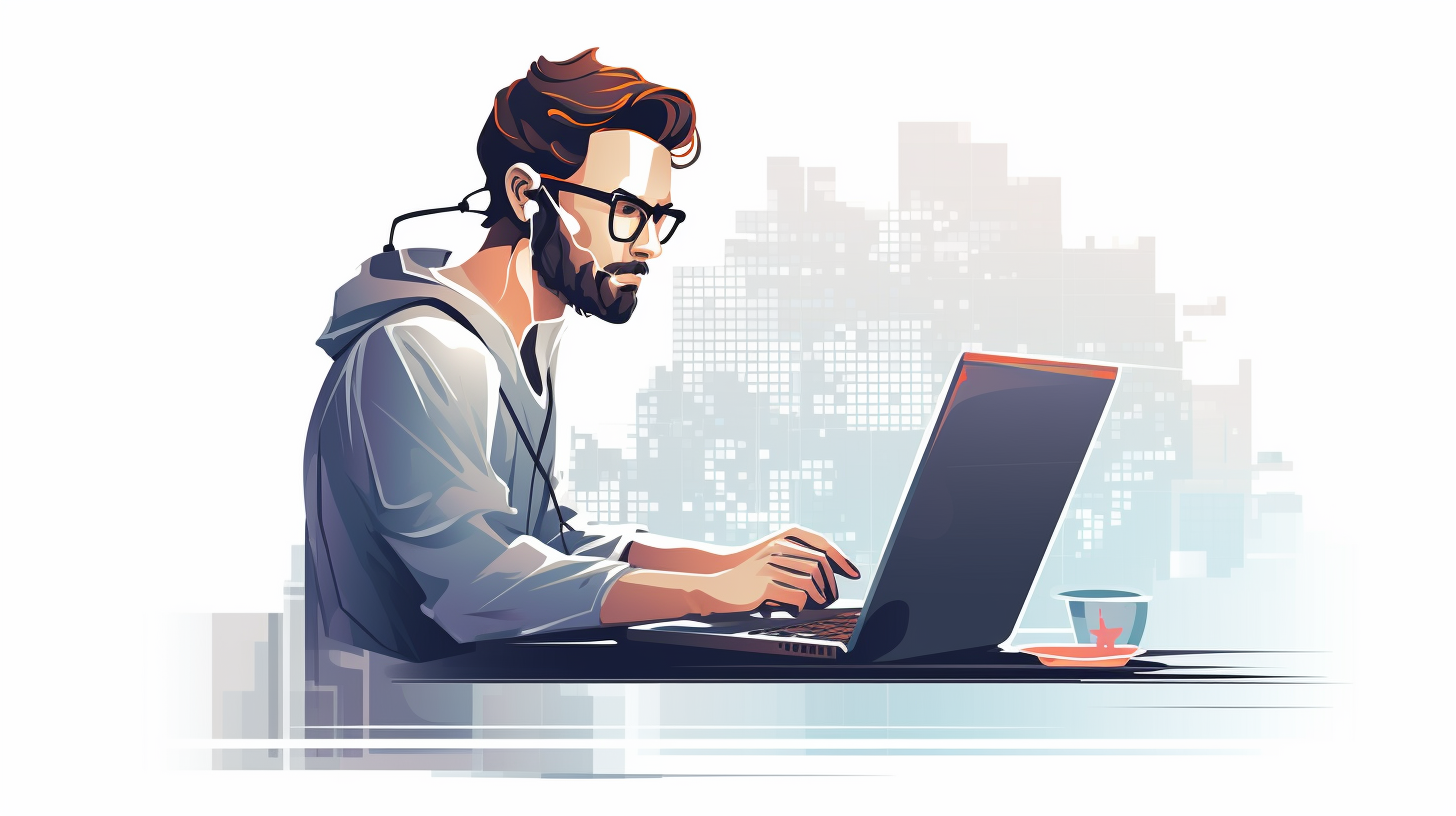
Swift and WidgetKit
WidgetKit is a powerful framework introduced by Apple that allows developers to create rich, informative widgets for iOS, macOS, and watchOS. It fundamentally changes how users interact with apps by providing glanceable information that resides right on the home screen or in the Today View. Understanding the architecture and functionality of WidgetKit very important for anyone looking to leverage this framework effectively.
