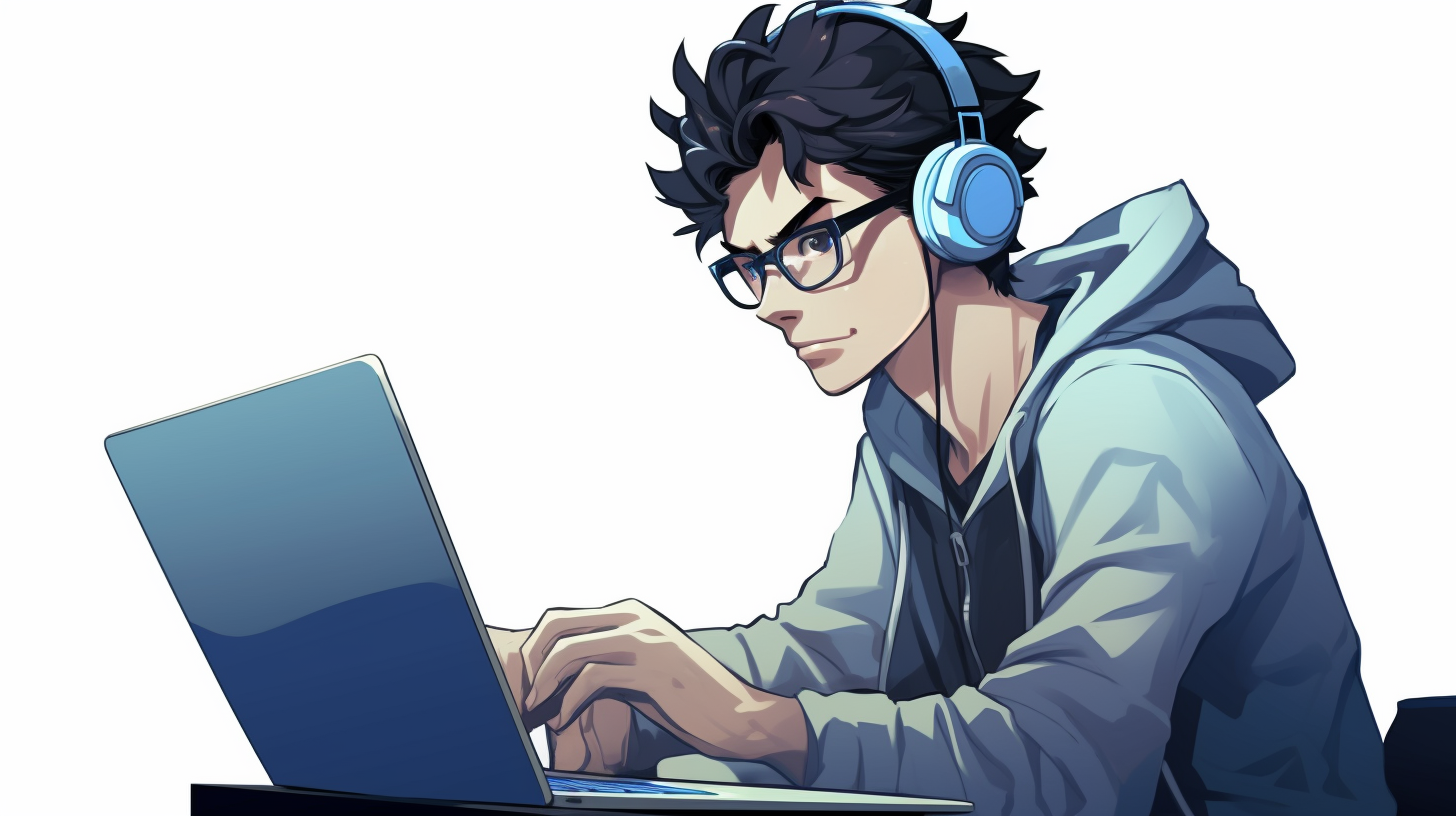
Structs in Swift
Swift structs are powerful building blocks that allow you to encapsulate data and functionality in a single, cohesive entity. Unlike classes, structs in Swift are value types, meaning that they are copied when they’re passed around in your code. This fundamental difference in behavior can lead to more predictable and safer code, particularly in concurrent programming scenarios.
