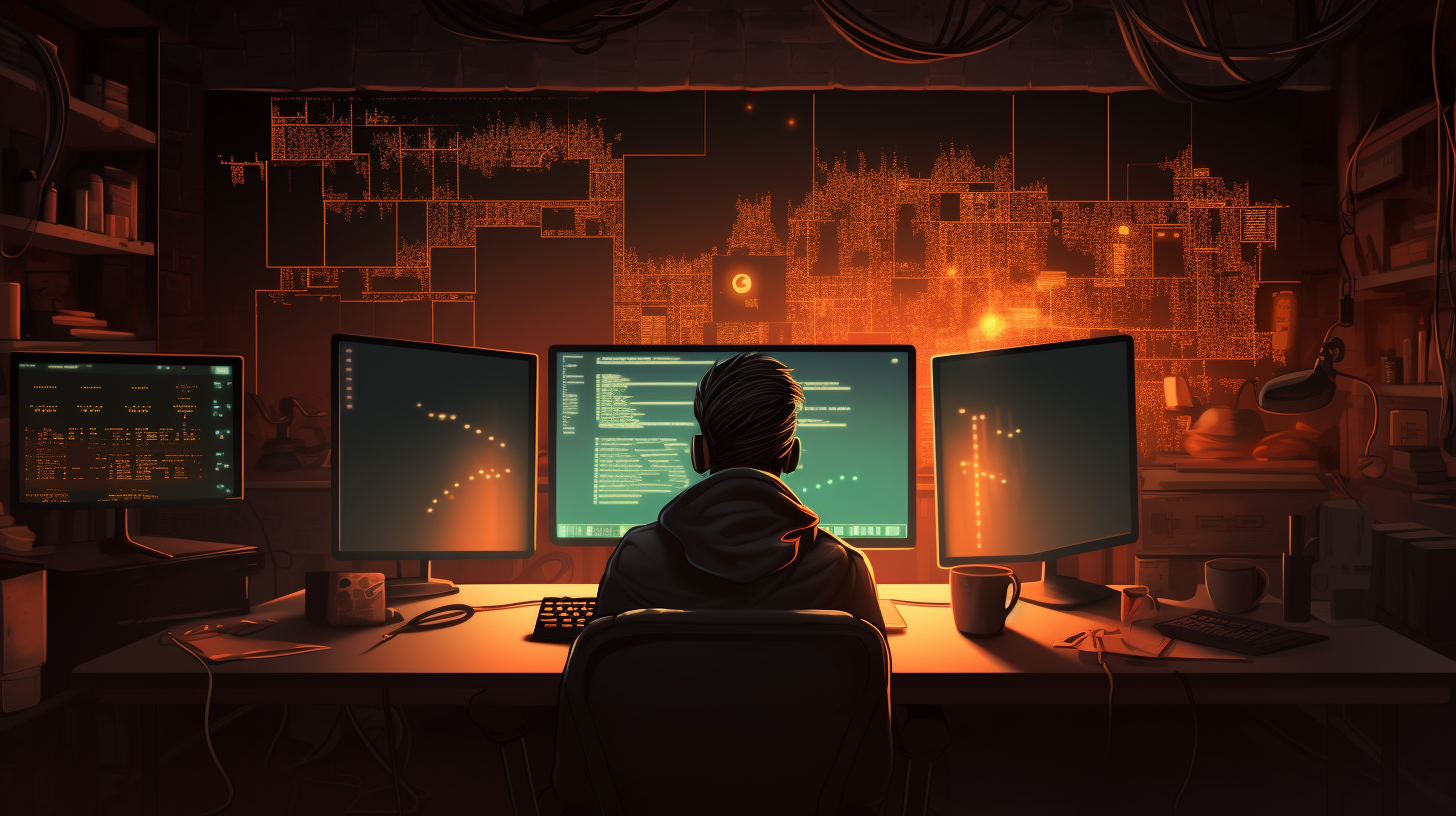
SQL for Data Relationship Mapping
Within the scope of databases, understanding data relationships is paramount; it’s the foundation upon which relational databases are built. Relationships define how data in one table relates to data in another, allowing us to create complex data structures that can efficiently manage and retrieve information. In SQL, recognizing these relationships is the first step toward effective data modeling and querying.
At its core, a data relationship is an association between two tables, often represented through shared keys. These keys help maintain data integrity and enforce constraints that ensure the accuracy of the data stored within our database. The primary types of data relationships include one-to-one, one-to-many, and many-to-many.
