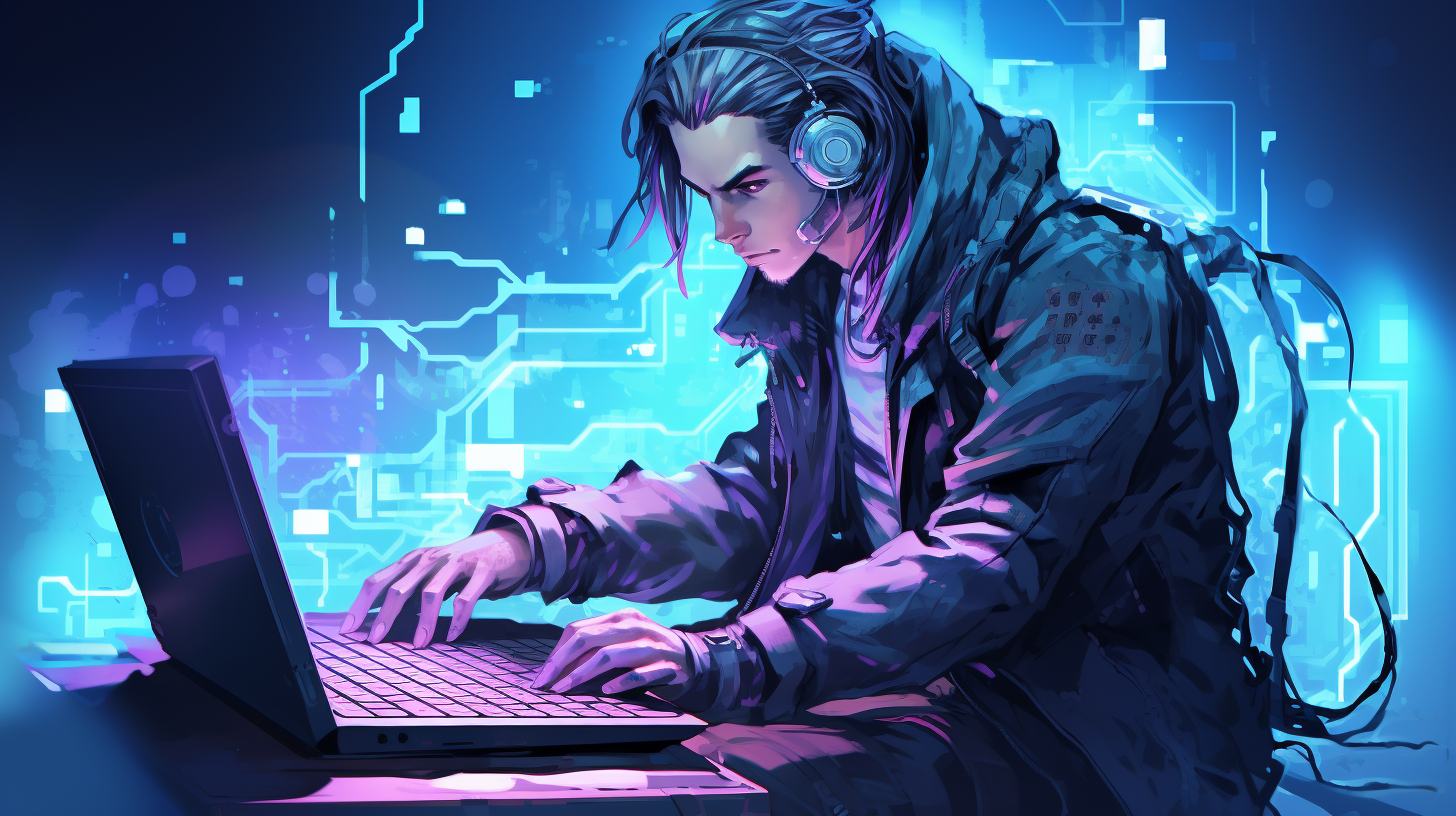
Python and Public Safety: Data Analysis
Data analysis plays a pivotal role in enhancing public safety by transforming raw data into actionable insights. At its core, the process involves collecting, processing, and analyzing diverse datasets to identify patterns, predict incidents, and allocate resources more effectively. The implications of these analyses extend beyond mere statistics; they can directly influence policy decisions, law enforcement strategies, and community engagement efforts.
