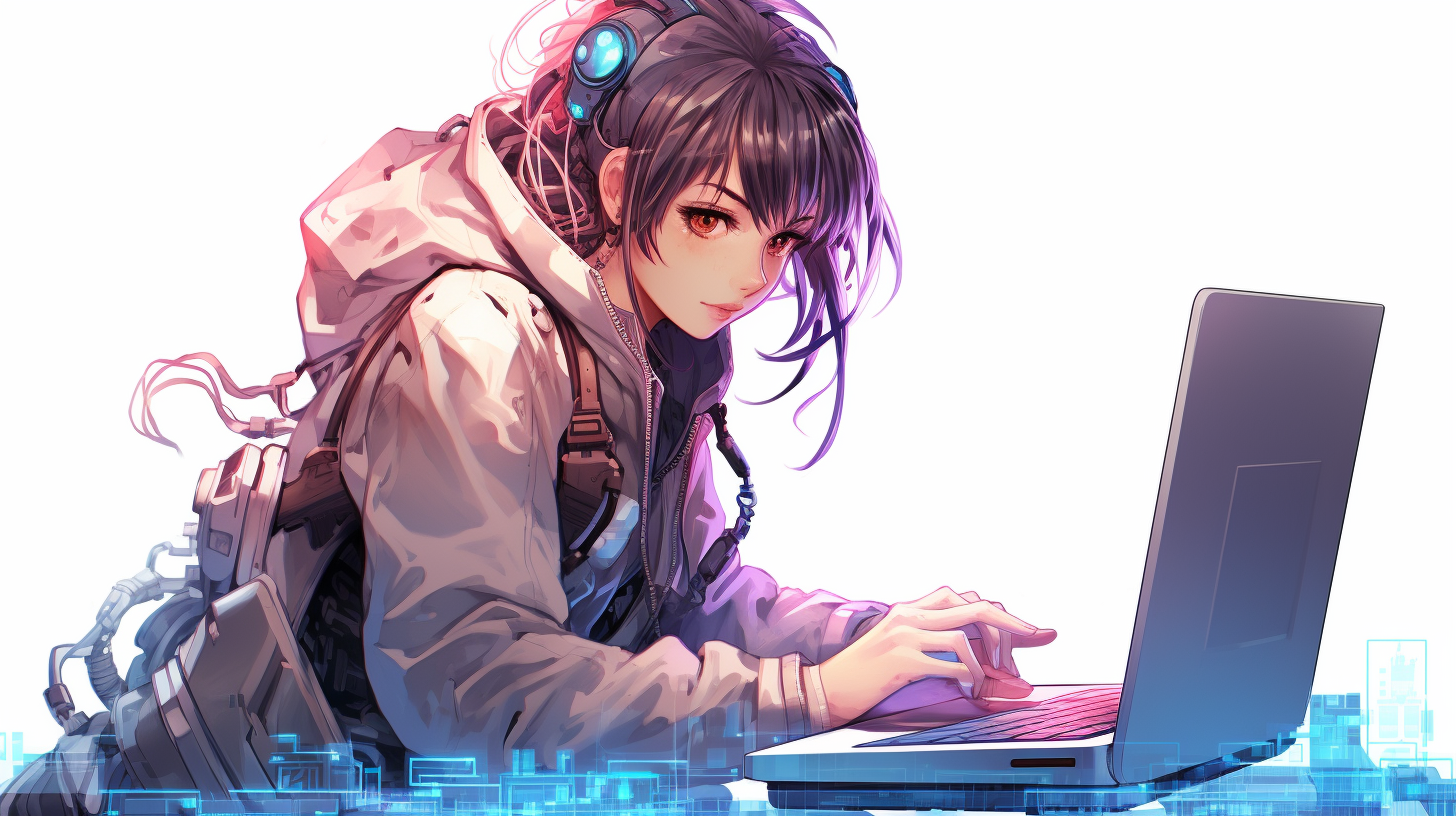
Java and Virtual Reality: Getting Started
Virtual reality (VR) is a technology that simulates a user’s physical presence in a virtual environment, allowing for immersive experiences that can range from gaming to training simulations. At its core, VR engages multiple senses, primarily sight and sound, to create an experience that feels real to the user. Understanding the fundamental concepts behind VR is essential for any Java developer looking to explore this exciting domain.