In a one-to-one relationship, a record in Table A corresponds to exactly one record in Table B. For instance, consider a database for a library where each book has a unique ISBN number. Here, each ISBN can belong to only one book, and each book can have only one ISBN.
CREATE TABLE Books ( ISBN VARCHAR(13) PRIMARY KEY, Title VARCHAR(255), AuthorID INT UNIQUE ); CREATE TABLE Authors ( AuthorID INT PRIMARY KEY, Name VARCHAR(255) );
The one-to-many relationship is perhaps the most common and occurs when a record in Table A can relate to multiple records in Table B. Using the library example again, an author can write multiple books. Therefore, the authors’ table can have multiple entries that correspond to the same author ID in the books table.
CREATE TABLE Authors ( AuthorID INT PRIMARY KEY, Name VARCHAR(255) ); CREATE TABLE Books ( ISBN VARCHAR(13) PRIMARY KEY, Title VARCHAR(255), AuthorID INT, FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID) );
In contrast, a many-to-many relationship occurs when multiple records in Table A relate to multiple records in Table B. For instance, ponder a scenario where students can enroll in multiple courses, and each course can have multiple students. To manage this relationship effectively, a junction table is necessary.
CREATE TABLE Students ( StudentID INT PRIMARY KEY, Name VARCHAR(255) ); CREATE TABLE Courses ( CourseID INT PRIMARY KEY, CourseName VARCHAR(255) ); CREATE TABLE Enrollments ( StudentID INT, CourseID INT, PRIMARY KEY (StudentID, CourseID), FOREIGN KEY (StudentID) REFERENCES Students(StudentID), FOREIGN KEY (CourseID) REFERENCES Courses(CourseID) );
Understanding these relationships not only helps in designing the database schema but also plays an important role in how we write queries to retrieve related data efficiently. The ability to navigate and manipulate these relationships using SQL is what empowers developers to create robust and responsive applications.
Types of Relationships: One-to-One, One-to-Many, and Many-to-Many
To fully grasp the implications of one-to-one, one-to-many, and many-to-many relationships, it’s vital to understand how they affect both the design of your database and the efficiency of your queries. Each type of relationship serves a specific purpose and can drastically influence the structure of your data model, affecting everything from normalization to query performance.
In a one-to-one relationship, the correspondence between records in Tables A and B is simpler. For example, if you decide to keep user profiles separate from their authentication credentials, you might create two tables: Users and UserCredentials. Each user in the Users table would correspond to exactly one entry in the UserCredentials table. This relationship ensures that sensitive data is compartmentalized, enhancing security.
CREATE TABLE Users ( UserID INT PRIMARY KEY, UserName VARCHAR(255) UNIQUE ); CREATE TABLE UserCredentials ( UserID INT PRIMARY KEY, PasswordHash VARCHAR(255), FOREIGN KEY (UserID) REFERENCES Users(UserID) );
In a one-to-many relationship, you increase complexity. This relationship is common in scenarios where a single entity (like a customer) can have multiple associated records (such as orders). Here, the Orders table would have a foreign key that references the Customers table, thereby allowing multiple orders for each customer without duplicating customer information.
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(255) ); CREATE TABLE Orders ( OrderID INT PRIMARY KEY, OrderDate DATETIME, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
With many-to-many relationships, the challenge escalates further. In this case, both tables involved can have multiple records that relate to each other, necessitating the use of a junction table to facilitate the relationship. In the university context, a single student can enroll in multiple courses, and a course can have multiple students. The Enrollments table acts as a bridge between Students and Courses, capturing this relationship effectively.
CREATE TABLE Enrollments ( StudentID INT, CourseID INT, PRIMARY KEY (StudentID, CourseID), FOREIGN KEY (StudentID) REFERENCES Students(StudentID), FOREIGN KEY (CourseID) REFERENCES Courses(CourseID) );
Recognizing these relationships very important when writing SQL queries. For instance, retrieving all courses a student is enrolled in involves a join between the Students, Enrollments, and Courses tables. Such queries can become intricate as the number of relationships grows, but they are essential for extracting meaningful insights from your data.
SELECT s.Name, c.CourseName FROM Students s JOIN Enrollments e ON s.StudentID = e.StudentID JOIN Courses c ON e.CourseID = c.CourseID WHERE s.Name = 'Luke Douglas';
Ultimately, understanding and implementing these relationships allows for a more organized database structure, which is key in ensuring data integrity and optimizing data retrieval processes. The choice of relationship type should align with the logic of your application, making it essential for developers to deliberate on the best approach for their specific use case.
