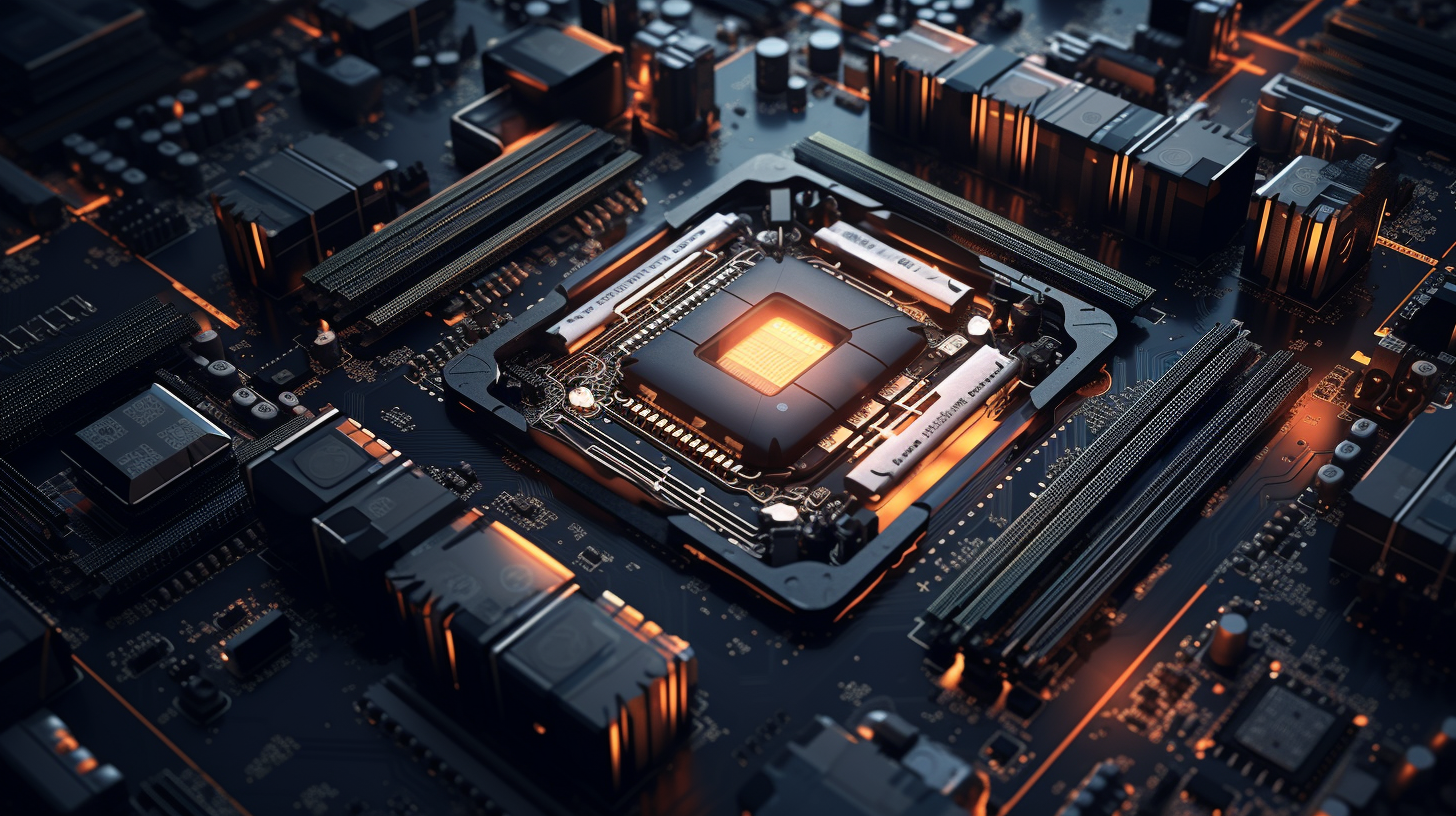
Bash Scripting for File Encryption
File encryption is an important technique for securing sensitive data, transforming readable information into an unreadable format that can only be reverted to its original form with a specific key. Understanding the various methods of file encryption can significantly enhance your ability to protect your information effectively.
