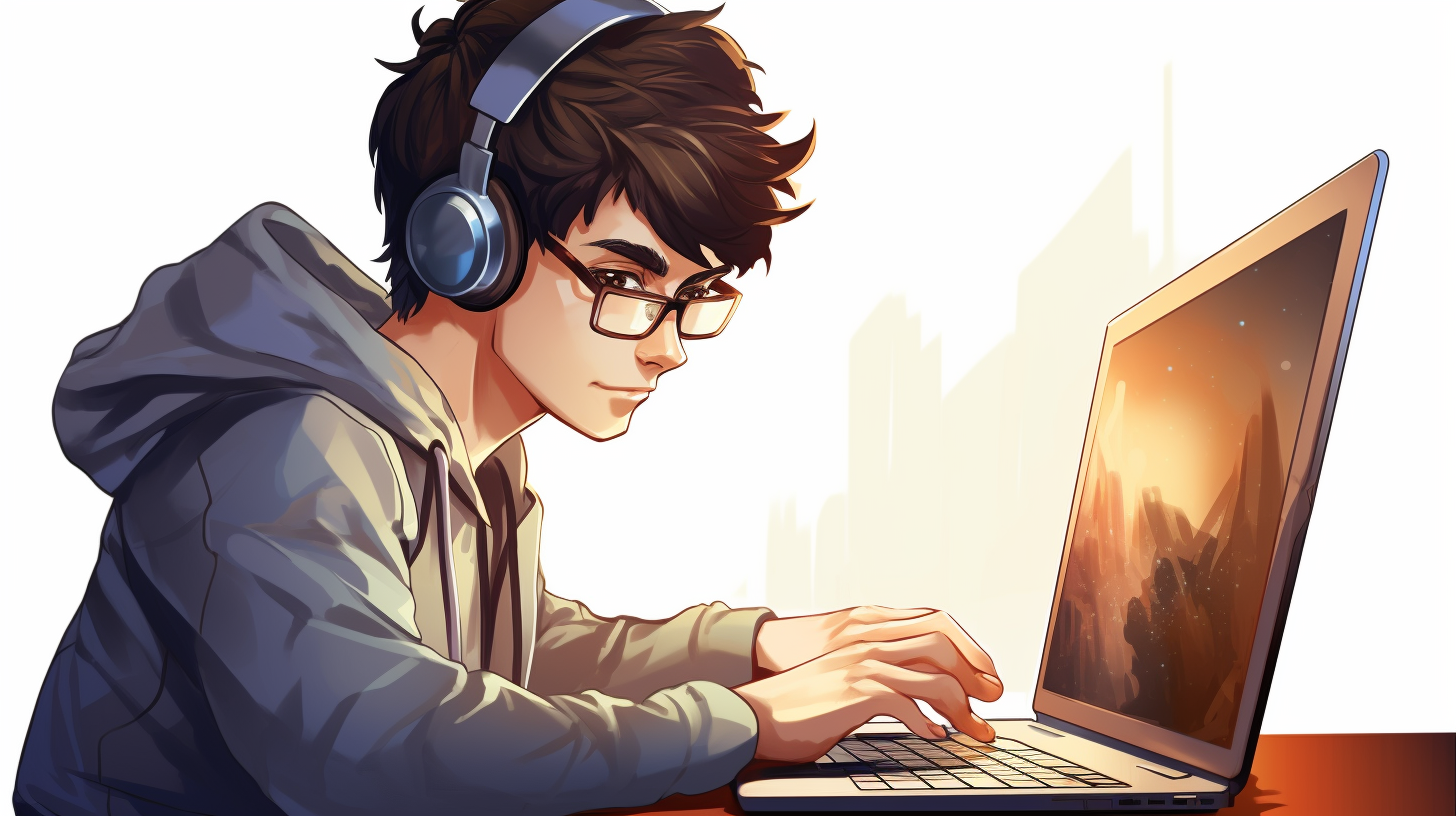
Bash Scripting for Network Mapping
Network mapping is an essential skill in the arsenal of anyone looking to manage networks effectively. It involves identifying the devices on a network, their interconnections, and the services they offer. Understanding network mapping concepts especially important for troubleshooting issues, optimizing network performance, and securing the network against unauthorized access.
At its core, network mapping revolves around discovering devices and services on a network. This can be achieved through various methods, including ping sweeps, port scanning, and service identification. Each method provides different insights into the network’s topology and operational status.
