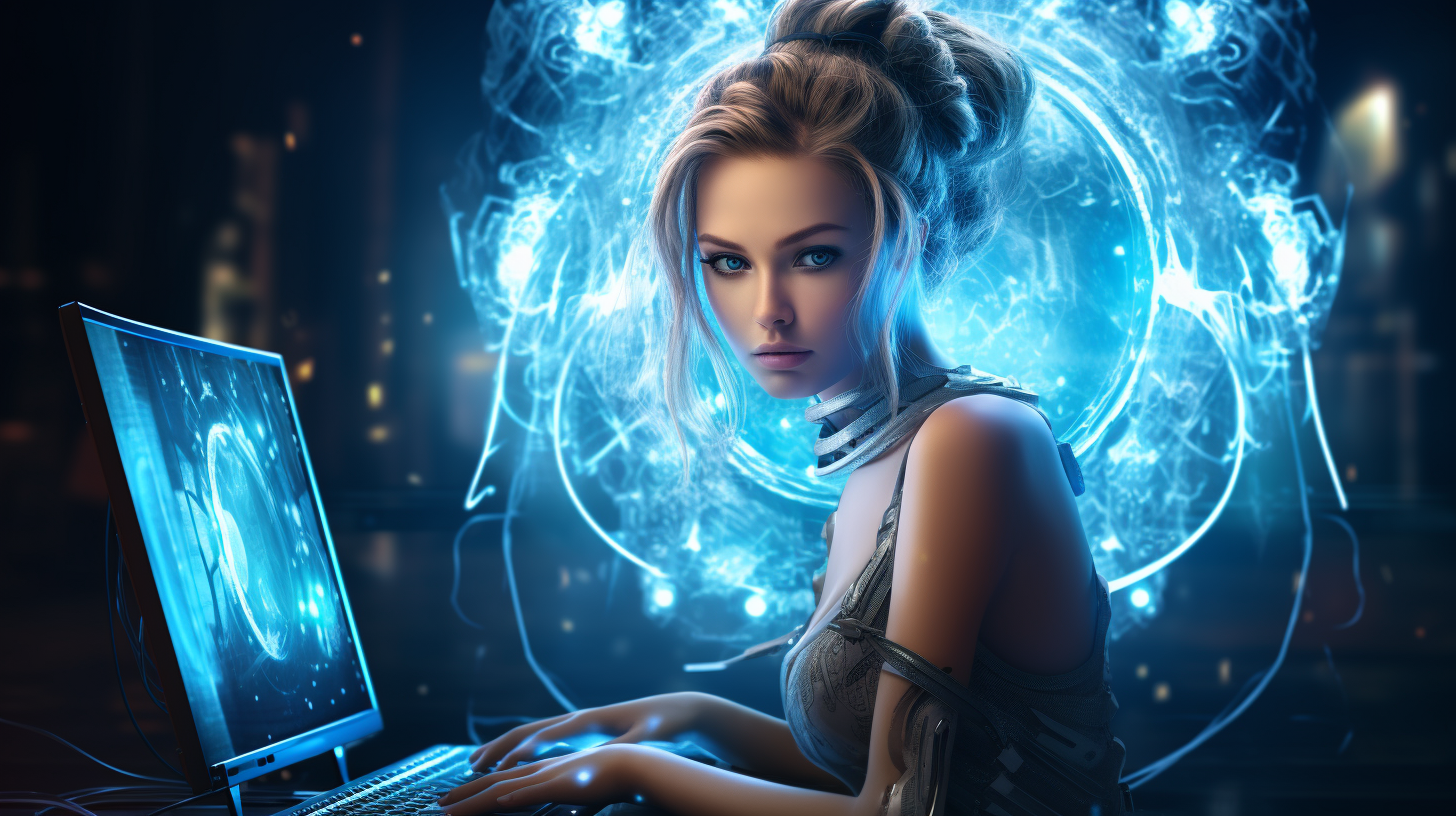
Bash Functions and Modules
Bash functions are a powerful feature that allows you to encapsulate a series of commands into a single callable unit. Understanding their syntax and structure especially important for efficient scripting and automation in the Bash environment.