There are two primary types of encryption methods: symmetrical and asymmetrical encryption.
Symmetrical encryption uses a single key for both the encryption and decryption processes. This means that the same key must be kept secret and securely shared between the parties involved. A common algorithm that utilizes symmetrical encryption is AES
(Advanced Encryption Standard), known for its speed and efficiency.
# Example of using AES for encryption and decryption openssl enc -aes-256-cbc -salt -in secret.txt -out secret.txt.enc -k YOURPASSWORD openssl enc -d -aes-256-cbc -in secret.txt.enc -out secret.txt -k YOURPASSWORD
On the other hand, asymmetrical encryption employs a pair of keys: a public key for encryption and a private key for decryption. This method allows for safer key exchange since the private key never needs to be shared. RSA
is a well-known algorithm that utilizes asymmetrical encryption.
# Example of RSA key generation and usage openssl genrsa -out private.pem 2048 openssl rsa -in private.pem -outform PEM -pubout -out public.pem openssl rsautl -encrypt -inkey public.pem -pubin -in secret.txt -out secret.txt.enc openssl rsautl -decrypt -inkey private.pem -in secret.txt.enc -out secret.txt
In practice, many encryption workflows often utilize a combination of both methods. A typical approach involves first generating a random symmetric key for encrypting the file itself, which is then encrypted using an asymmetrical public key. This combines the efficiency of symmetrical encryption with the security of asymmetrical encryption, ensuring both secure key exchange and rapid data processing.
Setting Up Your Bash Environment for Encryption
To effectively set up your Bash environment for file encryption, you need to ensure that you have the right tools and dependencies installed. A common utility for encryption in the Bash environment is OpenSSL, which provides robust support for both symmetric and asymmetric encryption algorithms. Before diving into scripting, let’s go through the necessary steps to prepare your environment.
First, you need to verify if OpenSSL is installed on your system. You can check this by running the following command in your terminal:
openssl version
If OpenSSL is not installed, you can install it using the package manager specific to your operating system. For instance, on a Debian-based system like Ubuntu, you can use:
sudo apt-get update sudo apt-get install openssl
For Red Hat-based systems, you can use:
sudo yum install openssl
Once OpenSSL is installed, you should also think setting up a dedicated directory for your encryption scripts and related files. This keeps your workspace organized and ensures that you can easily locate your scripts and keys. You can create a directory named “encryption_scripts” in your home folder as follows:
mkdir ~/encryption_scripts
Next, it’s a good practice to generate a strong password for symmetric encryption. You can do this using OpenSSL to create a random password of a specified length. Here’s how you can generate a 32-byte password:
openssl rand -base64 32
After you’ve generated a password, it’s wise to store it securely. You can create a file to keep your passwords, but ensure that it’s protected. For creating a password file, you might use:
echo "YOUR_GENERATED_PASSWORD" > ~/encryption_scripts/password.txt
Remember to set appropriate permissions on this file to restrict access. You can change the file permissions using:
chmod 600 ~/encryption_scripts/password.txt
In addition to OpenSSL, ensure you have the necessary Bash utilities available. Most systems should come pre-installed with basic utilities like `cat`, `echo`, and `read`. However, if you intend to use more advanced features such as process substitution or arrays, ensure your Bash version supports them. You can check your Bash version with:
bash --version
Creating a Simple Bash Script for File Encryption
Now that your Bash environment is properly set up for file encryption, it’s time to create a simple Bash script that can encrypt and decrypt files securely. This script will serve as the backbone for your encryption tasks and can be easily modified or expanded based on your needs. Below, I will guide you through the creation of a simpler Bash script that utilizes OpenSSL for file encryption using AES.
