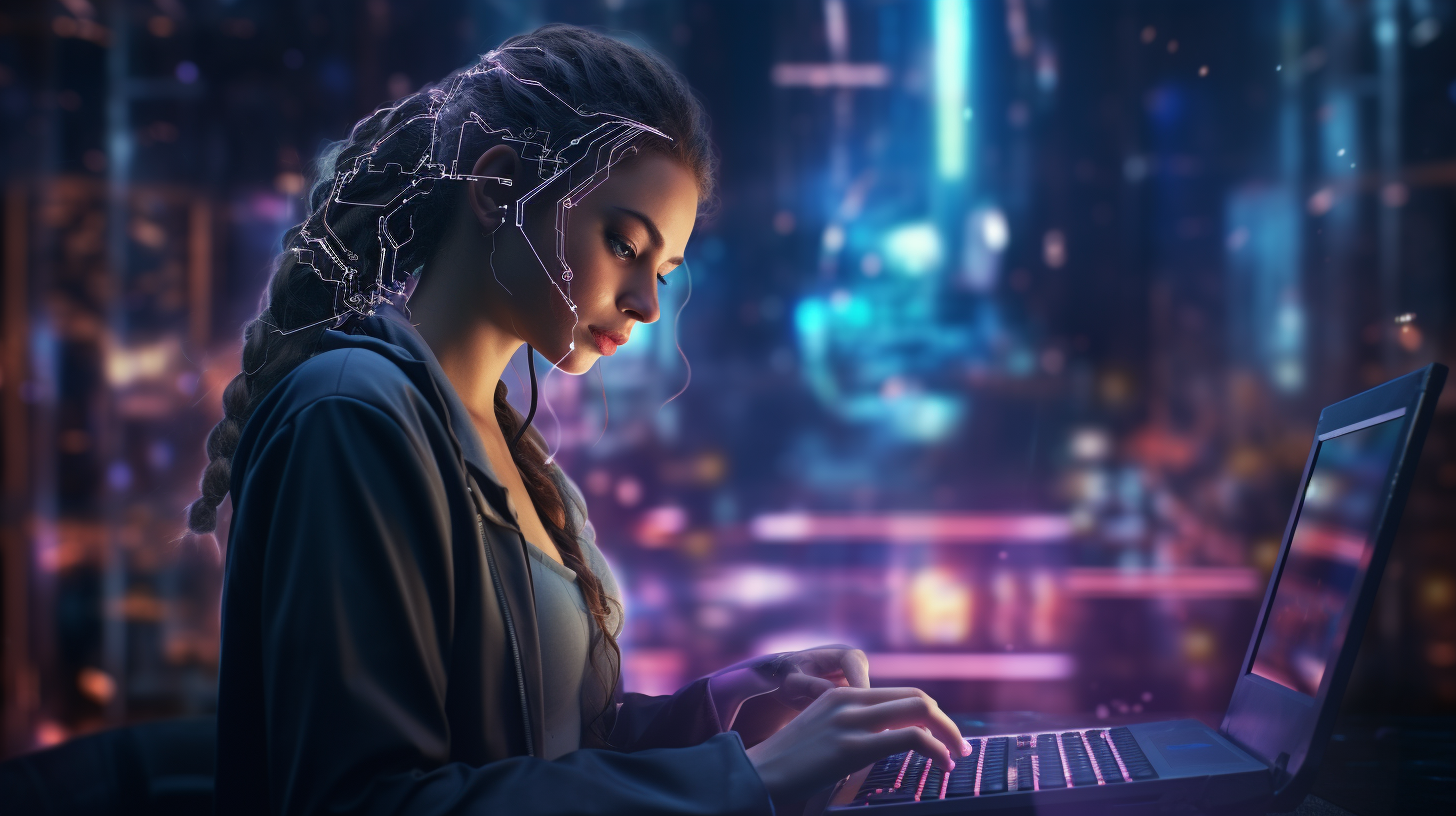
Java Arrays: Single and Multidimensional
In Java, an array is a data structure that holds a fixed number of values of a single type. The length of an array is established when it is created, and it cannot be changed dynamically like a list. Arrays in Java are zero-indexed, meaning the first element is accessed with an index of 0, the second element with an index of 1, and so on.