Structs can encapsulate both properties and methods, making them versatile for modeling various kinds of data structures. They’re often used in Swift for data representation, using their compactness and ease of use. For example, a simple 2D point can be represented as a struct:
struct Point { var x: Double var y: Double }
In this example, the Point
struct has two properties, x
and y
, both of which are of type Double
. By using a struct, you can easily create instances representing specific points with clear and concise syntax.
Structs are also equipped with automatic memberwise initializers, which allow you to create new instances without needing to define a separate init method:
let pointA = Point(x: 1.0, y: 2.0) let pointB = Point(x: 3.0, y: 4.0)
When you work with structs, you gain the benefit of value semantics. This means that when you assign a struct instance to another variable or pass it into a function, a copy is made. This behavior can help prevent unintended side effects that may arise from shared state, a common risk in reference types like classes.
For example, ponder the following code:
var originalPoint = Point(x: 1.0, y: 2.0) var copiedPoint = originalPoint copiedPoint.x = 5.0 print(originalPoint.x) // Output: 1.0 print(copiedPoint.x) // Output: 5.0
In this snippet, modifying copiedPoint
does not affect originalPoint
, showcasing the independence of value types. This property of structs not only maintains data integrity but also simplifies reasoning about code behavior, especially as programs grow in complexity.
Structs can also conform to protocols, enabling polymorphism and allowing for the creation of generic and reusable code components. The combination of encapsulation, value semantics, and protocol conformance makes structs a foundational aspect of Swift programming, providing a robust framework for managing data and behaviors together.
Defining and Initializing Structs
Defining a struct in Swift is simpler. You use the struct
keyword followed by the name of the struct and a set of curly braces to enclose its properties and methods. By convention, struct names start with an uppercase letter, which helps distinguish them from variable names. Here’s a simple example that illustrates how to define a struct for a rectangle:
struct Rectangle { var width: Double var height: Double func area() -> Double { return width * height } }
In this example, the Rectangle
struct has two properties: width
and height
, both of type Double
. It also includes a method called area()
that calculates and returns the area of the rectangle. This method can operate on the struct’s properties, reflecting a clear relationship between data and behavior.
When you instantiate a struct, you can do so using the memberwise initializer that Swift provides by default. This allows you to create a new instance of the Rectangle
struct and specify its properties at the same time:
let myRectangle = Rectangle(width: 5.0, height: 10.0)
After creating an instance, you can access its properties and methods easily:
print("Width: (myRectangle.width)") // Output: Width: 5.0 print("Height: (myRectangle.height)") // Output: Height: 10.0 print("Area: (myRectangle.area())") // Output: Area: 50.0
This succinct approach to defining and initializing structs is one of the reasons Swift is highly favored for developing robust applications. It is also worth noting that you can define default values for properties in your structs. This allows you to create instances without having to specify all property values explicitly, providing flexibility in your code design:
struct Circle { var radius: Double = 1.0 func area() -> Double { return .pi * radius * radius } }
Here, the Circle
struct has a default value for radius
. When you create a new instance, you can either use the default or specify a different value:
let defaultCircle = Circle() let customCircle = Circle(radius: 3.0) print("Default Circle Area: (defaultCircle.area())") // Output: Default Circle Area: 3.141592653589793 print("Custom Circle Area: (customCircle.area())") // Output: Custom Circle Area: 28.274333882308138
Such flexibility streamlines the initialization process, making it easier to manage complex data types in your applications. This harmonious balance of simplicity and functionality is what makes defining and initializing structs in Swift not just effective, but enjoyable for developers.
Properties and Methods in Structs
When diving deeper into Swift structs, it is crucial to understand how properties and methods function within these constructs. Structs can store various types of data in their properties, which can be defined as variables (using the keyword var
) or constants (using the keyword let
). This allows for a nuanced control over the mutability of struct instances.