At its core, WidgetKit operates on the principle of providing timely and relevant information while maintaining system performance and battery efficiency. This is achieved through a declarative syntax that allows developers to describe their widgets in a simpler manner. The framework abstracts away many complexities, letting developers focus on delivering the best user experience.
Widgets in WidgetKit are composed of three primary components: Timeline, Widget Configuration, and View. The Timeline defines how often your widget updates and what data it displays at any given time. By using a timeline, you can schedule updates to ensure your widget shows fresh content according to the user’s needs.
The Widget Configuration defines the parameters of the widget, such as its size and kind. Each widget can have multiple configurations, allowing developers to provide users with options tailored to their preferences. With the view, you define how the content appears visually. This separation of concerns promotes modularity, making it easier to manage and update your widgets.
Here is a simple example that illustrates how to define a basic widget using WidgetKit:
import WidgetKit import SwiftUI struct SimpleWidgetEntry: TimelineEntry { let date: Date let configuration: ConfigurationIntent } struct SimpleWidgetProvider: TimelineProvider { func placeholder(in context: Context) -> SimpleWidgetEntry { SimpleWidgetEntry(date: Date(), configuration: ConfigurationIntent()) } func getSnapshot(in context: Context, completion: @escaping (SimpleWidgetEntry) -> ()) { let entry = SimpleWidgetEntry(date: Date(), configuration: ConfigurationIntent()) completion(entry) } func getTimeline(in context: Context, completion: @escaping (Timeline) -> ()) { var entries: [SimpleWidgetEntry] = [] let currentDate = Date() let entry = SimpleWidgetEntry(date: currentDate, configuration: ConfigurationIntent()) entries.append(entry) let timeline = Timeline(entries: entries, policy: .atEnd) completion(timeline) } } struct SimpleWidget: Widget { let kind: String = "SimpleWidget" var body: some WidgetConfiguration { IntentConfiguration(kind: kind, provider: SimpleWidgetProvider()) { entry in SimpleWidgetEntryView(entry: entry) } .configurationDisplayName("Simple Widget") .description("A simple example widget.") .supportedFamilies([.systemSmall, .systemMedium]) } }
This example showcases a simple widget with a basic timeline provider. The SimpleWidgetEntry
serves as the data model, while SimpleWidgetProvider
deals with lifecycle events. The SimpleWidget
structure encapsulates the widget configuration, including details like display name and description.
By understanding these core concepts and structures, developers can create engaging, performant widgets that can seamlessly integrate into the user’s daily routine, enhancing their interaction with app data in a meaningful way.
Getting Started with Widget Creation
Creating your first widget with WidgetKit involves setting up a few essential components and understanding how they interact within the framework. The first step is ensuring that your app has the correct entitlements and capabilities enabled in the project settings. Make sure you have the appropriate permissions and that your app is configured to support widgets.
