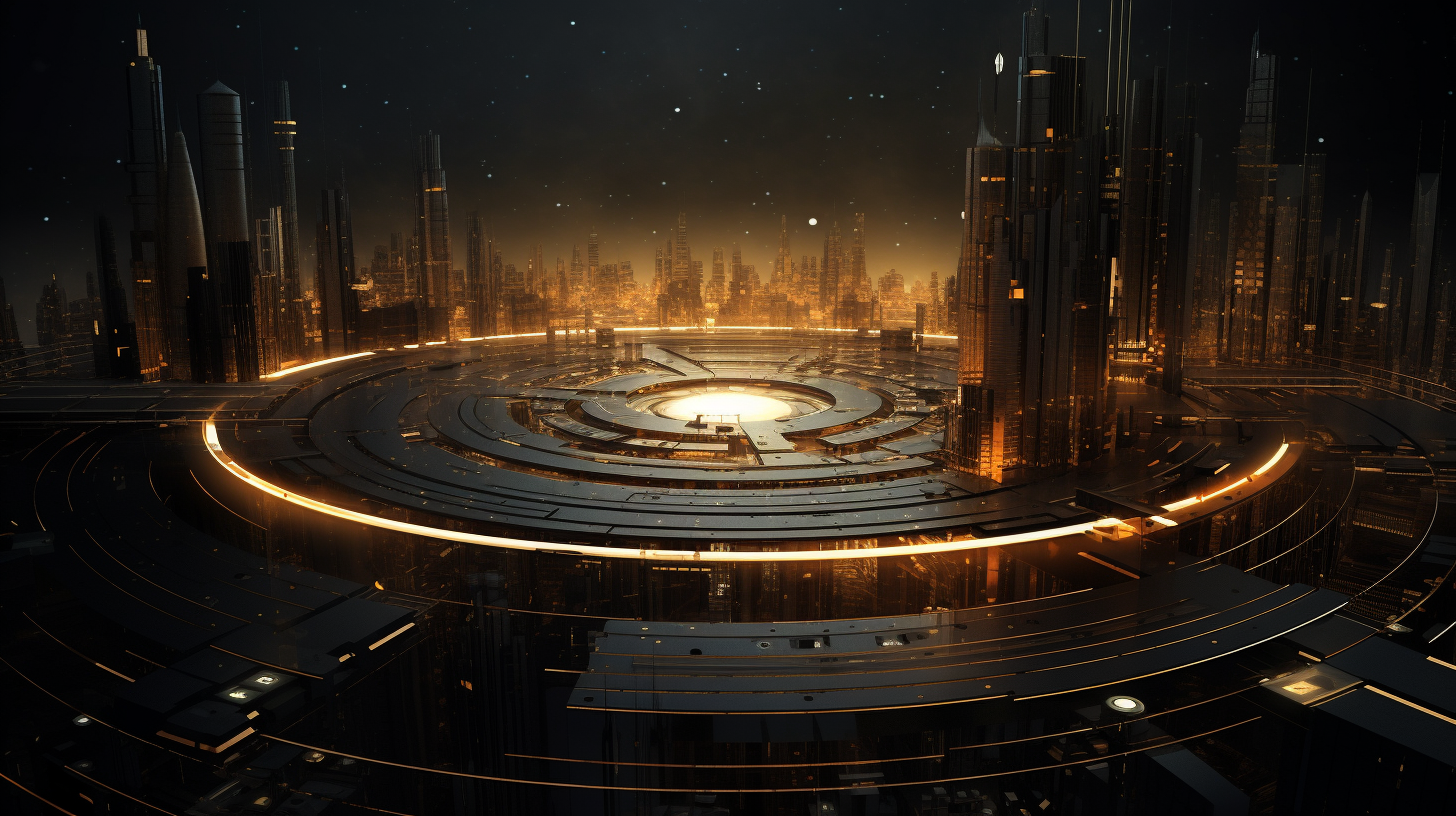
Swift and JSON Handling
JSON, or JavaScript Object Notation, is a lightweight data interchange format that’s easy for humans to read and write, and easy for machines to parse and generate. Its simplicity and readability have made it ubiquitous in web applications, particularly as a format for API responses.
A JSON structure is made up of key-value pairs, much like a dictionary in Swift. The keys are strings, while the values can be strings, numbers, objects, arrays, booleans, or null. This flexibility allows for a rich representation of data. JSON objects are defined by curly braces {}
, and arrays are denoted by square brackets []
.
Here’s a quick look at the fundamental components of JSON:
{ "name": "Alice", "age": 30, "isStudent": false, "courses": ["Math", "Science"], "address": { "street": "123 Main St", "city": "New York" } }
In this example, we have a JSON object representing a person. The name is a string, age is a number, isStudent is a boolean, courses is an array, and address is another JSON object containing more key-value pairs.
Arrays in JSON can hold multiple values of any type, allowing for complex data structures. Here’s an example of a JSON array:
[ { "name": "Alice", "age": 30 }, { "name": "Bob", "age": 25 } ]
This array contains two objects, each representing a person. The ability to nest objects and arrays within one another allows for a highly structured format that can model intricate data relationships.
