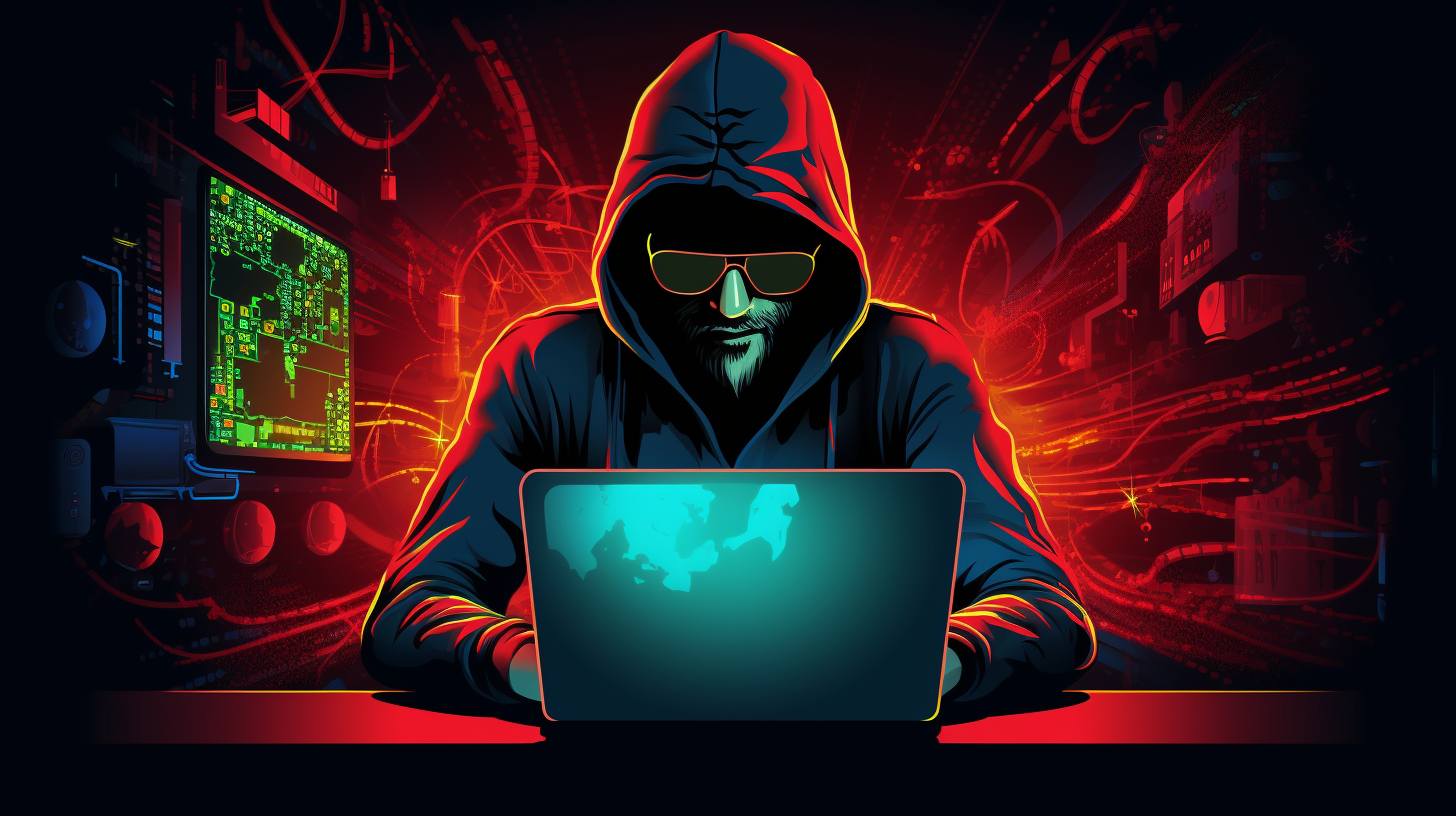
Implementing SQL for Data Synchronization
Data synchronization is an important aspect of modern applications as it ensures that data is consistent across multiple systems. The techniques employed for data synchronization vary widely, depending on the requirements of the application and the underlying architecture. Understanding these techniques is essential for implementing a robust synchronization strategy.