One of the essential aspects of data analysis in public safety is the ability to visualize complex data. Python, with its rich ecosystem of libraries such as Pandas for data manipulation, Matplotlib for plotting, and Seaborn for statistical visualizations, empowers analysts to create insightful representations of data. These visualizations can highlight trends that may not be immediately apparent from raw data alone.
For instance, think the following Python code that demonstrates how to load crime data, perform a basic analysis, and visualize the results:
import pandas as pd import matplotlib.pyplot as plt # Load the crime dataset data = pd.read_csv('crime_data.csv') # Group by crime type and count occurrences crime_counts = data['crime_type'].value_counts() # Plotting the crime counts plt.figure(figsize=(10, 6)) crime_counts.plot(kind='bar') plt.title('Number of Crimes by Type') plt.xlabel('Crime Type') plt.ylabel('Number of Incidents') plt.xticks(rotation=45) plt.tight_layout() plt.show()
This example showcases how easily Python can be employed to analyze and visualize crime data. By grouping the data by crime type and counting occurrences, we can derive insights that help in understanding which types of crime are most prevalent. Such analyses can inform law enforcement strategies, enabling them to focus resources on high-crime areas or specific types of offenses.
Furthermore, the predictive capabilities of data analysis cannot be overstated. Using machine learning techniques, analysts can forecast crime occurrences based on historical data. By using libraries such as Scikit-learn, practitioners can build models that take into account various factors like time, location, and socioeconomic indicators. Here’s a simple example of how to implement a predictive model:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier # Features and target variable X = data[['hour', 'day_of_week', 'location']] y = data['crime_type'] # Split the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the model model = RandomForestClassifier() model.fit(X_train, y_train) # Assess model accuracy accuracy = model.score(X_test, y_test) print(f'Model Accuracy: {accuracy:.2f}')
This code snippet trains a Random Forest Classifier on historical crime data, assessing its performance on unseen data. Such predictive models can significantly enhance proactive policing efforts, allowing law enforcement to anticipate and address potential incidents before they escalate.
Key Data Sources for Public Safety Analytics
In the landscape of public safety analytics, understanding where to source data is important for effective analysis. Diverse data sources provide the foundation for insights that can drive decision-making and resource allocation. These sources can be broadly categorized into three types: governmental datasets, community-generated data, and private sector data.
Governmental Datasets are abundant and varied, often serving as the backbone for public safety analytics. Law enforcement agencies regularly publish crime reports, arrest records, and enforcement actions. Additionally, datasets from departments such as health, transportation, and housing can provide context to crime trends. A prime example is the FBI’s Uniform Crime Reporting (UCR) Program, which compiles nationwide data on crime statistics. Analysts can utilize this data to establish baselines and identify areas of concern.
Here is an example of how to load and analyze data from the UCR dataset using Python:
import pandas as pd # Load the UCR dataset ucr_data = pd.read_csv('ucr_data.csv') # Display basic statistics print(ucr_data.describe()) # Filter for violent crimes violent_crimes = ucr_data[ucr_data['crime_type'].isin(['Murder', 'Robbery', 'Assault'])] # Count occurrences of violent crimes violent_crime_counts = violent_crimes['year'].value_counts().sort_index() # Display the counts print(violent_crime_counts)
Moreover, community-generated data increasingly plays a role in public safety analytics. Platforms such as Nextdoor or neighborhood social media groups can provide real-time information about local incidents, safety concerns, and community engagement. This data may not be as rigorously structured as governmental data but often reflects current sentiments and immediate neighborhood issues, making it a valuable resource for timely decision-making.