Using Foreign Keys to Establish Relationships
Foreign keys are an integral part of establishing relationships between tables in SQL, enabling the enforcement of referential integrity. By using foreign keys, you can ensure that the data in one table is directly linked to valid records in another table, helping to prevent orphaned records and maintain the accuracy of your database. A foreign key in a child table points to a primary key in a parent table, establishing a connection that defines how records in one table relate to those in another.
For instance, let’s revisit the library example. We previously defined a relationship between authors and books. It is time to ensure that every book entry has a valid author reference by implementing foreign keys. Here, the AuthorID in the Books table acts as a foreign key that refers to the primary key in the Authors table.
CREATE TABLE Authors ( AuthorID INT PRIMARY KEY, Name VARCHAR(255) ); CREATE TABLE Books ( ISBN VARCHAR(13) PRIMARY KEY, Title VARCHAR(255), AuthorID INT, FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID) );
In this schema, the foreign key constraint on AuthorID ensures that any value inserted into the Books table must match an existing AuthorID in the Authors table. If an attempt is made to insert a book with an AuthorID that does not exist, the database will reject the operation, thereby maintaining data integrity.
Furthermore, foreign keys can provide cascading updates and deletions, which streamline the management of related data. If you decide to delete an author, you can set up cascading deletes so that all books associated with that author are automatically removed. This behavior ensures that your database remains clean and avoids leftover references that could lead to confusion.
CREATE TABLE Authors ( AuthorID INT PRIMARY KEY, Name VARCHAR(255) ); CREATE TABLE Books ( ISBN VARCHAR(13) PRIMARY KEY, Title VARCHAR(255), AuthorID INT, FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID) ON DELETE CASCADE );
In this example, when an author is deleted from the Authors table, all corresponding entries in the Books table will also be deleted automatically due to the cascading delete rule specified. This behavior can be highly beneficial in maintaining the integrity of your data across multiple tables.
Additionally, foreign keys can also enhance query performance. By defining relationships through foreign keys, the database can optimize join operations, making data retrieval more efficient. For example, when retrieving all books along with their respective authors, the query engine can leverage the foreign key relationship to streamline the process.
SELECT b.Title, a.Name FROM Books b JOIN Authors a ON b.AuthorID = a.AuthorID;
This query effectively utilizes the foreign key relationship to join the Books and Authors tables, so that you can fetch results where each book is neatly associated with its author, all thanks to the foreign key constraint you established earlier.
Using foreign keys not only establishes clear relationships between your data but also serves as a mechanism to uphold the integrity and organization of your database. As you design your database schema, consider the relationships that are essential for your application’s functionality, and strategically implement foreign keys to support those relationships.
Creating and Querying Joins for Data Retrieval
In SQL, joins are the mechanisms that allow you to combine rows from two or more tables based on related columns, enabling you to extract meaningful insights from interconnected data. There are several types of joins, each serving a distinct purpose and yielding different results based on the nature of the relationship between the tables involved. Understanding how to effectively create and query joins is important for working with relational databases.
One of the most fundamental types of joins is the INNER JOIN. This join returns only the rows that have matching values in both tables. For example, if you want to retrieve all books along with their respective authors, you would use an INNER JOIN to combine the Books and Authors tables on the AuthorID field.
SELECT b.Title, a.Name FROM Books b INNER JOIN Authors a ON b.AuthorID = a.AuthorID;
This query effectively fetches the title of each book along with the name of its author, but only for those books that have a valid author reference. If a book exists with an AuthorID that doesn’t correspond to any entry in the Authors table, it will not appear in the results.
Another common join is the LEFT JOIN (or LEFT OUTER JOIN), which returns all records from the left table and the matched records from the right table. If there’s no match, NULL values are returned for columns from the right table. This can be useful when you want to include all entries from the left table, regardless of whether there’s a corresponding entry in the right table. For instance, if you want to list all authors and their books, including authors who may not have written any books, you could structure the query as follows:
SELECT a.Name, b.Title FROM Authors a LEFT JOIN Books b ON a.AuthorID = b.AuthorID;
In this case, the result set will include all authors, and for those authors without any books in the Books table, the Title will be NULL.
For scenarios where you want to retrieve records that have no matching entries in the right table, you would use a RIGHT JOIN (or RIGHT OUTER JOIN). This join is less commonly used compared to the LEFT JOIN, but it serves a similar purpose, returning all records from the right table and matching records from the left table. If there are no matches, NULL values will appear for the left table columns.
SELECT b.Title, a.Name FROM Books b RIGHT JOIN Authors a ON b.AuthorID = a.AuthorID;
This query retrieves all authors again, but emphasizes those who have authored books, leaving NULLs for books that do not have a corresponding author.