A key component of VR is the idea of a virtual environment. This environment is a computer-generated space where users can interact with various elements as if they were in a physical world. The immersion is achieved through the use of head-mounted displays (HMDs), gloves, and other hardware that tracks user movements and responds accordingly.
Another important concept is 3D rendering. In VR, rendering involves creating graphical objects that populate the virtual world. These objects must be rendered in three dimensions to provide depth perception, which is vital for making the experience feel real. Java offers libraries such as Java 3D and LWJGL (Lightweight Java Game Library) that facilitate 3D rendering.
Moreover, user interaction plays a significant role in VR. The user’s movements, gestures, and even voice commands can be captured and interpreted by the system to create a seamless interaction with the virtual environment. Understanding how to translate real-world actions into virtual actions is important for developers. This often involves using APIs that can handle input devices such as motion controllers.
Another concept to grasp is tracking technology. This technology is responsible for monitoring the user’s position and orientation in real-time. It can include various methods, from external sensors to internal systems in the HMD. Accurate tracking ensures that as a user moves their head or body, the virtual environment responds dynamically, enhancing the immersive experience.
To get a feel for how Java can interact with VR concepts, ponder the following simple code snippet that initializes a 3D scene using Java 3D:
import javax.media.j3d.*; import javax.vecmath.*; public class Simple3DScene { public static void main(String[] args) { // Create a simple scene SimpleUniverse universe = new SimpleUniverse(); BranchGroup group = new BranchGroup(); // Create a sphere Sphere sphere = new Sphere(0.5f); group.addChild(sphere); // Add the group to the universe universe.addBranchGraph(group); universe.getViewingPlatform().setNominalViewingTransform(); } }
This code snippet demonstrates the creation of a simple 3D scene with a sphere. The `SimpleUniverse` class sets up the rendering environment, while the `BranchGroup` holds the objects that will be displayed. The `Sphere` object represents a simple 3D shape, which can be a starting point for more complex designs.
Setting Up a Java Development Environment for VR
Setting up a Java development environment for virtual reality (VR) is an important step in using the potential of this immersive technology. The appropriate tools and libraries will enable you to create engaging VR applications. Here’s how to establish that environment.
First, ensure you have the latest version of the Java Development Kit (JDK) installed on your system. The JDK is essential, as it includes the Java Runtime Environment (JRE) and the development tools necessary for compiling and running your applications. You can download the JDK from the official Oracle website or adopt an open-source version like OpenJDK.
After installing the JDK, you should choose an Integrated Development Environment (IDE) that suits your workflow. Popular choices for Java development include:
- Known for its intelligent code assistance and ergonomic design, it greatly enhances productivity.
- An open-source IDE that supports various languages and is particularly effortless to handle for beginners.
- A highly extensible IDE, perfect for larger projects, with a plethora of plugins available.
Once you’ve installed your chosen IDE, configure it to recognize the JDK. This process typically involves setting the JDK path within the IDE preferences. After that, you are ready to start building your VR applications.
Next, integrating VR-specific libraries is essential. Libraries such as Java 3D, LWJGL (Lightweight Java Game Library), and jMonkeyEngine are particularly useful for developing VR content. Java 3D provides a robust framework for 3D rendering, while LWJGL offers low-level access to graphics hardware, making it a favored choice for performance-intensive applications.
