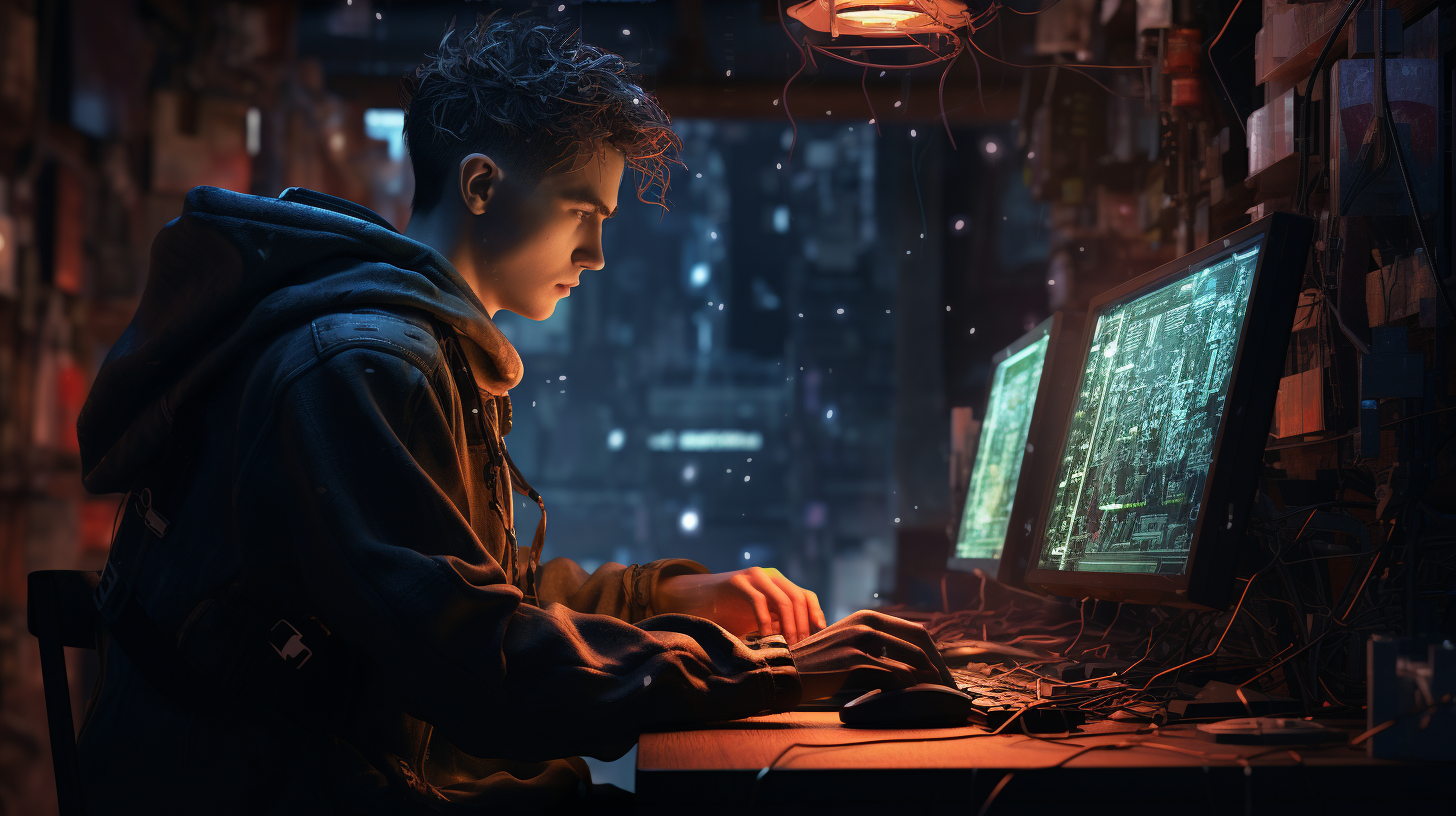
Bash Scripting for Data Analysis
When working with data in Bash, it’s essential to have a solid grasp of data manipulation techniques. Bash offers a suite of powerful tools that can help you process and manipulate data effectively, which will allow you to efficiently handle tasks ranging from simple transformations to complex data wrangling.