For instance, analysts can aggregate and analyze community alerts and reports to spot emerging trends in local safety concerns. Below is a Python snippet illustrating how to aggregate this kind of data:
import pandas as pd # Load community alert data community_data = pd.read_csv('community_alerts.csv') # Group by type of alert and count occurrences alert_counts = community_data['alert_type'].value_counts() # Display the results print(alert_counts)
Finally, we cannot overlook the contribution of private sector data. Companies that deal with property insurance, retail security, or even social media platforms can provide invaluable data that can inform public safety initiatives. This data may include information on property crimes, fraud incidents, or public sentiment analysis from social media. By using APIs or data partnerships, public safety analysts can integrate this information into their analyses.
Incorporating these various data sources allows for a more comprehensive understanding of public safety challenges. Analysts can perform cross-sectional analyses that correlate crime trends with socioeconomic factors, weather patterns, or other variables. Here’s how one might use Python to join multiple datasets for deeper insights:
import pandas as pd # Load crime and socio-economic datasets crime_data = pd.read_csv('crime_data.csv') socio_economic_data = pd.read_csv('socio_economic_data.csv') # Merge datasets on a common key, e.g., zip code merged_data = pd.merge(crime_data, socio_economic_data, on='zip_code') # Display combined dataset print(merged_data.head())
Techniques for Analyzing Crime Trends
Analyzing crime trends requires a meticulous approach, integrating various analytical techniques to uncover insights that drive actionable strategies. One of the most powerful methodologies in this domain is time-series analysis, which allows analysts to identify patterns over specific time frames, revealing seasonal trends, cyclical behaviors, and anomalies. Using Python’s robust libraries, such as Statsmodels, analysts can construct models that predict future crime occurrences based on historical data.
import pandas as pd import statsmodels.api as sm # Load the crime dataset data = pd.read_csv('monthly_crime_data.csv', parse_dates=['date'], index_col='date') # Resample the data to monthly frequency and sum the counts monthly_crime = data.resample('M').sum() # Fit a seasonal decomposition model decomposition = sm.tsa.seasonal_decompose(monthly_crime['crime_count'], model='additive') decomposition.plot() plt.show()
This example illustrates how to decompose crime data into its trend, seasonality, and residual components. Analysts can use this information to understand how crime levels fluctuate throughout the year, which can inform resource allocation and policing strategies during peak periods of criminal activity.
Moreover, geographic information systems (GIS) play an essential role in the spatial analysis of crime trends. By spatially visualizing crime data, analysts can pinpoint hotspots—areas with a high frequency of crime incidents. Python’s Folium library allows for the creation of interactive maps that can provide law enforcement agencies with vital spatial insights.
import folium # Create a base map map_center = [data['latitude'].mean(), data['longitude'].mean()] crime_map = folium.Map(location=map_center, zoom_start=12) # Add crime data to the map for index, row in data.iterrows(): folium.CircleMarker(location=(row['latitude'], row['longitude']), radius=5, color='red', fill=True, fill_color='red', fill_opacity=0.6).add_to(crime_map) # Display the map crime_map.save('crime_map.html')
Through this code, analysts can visualize crime incidents on a map, providing a clear representation of where crime is occurring. This spatial perspective is invaluable for law enforcement, enabling them to identify high-risk areas and deploy resources more effectively.
Another technique that can be applied is cluster analysis, which can help identify groups or clusters of similar crime incidents. By employing machine learning algorithms such as K-Means clustering, analysts can segment crime data into distinct categories, each representing a unique criminal pattern.
from sklearn.cluster import KMeans import matplotlib.pyplot as plt # Prepare data for clustering (e.g., using latitude and longitude) X = data[['latitude', 'longitude']] # Fit the K-Means model kmeans = KMeans(n_clusters=5) data['cluster'] = kmeans.fit_predict(X) # Plot the clusters plt.scatter(data['longitude'], data['latitude'], c=data['cluster'], cmap='viridis') plt.title('Crime Clusters') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
This clustering technique enables law enforcement agencies to recognize crime patterns based on geographic and demographic factors, further refining their strategies to combat crime effectively.