The basic syntax for defining a Bash function is as follows:
function function_name { # commands }
Alternatively, you can omit the function
keyword and define a function by simply using parentheses:
function_name() { # commands }
Both forms are valid and can be used interchangeably, although the parenthesis version is more common in scripts. The function body is enclosed in curly braces, and the commands are executed in the order they are written.
To call a function, simply use its name followed by any required arguments:
function_name arg1 arg2
Within the function, you can access these arguments using the special variables $1
, $2
, and so on, where $1
corresponds to the first argument, $2
to the second, and so forth. The variable $#
holds the total number of arguments passed to the function, and $@
or $*
can be used to access all the arguments as a list.
Here’s an example function that demonstrates these concepts:
greet() { echo "Hello, $1! You are visitor number $2." } greet "Alice" 5
In this example, calling greet "Alice" 5
results in the output: Hello, Alice! You are visitor number 5.
Another important aspect of function structure is the ability to return values. In Bash, functions do not return values in the way that traditional programming languages do; instead, they can set an exit status with the return
command. By convention, an exit status of 0
indicates success, while any non-zero value indicates an error:
divide() { if [ $2 -eq 0 ]; then echo "Error: Division by zero." return 1 fi echo "Result: $(( $1 / $2 ))" return 0 } divide 10 2 divide 10 0
In the example above, the divide
function checks for division by zero and returns an appropriate error message and exit status. It showcases both the control flow and the importance of proper error handling in function design.
Bash functions also support local variables, which can be defined using the local
keyword. That’s critical for avoiding variable name collisions:
count() { local value=0 ((value++)) echo "Count: $value" } count count
In this case, each call to count
initializes value
to 0
, demonstrating that the variable retains no value between invocations.
Understanding these fundamental aspects of function syntax and structure in Bash lays the groundwork for more advanced scripting techniques, allowing you to write clean, maintainable, and efficient shell scripts.
Creating and Using Functions in Bash
Creating functions in Bash is not just about packaging commands; it’s about designing logical units of work that can be reused with ease. The capability to create and utilize functions efficiently is key to writing scripts that are both concise and maintainable.
To create a function, you start with the syntax discussed previously. However, let’s explore some practical examples to illustrate their creation and application in real-world scenarios. Consider a scenario where you need to perform repetitive tasks, such as calculating the factorial of a number. A function allows you to encapsulate this logic neatly:
factorial() { local num=$1 local result=1 for (( i=1; i<=num; i++ )); do result=$((result * i)) done echo "Factorial of $num is $result" } factorial 5
When you call factorial 5
, the output will be: Factorial of 5 is 120. This function demonstrates how to take an input, perform a calculation, and then output the result. Notice the use of a local variable result
to avoid naming conflicts with other variables outside the function.
Functions can also accept multiple parameters. For instance, if you need a function that formats a string with a prefix and suffix, it can be defined as follows:
format_string() { local prefix=$1 local text=$2 local suffix=$3 echo "${prefix}${text}${suffix}" } format_string ">>> " "Hello, World!" " <<<"
This would produce: >> Hello, World! <<<. The function takes three arguments and concatenates them to create a formatted string. This highlights how functions can be employed to create modular and reusable code segments.
It’s also essential to understand how functions interact with the rest of your script. Functions can modify global variables, but it’s generally advisable to use local variables inside functions to prevent unexpected behavior. Here’s an example illustrating this point:
global_var="I am global" modify_var() { local global_var="I am local" echo "Inside function: $global_var" } modify_var echo "Outside function: $global_var"
When executing this script, you’ll see:
Inside function: I am local
Outside function: I am global
This illustrates how the local variable global_var
within the function does not affect the global variable of the same name outside the function. Such clarity in variable scope is critical for writing robust scripts.
