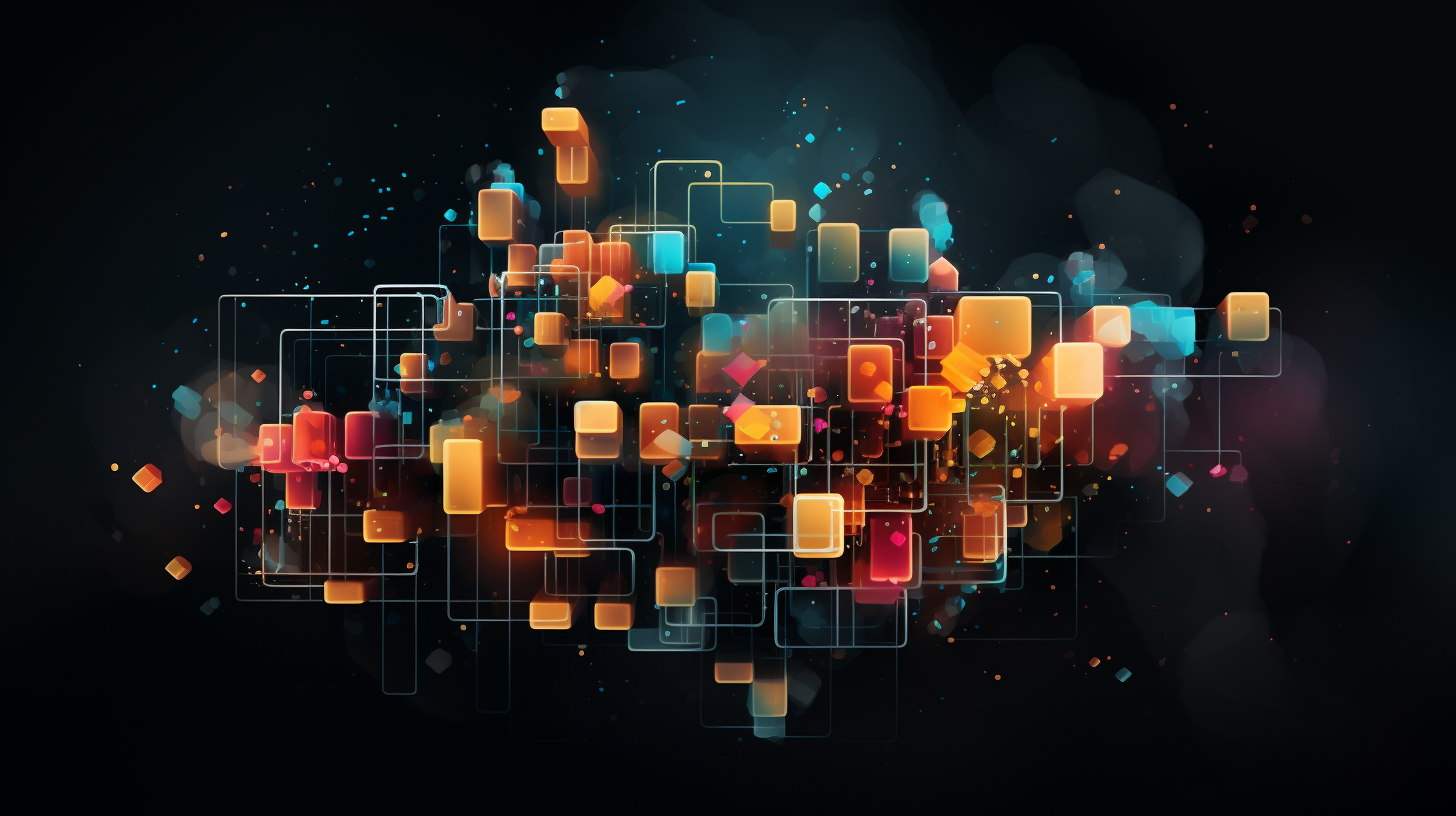
Error Handling in Python with Try-Except
Error handling is an essential aspect of robust programming, and in Python, it is primarily managed through the use of exceptions. When a Python program encounters an error, it raises an exception, which is an object representing the error. If not properly handled, these exceptions can cause the program to terminate abruptly, potentially leading to data loss or corruption. Understanding the basics of error handling is important for developing applications that can gracefully handle unexpected issues.
In Python, errors are categorized into two main types: syntax errors and exceptions. Syntax errors occur when the Python parser encounters code that does not conform to the language’s grammar. These errors are typically caught before the program runs. On the other hand, exceptions are raised during runtime, allowing the program to continue executing after handling the error appropriately.
