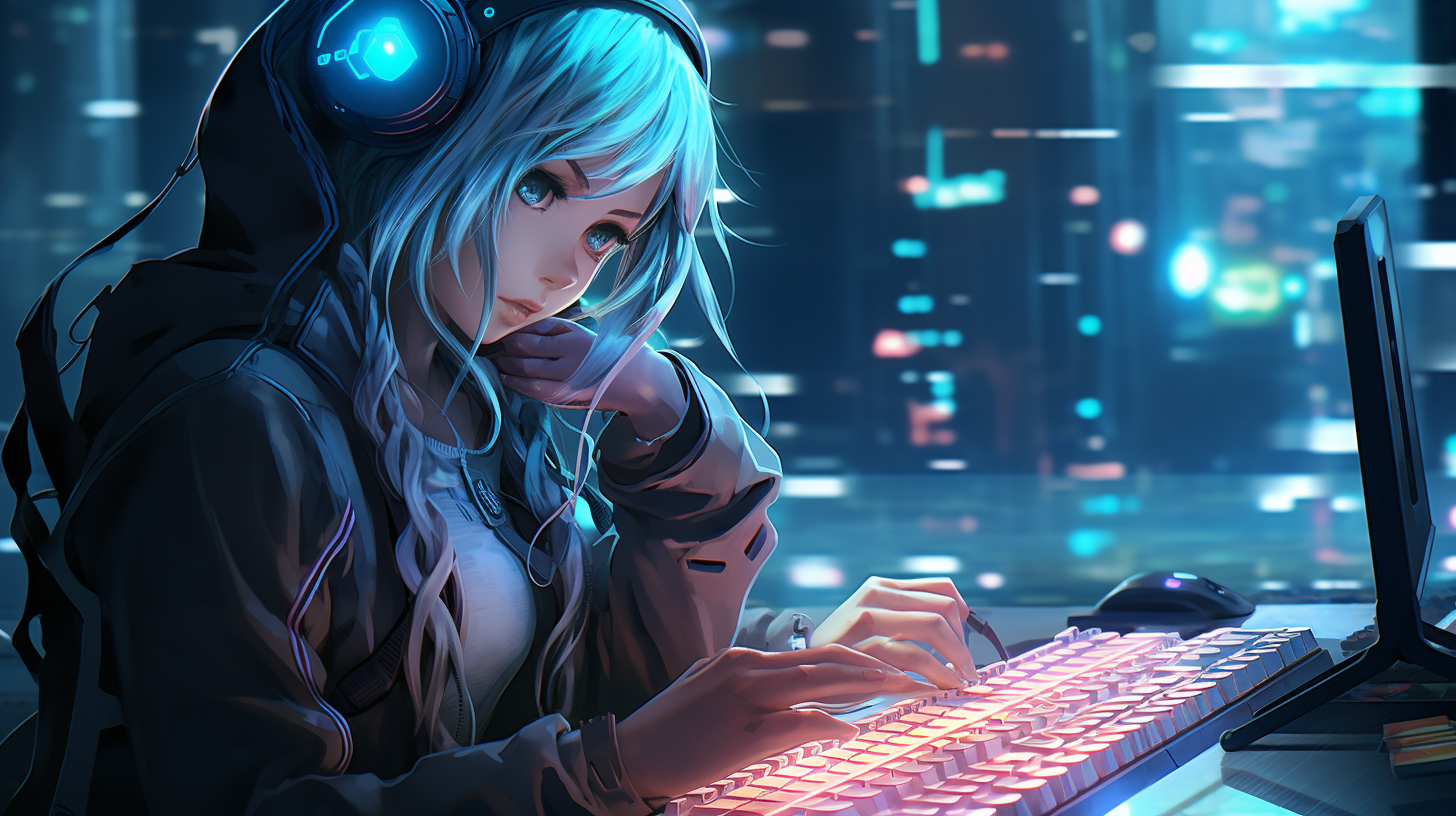
SQL for Time-Efficient Data Queries
When delving into the world of SQL and database management, it is crucial to grasp the performance metrics that define the efficiency of your queries. Query performance metrics provide insights into how well your SQL statements are executed, enabling you to identify bottlenecks and optimize your database interactions.
Key performance metrics to consider include:
- The total time taken to execute a query, from the moment it’s sent to the database until the results are returned. This metric is fundamental in assessing the responsiveness of your application.
- The amount of CPU resources consumed during the query execution. High CPU usage can indicate inefficient queries that require optimization.
- The number of read and write operations performed on the disk. Excessive I/O can slow down query performance, as accessing data from disk is significantly slower than from memory.
- The amount of memory used during query execution. Efficiently designed queries should minimize memory usage to avoid thrashing and enable faster execution.
- The number of rows processed by the query. High row counts can lead to slower performance, especially if filtering and sorting are not managed properly.
