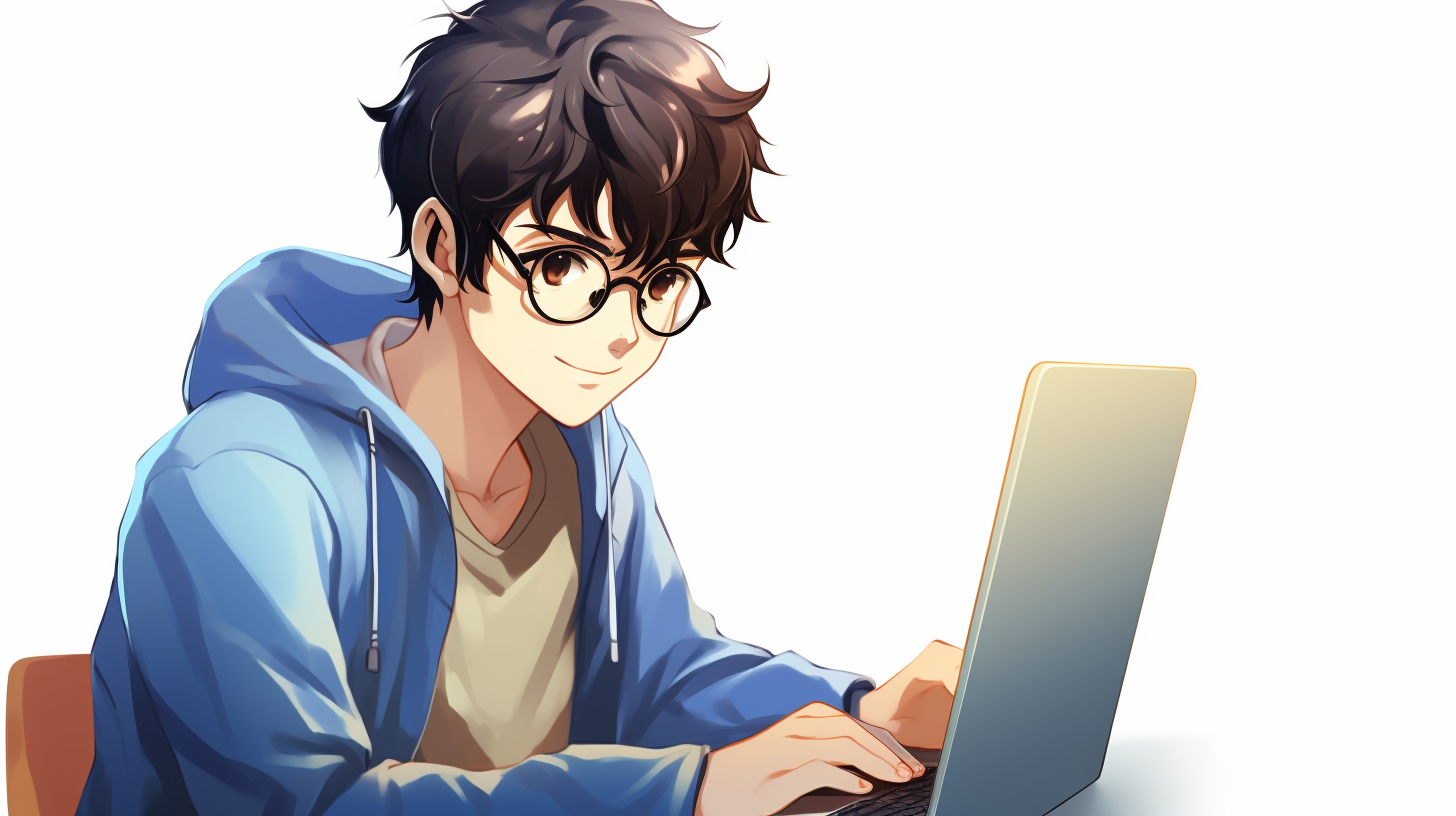
SQL for Data Encryption and Security
Data encryption is a fundamental aspect of data security, particularly in SQL databases, where sensitive information must be safeguarded against unauthorized access. Understanding the various data encryption techniques available in SQL is essential for ensuring that data remains confidential and protected throughout its lifecycle.
SQL databases typically employ two main types of encryption: Data-at-Rest Encryption and Data-in-Transit Encryption. Each serves a distinct purpose and can be implemented using various methods.
-
Data-at-Rest Encryption: This technique protects data stored on disk. It ensures that even if an unauthorized person gains access to the physical storage, the data remains unreadable without the appropriate decryption keys.
-
Transparent Data Encryption (TDE): TDE encrypts the entire database at the file level. This means that the encryption process is transparent to the applications and end-users.