First, create a new script file in your dedicated scripts directory:
touch ~/encryption_scripts/encrypt_decrypt.sh
Next, open the script file in your preferred text editor. For example, using nano:
nano ~/encryption_scripts/encrypt_decrypt.sh
Now, let’s start writing the script. The script will accept parameters for the operation (encrypt or decrypt), the input file, the output file, and the password. Here’s how you can structure the script:
#!/bin/bash # Check if the correct number of arguments is provided if [ "$#" -ne 4 ]; then echo "Usage: $0 [encrypt|decrypt] input_file output_file password" exit 1 fi # Assign arguments to variables operation=$1 input_file=$2 output_file=$3 password=$4 # Function for encryption encrypt_file() { openssl enc -aes-256-cbc -salt -in "$input_file" -out "$output_file" -k "$password" echo "File encrypted: $output_file" } # Function for decryption decrypt_file() { openssl enc -d -aes-256-cbc -in "$input_file" -out "$output_file" -k "$password" echo "File decrypted: $output_file" } # Check the operation type and call the corresponding function if [ "$operation" == "encrypt" ]; then encrypt_file elif [ "$operation" == "decrypt" ]; then decrypt_file else echo "Invalid operation: $operation" exit 1 fi
In this script, we first check if the correct number of arguments has been given. If not, it prompts the user with the correct usage. The script then assigns the parameters to variables for better readability.
The `encrypt_file` function handles the encryption process using the `openssl` command with AES-256-CBC. It takes the input file, encrypts it, and outputs it to the specified output file. Similarly, the `decrypt_file` function performs the decryption operation.
Now, save the file and exit your text editor. To make the script executable, run the following command:
chmod +x ~/encryption_scripts/encrypt_decrypt.sh
With your script ready, you can now use it to encrypt and decrypt files. Here’s how you can execute the script:
# To encrypt a file ~/encryption_scripts/encrypt_decrypt.sh encrypt secret.txt secret.txt.enc YOURPASSWORD # To decrypt a file ~/encryption_scripts/encrypt_decrypt.sh decrypt secret.txt.enc decrypted.txt YOURPASSWORD
Make sure to replace `YOURPASSWORD` with the actual password you generated previously. This simple yet effective script allows you to manage file encryption and decryption directly from the command line, integrating seamlessly into your workflow.
Decrypting Files with Bash: A Step-by-Step Guide
When it comes to decrypting files using Bash, the process is closely aligned with encryption, but it requires a proper understanding of the keys and methods used during the encryption phase. In this guide, we will walk through the steps necessary to decrypt files securely and efficiently using a Bash script.
To begin with, you’ll want to ensure that you have the encrypted file, the original password used for encryption, and a designated output file name where the decrypted content will be saved. Since we are using AES encryption for our example, the decryption command will mirror the encryption command, with slight modifications.
Let’s modify our previous script to ensure that it handles both encryption and decryption seamlessly through command-line arguments. Here’s a focused look at the decryption aspect:
#!/bin/bash # Check if the correct number of arguments is provided if [ "$#" -ne 4 ]; then echo "Usage: $0 [encrypt|decrypt] input_file output_file password" exit 1 fi # Assign arguments to variables operation=$1 input_file=$2 output_file=$3 password=$4 # Function for decryption decrypt_file() { if [ ! -f "$input_file" ]; then echo "Input file does not exist!" exit 1 fi openssl enc -d -aes-256-cbc -in "$input_file" -out "$output_file" -k "$password" if [ $? -eq 0 ]; then echo "File decrypted successfully: $output_file" else echo "Decryption failed. Please check your password or the input file." fi } # Check the operation type and call the corresponding function if [ "$operation" == "decrypt" ]; then decrypt_file else echo "Invalid operation: $operation" exit 1 fi
In this script, we first check to ensure that the input file exists before attempting decryption. This is an important step to avoid errors that can arise from referencing non-existent files. The decryption itself uses the `openssl enc -d` command, which specifies that we are decrypting the content. The output is directed to the specified output file, rendering it effortless to access the decrypted data.
After executing the decryption command, the script checks the exit status of the `openssl` command. If the exit status is `0`, it means the decryption was successful, and a confirmation message is displayed. If not, an error message is printed, providing feedback on potential issues such as incorrect password or an unreadable input file.
To execute the decryption process, use the following command:
~/encryption_scripts/encrypt_decrypt.sh decrypt secret.txt.enc decrypted.txt YOURPASSWORD
As you can see, by simply changing the operation to “decrypt,” you can seamlessly convert your encrypted data back into a readable format. This flexibility highlights the power of Bash scripting, as it allows you to handle both encryption and decryption in a cohesive manner with minimal effort.