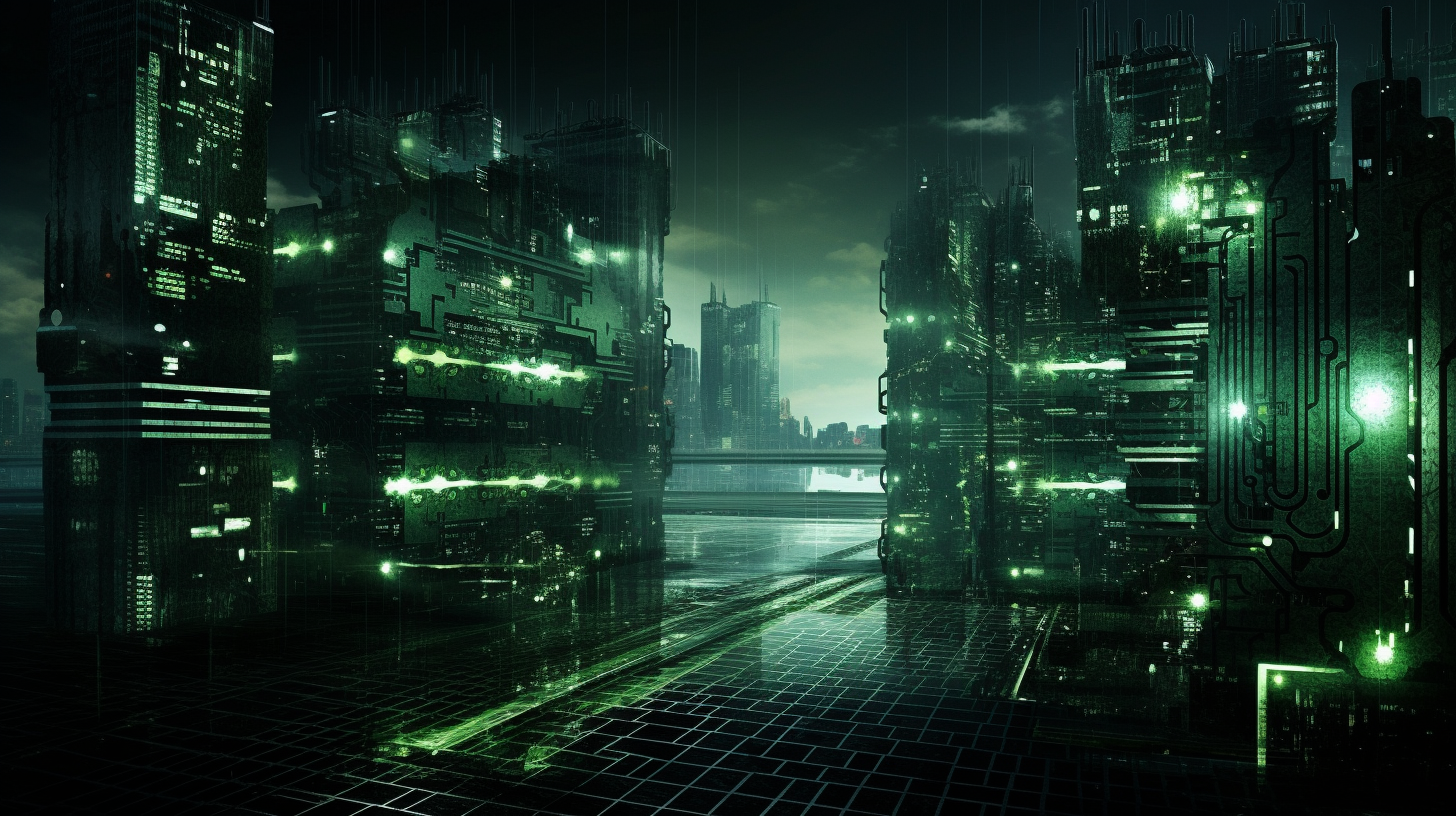
SwiftUI Advanced Techniques
Creating engaging user interfaces in SwiftUI often requires the addition of custom animations that can breathe life into your applications. Achieving these animations involves understanding the fundamental animation capabilities provided by SwiftUI and how to tailor them to suit specific needs.
In SwiftUI, animations can be easily applied to any view by using the withAnimation
function. This function triggers a transition and allows for smooth changes between states. It is essential to define what exactly changes when the animation is triggered, typically through state variables.