One of the fundamental tools for data manipulation in Bash is the use of built-in commands such as cut
, sort
, uniq
, and paste
. These commands can be combined in various ways to filter and transform data streams. For instance, if you have a text file where each line represents a record with fields separated by commas, you can use cut
to extract specific fields:
cut -d',' -f1,3 data.csv
In the example above, the -d
option specifies the delimiter (a comma, in this case), while -f1,3
indicates that we want to extract the first and third fields from each line.
Sorting data is another common manipulation task. The sort
command can organize data based on different criteria, such as numerical or lexicographical order. Think a scenario where you want to sort a list of names:
sort names.txt
If you need to sort numerically, you can use the -n
option:
sort -n numbers.txt
To find unique entries in sorted data, the uniq
command comes into play. It’s often used in combination with sort
:
sort data.txt | uniq
This command sorts the data and then filters out duplicate lines, leaving you with a clean list of unique entries.
Another useful command is paste
, which allows you to merge lines from multiple files. For example, if you have two files and you want to combine them side-by-side, you can do the following:
paste file1.txt file2.txt
In addition to these commands, you can leverage loops and conditional statements in Bash to create more complex data manipulation scripts. For example, using a while
loop to read through a file line by line allows you to apply specific transformations or checks on each line. Here’s a simple script that processes a text file, converting each line to uppercase:
#!/bin/bash while read line; do echo "$line" | tr '[:lower:]' '[:upper:]' done < input.txt
This script employs the tr
command to transform all lowercase letters to uppercase, showcasing how Bash can be harnessed for line-by-line data processing.
These are just a few of the many data manipulation techniques available in Bash. The flexibility and power of these commands, combined with the ability to script complex workflows, make Bash an invaluable tool for data analysis tasks.
Automating Data Processing with Scripts
Automating data processing tasks in Bash can significantly enhance efficiency and accuracy. By writing scripts, you can streamline repetitive tasks that would otherwise require manual intervention, saving both time and effort. The beauty of automation lies in its ability to execute complex sequences of commands with a single command, allowing for the rapid processing of data.
To get started, you can write a simple Bash script that processes a dataset, applies transformations, and generates outputs. Ponder the following example, which reads a CSV file, filters specific columns, sorts the data, and outputs the results to a new file:
#!/bin/bash # Define input and output files input_file="data.csv" output_file="sorted_output.txt" # Extract the first and third columns, sort, and save to output file cut -d',' -f1,3 "$input_file" | sort > "$output_file" echo "Data processing complete. Results saved to $output_file."
In this script, the input file is specified, and the output file is where the processed data will be stored. The cut command extracts the desired columns, and the sort command organizes them before writing the final output. This example showcases how automating a sequence of commands can be achieved in a compact format.
Moreover, you can enhance your scripts by adding error handling and input validation to make them robust. For instance, checking if the input file exists before processing can prevent runtime errors:
#!/bin/bash # Define input and output files input_file="data.csv" output_file="sorted_output.txt" # Check if input file exists if [[ ! -f "$input_file" ]]; then echo "Error: Input file '$input_file' does not exist." exit 1 fi # Extract the first and third columns, sort, and save to output file cut -d',' -f1,3 "$input_file" | sort > "$output_file" echo "Data processing complete. Results saved to $output_file."
This addition ensures that the script gracefully handles the case where the input file might not be present, thereby enhancing its reliability.
Another advantage of automation is the ability to parameterize your scripts to handle different datasets or configurations without requiring code changes. This can be achieved by passing arguments to the script:
#!/bin/bash # Check for the correct number of arguments if [[ $# -ne 2 ]]; then echo "Usage: $0 input_file output_file" exit 1 fi # Assign input parameters to variables input_file="$1" output_file="$2" # Extract the first and third columns, sort, and save to output file cut -d',' -f1,3 "$input_file" | sort > "$output_file" echo "Data processing complete. Results saved to $output_file."
In this script, the user provides the input and output file names as command-line arguments. The script checks for the correct number of arguments and then processes the specified input file, demonstrating a more flexible approach to automation.
By using the power of Bash scripting for automation, you can efficiently manage your data processing tasks, reduce the risk of human error, and free up valuable time for deeper data analysis. This approach not only enhances productivity but also allows for reproducibility in data workflows, a critical aspect of data analysis.
Using AWK and Sed for Text Analysis
When it comes to advanced text analysis in Bash, two powerhouse utilities stand out above the rest: AWK and Sed. These tools provide unparalleled flexibility and efficiency for processing text data, allowing users to perform complex operations with minimal code.