To demonstrate how to set up a simple project using LWJGL, ponder the following steps:
// Sample code to initialize an LWJGL window import org.lwjgl.*; import org.lwjgl.glfw.*; import org.lwjgl.opengl.*; public class SimpleLWJGL { public static void main(String[] args) { // Initialize GLFW if (!glfwInit()) { throw new IllegalStateException("Unable to initialize GLFW"); } // Create a windowed mode window and its OpenGL context long window = glfwCreateWindow(800, 600, "Hello LWJGL", 0, 0); if (window == 0) { throw new RuntimeException("Failed to create the GLFW window"); } // Make the window's context current glfwMakeContextCurrent(window); glfwShowWindow(window); // Initialize OpenGL GL.createCapabilities(); // Main loop while (!glfwWindowShouldClose(window)) { glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); // Swap buffers glfwSwapBuffers(window); glfwPollEvents(); } glfwDestroyWindow(window); glfwTerminate(); } }
This code snippet sets up a basic LWJGL application that creates a window and initializes an OpenGL context. It represents the foundation upon which you can build more complex VR scenes and interactions.
Lastly, don’t forget to explore the VR hardware that you plan to use. Popular head-mounted displays (HMDs) like the Oculus Rift, HTC Vive, and Valve Index often come with their SDKs. These SDKs provide additional tools and libraries that facilitate interaction with the physical devices, allowing you to track user movements and gestures seamlessly.
Exploring Java VR Libraries and Frameworks
As you progress in your journey of exploring Java VR libraries and frameworks, it’s crucial to understand the landscape of tools available to you. Java has several libraries that cater specifically to the needs of VR development, each bringing unique features and capabilities to the table. Here, we will delve into some of the most notable libraries that can elevate your VR applications, making your experiences not only immersive but also engaging.
One of the first libraries to think is Java 3D. This high-level API simplifies the task of creating 3D graphics in Java. Although it is been around for a while, Java 3D remains an excellent choice for developers looking to create interactive 3D environments. It provides built-in support for rendering geometry, applying textures, and handling user inputs. The library is also designed to work seamlessly with Java’s rendering pipeline, enabling you to incorporate hardware acceleration as needed.
Here’s a simple example illustrating how you might use Java 3D to create a spinning cube:
import javax.media.j3d.*; import javax.vecmath.*; import com.sun.j3d.utils.universe.*; public class SpinningCube { public static void main(String[] args) { SimpleUniverse universe = new SimpleUniverse(); BranchGroup group = new BranchGroup(); // Create a cube ColorCube cube = new ColorCube(0.3); group.addChild(cube); // Set up the rotation TransformGroup rotation = new TransformGroup(); rotation.setCapability(TransformGroup.ALLOW_TRANSFORM_WRITE); rotation.addChild(cube); group.addChild(rotation); // Add rotation behavior new RotationBehavior(rotation).setSchedulingBounds(new BoundingSphere(new Point3d(0.0, 0.0, 0.0), 100.0)); universe.addBranchGraph(group); universe.getViewingPlatform().setNominalViewingTransform(); } }
In the example above, the cube rotates continuously, demonstrating basic interactivity within a 3D space.
Moving on, we have the Lightweight Java Game Library (LWJGL). This library is tailored for high-performance gaming and graphical applications. LWJGL provides bindings to OpenGL, OpenAL, and OpenCL, allowing developers to harness advanced rendering techniques, sound management, and compute capabilities. If your VR application demands a sophisticated graphics pipeline, LWJGL might be the perfect fit.
For instance, initializing a simple window using LWJGL can be achieved with the following code:
import org.lwjgl.*; import org.lwjgl.glfw.*; import org.lwjgl.opengl.*; public class LWJGLWindow { public static void main(String[] args) { // Initialize GLFW if (!glfwInit()) { throw new IllegalStateException("Unable to initialize GLFW"); } // Create a window long window = glfwCreateWindow(800, 600, "LWJGL VR", 0, 0); if (window == 0) { throw new RuntimeException("Failed to create the GLFW window"); } glfwMakeContextCurrent(window); glfwShowWindow(window); GL.createCapabilities(); // Main loop while (!glfwWindowShouldClose(window)) { glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); glfwSwapBuffers(window); glfwPollEvents(); } glfwDestroyWindow(window); glfwTerminate(); } }
This code sets up a basic window where you can start integrating your VR elements.