Lastly, it’s worth mentioning that functions can be defined in a script and then called multiple times, allowing for a DRY (Don’t Repeat Yourself) approach. If you find yourself repeating the same lines of code, ponder encapsulating that logic in a function. The following example demonstrates a function that logs messages with timestamps:
log_message() { local message=$1 echo "$(date '+%Y-%m-%d %H:%M:%S') - $message" } log_message "Starting the script..." # Some operations log_message "Operations completed."
This approach not only saves time but also enhances the readability of your script. By creating functions for specific tasks, you can build complex scripts that are easier to understand and maintain.
Module Creation and Management in Bash
When it comes to modular programming in Bash, the concept of modules is essential for organizing and managing code efficiently. Modules allow you to encapsulate related functions into a single file, which can then be sourced into your scripts. This modularity promotes code reuse, improves readability, and facilitates easier maintenance.
To create a Bash module, you typically start by defining a file that contains your functions. For example, you might create a file named math_functions.sh
for mathematical operations:
# math_functions.sh add() { echo "Result: $(( $1 + $2 ))" } subtract() { echo "Result: $(( $1 - $2 ))" } multiply() { echo "Result: $(( $1 * $2 ))" } divide() { if [ $2 -eq 0 ]; then echo "Error: Division by zero." return 1 fi echo "Result: $(( $1 / $2 ))" }
Once you have defined your functions within a module file, you can utilize them in other scripts by sourcing the module. That is accomplished using the source
command or the shorthand dot operator .
followed by the path to the module file:
# main_script.sh source math_functions.sh add 10 5 subtract 10 5 multiply 10 5 divide 10 5 divide 10 0
When you run main_script.sh
, it will output:
Result: 15 Result: 5 Result: 50 Result: 2 Error: Division by zero.
This demonstrates how functions defined in math_functions.sh
can be called seamlessly in main_script.sh
. The use of modules not only simplifies your main script but also keeps your code organized.
Moreover, proper management of modules involves adhering to a few best practices. Naming your module files descriptively based on their functionality aids in the quick identification of their purpose. Additionally, maintaining a consistent function naming convention across your modules can prevent confusion and collisions.
Another critical aspect of module management is ensuring that your functions are idempotent. This means that repeated calls to the same function with the same arguments should produce the same output without causing unintended side effects. That’s particularly crucial in scripts that may run multiple times or in parallel environments.
Version control for your modules is also recommended. By maintaining a version history, you can track changes and revert to earlier versions if necessary, allowing for robust development and testing processes.
Lastly, documentation within your module files will greatly enhance usability for yourself and others. Including comments and usage examples at the beginning of each module provides clarity on how to utilize the functions effectively.
Managing modules in Bash involves creating function files, sourcing them into scripts, following naming conventions, ensuring idempotency, using version control, and maintaining thorough documentation. These practices will not only enhance your scripting capabilities but also contribute to a well-structured codebase that can be easily maintained and shared.
Best Practices for Writing Bash Functions
When writing Bash functions, adhering to best practices can significantly enhance your code’s readability, maintainability, and overall performance. Below are several guidelines that serve as cornerstones for effective function design in Bash.
1. Use Descriptive Function Names:
Function names should clearly indicate their purpose. This not only helps you remember what each function does but also assists others who may work with your code. For example, instead of naming a function do_it
, a more descriptive name like calculate_statistics
would be preferable.
2. Keep Functions Focused:
Each function should ideally perform a single task or a closely related set of tasks. This principle, often referred to as the Single Responsibility Principle, helps in understanding and testing your functions independently. If a function becomes too complex, consider breaking it into smaller sub-functions.
calculate_statistics() { local sum=0 local count=0 for number in "$@"; do sum=$((sum + number)) count=$((count + 1)) done echo "Average: $((sum / count))" }
3. Use Local Variables:
To prevent variable name collisions, always declare variables as local within your functions using the local
keyword. This keeps your functions self-contained and reduces the risk of unintended side effects.
compute_area() { local radius=$1 local area=$(echo "3.14 * $radius^2" | bc) echo "Area: $area" }
4. Handle Errors Gracefully:
Robust error handling is essential for any script. Use conditional statements to validate inputs and handle errors gracefully, providing meaningful error messages when something goes wrong.
safe_divide() { if [ "$2" -eq 0 ]; then echo "Error: Division by zero." return 1 fi echo "Result: $(( $1 / $2 ))" }
5. Document Your Functions:
In-line comments and documentation within your functions can dramatically improve the understandability of your code. Provide a brief description of what the function does, its parameters, and return values.