AWK is a domain-specific language that excels at pattern scanning and processing. It operates on the principle of reading data line by line, splitting each line into fields based on a specified delimiter, and applying user-defined actions. The structure of an AWK command typically looks like this:
awk '{ action }' file
For example, if you have a CSV file and want to print only the second and fourth columns, you can use:
awk -F',' '{ print $2, $4 }' data.csv
In this command, -F’,’ sets the field delimiter to a comma, and { print $2, $4 } specifies the action to print the second and fourth fields. AWK also allows for conditional expressions, making it a powerful tool for filtering data. For instance, if you want to print records where the value in the second column is greater than 100, you can do:
awk -F',' '$2 > 100 { print }' data.csv
This command illustrates how you can seamlessly integrate logical conditions into your data processing flows, making AWK a critical ally when handling large datasets.
On the other hand, Sed, short for stream editor, is designed for parsing and transforming text in a pipeline. It excels at substitution and can manipulate text in powerful ways. A basic structure of a Sed command is as follows:
sed 's/pattern/replacement/' file
To illustrate, if you want to replace all occurrences of a word in a text file, the command could look like this:
sed 's/oldword/newword/g' file.txt
Here, /g at the end of the substitution command ensures that all occurrences of oldword are replaced with newword throughout the file. Sed can also be used for more complex manipulations, such as deleting lines, inserting new lines, and using regular expressions to match patterns.
Combining these two tools can yield even more powerful results. For example, if you have a log file and need to extract specific timestamps while keeping only those entries that contain a certain keyword, you could first use AWK to filter the logs and then pipe the result into Sed for formatting:
awk '/keyword/ { print $1, $2 }' log.txt | sed 's/2023/2024/g'
In this example, AWK filters lines containing “keyword” and prints the first two fields (likely a timestamp), while Sed modifies any occurrence of “2023” to “2024” in the output. This layered approach exemplifies the versatility of using AWK and Sed together for efficient text analysis.
Moreover, both AWK and Sed can be used in scripts to automate text transformation processes. For instance, you can create a script that processes multiple files using AWK and formats the output with Sed:
#!/bin/bash for file in *.log; do awk '/keyword/ { print $1, $2 }' "$file" | sed 's/somePattern/replacement/g' > "${file%.log}_processed.txt" done
In this script, each .log file in the directory is processed to extract relevant timestamps with AWK, and then those timestamps are formatted with Sed before being saved to a new file. This illustrates a streamlined approach to bulk text processing, showcasing the efficiency of Bash for data analysis tasks.
Handling CSV Files with Bash
Handling CSV files in Bash requires a strategic approach as these files often serve as the backbone for data analysis tasks. CSV (Comma-Separated Values) files are commonly used due to their simplicity and widespread compatibility with various data processing tools. However, manipulating CSV data effectively demands familiarity with Bash commands and scripting techniques to ensure smooth processing without errors.
One of the primary challenges when dealing with CSV files is correctly parsing the data, especially when fields may contain embedded commas or newline characters. To begin with the basics, you can utilize the cut
command to extract specific fields, as shown earlier. But when you need to handle more complex scenarios, tools like awk
and sed
can prove invaluable.
Using awk
, you can easily process CSV data by defining the delimiter. Here’s an example that demonstrates how to read a CSV file while handling quotes around fields:
awk -F',' '{gsub(/"/, "", $1); print $1, $3}' data.csv
In this command, -F','
specifies that the fields are separated by commas. The gsub(/"/, "", $1)
function removes any double quotes that might surround the data in the first field, making it cleaner for output.
For more refined control, especially when working with CSV files that may have uneven columns, error handling is essential. A simple way to check if a CSV file adheres to expected formatting is to examine the number of fields in each line:
awk -F',' 'NF != expected_fields {print "Line " NR " has " NF " fields instead of " expected_fields}' data.csv
In this snippet, replace expected_fields
with the number of fields you anticipate in each row. This command will alert you to any discrepancies, which will allow you to address issues before further processing.
Next, when you want to perform more significant transformations, like filtering or aggregating data based on specific conditions, awk
can also facilitate this. For instance, if you want to sum a particular numeric column based on a condition in another column, ponder the following example:
awk -F',' '$2 == "ConditionValue" {sum += $3} END {print sum}' data.csv
This command checks if the second field matches a specific condition and accumulates the values of the third field, printing the result at the end. Such aggregations are common in data analysis and can be done in just a single command line.