To obtain these metrics, many database management systems (DBMS) offer built-in tools. For instance, in SQL Server, you can use the following command to retrieve execution statistics:
SET STATISTICS TIME ON; SET STATISTICS IO ON; SELECT * FROM your_table WHERE some_column = 'some_value'; SET STATISTICS TIME OFF; SET STATISTICS IO OFF;
This command will display execution times and I/O statistics for the query you execute, helping you analyze its performance.
Additionally, you can leverage the database’s query profiling tools. For example, MySQL provides the EXPLAIN
statement, which gives insights into how the database engine plans to execute a query:
EXPLAIN SELECT * FROM your_table WHERE some_column = 'some_value';
This statement will return a detailed breakdown of the query execution plan, including information about indexes used, potential full table scans, and join operations, so that you can spot inefficiencies.
Understanding these performance metrics is the first step toward optimizing your SQL queries. By continuously monitoring and analyzing these metrics, you can refine your SQL statements, ensuring that your database interactions remain efficient and responsive.
Indexing Strategies for Faster Access
Indexing is a fundamental technique for enhancing the speed of SQL queries, akin to how an index in a book helps readers locate information quickly without sifting through every page. By strategically creating indexes on your tables, you can drastically reduce the amount of data the database engine needs to scan, leading to faster query responses.
When you create an index on a column or a set of columns, the database constructs a separate data structure that maintains the values in those columns in a sorted order, facilitating rapid lookups. However, it’s essential to approach indexing thoughtfully, as excessive or poorly planned indexes can lead to performance degradation during data modification operations like INSERT, UPDATE, or DELETE.
There are several types of indexes you can implement:
- The default type of index in many database systems, optimized for quick searching, especially for equality and range queries.
- Best suited for equality comparisons, hash indexes provide extremely fast lookups but do not support range queries.
- These are indexes that involve multiple columns. They are particularly useful for queries that filter based on several criteria.
- Perfect for searching large text fields, allowing for searches based on words and phrases rather than exact matches.
When considering which columns to index, focus on:
- Columns frequently used in WHERE clauses.
- Columns involved in JOIN conditions.
- Columns used in ORDER BY and GROUP BY clauses.
Here’s a simple illustration of how to create an index in SQL:
CREATE INDEX idx_some_column ON your_table (some_column);
If you have a query that frequently filters based on multiple columns, a composite index can significantly improve performance. For example:
CREATE INDEX idx_multiple_columns ON your_table (column1, column2);
However, it’s crucial to monitor the impact of your indexes. Use the query execution plan to analyze whether your indexes are being utilized effectively. In SQL Server, you can utilize the following command to view the execution plan:
SET SHOWPLAN_XML ON; GO SELECT * FROM your_table WHERE some_column = 'some_value'; GO SET SHOWPLAN_XML OFF;
In MySQL, you would use:
EXPLAIN SELECT * FROM your_table WHERE some_column = 'some_value';
If you find that some indexes are not being used or not providing the expected performance improvements, consider dropping them to save on maintenance costs. You can drop an index using a simple command:
DROP INDEX idx_some_column ON your_table;
Ultimately, the objective is to strike a balance between read and write performance when implementing indexes. By thoughtfully applying indexing strategies, you can transform your data access patterns, making your SQL queries not only faster but also more efficient.
Optimizing SQL Queries with Efficient Joins
Efficient joins are essential in optimizing SQL queries for performance, particularly when dealing with large datasets or complex relationships among tables. A join operation combines rows from two or more tables based on a related column, facilitating data retrieval. However, inefficient joins can lead to significant performance bottlenecks. Thus, understanding how to optimize these operations is paramount.
When crafting joins, it’s critical to choose the right type of join for your data retrieval needs. SQL supports several types of joins, including INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. The INNER JOIN is often the most efficient, as it returns rows when there is a match in both tables, minimizing the volume of data processed. In contrast, OUTER JOINs may produce larger result sets, which can increase execution time. Here’s a basic example of an INNER JOIN:
SELECT a.column1, b.column2 FROM table_a a INNER JOIN table_b b ON a.common_id = b.common_id;
Another critical aspect of optimizing joins is ensuring that the columns used for join conditions are indexed. Indexing the join columns can dramatically reduce the amount of data the database engine needs to process, resulting in faster query execution. For example, if you frequently join on the `common_id` column, consider adding an index:
CREATE INDEX idx_common_id ON table_a (common_id); CREATE INDEX idx_common_id ON table_b (common_id);
Additionally, the order of joins can impact performance. When executing multiple joins, the order in which they’re processed can influence the efficiency of the query. The database’s query optimizer typically handles this, but understanding the data and potential execution paths can help refine your approach. In general, it’s beneficial to join smaller tables first or to filter results early in the query process.