CREATE DATABASE MyEncryptedDB WITH ENCRYPTION (ALGORITHM = AES_256, SERVER ENCRYPTION = ON);
-
Column-Level Encryption: This allows specific columns within a table to be encrypted, providing more granular control.
CREATE TABLE Users ( UserID INT PRIMARY KEY, UserName NVARCHAR(100), UserPassword VARBINARY(128) ENCRYPTED WITH (KEY = MyEncryptionKey) );
-
Transparent Data Encryption (TDE): TDE encrypts the entire database at the file level. This means that the encryption process is transparent to the applications and end-users.
-
Data-in-Transit Encryption: This encryption secures data as it travels over the network. Implementing this ensures that sensitive information is not intercepted by malicious actors during transmission.
-
SSL/TLS Encryption: Most modern SQL servers support SSL (Secure Sockets Layer) or TLS (Transport Layer Security) to encrypt data-in-transit.
-- Example: Enabling SSL on a SQL Server connection string Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;Encrypt=true;TrustServerCertificate=true;
-
SSL/TLS Encryption: Most modern SQL servers support SSL (Secure Sockets Layer) or TLS (Transport Layer Security) to encrypt data-in-transit.
When implementing these encryption techniques, it’s important to manage encryption keys securely. Key management solutions should be utilized to protect, rotate, and audit keys while ensuring they are accessible only to authorized personnel.
Implementing Row-Level Security in SQL
Row-Level Security (RLS) is a powerful feature in SQL that enables fine-grained access control to rows within a database table based on user roles and attributes. Implementing RLS allows organizations to enforce security policies at the row level, ensuring that users can only view or modify data that they are authorized to access. This approach is particularly beneficial in multi-tenant applications or environments where sensitive information must be segregated based on user context.
To implement Row-Level Security in SQL Server, you first need to create a security policy and a predicate function that determines whether a user has access to a specific row. The following steps outline the implementation process:
-- Step 1: Create a sample table CREATE TABLE EmployeeData ( EmployeeID INT PRIMARY KEY, EmployeeName NVARCHAR(100), Department NVARCHAR(50), Salary DECIMAL(10, 2) ); -- Step 2: Insert sample data INSERT INTO EmployeeData (EmployeeID, EmployeeName, Department, Salary) VALUES (1, 'Alice', 'HR', 60000), (2, 'Bob', 'Finance', 70000), (3, 'Charlie', 'IT', 80000), (4, 'David', 'HR', 65000); -- Step 3: Create a user-defined function to filter rows CREATE FUNCTION Security.fn_securitypredicate(@Department AS NVARCHAR(50)) RETURNS TABLE WITH SCHEMABINDING AS RETURN SELECT 1 AS result WHERE @Department = USER_NAME(); -- Assuming USER_NAME() returns the user's department -- Step 4: Create a security policy that applies the predicate function CREATE SECURITY POLICY EmployeeSecurityPolicy ADD filter predicate Security.fn_securitypredicate(Department) ON dbo.EmployeeData WITH (STATE = ON);
In this example, we begin by creating a sample table named EmployeeData that contains employees’ details. We then insert some sample data into the table. The core of RLS implementation lies in the user-defined function fn_securitypredicate, which filters the rows based on the department associated with the current user.
Once the function is defined, we create a security policy called EmployeeSecurityPolicy that applies the filtering logic to the EmployeeData table. The result is that users will only see rows where the Department matches their user context.
Testing the implementation is important to ensure that RLS behaves as expected. You can run the following queries to see how different users access the data:
-- Simulate user 'HR' EXECUTE AS USER = 'HR'; SELECT * FROM EmployeeData; -- Should return Alice and David -- Revert back to original user REVERT; -- Simulate user 'Finance' EXECUTE AS USER = 'Finance'; SELECT * FROM EmployeeData; -- Should return only Bob -- Revert back to original user REVERT;
By using the EXECUTE AS statement, you can impersonate different users to test the row-level security policy. This method ensures that users only see rows they’re authorized to access, thus enhancing the overall security of the database.
Best Practices for SQL Security Management
When it comes to securing SQL databases, best practices play a pivotal role in fortifying the data against unauthorized access and potential breaches. Here are several key strategies that organizations should adopt for effective SQL security management:
1. Principle of Least Privilege
Adhering to the principle of least privilege (PoLP) ensures that users are granted only those permissions necessary for their job functions. This minimizes the risk of accidental or malicious data exposure. Regular audits should be conducted to review user access levels and adjust them as necessary.
-- Example: Revoking unnecessary permissions REVOKE SELECT, INSERT, DELETE ON Employees FROM UserName;
2. Strong Authentication Mechanisms
Implementing strong authentication methods, such as multi-factor authentication (MFA), adds an additional layer of security. This means that even if a user’s password is compromised, unauthorized access to the database can be prevented.