Once you have ensured that everything is configured correctly, you can start by defining your widget’s timeline. The timeline especially important as it governs the updating mechanism of your widget, specifying when to refresh the displayed content. Let’s expand our example to include a simple data model that our widget will use:
struct QuoteEntry: TimelineEntry { let date: Date let quote: String }
Next, we will modify our `TimelineProvider` to fetch daily quotes. This will involve fetching data asynchronously, allowing users to see a new quote every day:
struct QuoteProvider: TimelineProvider { func placeholder(in context: Context) -> QuoteEntry { QuoteEntry(date: Date(), quote: "Keep pushing your limits!") } func getSnapshot(in context: Context, completion: @escaping (QuoteEntry) -> ()) { let entry = QuoteEntry(date: Date(), quote: "Live as if you were to die tomorrow.") completion(entry) } func getTimeline(in context: Context, completion: @escaping (Timeline) -> ()) { var entries: [QuoteEntry] = [] let currentDate = Date() // Create an entry for today let todayEntry = QuoteEntry(date: currentDate, quote: fetchQuote()) entries.append(todayEntry) // Create an entry for tomorrow let nextDate = Calendar.current.date(byAdding: .day, value: 1, to: currentDate)! let tomorrowEntry = QuoteEntry(date: nextDate, quote: fetchQuote()) entries.append(tomorrowEntry) let timeline = Timeline(entries: entries, policy: .after(nextDate)) completion(timeline) } func fetchQuote() -> String { // Placeholder function to simulate fetching a quote // In a real app, you might fetch this from an API or a local database return "The only limit to our realization of tomorrow is our doubts of today." } }
In this modification, the `QuoteProvider` is responsible for generating timeline entries with unique quotes. The `fetchQuote` function simulates the acquisition of a quote, which can eventually be replaced with real data-fetching logic as needed.
After defining the timeline provider, we need to update our widget view to display the quotes. The view will take an entry and present the quote in a visually appealing way. Here’s how you can implement the view for our widget:
struct QuoteWidgetEntryView : View { var entry: QuoteEntry var body: some View { Text(entry.quote) .font(.headline) .padding() .background(Color.blue.opacity(0.1)) .cornerRadius(8) } }
This view simply displays the quote in a styled text view. The use of SwiftUI allows for easy customization of the appearance of the widget, which is important for ensuring it fits well within the overall design of the user’s home screen.
Finally, the widget configuration must be updated to reference the new provider and view:
struct QuoteWidget: Widget { let kind: String = "QuoteWidget" var body: some WidgetConfiguration { StaticConfiguration(kind: kind, provider: QuoteProvider()) { entry in QuoteWidgetEntryView(entry: entry) } .configurationDisplayName("Daily Quote Widget") .description("Displays a new motivational quote every day.") .supportedFamilies([.systemSmall, .systemMedium]) } }
Now you have a functional widget that displays a new quote each day, keeping the content fresh and engaging for users. As you continue to explore WidgetKit, consider adding more interactive elements, such as tapping on the widget to launch the app with more details or additional options.
Design Principles for Widgets
Designing widgets in WidgetKit requires a keen understanding of user experience and aesthetic considerations. When crafting a widget, developers must strive to create a visually appealing interface that communicates information effectively at a glance. This process begins with defining the widget’s purpose and identifying the primary content that users will find valuable.
Clarity and Brevity: A fundamental principle in widget design is clarity. Given the limited space available on the home screen, it is critical to convey the most pertinent information without overwhelming the user. Each element within the widget should serve a purpose. For instance, if you are displaying weather data, focus solely on the most relevant metrics like temperature and conditions, rather than cluttering the interface with unnecessary details.
Ponder this example, where we simplify a weather widget to focus only on essential information:
struct WeatherWidgetEntryView: View { var entry: WeatherEntry var body: some View { VStack { Text(entry.temperature) .font(.largeTitle) .fontWeight(.bold) Text(entry.condition) .font(.subheadline) .foregroundColor(.gray) } .padding() .background(Color.white) .cornerRadius(12) .shadow(radius: 4) } }
In this view, we ensure that the temperature and condition text are the focal points, creating a clean and engaging experience. The use of padding, background color, and corner radius enhances the visual hierarchy, making it more inviting to the user.
Consistency: Maintaining a consistent design language across your widgets and the app itself is vital. This includes colors, typography, and iconography. Users should instantly recognize the widget as part of your app’s ecosystem. Consistent design fosters trust and enhances usability.