To illustrate the difference, think the following examples:
# Syntax Error Example print("Hello, World!"
# Exception Example number = int(input("Enter a number: ")) result = 10 / number print("Result is:", result)
In the first snippet, a syntax error occurs because of a missing parenthesis, which prevents the program from running. In the second snippet, if the user enters 0, a runtime exception (specifically, a ZeroDivisionError) will be raised when attempting to divide by zero. This exemplifies the need for error handling to manage such scenarios effectively.
Python provides a built-in mechanism to catch and handle exceptions, allowing developers to write cleaner, more resilient code. By using the try statement, we can attempt to execute a block of code that might raise an exception. If an exception occurs, control is transferred to an associated except block where the error can be handled without crashing the program.
Here’s a simple example demonstrating how to handle an exception using a try-except block:
try: number = int(input("Enter a number: ")) result = 10 / number print("Result is:", result) except ValueError: print("That's not a valid number!") except ZeroDivisionError: print("You can't divide by zero!")
In this example, if the user enters something that isn’t a number, a ValueError is caught, and an appropriate message is printed. Similarly, if the user inputs zero, the ZeroDivisionError is caught before it can crash the program. This structure allows for a more controlled response to errors, enhancing the user experience and maintaining application stability.
Understanding Exceptions
Understanding exceptions is critical when developing in Python, as they serve as the primary mechanism for signaling errors and unexpected situations. Exceptions are not merely indicators of failure; they encapsulate valuable information about the state of the program at the moment an error occurs. Each exception object contains attributes that can provide context, such as the type of error and a message detailing what went wrong.
Python’s exception hierarchy is organized in a way that allows developers to catch broad categories of errors or specific ones, depending on their needs. The base class for all exceptions in Python is BaseException
, but the more commonly used base class is Exception
. Understanding the different exceptions available in Python is essential for effective error handling.
For instance, the built-in exceptions include AttributeError
, IOError
, and IndexError
, among others, each representing a specific type of error condition. This categorization allows developers to tailor their exception handling to the types of errors they anticipate. Ponder the following:
try: my_list = [1, 2, 3] print(my_list[5]) except IndexError as e: print(f"Index error occurred: {e}")
In this example, attempting to access an index that does not exist raises an IndexError
. By catching this specific exception, the developer can provide a meaningful response, such as logging the error or notifying the user, while preventing the application from crashing.
Another key aspect of exceptions is that they can be raised deliberately using the raise
statement. This functionality allows developers to create custom error messages or define their exceptions, enhancing the robustness of their applications. For example:
def divide(a, b): if b == 0: raise ValueError("The denominator cannot be zero.") return a / b try: result = divide(10, 0) except ValueError as e: print(e)
In the divide
function, an error condition is checked, and a ValueError
is raised if the denominator is zero. This pattern is particularly useful for validating inputs and ensuring that functions are used correctly, allowing the rest of the program to operate with the assumption that inputs are valid.
By mastering the understanding of exceptions, programmers can create more maintainable code that not only handles errors gracefully but also improves the overall user experience. This capability is especially important in larger applications where the complexity and potential for errors increase significantly.
Using Try-Except Blocks
To utilize try-except blocks effectively, it’s essential to grasp how to implement them in various scenarios. The structure of a try-except block is straightforward: the code that may raise an exception is placed within the try
section, while the handling of the exception occurs in the except
section. This design empowers developers to manage errors without disrupting the flow of the program.
Here’s a more detailed example illustrating the use of try-except blocks in practice:
try: file = open("example.txt", "r") content = file.read() print(content) except FileNotFoundError: print("The file was not found. Please check the file path and try again.") except IOError: print("An I/O error occurred. Please check your permissions or the file system.") finally: if 'file' in locals(): file.close()
In this example, the program attempts to open and read a file named example.txt
. If the file does not exist, a FileNotFoundError
is raised, and a easy to use message is displayed without crashing the program. If any I/O errors occur during the reading process, they’re caught by the IOError
exception. The finally
block ensures that the file is closed if it was successfully opened, maintaining good resource management practices.
It’s worth noting that you can also use a generic except
clause to catch any exception that wasn’t explicitly handled in previous clauses. However, employing broad exception handling should be done cautiously, as it may obscure bugs and lead to unintended behavior. Here’s an example:
try: result = 10 / int(input("Enter a number: ")) print("Result is:", result) except (ValueError, ZeroDivisionError) as e: print(f"An error occurred: {e}") except Exception as e: print(f"An unexpected error occurred: {e}")
In this case, both ValueError
and ZeroDivisionError
are caught and handled with a single except
clause. If any other unexpected exception occurs, it will be caught by the more general Exception
clause. This pattern can be advantageous for logging errors or raising alerts when unexpected conditions arise.