Another optimization technique involves using subqueries or Common Table Expressions (CTEs) to reduce the complexity of joins. By breaking down a complex query into smaller, manageable parts, you can improve readability and potentially enhance performance. Here’s an example of a CTE:
WITH FilteredData AS ( SELECT * FROM table_a WHERE some_condition ) SELECT fd.column1, b.column2 FROM FilteredData fd INNER JOIN table_b b ON fd.common_id = b.common_id;
It’s also essential to be aware of the data types and sizes of the columns involved in join conditions. Mismatched data types can lead to implicit conversions, which degrade performance. Ensure that the columns being joined are of the same data type to avoid unnecessary overhead.
Lastly, always analyze your query’s execution plan. Tools like EXPLAIN in MySQL or SET SHOWPLAN_XML in SQL Server will provide insights into how your joins are being executed. You can identify whether indexes are being utilized effectively and spot any full table scans that may be occurring:
EXPLAIN SELECT a.column1, b.column2 FROM table_a a INNER JOIN table_b b ON a.common_id = b.common_id;
By taking these steps—selecting the appropriate join type, indexing your join columns, optimizing join order, using CTEs or subqueries, ensuring data type compatibility, and analyzing execution plans—you can significantly enhance the performance of your SQL queries. Efficient joins are not just about retrieving data; they’re a critical component of a well-optimized database system.
Using Caching Mechanisms
Caching mechanisms play a vital role in enhancing the performance of SQL queries by reducing the need to repeatedly access disk storage for frequently requested data. In essence, caching stores copies of data that can be retrieved much faster than if they were fetched from the underlying database every time a query is executed. This can lead to significant improvements in the speed and responsiveness of applications that rely on database interactions.
There are several types of caching strategies that can be implemented to optimize SQL query performance, including:
- Many database management systems (DBMS) have built-in caching mechanisms that store the results of specific queries. If the same query is executed again, the DBMS can return the cached result without re-executing the query, greatly reducing response times. For example, in MySQL, you can enable query caching by setting the
query_cache_size
parameter. - This method involves storing query results at the application level, often using in-memory data stores like Redis or Memcached. This allows applications to retrieve frequently accessed data without hitting the database, providing extremely low-latency responses. In a typical use case, an application would first check the cache for the required data; if it’s not found, the application would query the database and subsequently store the result in the cache for future use.
- Similar to application-level caching, object caching involves storing the results of database queries in objects, which can be serialized and stored in memory. Frameworks like Hibernate and Entity Framework provide built-in support for object caching, allowing developers to configure caching strategies based on their needs. This approach is beneficial in scenarios where the same data objects are accessed repeatedly within a single application session.
To implement query caching effectively, it is essential to ponder the nature of your data and how frequently it changes. Caching data that is relatively static can yield the best performance gains, while frequently changing data may lead to stale cache issues. Therefore, implementing a cache invalidation strategy is important. You can set expiration times for cached data or clear the cache when changes occur in the underlying database.
Here’s an example of how you might implement caching in a typical application using Redis:
// Pseudo-code for caching with Redis const cacheKey = 'query_result_key'; const cachedResult = redis.get(cacheKey); if (cachedResult) { return cachedResult; // Return cached result } else { const queryResult = database.query('SELECT * FROM your_table WHERE some_condition'); redis.set(cacheKey, queryResult, 'EX', 3600); // Cache for 1 hour return queryResult; }
Implementing caching not only improves performance but also reduces the load on your database, allowing it to handle more concurrent connections and queries. However, it’s crucial to monitor cache hit rates and performance metrics to assess the effectiveness of your caching strategies. Tools such as Redis’ monitoring commands can provide insights into cache performance:
// Monitor Redis cache performance INFO stats
Using caching mechanisms can significantly enhance the speed and efficiency of SQL queries by minimizing the need for repetitive data retrieval from the disk storage. By thoughtfully implementing query caching, application-level caching, or object caching, and carefully managing cache invalidation, you can dramatically improve your application’s performance while ensuring that users receive timely and accurate data.
Using Partitioning for Large Datasets
Partitioning is a powerful strategy for managing large datasets in SQL environments, enabling efficient data retrieval and improved performance by dividing a table into smaller, more manageable pieces known as partitions. Each partition can be accessed and queried independently, which can significantly speed up query performance, especially when dealing with vast amounts of data. Understanding how to utilize partitioning effectively very important for developers and database administrators alike.
There are several types of partitioning strategies, including:
- This type divides data into partitions based on a specified range of values, such as dates or numerical ranges. For example, data could be partitioned by year, allowing for faster queries targeting specific time frames.
- List partitioning involves dividing data into partitions based on a predefined list of values. This method is suitable when certain discrete categories or statuses are frequently queried.