The FULL OUTER JOIN combines the results of both LEFT JOIN and RIGHT JOIN. It returns all rows from both tables, with NULLs in places where there is no match. That is particularly useful when you want a comprehensive view of two tables, regardless of whether there are matching records in the other table.
SELECT a.Name, b.Title FROM Authors a FULL OUTER JOIN Books b ON a.AuthorID = b.AuthorID;
This query will return all authors and all books, showing NULL for authors without books and books without authors.
Another important join type is the CROSS JOIN, which produces a Cartesian product between the two tables. This means that each row from the first table is combined with every row from the second table, resulting in a potentially large dataset. CROSS JOIN is less frequently used but can be helpful in specific scenarios where you need to evaluate all possible combinations.
SELECT a.Name, b.Title FROM Authors a CROSS JOIN Books b;
This will return a list of every author with every book, regardless of actual relationships, often resulting in a large number of records.
When working with joins, it’s essential to be mindful of performance implications, especially as the size of the tables increases. Efficiently managing indices on the join keys can significantly improve query performance. Additionally, using the appropriate type of join based on your data needs will help you retrieve the relevant data efficiently.
As you build complex queries that involve multiple joins, it’s crucial to understand how to read and structure these queries clearly. Using aliases for your tables, as seen in the examples above, enhances readability and helps avoid confusion. Moreover, always test your queries to ensure they return the expected results, especially when working with large datasets where the implications of joins can change the nature of the result set dramatically.
Best Practices for Data Relationship Mapping in SQL
When it comes to data relationship mapping in SQL, adhering to best practices is essential for designing robust, efficient, and maintainable database systems. These practices not only enhance the clarity of your database schema but also ensure that your queries run efficiently, enabling smoother data retrieval and manipulation.
One of the cornerstones of effective data relationship mapping is normalization. Normalization is the process of organizing data to minimize redundancy and dependency. By dividing large tables into smaller, related tables and defining relationships between them, you maintain a clean data structure. This practice reduces the risk of data anomalies and enhances the integrity of your database. Common normalization forms include the first (1NF), second (2NF), and third normal forms (3NF), each with specific rules to ensure that data is stored logically.
For instance, consider a scenario where we have a table for orders that includes customer information alongside order details. To better normalize this structure, you could separate customer data into its own table, linking it to the orders table through a foreign key:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(255) ); CREATE TABLE Orders ( OrderID INT PRIMARY KEY, OrderDate DATETIME, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
This separation adheres to normalization principles, reducing redundancy as customer information is stored in one place, while orders reference this data using foreign keys.
Another best practice involves clearly defining your primary keys and foreign keys. Primary keys uniquely identify each record in a table, while foreign keys create a connection between tables. Choose meaningful keys that reflect the entities they represent. This aids in understanding the relationships and enhances query performance. Additionally, ponder indexing your foreign keys to speed up join operations.
For example, if you have a foreign key on the Orders table referencing the Customers table, indexing the CustomerID on both tables can significantly improve the performance of queries that involve joins:
CREATE INDEX idx_CustomerID ON Orders(CustomerID); CREATE INDEX idx_CustomerID ON Customers(CustomerID);
When querying your data, using joins effectively is another critical best practice. Always use the most appropriate type of join for your use case—INNER JOINs for matching records, LEFT JOINs for including all records from one table regardless of matches, and so on. This ensures that your queries remain efficient and return the desired results without unnecessary complexity.
For complex relationships, consider using Common Table Expressions (CTEs) for better readability and organization of your queries. CTEs can simplify complex joins and make your SQL statements easier to understand:
WITH CustomerOrders AS ( SELECT O.OrderID, O.OrderDate, C.CustomerName FROM Orders O JOIN Customers C ON O.CustomerID = C.CustomerID ) SELECT * FROM CustomerOrders;
Additionally, always validate and sanitize input data to prevent SQL injection attacks and maintain the integrity of your database. Employ parameterized queries whenever possible, as they provide a safeguard against malicious input:
SELECT * FROM Customers WHERE CustomerID = ?;
Finally, document your database schema and relationships thoroughly. Clear documentation aids in onboarding new developers and makes maintaining the database structure over time much easier. It also serves as a reference point when you need to revisit your design or troubleshoot issues.
Implementing these best practices for data relationship mapping in SQL not only fosters a cleaner and more efficient database design but also paves the way for effective data handling within your applications. By focusing on normalization, key definitions, appropriate joins, and comprehensive documentation, you can create a solid foundation for your database that supports both current and future requirements.