Adaptability: Given the various widget sizes and configurations, designing adaptable layouts is essential. Widgets should look good in both small and medium sizes, requiring careful layout decisions. SwiftUI’s flexible layout system allows developers to use techniques such as stacks and spacers to achieve a responsive design.
struct AdaptiveWidgetEntryView: View { var entry: AdaptiveEntry var body: some View { Group { if entry.size == .small { Text(entry.shortInfo) } else { VStack { Text(entry.title) .font(.headline) Text(entry.fullInfo) .font(.subheadline) } } } .padding() .background(Color.gray.opacity(0.1)) .cornerRadius(10) } }
This example demonstrates a widget that adapts its content based on its size, ensuring that users get a tailored experience regardless of the widget’s dimensions.
User Engagement: Design principles should also aim to improve user engagement. Think integrating compelling visuals, animations, or subtle interactions, such as tapping on the widget to open the app. Widgets should invite users to take action while still delivering information at a glance. Using SwiftUI’s gesture recognizers can facilitate interactions smoothly.
struct InteractiveWidgetEntryView: View { var entry: InteractiveEntry var body: some View { VStack { Text(entry.title) .font(.title) .onTapGesture { // Action to open the app when the widget is tapped openApp() } } .padding() .background(Color.green.opacity(0.1)) .cornerRadius(12) } func openApp() { // Function to open the app } }
In this code snippet, the widget responds to user taps by executing a function, thus creating a bridge between the widget and the main app experience.
Accessibility: Lastly, designing for accessibility is imperative. Ensure that text and interactive elements are easy to read and interact with for all users. Use appropriate color contrasts and think screen reader support. WidgetKit provides accessibility features that can enhance the experience for users with disabilities, making your widget inclusive.
By adhering to these design principles, developers can create widgets that not only look good but also provide users with valuable, engaging information. The balance between aesthetics and functionality is key, and with thoughtful consideration, your widgets can significantly enhance user interaction with your applications.
Interactivity and User Engagement
Interactivity in widgets is paramount for enhancing user engagement. While widgets are primarily designed for glanceable information, incorporating interactive elements can significantly enrich the user experience. Users should feel invited to explore more content or features when they interact with a widget, making it a portal to richer app functionality.
One of the simplest ways to introduce interactivity is through tap gestures. By responding to user taps, developers can create actions that transition users from the widget to the main application or trigger specific functions within the widget itself. For instance, let’s think a weather widget that allows users to tap on it for more detailed weather information:
struct WeatherWidgetEntryView: View { var entry: WeatherEntry var body: some View { VStack { Text(entry.temperature) .font(.largeTitle) .fontWeight(.bold) Text(entry.condition) .font(.subheadline) .foregroundColor(.gray) } .padding() .background(Color.white) .cornerRadius(12) .shadow(radius: 4) .onTapGesture { openWeatherApp() } } func openWeatherApp() { // Code to open the weather app } }
In this example, the `onTapGesture` modifier allows the widget to respond to user interactions. By implementing an `openWeatherApp` function, developers can specify the action taken when the widget is tapped, such as launching the app with detailed weather information.
Another effective way to engage users is by providing dynamic content updates. Widgets can respond to changes in data and represent them interactively. For instance, a news widget could display the latest headlines and allow users to tap on a headline to read the full article in the app:
struct NewsWidgetEntryView: View { var entry: NewsEntry var body: some View { VStack { ForEach(entry.headlines, id: .self) { headline in Text(headline) .font(.headline) .onTapGesture { openArticle(headline) } } } .padding() .background(Color.yellow.opacity(0.1)) .cornerRadius(12) } func openArticle(_ headline: String) { // Code to open the article in the app } }
In this snippet, the widget displays multiple headlines, and each headline can be tapped to trigger a function that opens the corresponding article. This interactive feature not only provides value to users but also encourages them to engage further with the app.