-- Example: Enforcing strong password policies in SQL Server ALTER LOGIN UserName WITH CHECK_POLICY = ON;
3. Regular Backups and Recovery Plans
Regularly scheduled backups are essential for ensuring data integrity and availability. In the event of data loss or corruption, a robust recovery plan allows organizations to restore their databases to a secure state without significant downtime.
-- Example: Backing up a database BACKUP DATABASE MyDatabase TO DISK = 'C:BackupsMyDatabase.bak';
4. Encryption Practices
As previously discussed, encryption both at rest and in transit is critical. SQL databases should leverage built-in encryption features such as Transparent Data Encryption (TDE) and column-level encryption, ensuring that sensitive data remains protected throughout its lifecycle.
-- Example: Encrypting specific columns ALTER TABLE Users ALTER COLUMN UserPassword ADD ENCRYPTED WITH (KEY = MyEncryptionKey);
5. Update and Patch Management
Keeping the SQL server and any associated applications up to date with the latest security patches is vital. Many breaches exploit known vulnerabilities that could easily be mitigated through timely updates.
-- Example: Checking for updates on SQL Server EXEC sp_helpserver;
6. Security Auditing and Monitoring
Implementing comprehensive auditing and monitoring systems can alert administrators to suspicious activities. SQL Server provides features such as SQL Server Audit, which allows tracking of changes to data and user access patterns.
-- Example: Creating an audit specification CREATE SERVER AUDIT MyAudit TO FILE (FILEPATH = 'C:AuditLogs'); CREATE DATABASE AUDIT SPECIFICATION MyDatabaseAudit FOR SERVER AUDIT MyAudit ADD (SELECT, INSERT, UPDATE, DELETE ON dbo.Users BY public) WITH (STATE = ON);
7. Application Security
Ensuring that applications interfacing with the SQL database are secure is just as important as securing the database itself. Use parameterized queries to prevent SQL injection attacks, and regularly review application code for vulnerabilities.
-- Example: Using parameterized queries in a stored procedure CREATE PROCEDURE GetUser @UserID INT AS BEGIN SELECT * FROM Users WHERE UserID = @UserID; END;
Auditing and Monitoring SQL Database Security
Auditing and monitoring SQL database security is a critical aspect of maintaining data integrity and confidentiality, ensuring compliance with regulations, and detecting potential security breaches. Effective auditing provides insights into user activities and system changes, while monitoring establishes a real-time view of the database environment, enabling administrators to respond to threats promptly.
SQL Server offers robust auditing features that allow organizations to track and log changes to data and user access patterns. One of the key tools available for this purpose is SQL Server Audit, which provides a structured way to create and manage audit specifications for both server and database-level activities.
To implement auditing, you can follow these steps:
-- Step 1: Create a server audit which defines the audit destination CREATE SERVER AUDIT MyAudit TO FILE (FILEPATH = 'C:AuditLogs', MAXSIZE = 10 MB, MAX_ROLLBACKS = 5) WITH (STATE = ON); -- Step 2: Create a database audit specification to log specific actions CREATE DATABASE AUDIT SPECIFICATION MyDatabaseAudit FOR SERVER AUDIT MyAudit ADD (SELECT, INSERT, UPDATE, DELETE ON dbo.Users BY public) -- Adjust actions and targets as needed WITH (STATE = ON);
In this example, we first create a server audit named MyAudit
, which specifies where the audit logs will be stored. The audit is configured to log to a file with a maximum size and retention policy to prevent excessive use of storage. Next, we define a database audit specification named MyDatabaseAudit
that captures various data manipulation actions on the Users
table. This specification is linked to the server audit we previously created.
Once auditing is set up, you can query the audit logs to review actions taken against your database, which is important for identifying unauthorized access attempts or changes to sensitive data:
-- Querying audit logs SELECT * FROM sys.fn_get_audit_file('C:AuditLogs*', DEFAULT, DEFAULT);
This query allows you to retrieve records from the audit logs, providing visibility into the logged activities. Regularly reviewing these logs enables database administrators to spot anomalies and take action against potential security threats.
Moreover, monitoring SQL databases goes beyond auditing alone; it involves observing performance metrics, user activities, and system health. Tools such as SQL Server Profiler and built-in Dynamic Management Views (DMVs) can assist in monitoring database performance and security.
For instance, you can use DMVs to assess user activity and lock contention:
-- Check active user sessions SELECT session_id, login_name, status, last_request_start_time FROM sys.dm_exec_sessions WHERE is_user_process = 1; -- Monitor lock contention SELECT resource_type, request_mode, request_session_id, blocking_session_id FROM sys.dm_tran_locks;
These queries give insight into who is currently connected to the SQL Server and any lock contention issues that might be impacting performance. Regular monitoring aids in identifying trends and potential vulnerabilities, allowing organizations to proactively strengthen their database security measures.