To illustrate this, ponder a simple example where a circle expands in size when tapped. Here’s how to implement that:
struct AnimatedCircle: View { @State private var isExpanded = false var body: some View { Circle() .fill(Color.blue) .frame(width: isExpanded ? 200 : 100, height: isExpanded ? 200 : 100) .onTapGesture { withAnimation(.easeInOut(duration: 0.5)) { isExpanded.toggle() } } } }
In this example, we define a state variable isExpanded
that tracks whether the circle is in its expanded state. The frame
modifier adjusts the size based on this state. By wrapping the state change in withAnimation
, we provide a smooth transition that utilizes the easeInOut
timing curve, making the change feel more natural.
Custom animations can also be defined using the Animation struct. You can create more complex animations with custom duration and timing curves, or even chain multiple animations together. For instance, if you want to add a rotation effect alongside the size change, you can modify the code as follows:
struct AnimatedCircleWithRotation: View { @State private var isExpanded = false var body: some View { Circle() .fill(Color.blue) .frame(width: isExpanded ? 200 : 100, height: isExpanded ? 200 : 100) .rotationEffect(.degrees(isExpanded ? 360 : 0)) .onTapGesture { withAnimation(.easeInOut(duration: 0.5)) { isExpanded.toggle() } } } }
In this version, we introduce the rotationEffect
modifier, which applies a rotation based on the current state. The result is a visually appealing animation that combines both scaling and rotation, enhancing user interaction.
Another powerful feature of SwiftUI is the ability to create reusable custom animations. You can encapsulate animation logic within a custom modifier to apply it wherever needed. Here’s how you could create a custom animation modifier:
struct CustomAnimationModifier: AnimatableModifier { var scale: CGFloat func body(content: Content) -> some View { content .scaleEffect(scale) .animation(.easeInOut(duration: 0.5), value: scale) } } extension View { func customAnimation(scale: CGFloat) -> some View { self.modifier(CustomAnimationModifier(scale: scale)) } }
Now, you can use this modifier in any view, allowing for a consistent and reusable animation style throughout your app. Here’s how you might apply it:
struct ContentView: View { @State private var isExpanded = false var body: some View { Circle() .fill(Color.blue) .frame(width: 100, height: 100) .customAnimation(scale: isExpanded ? 2.0 : 1.0) .onTapGesture { isExpanded.toggle() } } }
By employing these techniques, you can create visually compelling and interactive experiences in your SwiftUI applications. Mastery of custom animations not only enhances the aesthetic of your app but also improves user engagement significantly.
Using Property Wrappers for State Management
In SwiftUI, property wrappers play a pivotal role in state management, enabling developers to efficiently manage and respond to changes in data within their user interface. Understanding how to leverage these property wrappers can significantly enhance the responsiveness and maintainability of your SwiftUI applications.
The most commonly used property wrappers include @State, @Binding, @ObservedObject, and @EnvironmentObject. Each serves a unique purpose in managing data flow and state across your views.
The @State property wrapper is perfect for managing local state within a view. It allows SwiftUI to automatically update the user interface when the state changes. Here’s an example illustrating its usage:
struct CounterView: View { @State private var count = 0 var body: some View { VStack { Text("Count: (count)") .font(.largeTitle) Button(action: { count += 1 }) { Text("Increment") .padding() .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) } } } }
In this example, the count variable is declared with @State. Every time the button is tapped, count is incremented, and SwiftUI automatically re-renders the Text view to reflect the updated count.
When you need to pass data between views, @Binding is an invaluable tool. It creates a reference to a state variable that exists in a parent view, allowing child views to read and write to that state. Here’s an example:
struct ParentView: View { @State private var isToggled = false var body: some View { ToggleView(isToggled: $isToggled) } } struct ToggleView: View { @Binding var isToggled: Bool var body: some View { Toggle("Toggle Me", isOn: $isToggled) .padding() } }
In this setup, ToggleView can modify the isToggled state variable directly, thanks to the binding. This creates a clear data flow and reduces the complexity of managing state across views.
For more complex data models, @ObservedObject and @EnvironmentObject are used in conjunction with classes that conform to the ObservableObject protocol. This allows you to manage shared state effectively across multiple views.
Here’s a simple example using @ObservedObject:
class CounterModel: ObservableObject { @Published var count = 0 } struct ObservedCounterView: View { @ObservedObject var model = CounterModel() var body: some View { VStack { Text("Count: (model.count)") .font(.largeTitle) Button(action: { model.count += 1 }) { Text("Increment") .padding() .background(Color.green) .foregroundColor(.white) .cornerRadius(10) } } } }
In this example, the CounterModel class holds the count and publishes changes. The ObservedCounterView listens for changes in the model, updating the UI whenever count is modified.