Feedback is also essential in interactive design. Visual feedback, such as changing the color or scale of a widget element upon interaction, can confirm that the user’s action has been recognized. SwiftUI’s animation capabilities can be employed to create smooth transitions and feedback animations that enhance overall user satisfaction:
struct InteractiveFeedbackWidgetView: View { @State private var isTapped = false var body: some View { Text("Tap me!") .font(.title) .padding() .background(isTapped ? Color.blue : Color.gray) .cornerRadius(12) .scaleEffect(isTapped ? 1.1 : 1.0) .onTapGesture { withAnimation { isTapped.toggle() } performAction() } } func performAction() { // Code to perform the desired action } }
This example employs a scaling effect and color change to provide immediate visual feedback when the widget is tapped. Such feedback mechanisms are crucial in making the user interface feel responsive and alive.
Furthermore, it’s important to think the broader context in which users interact with widgets. Integration with system features like notifications or the ability to reflect real-time updates enhances the interactivity of widgets. For example, a fitness widget could update its display based on activity tracking, motivating users to achieve their fitness goals:
struct FitnessWidgetEntryView: View { var entry: FitnessEntry var body: some View { VStack { Text("Steps: (entry.steps)") .font(.headline) .onTapGesture { openFitnessApp() } } .padding() .background(Color.green.opacity(0.1)) .cornerRadius(12) } func openFitnessApp() { // Code to open the fitness app } }
By integrating real-time data with engaging interactions, you can significantly enhance the user experience, encouraging users to return to the widget frequently. This cycle of interaction not only enriches user engagement but also solidifies the role of your widget as an indispensable part of the user’s daily routine.
Best Practices for Widget Performance
Performance optimization is a key aspect of developing widgets using WidgetKit. Given the limited resources available on devices, maintaining high performance while delivering a rich user experience is essential. This means developers need to be mindful of various factors that influence performance, such as data fetching strategies, memory management, and efficient rendering.
One of the primary considerations is how often your widget updates. Widgets should refresh only when necessary to avoid unnecessary resource consumption. When defining a timeline, choose update intervals judiciously. For instance, if your widget displays static content that doesn’t change frequently, consider a longer update frequency. Here is an adjusted timeline provider that updates every hour:
struct HourlyTimelineProvider: TimelineProvider { func placeholder(in context: Context) -> QuoteEntry { QuoteEntry(date: Date(), quote: "Temporary Placeholder Quote") } func getSnapshot(in context: Context, completion: @escaping (QuoteEntry) -> ()) { let entry = QuoteEntry(date: Date(), quote: "Snapshot Quote") completion(entry) } func getTimeline(in context: Context, completion: @escaping (Timeline) -> ()) { var entries: [QuoteEntry] = [] let currentDate = Date() // Create an entry for every hour for today for hourOffset in 0 .. String { // Method to fetch a quote from a data source return "Your hourly motivation goes here!" } }
This example demonstrates a timeline provider that generates entries for each hour, refreshing the widget with new content while minimizing unnecessary updates.
Memory usage is another critical aspect of performance. Every widget runs in a separate process, and any resource hog can lead to performance issues or even crashes. SwiftUI’s view system is optimized but can still create performance bottlenecks if views are overly complex. Strive for simplicity in your view structure. If certain parts of the UI don’t change often, think creating static views instead of dynamic ones to conserve memory.
struct SimplisticView: View { var entry: QuoteEntry var body: some View { VStack { Text(entry.quote) .font(.headline) .padding() // Separate static view for other content StaticContentView() } .background(Color.white) .cornerRadius(12) .shadow(radius: 4) } } struct StaticContentView: View { var body: some View { Text("Static content that doesn't change often") .font(.subheadline) .foregroundColor(.gray) } }
By extracting static content into its own view, we reduce the need to recalculate and re-render parts of the widget unnecessarily. This modularization keeps the widget responsive and reduces the likelihood of performance degradation.
Another important factor is the use of asynchronous data loading. Fetching data synchronously can block the main thread, leading to a poor user experience. Instead, utilize asynchronous calls to fetch data in the background, ensuring your widget remains responsive. Here’s how you could implement async fetching of data:
func fetchDataAsync(completion: @escaping (String) -> Void) { DispatchQueue.global(qos: .background).async { // Simulate network delay sleep(2) let quote = "Fetched quote from an API" completion(quote) } } func getTimeline(in context: Context, completion: @escaping (Timeline) -> ()) { fetchDataAsync { fetchedQuote in let entry = QuoteEntry(date: Date(), quote: fetchedQuote) let timeline = Timeline(entries: [entry], policy: .after(Date().addingTimeInterval(3600))) completion(timeline) } }
This approach ensures that your data fetching is done off the main thread, thereby maintaining a smooth user interface while the widget operates in the background.