Ping Sweeping is a technique used to identify active devices on a network. By sending ICMP echo requests to a range of IP addresses, you can determine which devices are reachable. That is often the first step in network mapping.
#!/bin/bash # Simple ping sweep script for i in {1..254}; do ping -c 1 192.168.1.$i > /dev/null if [ $? -eq 0 ]; then echo "192.168.1.$i is up" fi done
Next, port scanning allows you to discover open ports on a device, revealing which services are running. Tools like nmap
are often employed for this purpose. Port scanning can help identify vulnerabilities in networked devices.
# Basic nmap command for port scanning nmap -sS 192.168.1.1
Another important concept is service identification, which is used to determine the software and versions running on open ports. This information can be critical for both security assessments and network optimization.
In addition to these basic techniques, more advanced concepts such as topology mapping can provide a visual representation of the network layout, showing how devices are interconnected. This can be achieved through various tools and protocols that collect data about the network’s structure.
Understanding these foundational concepts of network mapping allows you to utilize Bash scripting to automate the discovery and analysis process, making your network management tasks more efficient and effective.
Essential Bash Commands for Network Discovery
When it comes to network discovery, a few essential Bash commands can usher in a new level of efficiency and automation. These commands not only help gather information about active devices on a network but also provide insights into their operational states and configurations.
One of the most simpler yet powerful commands for network discovery is ping
. As mentioned earlier, pinging a range of IP addresses can reveal which devices are active. However, for more comprehensive discovery, the following commands become invaluable:
1. ARP (Address Resolution Protocol)
The arp
command allows you to view the MAC addresses of devices communicating with your system. It’s particularly useful for confirming the presence of devices on the same local network.
arp -a
This command will list all IP addresses and their corresponding MAC addresses in your local ARP table. If you’ve recently pinged devices on your network, this will show you which devices replied.
2. Netstat
The netstat
command can be used to display current network connections, routing tables, and interface statistics. That is useful not only for monitoring active connections but also for diagnosing network issues.
netstat -tuln
This command provides a list of all listening ports and their associated services. The flags -t
, -u
, -l
, and -n
specify TCP, UDP, listening state, and numerical output, respectively, making it simple to see which services are available on your device.
3. Nmap
Though a more advanced tool, nmap
is invaluable for performing deeper scans of your network. With just a few options, you can discover hosts, open ports, and identify services running on those ports.
nmap -sP 192.168.1.0/24
This command performs a ping scan and lists all devices that are up within the specified subnet, giving you a quick overview of your network devices.
4. traceroute
The traceroute
command is another essential tool for network mapping. It traces the route packets take to reach a specific destination, showing each hop along the way.
traceroute 8.8.8.8
This can help diagnose where packets are being lost or delayed, giving insight into potential bottlenecks or misconfigurations in the network.
5. curl
The curl
command can be used to request information from web services, making it a useful tool for checking the availability of services running on your devices.
curl -I http://192.168.1.1
This command retrieves the HTTP headers from the web service running on the specified IP, which will allow you to see if the service is up and what responses it provides.
By using these essential Bash commands, network discovery can be both simplified and enhanced. Employing scripts that incorporate these commands allows for automated processes that can quickly gather comprehensive information about the network landscape, empowering administrators to make informed, data-driven decisions.
Creating a Basic Network Mapping Script
Creating a basic network mapping script in Bash can serve as a practical gateway to automating network discovery tasks. By using the essential commands previously discussed, you can develop a script that scans your network for active devices, identifies open ports, and retrieves service information. This foundational script will not only provide a hands-on introduction to network mapping but also lay the groundwork for more advanced scripting techniques.
Here’s a simple example of how to create a Bash script to perform a basic network scan using ping and nmap:
#!/bin/bash # Network address to scan NETWORK="192.168.1" # File to store results OUTPUT_FILE="network_scan_results.txt" # Clear previous results > $OUTPUT_FILE echo "Starting network scan on $NETWORK.0/24" | tee -a $OUTPUT_FILE # Step 1: Ping sweep to find active devices for i in {1..254}; do if ping -c 1 -W 1 $NETWORK.$i > /dev/null; then echo "$NETWORK.$i is up" | tee -a $OUTPUT_FILE # Step 2: Perform nmap scan on the active device echo "Scanning $NETWORK.$i for open ports..." | tee -a $OUTPUT_FILE nmap -sS $NETWORK.$i | tee -a $OUTPUT_FILE echo "" | tee -a $OUTPUT_FILE # Add a blank line for readability fi done echo "Network scan completed. Results saved to $OUTPUT_FILE." | tee -a $OUTPUT_FILE
In this script, we first specify the network address we want to scan. The script initializes an output file that will contain the results of the scan. It then performs a ping sweep across the entire subnet, checking each IP address from .1 to .254 to see which devices are active.