Another aspect to ponder is that JSON does not support comments. This can be both a blessing and a curse; it keeps the data clean but can make it harder to document complex data structures without external references.
When working with Swift, understanding the JSON structure especially important, as it directly influences how we map these data structures to Swift types. Swift provides a powerful mechanism through the Codable protocol, which simplifies the encoding and decoding of JSON data into native Swift objects. This protocol is key to translating the JSON format into a usable form in Swift applications.
The basic understanding of JSON structure and format lays the groundwork for effective data interchange in Swift applications. By grasping how to represent complex data with JSON, developers can leverage Swift’s powerful features to seamlessly integrate and manipulate that data.
Swift’s Built-in JSON Handling with Codable
Swift’s Codable protocol is an essential part of the language’s ecosystem for handling JSON data. By conforming to the Codable protocol, you enable your custom types to be easily encoded and decoded to and from JSON, streamlining the process of working with external data sources. The Codable protocol combines two other protocols: Encodable and Decodable, which allow your types to be serialized into a format suitable for JSON and deserialized back into Swift types, respectively.
To show how Codable works, ponder the following example where we create a simple Swift struct that represents a person:
struct Person: Codable { let name: String let age: Int let isStudent: Bool let courses: [String] let address: Address } struct Address: Codable { let street: String let city: String }
In this structure, the Person conforms to Codable, which means it can be easily mapped from and to JSON. Note that the Address struct also conforms to Codable, which reflects the nested nature of our JSON data.
Now, let’s see how to encode a Person instance into JSON data:
let person = Person(name: "Alice", age: 30, isStudent: false, courses: ["Math", "Science"], address: Address(street: "123 Main St", city: "New York")) do { let encoder = JSONEncoder() encoder.outputFormatting = .prettyPrinted // For easier readability let jsonData = try encoder.encode(person) let jsonString = String(data: jsonData, encoding: .utf8) print(jsonString ?? "Failed to convert data to string") } catch { print("Error encoding person: (error)") }
In this code snippet, we create a JSONEncoder instance and use it to encode the person instance. The result is a JSON representation of the object, which is then printed as a formatted string. It’s worth noting the use of outputFormatting to make the JSON more readable.
Conversely, decoding JSON data back into a Swift type is just as simpler. Suppose we have the following JSON data representing a person:
let jsonString = """ { "name": "Alice", "age": 30, "isStudent": false, "courses": ["Math", "Science"], "address": { "street": "123 Main St", "city": "New York" } } """
We can decode this JSON into a Swift object like this:
let jsonData = jsonString.data(using: .utf8)! do { let decoder = JSONDecoder() let decodedPerson = try decoder.decode(Person.self, from: jsonData) print("Decoded person: (decodedPerson)") } catch { print("Error decoding JSON: (error)") }
In this example, we first convert the JSON string into Data using data(using: .utf8). We then use a JSONDecoder to decode this data back into a Person instance. The use of try indicates that decoding can throw an error, which we handle with a do-catch block.
Through the power of the Codable protocol, Swift simplifies the tedious task of manually parsing JSON objects. Whenever you define a custom struct or class, simply conform to Codable, and the heavy lifting of encoding and decoding is handled for you. This not only improves code readability but also reduces the potential for bugs during data conversion, thereby enhancing the robustness of your application.
Parsing JSON Data: Practical Examples
let jsonArrayString = """ [ { "name": "Alice", "age": 30, "isStudent": false, "courses": ["Math", "Science"], "address": { "street": "123 Main St", "city": "New York" } }, { "name": "Bob", "age": 25, "isStudent": true, "courses": ["English", "History"], "address": { "street": "456 Elm St", "city": "Los Angeles" } } ] """
When handling arrays of JSON objects, the decoding process remains efficient and clear. For instance, given the JSON array we just defined, we can decode it into an array of `Person` objects as follows:
let jsonArrayData = jsonArrayString.data(using: .utf8)! do { let decoder = JSONDecoder() let decodedPersons = try decoder.decode([Person].self, from: jsonArrayData) decodedPersons.forEach { person in print("Decoded person: (person)") } } catch { print("Error decoding JSON array: (error)") }
In this case, we specify that we want to decode the JSON data into an array of `Person` instances. The decoder handles the array’s structure seamlessly, allowing us to work with multiple objects without additional code complexity.
Additionally, when dealing with possible variations in the JSON structure—such as optional fields or varying data types—Swift allows us to improve our models with optionals and provide default values. For example, if we modify our `Person` struct to allow for a nullable `courses` field, we can do so as follows:
struct Person: Codable { let name: String let age: Int let isStudent: Bool let courses: [String]? let address: Address }
This adjustment ensures that if the `courses` field is absent in the JSON data, our application won’t crash, and we can simply handle the `nil` case in our business logic.