When employing try-except blocks, it’s also possible to use the else
statement alongside the try
block. The code within the else
block runs only if the try
block succeeds without raising any exceptions. This can help in organizing code better and separating error handling from normal execution:
try: number = int(input("Enter a number: ")) except ValueError: print("That's not a valid number!") else: print("You entered the number:", number)
In this example, if a ValueError
is raised, the message is printed, but if the input is valid, the program will output the entered number. This structure enhances readability and clarity in the code.
By understanding and using try-except blocks effectively, you can develop Python applications that are not only robust but also effortless to handle, minimizing the risk of program crashes and enhancing the overall user experience.
Multiple Exceptions and Catching Them
In Python, handling multiple exceptions efficiently is a critical aspect of writing resilient code. When a block of code has the potential to raise different types of exceptions, developers can catch these exceptions using multiple except clauses. This allows for precise control over how each specific error type is managed, facilitating tailored responses to various error conditions.
To illustrate the concept, ponder a scenario where a program processes user input for both a numerical value and a filename. Here, multiple issues could arise, such as invalid input for the number or a missing file. By implementing multiple except blocks, each exception can be addressed appropriately:
try: number = int(input("Enter a number: ")) with open("output.txt", "w") as file: file.write(f"The number you entered is: {number}") except ValueError: print("That's not a valid number! Please enter a numeric value.") except FileNotFoundError: print("The specified file could not be found. Please check the file name.") except IOError: print("An I/O error occurred while trying to write to the file.")
In this example, if the user enters a non-numeric value, the program will catch the ValueError
. If the program fails to locate output.txt
, it will handle the FileNotFoundError
. Additionally, any other I/O related errors encountered during the writing process will be addressed by the IOError
catch block. This structured approach provides clarity and improves the overall reliability of the application.
Moreover, Python allows you to handle multiple exceptions in a single except block by providing a tuple of exception types. This can be particularly useful when the handling logic for different exceptions is similar:
try: number = int(input("Enter a number: ")) result = 10 / number except (ValueError, ZeroDivisionError) as e: print(f"An error occurred: {e}")
In this case, both the ValueError
and ZeroDivisionError
are caught, and a unified message is printed. This method reduces redundancy in error handling, making the code cleaner while maintaining functionality.
While it’s advantageous to handle multiple exceptions, it’s essential to order the except blocks from most specific to the most general. This ensures that specific exceptions are caught before the more general ones, preventing unintended behavior. For instance, if you were to place a catch-all except Exception:
block before a specific exception, that specific exception would never be reached:
try: number = int(input("Enter a number: ")) result = 10 / number except Exception as e: print("An unexpected error occurred:", e) except ZeroDivisionError: print("You cannot divide by zero!")
In this flawed example, the program would never print a message for division by zero since the generic exception block would capture it first. Thus, always be mindful of the order in which you define your exception handlers.
Another powerful feature of Python’s exception handling is the ability to define custom exceptions. This can be particularly beneficial when building larger applications that require specific error management tailored to the application’s logic. By creating a custom exception class, developers can provide meaningful error messages and context:
class CustomError(Exception): pass try: raise CustomError("This is a custom error message.") except CustomError as e: print(f"Caught an error: {e}")
In this example, a custom exception CustomError
is raised, and the corresponding except block captures and handles this exception gracefully. This flexibility allows developers to extend Python’s error handling capabilities, enhancing the overall structure and maintainability of their code.
By using the ability to catch multiple exceptions and employing custom exceptions, developers can create robust applications that respond appropriately to a wide range of error conditions, ensuring a smooth user experience even in the face of unexpected situations.
Finally Clause and Clean-Up Actions
The finally clause in Python’s exception handling mechanism plays an important role in ensuring that certain code runs regardless of whether an exception was raised or not. This is particularly important for executing clean-up actions, such as closing files, releasing resources, or committing transactions. A finally block is guaranteed to execute after the try and except blocks, allowing developers to manage resources effectively, even in the face of errors.
Consider a scenario where you’re working with a file that needs to be read. You want to ensure that the file is closed properly, regardless of whether the reading operation succeeds or fails. The following example demonstrates this concept:
try: file = open("example.txt", "r") content = file.read() print(content) except FileNotFoundError: print("The file was not found. Please check the file path and try again.") except IOError: print("An I/O error occurred. Please check your permissions or the file system.") finally: if 'file' in locals(): file.close() print("File has been closed.")
