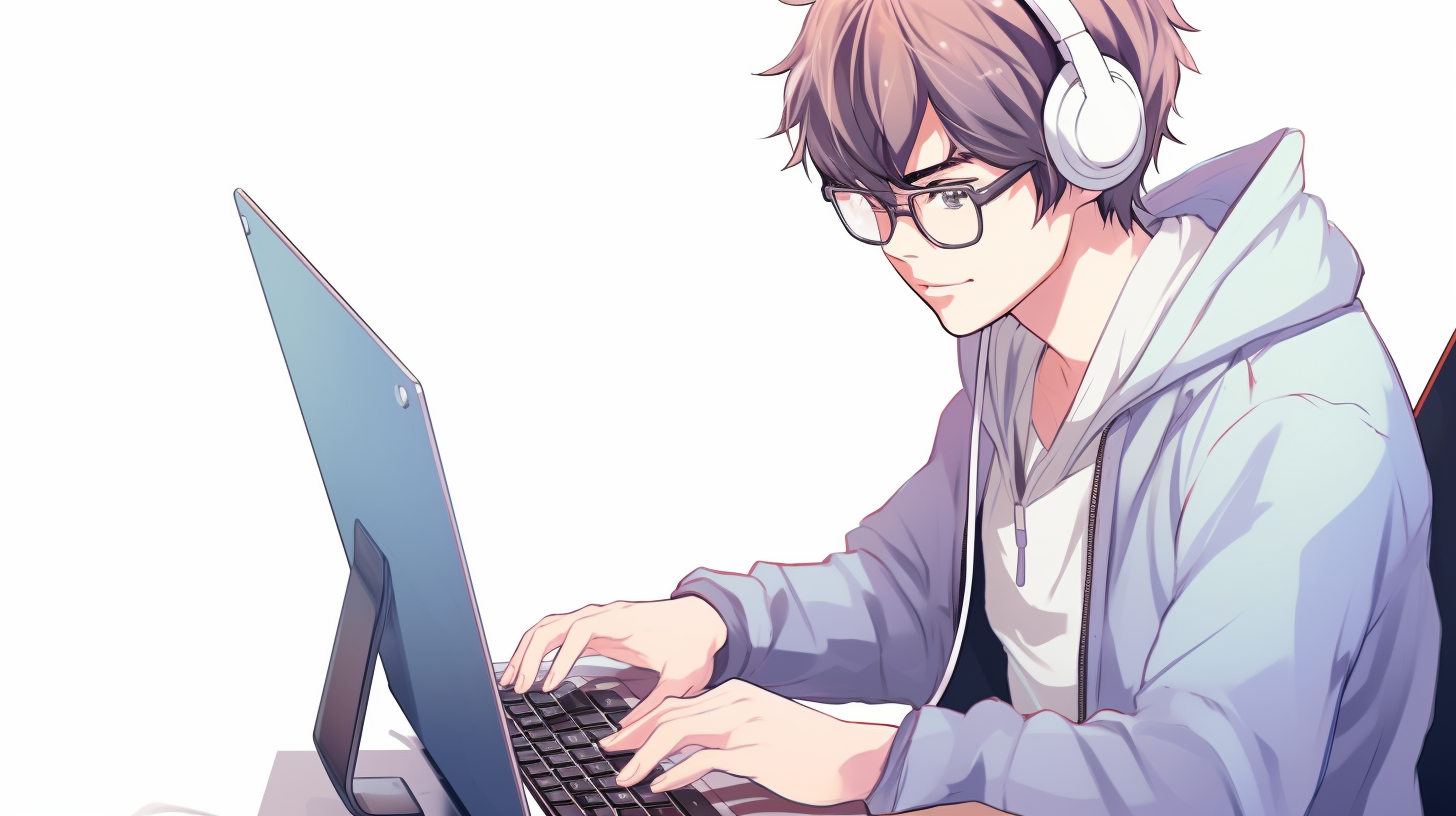
Swift and Multipeer Connectivity
Multipeer Connectivity is a powerful framework provided by Apple that allows iOS and macOS devices to communicate with one another in close proximity, enabling seamless peer-to-peer networking. This framework opens up a realm of possibilities for developers, making it easier to create collaborative applications that can exchange data efficiently, whether for games, file-sharing, or any interactive experience where multiple devices are involved.
The core of the Multipeer Connectivity framework revolves around the MCPeerID, MCSession, and MCNearbyServiceAdvertiser classes, which work together to discover and send data to nearby peers. Each device in a session is identified by a unique peer ID, allowing for easy identification and communication channels among connected devices.
To set the stage for communication, devices use MCNearbyServiceAdvertiser to announce their availability to nearby peers, while MCNearbyServiceBrowser can be used to look for available peers that are advertising their services. Once a connection is established, devices can create an MCSession to manage the communication and data transfer.