Lastly, we cannot overlook jMonkeyEngine, a powerful game engine that supports both 2D and 3D graphics. jMonkeyEngine is particularly suited for VR development due to its extensive feature set, including physics, networking, and terrain management. The engine is built on top of Java and allows for rapid prototyping and development.
To give you a glimpse into jMonkeyEngine, here’s a simple code snippet for creating a basic scene:
import com.jme3.app.SimpleApplication; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.material.Material; public class BasicScene extends SimpleApplication { public static void main(String[] args) { BasicScene app = new BasicScene(); app.start(); } @Override public void simpleInitApp() { Box b = new Box(1, 1, 1); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); geom.setMaterial(mat); rootNode.attachChild(geom); } }
With jMonkeyEngine, creating 3D objects and applying materials becomes a simpler task, giving you the freedom to focus more on the creative aspects of your VR application.
Building Your First Java Virtual Reality Application
import com.jme3.app.SimpleApplication; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; public class BasicScene extends SimpleApplication { public static void main(String[] args) { BasicScene app = new BasicScene(); app.start(); } @Override public void simpleInitApp() { Box b = new Box(1, 1, 1); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); geom.setMaterial(mat); rootNode.attachChild(geom); } }
Building your first Java virtual reality application is akin to laying the foundation of a grand structure; it requires a careful selection of tools and a clear plan. Let’s dive into creating a simple yet interactive VR experience using the jMonkeyEngine, known for its ease of use and powerful capabilities.
First, ensure you have jMonkeyEngine installed. You can download it from the jMonkeyEngine website, where you will find an SDK that simplifies the development process. The SDK includes everything you need to start building, from project templates to integrated support for assets.
Once your environment is set up, let’s create a basic scene. The essence of VR lies in interaction and immersion, so we’ll start by placing a simple 3D object in a scene and making it respond to user input. Our initial example will create a colored cube that can rotate, establishing a visual focal point in the environment.
The following code snippet demonstrates how to set up the scene and add a basic cube:
import com.jme3.app.SimpleApplication; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.input.InputManager; import com.jme3.input.controls.ActionListener; public class InteractiveCube extends SimpleApplication implements ActionListener { private Geometry cube; public static void main(String[] args) { InteractiveCube app = new InteractiveCube(); app.start(); } @Override public void simpleInitApp() { Box box = new Box(1, 1, 1); cube = new Geometry("Cube", box); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); cube.setMaterial(mat); rootNode.attachChild(cube); // Configure input inputManager.addMapping("Rotate", new KeyTrigger(KeyInput.KEY_SPACE)); inputManager.addListener(this, "Rotate"); } @Override public void onAction(String name, boolean isPressed, float tpf) { if (name.equals("Rotate") && isPressed) { cube.rotate(0, 0.5f, 0); } } }
In this example, the `InteractiveCube` class extends `SimpleApplication`, which is jMonkeyEngine’s base class for applications. In the `simpleInitApp()` method, we create a cube and apply a blue material to it. We also set up an input mapping that listens for the space key. When pressed, it triggers a rotation on the cube, allowing users to interact with it dynamically.
This foundational example sets the stage for more complex interactions. From here, you can expand the application by adding more geometric shapes, introducing textures, or even integrating sound effects to improve immersion. Each additional element builds upon the immersive quality of the VR experience, drawing users deeper into the virtual world you’ve created.
As you grow more comfortable with jMonkeyEngine, consider exploring more sophisticated features such as physics engines, particle systems, and lighting effects. These components will elevate your application, making the virtual environment not just a backdrop, but a fully-fledged world with depth and interactivity.