Broadly, data synchronization can be classified into two categories: real-time synchronization and batch synchronization.
- This approach updates data in real-time, making changes to the database immediately as they occur. It’s often achieved using methods like change data capture (CDC) or event-driven architectures. This technique is perfect for applications requiring instant access to the latest data.
- In contrast, batch synchronization processes data in intervals, aggregating changes and applying them at set times. This method is typically more efficient in terms of resources but may not be suitable for applications demanding up-to-the-minute accuracy.
Another important concept is one-way vs. two-way synchronization. One-way synchronization allows data to flow in a single direction—from a source to a target—while maintaining data integrity on the target. Two-way synchronization, on the other hand, allows changes to be made at both ends, necessitating mechanisms to handle conflicts that may arise when the same data is changed in both systems.
Considerations regarding network performance and data volume also significantly influence the approach to synchronization. For instance, when large datasets are involved, it may be more efficient to employ a selective synchronization strategy instead of syncing entire tables.
In any synchronization strategy, it’s vital to incorporate error handling and monitoring to detect and respond to issues as they occur. Regular logging of synchronization activities can also help identify performance bottlenecks and system errors.
Here’s an example of a basic SQL script for implementing a real-time data synchronization mechanism using triggers:
CREATE TRIGGER after_insert_customers AFTER INSERT ON customers FOR EACH ROW BEGIN INSERT INTO customer_sync (customer_id, name, email) VALUES (NEW.customer_id, NEW.name, NEW.email); END;
This trigger automatically pushes new records from the customers table to a customer_sync table whenever a new record is inserted, facilitating real-time data synchronization.
Choosing the Right Database for Synchronization
When it comes to choosing the right database for synchronization, several factors play a pivotal role in determining the most suitable option for your specific needs. The decision is not merely a matter of preference but involves an in-depth analysis of the application’s requirements, scalability, performance, and the nature of the data being synchronized.
One crucial aspect to ponder is the type of database management system (DBMS) that aligns with your synchronization goals. Relational databases like PostgreSQL and MySQL offer robust support for SQL queries and are optimal for applications requiring structured data. Conversely, NoSQL databases like MongoDB or Cassandra excel in scenarios where flexibility and scalability are paramount, especially for handling unstructured or semi-structured data.
Scalability is another significant factor. As your application grows, the database should be able to handle increased loads without degradation in performance. For instance, if the application involves heavy read and write operations, a distributed database system may be necessary to balance the load effectively. On the other hand, if the application operates in a predominantly read-heavy environment, options like read replicas could be beneficial.
Performance characteristics of the database also impact synchronization efficiency. Some databases offer built-in support for change data capture (CDC), which can facilitate real-time data synchronization with minimal latency. Understanding how a database manages transaction logs and how quickly it can propagate changes across instances will inform decisions on synchronization strategy.
Data consistency models are essential to evaluate as well. Different databases implement consistency in various ways—some favor eventual consistency, while others provide strong consistency guarantees. Choosing a database that aligns with the desired consistency model for your application can help avoid data anomalies during synchronization. For example:
SELECT * FROM orders WHERE order_status = 'pending' AND last_updated > NOW() - INTERVAL 1 HOUR;
This query highlights how one might retrieve records in a pending state that have been updated in the last hour, a critical consideration when ensuring that synchronization processes maintain the integrity of the data being transferred.
Finally, consider the operational overhead of maintaining the chosen database system. Some databases require extensive administrative resources to ensure optimal performance and security, while others come with managed services that reduce the burden on development teams. Using cloud-based databases can simplify synchronization processes by providing built-in features for scalability and real-time data access.
SQL Queries for Efficient Data Sync
When it comes to the actual execution of data synchronization, SQL queries play a pivotal role in ensuring that the process is efficient and reliable. Crafting optimized SQL queries is essential for minimizing data transfer times and reducing the load on both the source and target databases. This section highlights various SQL strategies that can be employed for efficient data synchronization.