For each responsive device, it uses nmap to perform a TCP SYN scan to identify open ports. The results are appended to the output file, which can be reviewed after the scan completes. This simple structure allows easy modifications; for example, you could enhance the nmap command with additional options to retrieve more detailed service information or conduct different types of scans.
Executing this script will yield valuable data about your network’s status and layout. Not only does it provide insights into active devices, but it also reveals potential vulnerabilities by listing open ports. This foundational script can be expanded with error handling, optimizations for larger networks, or integrated into more advanced network management systems.
As you become more comfortable with Bash scripting for network mapping, consider exploring additional features such as logging, scheduled runs with cron jobs, or integrating with tools like grep and awk for focused data extraction. This ongoing enhancement will deepen your understanding and effectiveness in managing network environments.
Advanced Techniques for Automated Network Analysis
When venturing into advanced techniques for automated network analysis, it’s essential to go beyond simple discovery scripts. It involves not only gathering information but also analyzing it dynamically and adapting your approach based on real-time data. This means integrating various tools and methodologies to create a comprehensive network monitoring solution that can respond to changes and provide deeper insights.
One powerful approach is the combination of Bash scripting with network analysis tools like nmap, tcpdump, and snort. By using these tools, you can set up scripts that not only scan and discover devices but also monitor traffic patterns and detect anomalies.
For instance, you can create a script that runs nmap periodically to check for new devices or changes in the network configuration. This can be particularly useful in environments that experience frequent changes, such as dynamic IP allocations or new device connections.
#!/bin/bash # Network address to monitor NETWORK="192.168.1.0/24" # File to log changes LOG_FILE="network_changes.log" # Function to perform nmap scan and log results scan_network() { echo "Scanning network $NETWORK for changes..." | tee -a $LOG_FILE nmap -sn $NETWORK | grep "Nmap scan report" | tee -a $LOG_FILE } # Initial scan scan_network # Monitor for changes (run every 60 seconds) while true; do sleep 60 NEW_SCAN=$(nmap -sn $NETWORK | grep "Nmap scan report") if [ "$NEW_SCAN" != "$(tail -n 10 $LOG_FILE)" ]; then echo "Network change detected!" | tee -a $LOG_FILE scan_network fi done
In this script, we use nmap to perform a ping scan and log the results. The script runs an initial scan and enters an infinite loop where it sleeps for 60 seconds before scanning again. If changes are detected based on the output, the script logs those changes and rescans the network. This allows for continuous monitoring of the network and immediate awareness of changes.
Another advanced technique is using tcpdump for traffic analysis. This tool allows you to capture and analyze packets in real-time, providing insights into what data flows across your network. Integrating tcpdump with Bash can enhance your ability to monitor network health and detect suspicious behavior.
#!/bin/bash # Interface to monitor INTERFACE="eth0" # File to store packet capture CAPTURE_FILE="network_traffic.pcap" # Start tcpdump to capture packets echo "Capturing network traffic on $INTERFACE..." | tee -a $CAPTURE_FILE tcpdump -i $INTERFACE -w $CAPTURE_FILE
This script captures network packets on the specified interface and writes them to a file. You can later analyze this file with tools like Wireshark for a detailed examination of the traffic patterns, which can help in identifying unauthorized access or performance bottlenecks.
To further enhance your automation, consider scheduling these scripts using cron. This allows you to run your network scans and traffic analysis at regular intervals, creating a proactive monitoring environment. Here’s an example of how to add a cron job:
# Open the cron table for editing crontab -e # Add the following line to run the network scan every hour 0 * * * * /path/to/your/network_scan_script.sh
Additionally, using tools like snort can help you analyze traffic for potential threats. Snort can be configured to run alongside your scripts, providing alerts for suspicious activities based on predefined rules. Integrating such a system creates a robust framework for automated network analysis, ensuring that you’re not only aware of changes but are also equipped to respond effectively.
By mastering these advanced techniques, you can transform your Bash scripts into powerful tools for real-time network analysis, ensuring that you maintain control over your network’s security and performance. The level of automation and analysis achievable with these methods can significantly enhance your operational efficiency and responsiveness to network events.