Multipeer Connectivity supports various types of data transfers, including streams, files, and direct messages, making it flexible for numerous applications. The framework abstracts many of the complexities associated with network programming, allowing developers to focus on creating responsive and engaging user experiences.
The following Swift code snippet demonstrates the creation of a basic peer ID and session:
let peerID = MCPeerID(displayName: UIDevice.current.name) let session = MCSession(peer: peerID, securityIdentity: nil, encryptionPreference: .required)
In this code, we create a MCPeerID using the device’s name as the display name, which will be visible to other peers. We then initialize an MCSession that will facilitate communication between this peer and others. By setting the encryption preference to .required, we ensure that all data exchanged is encrypted, enhancing security in peer-to-peer interactions.
Setting Up a Multipeer Session
To properly set up a Multipeer session, you’ll need to follow a series of steps that involve initializing your peer ID, session, and the services for advertising and browsing. These components need to be configured to establish a robust peer-to-peer connection. Let’s break down the process into manageable sections.
First, after creating your MCPeerID
and MCSession
, the next step involves initializing the MCNearbyServiceAdvertiser
and MCNearbyServiceBrowser
. The advertiser is responsible for broadcasting your device’s availability, while the browser looks for other devices that are advertising. Here’s how you can set them up:
let serviceType = "example-service" // Define a unique service type let advertiser = MCNearbyServiceAdvertiser(peer: peerID, discoveryInfo: nil, serviceType: serviceType) let browser = MCNearbyServiceBrowser(peer: peerID, serviceType: serviceType)
In this snippet, we define a serviceType
, which should be a unique string identifier for your service. This string is important because it allows devices to discover each other based on the same service type. Next, we create instances of MCNearbyServiceAdvertiser
and MCNearbyServiceBrowser
, passing the peer ID and service type.
The next step is to implement the necessary delegate methods to respond to events occurring in the advertising and browsing processes. For the MCNearbyServiceAdvertiser
, you need to handle invitations and manage the session:
extension YourViewController: MCNearbyServiceAdvertiserDelegate { func advertiser(_ advertiser: MCNearbyServiceAdvertiser, didReceiveInviteFromPeer peerID: MCPeerID, withContext context: Data?, invitationHandler: @escaping (Bool, MCSession?) -> Void) { // Accept the invitation invitationHandler(true, session) } }
In this code, the advertiser(_:didReceiveInviteFromPeer:withContext:invitationHandler:)
method is called when another device sends an invitation to connect. By calling the invitationHandler
with true
and the session, you accept the invitation and establish a connection.
For the MCNearbyServiceBrowser
, you need to handle found peers and lost peers:
extension YourViewController: MCNearbyServiceBrowserDelegate { func browser(_ browser: MCNearbyServiceBrowser, foundPeer peerID: MCPeerID, withDiscoveryInfo info: [String : String]?) { // Invite the found peer to join the session browser.invitePeer(peerID, to: session, withContext: nil, timeout: 30) } func browser(_ browser: MCNearbyServiceBrowser, lostPeer peerID: MCPeerID) { // Handle the loss of a peer } }
In the foundPeer
method, once a peer is discovered, you can invite them to join the established session. Similarly, the lostPeer
method allows you to manage the state when a peer disconnects or is no longer available.
Finally, don’t forget to start advertising and browsing by calling the respective start
methods:
advertiser.startAdvertisingPeer() browser.startBrowsingForPeers()
This will make your device visible to others and start listening for incoming connections. Properly managing these components very important for ensuring that your Multipeer session is both initiated and maintained effectively.
Sending and Receiving Data
When it comes to sending and receiving data using the Multipeer Connectivity framework, the process is both intuitive and efficient. Once you have established your MCSession and connected to peers, the next step is to implement the methods for data transmission. The framework supports various data types, allowing you to send simple text messages, images, files, or even complex objects as long as they conform to the NSCoding protocol.
To send data, you’ll make use of the send(_:toPeers:with:)` method from the `MCSession` class. This method requires the data to be sent, a list of peers to send it to, and the type of data transmission. Here's a simple example of how to send a string message:
func sendMessage(_ message: String) { if let data = message.data(using: .utf8) { do { try session.send(data, toPeers: session.connectedPeers, with: .reliable) } catch { print("Error sending message: (error.localizedDescription)") } } }
In this example, we convert the string message into `Data` using UTF-8 encoding, then attempt to send it to all connected peers using the reliable mode, which ensures that the message delivery is guaranteed. If sending the data fails, an error message will be printed to the console, which is helpful for debugging.