Properties in a struct can also have default values, making it easier to create instances without providing values for every property upon initialization. For instance, here’s how you can create a struct representing a car:
struct Car { var make: String var model: String var year: Int = 2023 // Default value for year func description() -> String { return "(year) (make) (model)" } }
In this Car
struct, the year
property has a default value. This means if you create a new Car
instance without explicitly specifying the year, it will default to 2023. Here’s how to instantiate it:
let myCar = Car(make: "Toyota", model: "Camry") print(myCar.description()) // Output: 2023 Toyota Camry
Notice how the description
method provides a clear textual representation of the car instance. This encapsulation of behavior with data is what makes structs particularly powerful in Swift.
Additionally, structs can have computed properties, which do not store a value but instead provide a getter and an optional setter to indirectly access and modify other properties. Ponder the following example:
struct Rectangle { var width: Double var height: Double var area: Double { return width * height } }
In this Rectangle
struct, area
is a computed property that dynamically calculates the area whenever it’s accessed. You do not need to call a method to get the area; instead, you can access it as if it were a property:
let myRectangle = Rectangle(width: 5.0, height: 10.0) print("Area: (myRectangle.area)") // Output: Area: 50.0
Swift structs also support methods that can modify their properties. However, such methods must be marked with the mutating
keyword, signaling that the method will change the instance itself. Here’s a quick example:
struct Counter { var count: Int = 0 mutating func increment() { count += 1 } }
To use the increment
method, you must declare the instance as mutable:
var myCounter = Counter() myCounter.increment() print(myCounter.count) // Output: 1
This ability to modify properties through methods enhances the encapsulation of behavior within your structs, maintaining a clear and manageable interface for interacting with the data. By using properties and methods together, Swift structs become a powerful tool for creating self-contained, modular components in your applications. This balance of simplicity and functionality is at the heart of effective Swift programming.
Value Semantics and Mutability in Structs
Value semantics are a hallmark of Swift structs, profoundly influencing how data is managed and manipulated throughout your application. When you declare a struct, you are not just creating a collection of properties and methods; you’re establishing a distinct entity that carries its own state. This distinction becomes particularly important when you ponder how these entities behave under various operations, such as assignment and function passing.
When you assign one struct to another, as previously mentioned, you’re working with a copy. This is a critical concept in understanding value semantics. For instance, if you define a struct representing a book:
struct Book { var title: String var author: String }
And then instantiate a book:
var originalBook = Book(title: "1984", author: "George Orwell") var copiedBook = originalBook
At this point, originalBook and copiedBook are independent of one another. If you modify copiedBook:
copiedBook.title = "Animal Farm"
The original instance remains unchanged:
print(originalBook.title) // Output: 1984 print(copiedBook.title) // Output: Animal Farm
This behavior not only fosters reliability but also simplifies debugging, as you don’t have to track the effects of shared state across different parts of your application.
However, while this value semantics is powerful, mutability within structs requires careful consideration. Even though structs are value types, it is possible to create mutable instances by marking them with the var keyword. Here’s an example:
struct Person { var name: String var age: Int mutating func birthday() { age += 1 } } var person = Person(name: "Alice", age: 30) person.birthday() print("Name: (person.name), Age: (person.age)") // Output: Name: Alice, Age: 31
In this case, the birthday method is marked as mutating, allowing it to modify the instance of the struct directly. That is an important distinction because methods in structs cannot change properties unless they’re explicitly designated as mutating. You must declare an instance as mutable by using var, enabling such manipulation.
While this might seem simpler, it brings to light an essential aspect of value semantics: the immutability by default of structs. If you were to create an instance using let instead of var, you would not be able to call any mutating methods:
let immutablePerson = Person(name: "Bob", age: 25) // immutablePerson.birthday() // This will produce a compile-time error
This serves to enforce a disciplined approach to data handling, ensuring that the programmer consciously opts for mutability when appropriate. By leaning into this paradigm, Swift encourages developers to ponder critically about state management and its implications on software design.
Value semantics and mutability in structs are not just technical details; they form the bedrock of Swift’s approach to safety and manageability in programming. Whether you are working with simple data structures or complex data models, understanding these concepts allows you to write clearer, more reliable code that stands the test of time.