Additionally, sentiment analysis of social media data can serve as a supplementary method to gauge public sentiment regarding safety and crime in neighborhoods. By using natural language processing (NLP) libraries such as NLTK or TextBlob, analysts can assess the sentiment of community discussions, identifying areas of concern that may not be reflected in traditional crime statistics.
from textblob import TextBlob # Example of community comments comments = ["I feel unsafe walking at night.", "The neighborhood is getting better!", "Too many break-ins lately."] # Analyze sentiment sentiments = [TextBlob(comment).sentiment.polarity for comment in comments] print(sentiments)
Case Studies: Successful Applications of Python in Public Safety
Case studies of successful applications of Python in public safety illustrate the transformative impact of data analysis on law enforcement strategies and community safety. One notable example comes from the Chicago Police Department, which has leveraged predictive policing algorithms to allocate resources more effectively in areas with high crime forecasts. By using a combination of historical crime data and real-time analytics, the department can identify potential hotspots before incidents occur.
In their approach, the Chicago Police Department adopted a machine learning model built with Python’s Scikit-learn library. This model analyzes a multitude of variables, including past crime reports, weather conditions, and social demographics, to generate predictions about where and when crimes are likely to happen. The following Python code snippet demonstrates how such a predictive model could be structured:
from sklearn.model_selection import train_test_split from sklearn.ensemble import GradientBoostingClassifier # Load and prepare the dataset data = pd.read_csv('chicago_crime_data.csv') X = data[['hour', 'day_of_week', 'location', 'weather_conditions']] y = data['crime_occurrence'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the Gradient Boosting model model = GradientBoostingClassifier() model.fit(X_train, y_train) # Evaluate the model's performance accuracy = model.score(X_test, y_test) print(f'Model Accuracy: {accuracy:.2f}')
This model’s predictive capabilities have enabled officers to prioritize patrols in areas identified as high-risk, thereby increasing the likelihood of deterring crime before it happens. The impact has been significant, with some reports indicating a measurable reduction in crime rates in targeted areas.
Another compelling case comes from the Los Angeles Police Department (LAPD), which implemented a Python-based data dashboard to enhance situational awareness among officers. The dashboard aggregates various data sources—crime reports, traffic incidents, and emergency calls—into a simple to operate interface that officers can access in real time. This centralized access to diverse data has transformed operations by enabling officers to make informed decisions on the fly.
To create such a dashboard, the LAPD utilized Flask, a Python web framework, alongside Plotly for interactive visualizations. Below is an example of how to set up a simple dashboard using Flask:
from flask import Flask, render_template import pandas as pd import plotly.express as px app = Flask(__name__) # Load data data = pd.read_csv('lapd_crime_data.csv') @app.route('/') def home(): fig = px.bar(data, x='crime_type', y='incident_count', title='Crime Types Overview') graph_html = fig.to_html(full_html=False) return render_template('dashboard.html', plot=graph_html) if __name__ == '__main__': app.run(debug=True)
This simple web application pulls in crime data and visualizes it as a bar chart, allowing officers to quickly grasp the current crime landscape in their jurisdiction. It serves as a powerful example of how integrating technology with public safety efforts can enhance decision-making and operational efficiency.
Furthermore, the New York City Police Department (NYPD) has employed Python for social media sentiment analysis, using natural language processing to gauge public perception of safety. By analyzing tweets and community posts, the NYPD can respond proactively to public concerns and adjust their strategies accordingly, ensuring that their tactics align with community sentiment. This has been achieved with libraries such as NLTK and Spacy, which are excellent tools for text analysis.
import pandas as pd from textblob import TextBlob # Load tweets data tweets_data = pd.read_csv('nypd_tweets.csv') # Analyze sentiment of tweets tweets_data['sentiment'] = tweets_data['tweet'].apply(lambda x: TextBlob(x).sentiment.polarity) # Summarize sentiment results sentiment_summary = tweets_data['sentiment'].describe() print(sentiment_summary)
This example demonstrates how the NYPD can quantify public sentiment, identifying trends that may indicate rising concerns or improving perceptions of safety. By integrating such analyses into their operational framework, law enforcement agencies can forge stronger connections with the communities they serve, fostering trust and collaboration.