Finally, for app-wide state management, @EnvironmentObject is used. This property wrapper allows you to inject an observable object into the SwiftUI environment, making it accessible across any view in the hierarchy without explicitly passing it down. Here’s how that looks:
class AppSettings: ObservableObject { @Published var isDarkMode = false } struct ContentView: View { @EnvironmentObject var settings: AppSettings var body: some View { VStack { Toggle("Dark Mode", isOn: $settings.isDarkMode) } } } @main struct MyApp: App { var body: some Scene { WindowGroup { ContentView() .environmentObject(AppSettings()) } } }
In this code, AppSettings is made available to the entire app. Any view that needs to access or modify the dark mode setting can do so without the need for cumbersome property passing. This approach enhances modularity and reusability across your SwiftUI application.
By effectively using property wrappers in SwiftUI, you can create highly responsive and maintainable applications capable of handling complex state scenarios with ease. Understanding these tools will empower you to build dynamic and intuitive interfaces that not only meet but exceed user expectations.
Building Complex Layouts with GeometryReader
When it comes to building complex layouts in SwiftUI, the GeometryReader is an invaluable tool that allows developers to tap into the size and position of views, providing a flexible framework for designing intricate interfaces. Unlike traditional layout systems that rely on fixed sizes and positions, GeometryReader empowers developers to create responsive designs that adapt to varying screen sizes and orientations.
The GeometryReader acts as a container that gives you access to the size and coordinate space of the parent view. This information can then be used to adjust the layout of child views dynamically, making it particularly useful for creating adaptive layouts, overlays, and positioning elements based on screen dimensions.
Here’s a fundamental example demonstrating how GeometryReader can be utilized to create a responsive layout:
struct GeometryReaderExample: View { var body: some View { GeometryReader { geometry in VStack { Text("Width: (geometry.size.width)") .padding() .frame(width: geometry.size.width * 0.5, height: 50) .background(Color.blue) .foregroundColor(.white) Text("Height: (geometry.size.height)") .padding() .frame(width: geometry.size.width * 0.5, height: 50) .background(Color.green) .foregroundColor(.white) } } .padding() } }
In this example, the GeometryReader provides the size of its parent view, which allows the child views to adjust their dimensions accordingly. The VStack contains two Text views that display the current width and height, and each is sized to 50% of the parent’s width. This ensures that the layout is adaptive, responding to changes in the overall size of the container.
Another powerful use case for GeometryReader is to create overlapping elements or complex responsive designs. By using the provided geometry, you can position views precisely. Here’s an example of a circle that overlaps a rectangle, with its size based on the parent view’s size:
struct OverlappingViews: View { var body: some View { GeometryReader { geometry in ZStack { Rectangle() .fill(Color.orange) .frame(width: geometry.size.width, height: geometry.size.height * 0.5) Circle() .fill(Color.blue) .frame(width: geometry.size.width * 0.5, height: geometry.size.width * 0.5) .offset(y: -geometry.size.height * 0.25) } } .edgesIgnoringSafeArea(.all) } }
In this layout, the Rectangle spans the full width and half the height of the parent view, while the Circle is centered and offset to create a visually interesting overlap. The use of ZStack allows for layering of views, rendering it effortless to create intricate designs that captures the user’s attention.
GeometryReader can also be particularly useful when combined with animations. By manipulating the size and position of views during transitions, you can create fluid, engaging interfaces. For instance, consider the following example where we animate the position of a circle within a GeometryReader:
struct AnimatedGeometryReader: View { @State private var offset: CGFloat = 0 var body: some View { GeometryReader { geometry in Circle() .fill(Color.red) .frame(width: 100, height: 100) .offset(x: offset, y: 0) .animation(.easeInOut(duration: 0.5), value: offset) .onTapGesture { offset = (offset == 0) ? geometry.size.width - 100 : 0 } } .edgesIgnoringSafeArea(.all) } }
In this code, the Circle moves horizontally across the screen when tapped, using the geometry to determine its final position. The animation provides a smooth transition, enhancing the user experience.
When employing GeometryReader, it’s crucial to be aware of its potential impact on performance, particularly if nested deeply within a view hierarchy. The view can introduce additional layout computations, so it’s best used judiciously. However, when applied thoughtfully, GeometryReader can elevate your SwiftUI projects with complex and responsive layouts that cater to a diverse range of devices and orientations.