- Hash partitioning distributes data across partitions based on a hashing algorithm, which can help balance data across partitions when the data distribution is unpredictable.
- This approach combines multiple partitioning strategies, such as range-list or range-hash, providing more granular control over data distribution.
Implementing partitioning requires careful consideration of how data is accessed. For instance, if you frequently query data by date, a range partitioning strategy based on date columns can lead to significant performance improvements. Here’s an example of how you might create a range partitioned table in SQL:
CREATE TABLE sales ( id INT, sale_date DATE, amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(sale_date)) ( PARTITION p2019 VALUES LESS THAN (2020), PARTITION p2020 VALUES LESS THAN (2021), PARTITION p2021 VALUES LESS THAN (2022) );
In this example, the sales table is partitioned by year, which allows the database engine to quickly locate relevant partitions when executing queries that filter by sale date.
Another advantage of partitioning is that it can simplify maintenance tasks. For instance, when data becomes obsolete, you can drop an entire partition, effectively deleting a large volume of data with minimal impact on performance. Here’s how you would drop a partition in SQL:
ALTER TABLE sales DROP PARTITION p2019;
However, while partitioning can offer substantial performance benefits, it isn’t without its challenges. It requires careful planning to determine the best partitioning key and strategy based on your specific query patterns and data access needs. Improperly chosen partitioning schemes can lead to performance degradation instead of improvement.
It’s also essential to ensure that your queries are designed to take advantage of partitioning. Queries that filter on the partition key will benefit most from this strategy. For example:
SELECT SUM(amount) FROM sales WHERE sale_date >= '2020-01-01' AND sale_date < '2021-01-01';
This query effectively targets the appropriate partition, resulting in faster execution times. Conversely, queries that do not filter by the partitioning key may still lead to full table scans, negating the performance benefits of partitioning.
Monitoring and analyzing the performance of your partitioned tables is critical. Use tools provided by your database management system to evaluate query execution plans and ensure that partitions are being utilized effectively. By continuously assessing and adjusting your partitioning strategies, you can maintain optimal performance in your SQL queries as your data evolves.
Best Practices for Query Execution Plans
Effective use of query execution plans is paramount in achieving optimal SQL performance. Query execution plans provide a roadmap for how the database engine intends to execute a given SQL statement, detailing the steps taken, the indexes used, and the methods for accessing data. By analyzing these plans, developers and database administrators can identify inefficiencies and make informed decisions on query optimization.
The process of generating an execution plan typically varies between database systems, but the fundamental concept remains the same: understanding the chosen execution path for a query. In SQL Server, the execution plan can be retrieved using the following command:
SET SHOWPLAN_XML ON; GO SELECT * FROM your_table WHERE some_column = 'some_value'; GO SET SHOWPLAN_XML OFF;
This command will return the execution plan in XML format, providing detailed insights into the plan’s operations. Similarly, in MySQL, the EXPLAIN statement can be employed:
EXPLAIN SELECT * FROM your_table WHERE some_column = 'some_value';
When reviewing the execution plan, you should pay attention to several critical aspects:
- Different join algorithms (nested loop, hash join, merge join) can have varying performance. Understanding which join method is used can guide optimizations.
- Check whether indexes are utilized effectively. If full table scans are occurring where indexed accesses are expected, it may indicate a need for index creation or query rewriting.
- Sorting can be resource-intensive, especially on large datasets. Identify if sorting can be minimized or avoided through proper indexing.
- Look for patterns in how data is accessed. For instance, if certain queries repeatedly access specific ranges of data, think if additional indexes or partitioning may enhance performance.
Once you analyze the execution plan, implementing improvements based on your findings is essential. Here are some best practices to consider:
- Simplify complex queries, break them down into smaller components, or eliminate unnecessary subqueries to enhance clarity and performance.
- Ensure that indexes exist on columns used in WHERE clauses, JOIN conditions, and ORDER BY statements. Additionally, review the effectiveness of existing indexes and remove any that are not utilized.
- Mismatched data types can lead to implicit conversions, slowing down performance. Ensure that the data types match in join and filter conditions.
- Use techniques like pagination or the LIMIT clause to reduce the number of rows processed and returned, especially in scenarios where only a subset of data is needed.
For instance, if you notice that a query is using a nested loop join inefficiently, think if rewriting the query to leverage a hash join could yield better performance. Similarly, if an index is not being utilized as expected, it may require adjustments in the query structure or indexing strategy.
Monitoring execution plans over time is also crucial. As data grows and schema evolves, the effectiveness of your query execution strategies may change. Regularly reviewing execution plans helps ensure that your queries remain efficient and responsive to user demands.
Mastering query execution plans is an essential skill for anyone involved in database management. By analyzing execution paths, applying best practices, and continuously monitoring performance, you can significantly enhance the efficiency of your SQL queries, delivering optimal performance for your applications.