Finally, always test your widgets on actual devices rather than the simulator, as performance can vary significantly between the two. Use Instruments and profiling tools to track memory usage and CPU consumption, enabling you to identify and rectify performance issues before your widget reaches users.
By following these best practices, you can create widgets that are not only engaging and useful but also performant, ensuring they enhance the overall user experience without frustrating delays or excessive resource consumption.
Future Trends in Widget Development with Swift
The future of widget development with Swift and WidgetKit is poised to evolve dramatically as user needs and technological capabilities progress. As developers become increasingly familiar with the WidgetKit framework, they are likely to explore deeper integrations and enhanced functionalities that make widgets not just static information displays, but dynamic, interactive experiences.
One area of focus will be on improving interactivity. Future widgets could leverage more sophisticated gesture recognizers, allowing for a wider range of interactions without overwhelming the user. For instance, imagine a calendar widget where users can swipe between different views (like daily or weekly) or tap to add new events directly from the widget itself. These enhancements could transform widgets into essential tools rather than mere placeholders for app data.
struct InteractiveCalendarWidgetView: View { var entry: CalendarEntry var body: some View { VStack { Text(entry.date, style: .date) .font(.headline) .onTapGesture { openCalendarApp() } // Swipe gesture to navigate between views } .padding() .background(Color.white) .cornerRadius(12) } func openCalendarApp() { // Code to launch the calendar app } }
Moreover, the integration of real-time data feed functionality into widgets will likely become more prevalent. As user expectations for up-to-date information rise, widgets will need to support live data streams. This could involve using background refresh capabilities more effectively, allowing widgets to present the latest news, social media updates, or even live sports scores without requiring the user to open the app. The challenge here will be to balance the frequency of updates with performance and power consumption concerns.
func getLiveDataTimeline(in context: Context, completion: @escaping (Timeline) -> ()) { fetchLiveData { liveData in let entry = LiveDataEntry(date: Date(), data: liveData) let timeline = Timeline(entries: [entry], policy: .after(Date().addingTimeInterval(60))) // Update every minute completion(timeline) } }
The expansion of customization options is another promising trend. Developers may provide users with more control over how their widgets appear and function. This could include customizable layouts, themes, and content selection, creating a more personalized user experience. Users could choose which data points they want visible on their home screens, fostering a sense of ownership and engagement with the widget.
Furthermore, as augmented reality (AR) technology becomes more accessible, we might see widgets that provide AR capabilities. Imagine a fitness widget that not only tracks your steps but also overlays your daily goals and achievements in your physical space through AR. This would create a compelling visual experience that ties the digital and physical worlds together, enhancing user motivation and interaction.
struct ARFitnessWidgetView: View { var entry: FitnessEntry var body: some View { VStack { // AR overlay for fitness goals Text("Steps: (entry.steps)") .font(.headline) .onTapGesture { openFitnessApp() } } .padding() .background(Color.green.opacity(0.1)) .cornerRadius(12) } func openFitnessApp() { // Code to open the fitness app } }
Lastly, as privacy and user data protection continue to be paramount, future widget development will need to incorporate robust security measures. This could involve using more secure data transmission methods for widgets that pull in sensitive information and ensuring compliance with privacy regulations. Transparency in how user data is used and stored will be crucial in building trust between the widget and its users.
The future of widget development with Swift is set to embrace interactivity, real-time data integration, user customization, augmented reality, and enhanced privacy measures. As developers harness the full potential of WidgetKit, they will redefine what users can expect from widgets, evolving them into indispensable components of the digital experience.