In this example, the finally block checks if the variable file
exists in the local scope (indicating that the file was successfully opened), and if so, it closes the file. This ensures that resources are released properly, preventing potential memory leaks or file locking issues.
The finally clause is especially useful in scenarios involving network connections or database transactions. For instance, when working with a database, you may want to ensure that a transaction is either committed or rolled back depending on whether an exception occurred:
connection = None try: connection = create_database_connection() cursor = connection.cursor() # Execute some database operations cursor.execute("INSERT INTO some_table VALUES ('data')") connection.commit() except DatabaseError as e: if connection: connection.rollback() print(f"An error occurred: {e}") finally: if connection: connection.close() print("Database connection has been closed.")
In this case, if a DatabaseError
occurs while executing the SQL command, the changes made during the transaction can be rolled back, maintaining the integrity of the database. The finally block ensures that the database connection is closed, regardless of the outcome of the operations.
It’s important to note that the finally block will execute even if there is an unhandled exception in the try or except blocks. This means that it can be a helpful tool for ensuring that necessary clean-up code is run, no matter what:
try: raise ValueError("An error occurred!") except ValueError as e: print(f"Caught an error: {e}") finally: print("This will always execute.")
Here, even though a ValueError is raised and caught, the message from the finally block is printed, demonstrating its guaranteed execution.
The finally clause is an indispensable part of Python’s error handling mechanism that allows developers to ensure that necessary clean-up actions are performed. By using finally effectively, you can enhance the robustness of your applications, safeguarding against resource leaks and ensuring that your programs maintain their integrity, even in the face of errors.
Best Practices for Error Handling
When it comes to error handling in Python, adhering to best practices is essential for developing robust applications. Effective error handling not only prevents unexpected crashes but also improves the overall user experience by providing clear feedback on issues that arise. Below are some key best practices that developers should think when working with exceptions and error handling.
1. Use Specific Exceptions
One of the most fundamental principles is to catch specific exceptions rather than using a broad except clause. Catching specific exceptions allows for tailored responses to different error conditions, making it easier to diagnose problems. For example:
try: result = 10 / int(input("Enter a number: ")) except ValueError: print("That's not a valid number!") except ZeroDivisionError: print("You can't divide by zero!")
This practice enhances code clarity and prevents masking of unrelated errors, which can complicate debugging efforts.
2. Avoid Silent Failures
Silencing exceptions by using a bare except clause can lead to hidden bugs and unpredictable behavior. Always log the exception or provide feedback to the user, ensuring that issues are not overlooked:
try: process_data() except Exception as e: print(f"An error occurred: {e}") # Log the error
This way, even if an unexpected error occurs, it’s documented, making it easier to address later.
3. Clean-Up Actions with Finally
Using the finally clause especially important for managing resources. Always ensure that resources like file handles, network connections, and database transactions are properly closed or rolled back in the event of an error:
file = None try: file = open("data.txt", "r") process_file(file) except IOError as e: print(f"File error: {e}") finally: if file: file.close() print("File closed.")
By following this practice, developers can avoid resource leaks and ensure that their applications remain reliable.
4. Document Your Exceptions
When creating functions or classes that may raise exceptions, document the expected exceptions in your docstrings. This practice informs users of your code about possible error conditions, aiding in proper error handling:
def divide(a, b): """Divides two numbers. Raises: ValueError: If the denominator is zero. """ if b == 0: raise ValueError("Denominator cannot be zero.") return a / b
Clear documentation helps other developers understand the potential pitfalls when using your code.
5. Use Custom Exceptions When Necessary
In cases where built-in exceptions do not convey the necessary context, consider defining custom exception classes. Custom exceptions can encapsulate additional information, improving the clarity of error handling:
class MyCustomError(Exception): """Custom exception for specific errors in my application.""" pass try: raise MyCustomError("This is a custom error message.") except MyCustomError as e: print(f"Caught an error: {e}")
This approach can enhance maintainability and readability of the code, particularly in larger applications.
6. Testing for Exceptions
In addition to implementing error handling, it’s equally important to test for exceptions in your code. Unit tests can be designed to ensure that exceptions are raised as expected under certain conditions:
import unittest class TestMathOperations(unittest.TestCase): def test_divide_by_zero(self): with self.assertRaises(ValueError): divide(10, 0) if __name__ == '__main__': unittest.main()
By writing tests for exception scenarios, you can ensure that your error handling logic is functioning correctly and that your application behaves as intended even when faced with invalid input.
By adopting these best practices, Python developers can build applications that are not only resilient in the face of errors but also provide an enhanced user experience through clear communication and effective error management. Robust error handling is a hallmark of professional-grade software development, laying the groundwork for maintainable and reliable applications.