Integrating SwiftUI with UIKit for Enhanced Functionality
Integrating SwiftUI with UIKit can significantly enhance the functionality of your applications, particularly when you need to leverage the strengths of both frameworks. While SwiftUI offers a declarative approach to building user interfaces, UIKit provides a mature, imperative framework with a wealth of pre-existing components and functionalities. By combining these two, developers can create rich, interactive applications that utilize the best features of each.
To use UIKit components within a SwiftUI view, you can take advantage of the UIViewControllerRepresentable
and UIViewRepresentable
protocols. These protocols allow you to wrap UIKit views and view controllers, making them available in your SwiftUI hierarchy.
Let’s start with a basic example of integrating a UIView
into SwiftUI. Suppose you want to use a simple UIKit UILabel
within your SwiftUI application:
struct UILabelWrapper: UIViewRepresentable { var text: String func makeUIView(context: Context) -> UILabel { let label = UILabel() label.textAlignment = .center label.font = UIFont.systemFont(ofSize: 24) return label } func updateUIView(_ uiView: UILabel, context: Context) { uiView.text = text } } struct ContentView: View { var body: some View { UILabelWrapper(text: "Hello from UIKit!") .padding() } }
In this example, we create a UILabelWrapper
struct that conforms to UIViewRepresentable
. The makeUIView
method initializes the UILabel, while the updateUIView
method updates its text whenever the SwiftUI state changes. This pattern allows seamless interaction between SwiftUI and UIKit.
An important feature of SwiftUI is its ability to manage state effectively. To create a more dynamic example, let’s integrate a UIKit UIButton
that updates a SwiftUI view:
struct ButtonWrapper: UIViewRepresentable { class Coordinator: NSObject { var parent: ButtonWrapper init(parent: ButtonWrapper) { self.parent = parent } @objc func buttonTapped() { parent.isTapped.toggle() } } @Binding var isTapped: Bool func makeCoordinator() -> Coordinator { Coordinator(parent: self) } func makeUIView(context: Context) -> UIButton { let button = UIButton(type: .system) button.setTitle("Tap me", for: .normal) button.addTarget(context.coordinator, action: #selector(Coordinator.buttonTapped), for: .touchUpInside) return button } func updateUIView(_ uiView: UIButton, context: Context) { uiView.setTitle(isTapped ? "Tapped!" : "Tap me", for: .normal) } } struct ContentView: View { @State private var isTapped = false var body: some View { VStack { ButtonWrapper(isTapped: $isTapped) .padding() Text(isTapped ? "Button was tapped!" : "Button not tapped yet") .font(.title) } } }
In this example, the ButtonWrapper
struct creates a UIButton that toggles a Boolean state in the parent SwiftUI view when tapped. The Coordinator
class acts as the delegate to handle button actions, bridging the gap between UIKit events and SwiftUI state management. This integration allows you to harness UIKit’s rich functionality while maintaining a reactive SwiftUI interface.
Moreover, if you need to use a full UIKit view controller, you can implement UIViewControllerRepresentable
. For instance, if we wanted to present a simple image picker using UIKit, we could do it like this:
import PhotosUI struct ImagePicker: UIViewControllerRepresentable { @Environment(.presentationMode) var presentationMode @Binding var selectedImage: UIImage? func makeCoordinator() -> Coordinator { Coordinator(self) } func makeUIViewController(context: Context) -> UIImagePickerController { let picker = UIImagePickerController() picker.delegate = context.coordinator return picker } func updateUIViewController(_ uiViewController: UIImagePickerController, context: Context) {} class Coordinator: NSObject, UIImagePickerControllerDelegate, UINavigationControllerDelegate { var parent: ImagePicker init(_ parent: ImagePicker) { self.parent = parent } func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) { if let image = info[.originalImage] as? UIImage { parent.selectedImage = image } parent.presentationMode.wrappedValue.dismiss() } func imagePickerControllerDidCancel(_ picker: UIImagePickerController) { parent.presentationMode.wrappedValue.dismiss() } } } struct ContentView: View { @State private var selectedImage: UIImage? var body: some View { VStack { Button("Select Image") { // Present the image picker } if let image = selectedImage { Image(uiImage: image) .resizable() .scaledToFit() .frame(height: 300) } } } }
This code snipped outlines how to create a custom image picker, allowing users to select images from their photo library. The Coordinator
class manages delegate methods to handle the selection and cancellation of the image picker, ensuring proper communication between UIKit and SwiftUI.
In scenarios where you want to maintain a cohesive design while using UIKit’s capabilities, SwiftUI’s integration can be a game changer. Whether you’re building a complex user interface with existing UIKit components or enhancing SwiftUI’s capabilities with UIKit’s robust features, this seamless integration provides the flexibility needed for modern app development.