One critical aspect of data synchronization is using SELECT statements effectively to identify which records need to be synchronized. This often involves tracking changes through timestamps or versioning columns. For instance, think a scenario where you want to synchronize records that have been updated since the last sync:
SELECT * FROM source_table WHERE last_modified > @last_sync_time;
This query retrieves all records from the source_table that have been modified after the last synchronization time, represented by the variable @last_sync_time. It very important to ensure that the data types for timestamps are consistent across systems to prevent discrepancies.
Once the changes are identified, the next step is to transfer them to the target database. This can be accomplished through INSERT and UPDATE statements. Here’s a sample of how you might insert new records into the target table:
INSERT INTO target_table (id, data, last_modified) VALUES (?, ?, ?) ON DUPLICATE KEY UPDATE data = VALUES(data), last_modified = VALUES(last_modified);
This query uses the ON DUPLICATE KEY UPDATE clause to handle records that might already exist in the target_table. By updating existing records with new values, it ensures that the target database remains current without creating redundant entries.
Moreover, handling deletions efficiently is also an important part of data synchronization. If records are removed from the source, the corresponding records should ideally be deleted from the target as well. A simple DELETE operation can be scripted as follows:
DELETE FROM target_table WHERE id NOT IN (SELECT id FROM source_table);
This command deletes records from the target_table that are no longer present in the source_table, ensuring that the target remains an accurate representation of the source.
Additionally, using transactions can help maintain data integrity during synchronization. Wrapping your inserts and updates within a transaction ensures that all operations succeed or fail together, which is vital in preventing partial updates that could lead to inconsistencies:
BEGIN; -- Insert new records INSERT INTO target_table (id, data, last_modified) VALUES (?, ?, ?); -- Update existing records UPDATE target_table SET data = ?, last_modified = ? WHERE id = ?; COMMIT;
Using transactions effectively also allows for implementing rollback mechanisms in case of errors, thus preserving data integrity during the synchronization process.
Lastly, indexing plays a critical role in optimizing the performance of synchronization operations. Indexes on columns that are frequently queried or updated can drastically improve the performance of SELECT and UPDATE operations. For example:
CREATE INDEX idx_last_modified ON source_table(last_modified);
Creating an index on the last_modified column ensures that the query used to fetch updated records runs efficiently, minimizing response times and reducing the overall load on the database.
Handling Conflicts in Data Synchronization
Handling conflicts in data synchronization is a nuanced challenge that can significantly impact the integrity and consistency of shared data across systems. As applications evolve and data flows between varied sources—like databases, cloud services, and microservices—the likelihood of conflicts increases, especially in two-way synchronization scenarios. Conflict resolution strategies become paramount to maintain a coherent state of data while ensuring that all updates are appropriately managed.
Conflicts often arise when the same data item is modified in multiple locations at once. Consider two users editing the same record in different systems; without a robust conflict resolution mechanism, the last change could overwrite the other, leading to potential data loss. Thus, defining clear rules for conflict resolution is essential, and several strategies can be employed to manage these situations effectively.
One common approach is the Last Write Wins (LWW) strategy, where the most recent update, based on a timestamp, is retained. While this method is simpler to implement, it may lead to undesirable outcomes if critical updates are inadvertently overwritten. For example, if one user updates a record just seconds before another, only the latest change will persist, which may not always reflect the intended outcome of both users.
UPDATE target_table SET data = new_data, last_modified = NOW() WHERE id = record_id AND last_modified < new_last_modified;
In the above SQL statement, the new update will only be accepted if its timestamp is later than the existing record’s timestamp, effectively implementing the LWW strategy.
Another widely used strategy is Version Control. Here, each record is assigned a version number that increments with each update. When synchronizing data, if the version number in the target does not match the source, a conflict is detected, and the system can prompt for human intervention or apply a pre-defined resolution strategy. This method provides more granular control over updates and helps in tracking changes more effectively.
UPDATE target_table SET data = new_data, version = version + 1 WHERE id = record_id AND version = old_version;
In this code snippet, the update will succeed only if the version in the target matches the expected version. If there’s a discrepancy, the application can alert the user or log the conflict for review.
