Control structures, like if statements and loops, enable you to dictate the flow of your program. Here’s a simple example demonstrating an if
statement and a for
loop:
let score = 85; if (score >= 70) { console.log("You passed!"); } else { console.log("You failed!"); } for (let i = 1; i <= 5; i++) { console.log("This is iteration " + i); }
Understanding these fundamental concepts is essential for any aspiring JavaScript developer. As you dive deeper into the language, you will encounter more advanced topics such as asynchronous programming, DOM manipulation, and APIs—all built upon these basic principles.
Setting Up Your Development Environment
Setting up your development environment very important to becoming a proficient JavaScript programmer. An optimal setup will enhance your productivity and provide you with the necessary tools to write, debug, and test your JavaScript code efficiently. In this section, we will go through the steps needed to establish a robust development environment for JavaScript.
First and foremost, you’ll need a good text editor or an Integrated Development Environment (IDE). Popular choices include Visual Studio Code, Sublime Text, and Atom. These editors come with features like syntax highlighting, code completion, and extensions that greatly aid the coding process.
To install Visual Studio Code, follow these steps:
# For macOS brew install --cask visual-studio-code # For Windows # Download and run the installer from https://code.visualstudio.com/ # For Linux sudo snap install code --classic
Once installed, you can customize Visual Studio Code by adding useful extensions such as:
- A linter that helps catch common coding errors and enforces coding conventions.
- A code formatter that automatically formats your code to ensure consistency.
- A development server with live reload capability that allows you to see your code changes immediately in the browser.
Next up is setting up a version control system. Git is the most widely used version control system and is essential for tracking changes in your code and collaborating with others. You can install Git by following these instructions:
# For macOS brew install git # For Windows # Download and install Git from https://git-scm.com/ # For Linux sudo apt-get install git
After installing Git, you can configure it with your username and email:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
If you’re planning to work on web applications, you should also install Node.js. Node.js provides a runtime environment for executing JavaScript code outside the browser and includes npm (Node Package Manager), which is used for managing libraries and dependencies. Here’s how to install Node.js:
# For macOS brew install node # For Windows # Download and run the installer from https://nodejs.org/ # For Linux sudo apt-get install nodejs npm
Once Node.js is installed, you can verify the installation by checking the versions of Node and npm:
node -v npm -v
With Node.js, you can initialize a new project by creating a package.json file, which keeps track of your project dependencies:
mkdir my-js-project cd my-js-project npm init -y
This command creates a new directory for your project and generates a default package.json file. You can then start installing packages as needed using npm.
Lastly, for testing your JavaScript code, think using testing frameworks such as Jest or Mocha. These frameworks streamline the testing process and allow for automated testing of your code:
# Install Jest npm install --save-dev jest
Establishing a well-structured development environment involves selecting the right editor, setting up version control with Git, installing Node.js for runtime capabilities, and incorporating testing frameworks. With this foundation, you will be better equipped to explore JavaScript programming and tackle more complex projects with confidence.
Core JavaScript Concepts
Now that we’ve covered the essentials of variables, data types, functions, and control structures, it’s time to delve deeper into more advanced core concepts that enrich your JavaScript experience. These concepts include scope, closures, and the idea of this, which are pivotal for mastering the language.
Scope defines the accessibility of variables in different parts of your code. There are two main types of scope: global scope, where variables are accessible throughout the entire program, and local scope, where variables are only accessible within the function or block they were declared in. Think the following example:
var globalVar = "I am global"; function checkScope() { var localVar = "I am local"; console.log(globalVar); // Accessible console.log(localVar); // Accessible } checkScope(); console.log(globalVar); // Accessible console.log(localVar); // Error: localVar is not defined
This distinction is important as it prevents variable collisions and unintended bugs in your code. However, local variables are not accessible from outside their respective function, which leads us to the next topic: closures.
A closure is a powerful feature in JavaScript that allows a function to remember the environment in which it was created. This means a function retains access to its lexical scope, even when the function is executed outside that scope. Here’s how closures work:
function createCounter() { let count = 0; // count is a private variable return function() { count += 1; // This inner function has access to count return count; }; } const counter = createCounter(); console.log(counter()); // Outputs 1 console.log(counter()); // Outputs 2
In this example, the inner function maintains access to the count variable, thus preserving its state between calls. This encapsulation of state is a foundational concept in functional programming and is widely used in JavaScript.
Next, we must address the peculiar behavior of the this keyword. The value of this is determined by how a function is called, not where it is defined. In the global context, this refers to the global object (window in browsers). Within a method, it refers to the object that the method was called on. Think the following example:
const user = { name: "Alice", greet: function() { console.log("Hello, " + this.name); } }; user.greet(); // Outputs: Hello, Alice const greetFunction = user.greet; greetFunction(); // Outputs: Hello, undefined (this refers to the global object)
To address such issues with this, developers often use methods like call, apply, or bind to explicitly set the value of this when needed. For example:
const greetFunctionBound = user.greet.bind(user); greetFunctionBound(); // Outputs: Hello, Alice
Understanding these core concepts—scope, closures, and the this keyword—will provide a solid foundation as you continue your journey with JavaScript. Mastery of these concepts will not only improve your coding abilities but also enhance your overall problem-solving skills in programming.
Building Your First JavaScript Application
Now that we’ve covered the foundational elements of JavaScript, let’s dive into the exciting process of building your first JavaScript application. This project will help solidify your understanding of the concepts we’ve discussed while providing a tangible outcome that showcases your skills.
For our initial application, we’ll create a simple interactive web page that allows users to input their name and receive a personalized greeting. This simpler project will involve HTML for structure, CSS for styling, and JavaScript to implement the logic.
Begin by creating an HTML file named index.html
and set up the basic structure:
Greeting Application Welcome to the Greeting App
In this HTML structure, we have an input field for users to enter their name, a button to trigger the greeting action, and a paragraph to display the greeting message. Next, we will create a CSS file named styles.css
to add some basic styling:
body { font-family: Arial, sans-serif; display: flex; justify-content: center; align-items: center; height: 100vh; background-color: #f4f4f4; } #app { text-align: center; background: white; padding: 20px; border-radius: 10px; box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1); }
Now, let’s add the JavaScript logic to make the app functional. Create a file named script.js
and implement the following code:
document.getElementById('greetButton').addEventListener('click', function() { const nameInput = document.getElementById('nameInput').value; const greetingMessage = document.getElementById('greetingMessage'); if (nameInput) { greetingMessage.textContent = 'Hello, ' + nameInput + '! Welcome to our application.'; } else { greetingMessage.textContent = 'Please enter your name.'; } });
In this JavaScript code, we first attach an event listener to the button that waits for a click event. Once clicked, we retrieve the value entered in the input field. If the input isn’t empty, we construct a greeting message; otherwise, we prompt the user to input their name. This interactive element reinforces the concepts of variables, functions, and control structures we’ve covered earlier.
Now, to see your application in action, simply open index.html
in your web browser. You should see the input field and button. Enter your name and click the button to receive a personalized greeting!
Building this basic application not only familiarizes you with JavaScript’s role in web development but also sets the stage for more complex applications in the future. As you experiment and expand on this project, you can integrate additional features such as styling adjustments, more interactive elements, or even local storage to remember user input.