Sed is equally powerful when it comes to modifying CSV data. For example, if you want to anonymize sensitive information by replacing names with placeholders, you could use:
sed 's/Neil Hamilton/REDACTED/g' data.csv
This command will find all instances of “Neil Hamilton” in your CSV and replace them with “REDACTED,” ensuring that sensitive data is handled appropriately.
When it comes to outputting processed data, you might want to write your results to a new CSV file to avoid overwriting the original dataset. The redirection operator (>
) is useful here:
awk -F',' '{print $1, $3}' data.csv > output.csv
To combine both awk
and sed
in a single pipeline for more complex processing, think the following example, which first filters and then formats the data:
awk -F',' '$2 == "Active" {print}' data.csv | sed 's/,/;/g' > active_users.csv
This command filters for rows where the second column has the value “Active,” and then uses sed
to replace commas with semicolons in the output, which can be useful for subsequent processing or to meet specific formatting requirements.
Ultimately, effectively handling CSV files in Bash involves a combination of commands and techniques tailored to the specific data and analysis requirements. The versatility of tools like awk
and sed
empowers users to manipulate and analyze CSV data with precision, ensuring that they can extract meaningful insights efficiently.
Visualizing Data Outputs with Bash Tools
Visualizing data outputs in Bash can be a fulfilling yet challenging task. While Bash is not traditionally seen as a data visualization tool, it provides a variety of utilities that can help you generate visual representations of your data directly from the command line. This capability is particularly useful for quickly assessing data trends or patterns without the overhead of a graphical interface.
One of the simplest ways to visualize data in Bash is through the use of text-based plots. Tools such as gnuplot
and plotutils
allow you to create charts and graphs from data files. For instance, gnuplot
can read data from a file and generate plots based on specified commands. Here’s an example of how you can use gnuplot
to plot a simple line graph from a data file:
echo -e "1 1n2 4n3 9n4 16n5 25" > data.txt gnuplot -e "set terminal png; set output 'plot.png'; plot 'data.txt' using 1:2 with lines"
In this example, we create a simple dataset in data.txt
that represents a series of squares. The gnuplot
command specifies that the output should be a PNG image and then plots the data using the first column for the x-axis and the second column for the y-axis. The result is a visual representation of the data saved as plot.png
.
Another effective way to visualize data is by using histogram-like representations in the terminal. You can employ the awk
command to summarize data and generate a simple text-based histogram. For instance, consider the following approach to visualize the frequency of occurrences of values in a dataset:
awk '{count[$1]++} END {for (word in count) {printf "%s: ", word; for (i=0; i<count[word]; i++) printf "#"; print ""}}' data.txt
This command reads through data.txt
, counts occurrences of each unique value, and outputs a simple histogram with each value followed by a series of hash symbols representing its frequency. Such a quick visualization directly in the terminal can be incredibly useful for performing initial data assessments.
For more advanced visualization needs, you can leverage additional tools like R
or Python
via Bash scripts. You can call R scripts from within Bash using the Rscript
command, enabling you to produce high-quality plots with packages like ggplot2
. Here’s a simplified example:
echo -e "x,yn1,1n2,4n3,9n4,16n5,25" > data.csv Rscript -e "library(ggplot2); data <- read.csv('data.csv'); ggplot(data, aes(x=x, y=y)) + geom_line()"
This command first creates a CSV file with the data. The subsequent Rscript
command loads the data into R and generates a line plot using ggplot2
. This approach allows for much more sophisticated visualization capabilities than what is typically possible with Bash alone.
Finally, if you require a simple approach to visualize text output, you can use the column
command to format data neatly in tabular form. This can be especially helpful when dealing with structured datasets, making them easier to read at a glance:
column -t -s',' < data.csv
In this command, the -t
option creates a table and the -s'
option specifies that the input is comma-separated. The result is a well-aligned table in the terminal, facilitating quick visual checks for anomalies or trends in the data.
Although Bash may not be the first choice for data visualization, it offers a variety of tools and techniques that can be effectively harnessed to create meaningful visual outputs from your data analysis workflows. By combining these techniques with the power of external tools, you can achieve a satisfactory level of visualization directly from the command line.