Another common scenario is managing inconsistent key naming conventions between Swift properties and JSON keys. Swift’s `CodingKeys` enum provides a solution for this. For instance, if our JSON keys use snake_case naming, we can map them to Swift’s camelCase properties:
struct Person: Codable { let name: String let age: Int let isStudent: Bool let courses: [String]? let address: Address enum CodingKeys: String, CodingKey { case name case age case isStudent = "is_student" case courses case address } }
This technique allows us to retain Swift’s naming conventions while still accurately decoding the data from the outside world.
When parsing JSON data, it is essential to keep in mind the performance implications as well. While Swift’s Codable provides a high-level abstraction for JSON parsing, ensuring that the data is not overly complex can enhance performance. Striking a balance between the richness of the data structure and the efficiency of parsing will serve to enhance the overall responsiveness of your application.
To wrap it up, parsing JSON data in Swift using the Codable protocol transforms what could be a cumbersome task into an intuitive and streamlined process. With the right understanding and application of Swift’s powerful features, developers can navigate JSON handling with ease, integrating vast amounts of data into their applications efficiently and effectively.
Error Handling in JSON Parsing
Error handling is an integral part of parsing JSON data in Swift, as it ensures that your application can gracefully handle unexpected situations, such as malformed JSON or inconsistencies in the data structure. When working with JSON, a variety of errors can occur, such as type mismatches, network issues, or even problems with the JSON itself. Swift provides a robust error handling mechanism that allows you to catch and respond to these errors effectively.
When using the Codable protocol for decoding JSON, the decoding process is wrapped in a do-catch block to manage potential errors. Here’s a general structure you can follow:
do { let decoder = JSONDecoder() let decodedData = try decoder.decode(Person.self, from: jsonData) // Use decodedData here } catch let decodingError { // Handle the error print("Decoding error: (decodingError)") }
In this example, if the JSON data cannot be decoded into a `Person` object, the catch block will execute, so that you can log the error or take appropriate actions, like notifying the user or attempting to fetch the data again.
Swift’s error handling allows for the categorization of errors, which can be particularly useful when debugging. For instance, you may want to differentiate between a network error and a decoding error. You can create custom error types to represent specific issues in your JSON handling:
enum JSONError: Error { case invalidData case decodingError(Error) }
With this enumeration, you can modify your JSON parsing logic to throw specific errors when encountering problems:
func decodePerson(from jsonData: Data) throws -> Person { let decoder = JSONDecoder() do { return try decoder.decode(Person.self, from: jsonData) } catch { throw JSONError.decodingError(error) } }
This allows you to handle errors in a more granular way. For example, you can catch and log a decoding error specifically, improving your debugging capabilities:
do { let person = try decodePerson(from: jsonData) // Successfully decoded person } catch JSONError.decodingError(let error) { print("Decoding failed with error: (error)") } catch { print("An unexpected error occurred: (error)") }
In addition to handling decoding errors, consider potential issues with the network layer when fetching JSON data. Network operations should also be wrapped in error handling to manage connectivity issues or server errors:
func fetchJSON(from url: URL, completion: @escaping (Result) -> Void) { URLSession.shared.dataTask(with: url) { data, response, error in if let error = error { completion(.failure(.invalidData)) // Handle network error return } guard let data = data else { completion(.failure(.invalidData)) // Handle missing data return } do { let person = try decodePerson(from: data) completion(.success(person)) } catch let decodingError { completion(.failure(.decodingError(decodingError))) } }.resume() }
In this `fetchJSON` function, we handle both network errors and decoding errors, providing a clear path for error management. The completion handler uses a `Result` type, which allows the caller to easily understand whether the operation succeeded or failed.
Lastly, when defining your data models, consider using optionals for fields that may or may not be present in the JSON. This helps avoid forced unwrapping of values and makes your JSON parsing more resilient. Here’s how you might adjust a property in your `Person` model:
struct Person: Codable { let name: String let age: Int let isStudent: Bool let courses: [String]? let address: Address }
This approach ensures that your application can handle cases where the `courses` field is absent, preventing runtime crashes and making your JSON parsing logic more robust. By implementing comprehensive error handling strategies, you not only improve the reliability of your application but also enhance the overall user experience by providing clear feedback in the event of issues.
Best Practices for Working with JSON in Swift
When working with JSON in Swift, adhering to best practices can significantly enhance both the efficiency and maintainability of your code. Here are several recommendations that effectively leverage Swift’s capabilities while ensuring robust handling of JSON data.
1. Leverage Codable for Data Mapping
Using the Codable
protocol is a foundational practice for JSON serialization and deserialization in Swift. This allows for automatic mapping between JSON and Swift types, reducing boilerplate code and minimizing errors. Always ensure that your model structures conform to Codable
.
struct Person: Codable { let name: String let age: Int let isStudent: Bool let courses: [String] let address: Address } struct Address: Codable { let street: String let city: String }
2. Use Strong Typing and Optionals
Swift’s type system is powerful; utilize it to define your models precisely. Where potential null values exist in JSON, employ optionals to handle such cases gracefully. This prevents crashes due to unexpected nil</code