Additionally, Merge Strategies can be implemented, particularly in applications where multiple updates are valid. This approach may involve merging changes from both sources into a single record. For instance, if two users modify different fields of the same record, the system can combine their changes rather than choosing one over the other. This requires a well-designed schema that supports such operations and may involve significant logic on the application side to manage merging complex data structures.
UPDATE target_table SET field1 = COALESCE(source.field1, target.field1), field2 = COALESCE(source.field2, target.field2), last_modified = NOW() FROM source_table AS source WHERE target.id = source.id;
In this example, COALESCE
is used to retain values from both the source and target where applicable, allowing for a more nuanced update process, preserving data from both sides.
Moreover, employing a Conflict Log is beneficial for tracking discrepancies and resolutions over time. By maintaining a log of conflicts, teams can review how often conflicts occur and their nature, leading to improved strategies for future synchronization processes. This log can be implemented as follows:
INSERT INTO conflict_log (record_id, source_timestamp, target_timestamp, resolution) VALUES (record_id, source_timestamp, target_timestamp, 'Resolved by Version Control');
Lastly, user intervention remains an important aspect in complex scenarios where automatic resolution might lead to data inconsistency. Providing a simple to operate interface that allows users to review and resolve conflicts manually can enhance the overall user experience and ensure data integrity.
Best Practices for Maintaining Data Integrity
Maintaining data integrity during synchronization processes is paramount, as it ensures that the data remains accurate, consistent, and reliable across all systems involved. Several best practices can be adopted to uphold data integrity throughout synchronization activities, regardless of the techniques or technologies utilized.
One of the foundational principles is to establish a clear schema for your databases. This schema should define data types, constraints, and relationships among tables to minimize the risk of data anomalies during synchronization. Data validation rules should also be enforced, both at the application level and within the database, to ensure that only valid data enters the system. For example, consider a scenario where customer ages are synchronized. It is critical to enforce a constraint to validate that age is a positive integer:
ALTER TABLE customers ADD CONSTRAINT chk_age CHECK (age >= 0);
Another significant practice is the implementation of transactions when performing data synchronization operations. Transactions allow multiple operations to be grouped into a single unit, ensuring that either all operations succeed, or none do. This atomicity especially important for maintaining data integrity, as it prevents partial updates from leaving the database in an inconsistent state. Think the following SQL transaction that handles a customer update:
BEGIN; UPDATE customers SET email = '[email protected]' WHERE customer_id = 123; INSERT INTO customer_sync (customer_id, email, sync_time) VALUES (123, '[email protected]', NOW()); COMMIT;
In this example, both the update to the customers table and the insert into the customer_sync table are part of a single transaction. If either operation fails, the entire transaction is rolled back, ensuring that no partial changes persist in the system.
Regular audit trails are also essential for data integrity. By maintaining logs of all operations applied during synchronization, it becomes possible to track changes and identify potential issues. This practice allows for easier troubleshooting and compliance with data governance policies. An example of inserting an audit record might look like this:
INSERT INTO audit_log (operation, table_name, record_id, timestamp) VALUES ('UPDATE', 'customers', 123, NOW());
Additionally, data reconciliation processes should be established to periodically verify that the synchronized data across systems is consistent. This can be accomplished through checksum validations or by comparing row counts and sample records between source and target systems. A simple check for discrepancies might involve comparing sums, like so:
SELECT SUM(data_field) AS source_sum FROM source_table; SELECT SUM(data_field) AS target_sum FROM target_table;
If the sums do not match, it indicates a potential inconsistency that requires further investigation.
Furthermore, implementing safeguards against concurrency issues is vital when multiple processes might attempt to update the same data at the same time. Techniques such as optimistic concurrency control can be employed, where a version number or timestamp is checked before an update proceeds. This ensures that updates are only applied if the data has not been modified since it was last read:
UPDATE target_table SET data = new_data, last_modified = NOW() WHERE id = record_id AND last_modified = old_last_modified;
Finally, fostering a culture of regular backups and recovery planning is another essential aspect of maintaining data integrity. In the event of a synchronization failure or data corruption, having robust backup systems in place allows for quick restoration to a reliable state.