# Calculates the factorial of a number. # Arguments: # $1 - The number to calculate the factorial for. factorial() { local num=$1 local result=1 for (( i=1; i <= num; i++ )); do result=$((result * i)) done echo $result }
6. Avoid Global State:
Whenever possible, avoid relying on global variables within your functions. This makes your functions more predictable and easier to test. If you need to use a variable, pass it as an argument.
update_value() { local new_value=$1 # Avoid using a global variable echo "New value is: $new_value" }
7. Test Functions Independently:
Create a dedicated test suite for your functions. This will help you identify issues early and ensure that changes do not break existing functionality. Write test cases for both typical and edge cases.
test_factorial() { local result=$(factorial 5) if [ "$result" -eq 120 ]; then echo "Factorial test passed." else echo "Factorial test failed." fi }
By incorporating these best practices into your Bash functions, you can create scripts that are not only effective but also easier to maintain and extend over time. As you become more comfortable with these principles, you will find that your Bash scripting capabilities will evolve significantly, allowing you to tackle more complex automation tasks with confidence.
Debugging and Testing Bash Functions and Modules
Debugging and testing Bash functions and modules is an essential aspect of writing reliable and maintainable shell scripts. The complexity of scripts can often lead to subtle bugs that are not immediately apparent, making it crucial to adopt effective debugging strategies. Bash provides several built-in features and techniques to help identify issues and ensure that functions behave as expected.
One of the simplest yet most effective debugging techniques is to use the set command to enable options that facilitate tracking the execution of your script. For example, adding set -x
at the beginning of your script or function will print each command before it’s executed, providing insight into the flow of your script:
set -x my_function() { echo "This is a test function." return 0 } my_function
Running the above will display the command along with its expanded values before execution, making it easier to trace variable states and control flow. When debugging is complete, you can turn off this option with set +x
.
Another useful option is set -e
, which causes the script to exit immediately if any command exits with a non-zero status. That is particularly beneficial during testing, as it prevents the script from proceeding with potentially erroneous states:
set -e error_prone_function() { echo "This will fail." return 1 } error_prone_function echo "This will not be printed."
In this example, the second echo command will not execute because error_prone_function
exits with a non-zero status. This feature helps quickly identify points of failure in your functions.
Moreover, Bash provides a robust way to handle errors through conditional checks and trap commands. You can use trap
to catch errors and execute cleanup or logging functions. Here’s how you can implement this:
trap 'echo "An error occurred. Exiting..."; exit 1;' ERR sample_function() { echo "Doing something risky..." false # Simulates an error } sample_function
In this example, if sample_function
encounters an error (indicated by false
), the trap will activate and print a message before exiting the script gracefully.
When it comes to testing functions, creating a separate test script can be a good practice. This script can invoke the functions with various inputs to validate their outputs:
test_function() { result=$(my_function) expected="This is a test function." if [[ "$result" == "$expected" ]]; then echo "Test passed!" else echo "Test failed: expected '$expected', got '$result'" fi } test_function
By encapsulating tests in functions, you can easily run and rerun tests as your code evolves, ensuring that changes do not introduce new bugs. This disciplined approach to debugging and testing leads to more robust Bash scripts that can stand the test of time.
Effective debugging and rigorous testing are fundamental practices that enhance the reliability of Bash functions and modules. Using built-in debugging tools, error handling techniques, and structured testing methodologies can significantly elevate the quality and maintainability of your shell scripts.