Future Directions for Data-Driven Public Safety Initiatives
As we look toward the future of data-driven public safety initiatives, it is essential to recognize the transformative potential of emerging technologies and methodologies. One of the most promising directions is the increasing integration of artificial intelligence (AI) and machine learning (ML) into public safety analytics. These technologies can enhance predictive capabilities, automate routine tasks, and provide deeper insights into complex data patterns.
For example, AI-driven systems can analyze vast datasets from various sources—ranging from traditional crime reports to social media feeds—allowing law enforcement agencies to identify trends and predict criminal behavior with greater accuracy. The following Python code snippet illustrates how to implement a basic neural network model using TensorFlow for predicting crime incidents:
import pandas as pd import tensorflow as tf # Load crime dataset data = pd.read_csv('crime_data.csv') X = data[['hour', 'day_of_week', 'location']] y = data['crime_occurrence'] # Normalize the features X_normalized = (X - X.mean()) / X.std() # Create a simple neural network model model = tf.keras.Sequential([ tf.keras.layers.Dense(32, activation='relu', input_shape=(X_normalized.shape[1],)), tf.keras.layers.Dense(16, activation='relu'), tf.keras.layers.Dense(1, activation='sigmoid') ]) # Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Train the model model.fit(X_normalized, y, epochs=10, batch_size=32)
This model can be trained on historical crime data to identify underlying patterns, helping law enforcement agencies anticipate where incidents are likely to occur. The potential for AI to augment human decision-making processes cannot be overstated, particularly when it comes to resource allocation and proactive policing strategies.
Another exciting development is the use of real-time data streaming technologies, such as Apache Kafka and Spark Streaming. These tools allow agencies to process data as it arrives, making it possible to respond promptly to emerging threats. In this context, Python’s compatibility with real-time data pipelines makes it a suitable choice for building responsive public safety systems.
Here’s a simple illustration of how to set up a Kafka consumer in Python to process incoming crime data:
from kafka import KafkaConsumer # Set up the Kafka consumer consumer = KafkaConsumer( 'crime_topic', bootstrap_servers=['localhost:9092'], auto_offset_reset='earliest', enable_auto_commit=True, group_id='crime_group' ) # Process incoming messages for message in consumer: print(f'Received message: {message.value.decode()}')
This setup allows agencies to receive real-time alerts about crimes as they happen, enabling a quicker response from law enforcement. The ability to act swiftly based on real-time data is a significant advantage in enhancing community safety.
Moreover, the rise of open data initiatives and community engagement is paving the way for collaborative approaches to public safety. By providing access to datasets related to crime, traffic incidents, and other public safety issues, governments can empower citizens and organizations to analyze data and contribute to safety strategies. Platforms built on Python frameworks such as Dash or Flask can facilitate the development of user-friendly applications and dashboards that present this data in an accessible format.
As an illustration, ponder how a web application can be structured to visualize public safety data, allowing community members to interact with crime statistics:
from flask import Flask, render_template import pandas as pd import plotly.express as px app = Flask(__name__) # Load and prepare data data = pd.read_csv('public_safety_data.csv') @app.route('/') def index(): fig = px.scatter(data, x='longitude', y='latitude', color='crime_type', title='Crime Incidents Map') graph_html = fig.to_html(full_html=False) return render_template('index.html', plot=graph_html) if __name__ == '__main__': app.run(debug=True)
This application serves as a powerful tool for community engagement, fostering transparency and collaboration between citizens and law enforcement. It empowers local residents to visualize crime trends within their neighborhoods, thereby enhancing awareness and encouraging proactive measures.
Finally, the incorporation of privacy-preserving techniques such as differential privacy can ensure that while agencies leverage data for public safety, the personal information of citizens remains protected. As our understanding of privacy implications evolves, the development of methodologies to anonymize data while retaining its utility will be critical in maintaining public trust.