On the receiving end, you must implement the `session(_:didReceive:fromPeer:)` delegate method to handle incoming data. Here’s how you can receive and process the incoming message:
extension YourViewController: MCSessionDelegate { func session(_ session: MCSession, didReceive data: Data, fromPeer peerID: MCPeerID) { if let message = String(data: data, encoding: .utf8) { // Handle the received message print("Received message: (message) from (peerID.displayName)") } } }
In this delegate method, we check if the received data can be converted back into a string. If successful, we can process the message as needed, such as displaying it in the user interface or storing it for later use. This seamless exchange of data between peers is one of the key advantages of using the Multipeer Connectivity framework, making it ideal for collaborative applications.
An additional aspect of the Multipeer Connectivity framework is its ability to handle more complex data types. If you wish to send custom objects, make sure those objects conform to `NSCoding`. This will allow you to transform your objects into data and vice versa. Here's an example of how to encode and send a custom object:
class CustomObject: NSObject, NSCoding { var name: String var value: Int init(name: String, value: Int) { self.name = name self.value = value } required init(coder aDecoder: NSCoder) { name = aDecoder.decodeObject(forKey: "name") as! String value = aDecoder.decodeInteger(forKey: "value") } func encode(with aCoder: NSCoder) { aCoder.encode(name, forKey: "name") aCoder.encode(value, forKey: "value") } } func sendCustomObject(_ object: CustomObject) { do { let data = try NSKeyedArchiver.archivedData(withRootObject: object, requiringSecureCoding: false) try session.send(data, toPeers: session.connectedPeers, with: .reliable) } catch { print("Error sending custom object: (error.localizedDescription)") } }
In this example, we define a `CustomObject` class that conforms to `NSCoding`. We then serialize the object to `Data` using `NSKeyedArchiver` before sending it through the session. On the receiving side, you would deserialize the data back into a `CustomObject` using `NSKeyedUnarchiver` in the `session(_:didReceive:fromPeer:)` method.
Best Practices for Multipeer Connectivity Implementation
When implementing Multipeer Connectivity in your applications, adhering to best practices ensures a smooth user experience, efficient resource management, and robust error handling. By considering these practices, you can avoid common pitfalls that may arise in peer-to-peer networking.
1. Manage Session State
It is important to keep track of the session state and its connected peers. Using the MCSessionDelegate
methods effectively allows you to react to changes in the connection status, such as peers connecting or disconnecting. Implementing the session(_:peer:didChange:)
method is essential in this regard:
extension YourViewController: MCSessionDelegate { func session(_ session: MCSession, peer peerID: MCPeerID, didChange state: MCSessionPeerState) { switch state { case .connected: print("(peerID.displayName) connected") case .connecting: print("(peerID.displayName) is connecting") case .notConnected: print("(peerID.displayName) disconnected") @unknown default: fatalError("Unknown state for peer (peerID)") } } }
Properly updating your user interface and managing resources based on peer states enhances the interactivity of your application.
2. Optimize Data Transfer
When sending data, choose the appropriate delivery mode between .reliable
and .unreliable
. Reliable delivery guarantees that data is delivered but can introduce latency, while unreliable delivery allows for faster transmission at the cost of data integrity. For non-critical updates, such as game state changes, using .unreliable
can improve responsiveness. Here’s an example:
func sendGameUpdate(_ update: GameUpdate) { do { let data = try NSKeyedArchiver.archivedData(withRootObject: update, requiringSecureCoding: false) try session.send(data, toPeers: session.connectedPeers, with: .unreliable) } catch { print("Error sending game update: (error.localizedDescription)") } }
3. Handle Data Serialization Efficiently
When sending complex data, ensure that your data serialization and deserialization processes are efficient. Think using lightweight formats like JSON or Protocol Buffers for simpler structures. This can reduce the size of the data being transferred and lead to faster transmissions. For instance, using JSON for a simple object:
struct SimpleObject: Codable { let id: String let name: String } func sendSimpleObject(_ object: SimpleObject) { do { let data = try JSONEncoder().encode(object) try session.send(data, toPeers: session.connectedPeers, with: .reliable) } catch { print("Error sending simple object: (error.localizedDescription)") } }
4. Implement Robust Error Handling
Networking can be unpredictable. Implementing robust error handling can help maintain a good user experience. For example, manage errors when sending data to peers:
func sendData(_ data: Data) { do { try session.send(data, toPeers: session.connectedPeers, with: .reliable) } catch let error as MCSessionSendDataError { switch error { case .notConnected: print("Not connected to any peers.") case .dataTooBig: print("Data is too big to send.") case .other: print("Other sending error: (error.localizedDescription)") } } catch { print("Error sending data: (error.localizedDescription)") } }
5. Regularly Monitor Network Performance
Performance is important in peer-to-peer applications, especially when multiple devices are involved. Utilize logging and analytics to monitor connection quality and data transfer rates. This allows you to identify performance bottlenecks and optimize your application accordingly.