Arrays can be of any primitive data type, such as int, char, double, etc., or they can hold objects of any class type. This versatility makes arrays a fundamental part of Java programming.
Here’s a quick illustration of how you can define and use a simple array in Java:
// Declaration and initialization of an array int[] numbers = {1, 2, 3, 4, 5}; // Accessing elements in an array for (int i = 0; i < numbers.length; i++) { System.out.println("Element at index " + i + ": " + numbers[i]); }
When working with arrays, it’s important to be aware of their limitations. Since arrays have a fixed size, attempting to access an index outside the bounds of the array will result in an ArrayIndexOutOfBoundsException. For example:
// Attempting to access an invalid index try { System.out.println(numbers[5]); // This will cause an exception } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Caught an exception: " + e.getMessage()); }
Arrays also have built-in methods that allow you to perform various operations, making them a powerful tool in your programming arsenal. For instance, the Arrays class in the java.util package provides static methods to manipulate arrays, including sorting and searching functionalities.
To summarize, arrays are a cornerstone of data handling in Java, and understanding their structure and functionality is vital for effective programming. They facilitate storage and manipulation of collections of data, forming the basis for more complex data structures such as lists and maps.
Declaring and Initializing Single-Dimensional Arrays
Declaring and initializing single-dimensional arrays in Java is simpler and can be done in several ways, depending on your specific requirements. The most common methods include inline initialization, separate declaration and initialization, and using the `new` keyword.
When you declare an array, you can do so with a brief inline initialization, where you define the array and fill it with values at once. This method is concise and often used for small arrays where you know the elements in advance. Here’s how you can do it:
int[] numbers = {10, 20, 30, 40, 50};
In this example, an array named `numbers` is declared and initialized with five integer values. The length of this array is determined automatically by the number of elements enclosed within the braces.
If you prefer to separate the declaration from the initialization, you can first declare the array and then allocate memory for it using the `new` keyword. This is particularly useful when the size of the array is determined at runtime:
int[] scores; // Declaration scores = new int[5]; // Initialization
In this case, you declare an array called `scores` and then create an array of integers with a length of 5. Initially, all elements in the `scores` array will be set to the default value of 0 for integers.
Another way to declare and initialize an array is to do both in one line, using the `new` keyword along with the specific values you want to assign:
String[] fruits = new String[] {"Apple", "Banana", "Cherry"};
This method is especially useful when you want to create an array of objects or strings and still specify the values during declaration. Here, `fruits` is an array of `String` objects initialized with three fruit names.
It is worth noting that in Java, arrays are objects in themselves. This means that you can use methods and properties associated with objects, such as getting the length of the array:
System.out.println("Length of the array: " + numbers.length);
As arrays are indexed collections, accessing and modifying their elements is just as simple. You can assign values to specific indices directly:
scores[0] = 75; // Assigning 75 to the first element System.out.println("Score at index 0: " + scores[0]);
Keep in mind that if you attempt to assign a value to an index that exceeds the declared size of the array, you will run into an `ArrayIndexOutOfBoundsException`, as mentioned previously. Understanding how to declare and initialize arrays properly very important for efficient data management within your Java applications.
Working with Multidimensional Arrays
When delving into the realm of arrays, one of the most powerful constructs provided by Java is the multidimensional array. Consider of multidimensional arrays as arrays of arrays, allowing you to create complex data structures that can represent data in multiple dimensions. The most common type is the two-dimensional array, which essentially creates a table-like structure.
To declare a two-dimensional array in Java, you can follow a similar syntax to that of a single-dimensional array. The declaration involves specifying the type of the elements, followed by two sets of square brackets. Here’s an example:
int[][] matrix;
After declaring the array, you must initialize it. This can be done either by specifying the size of each dimension or by directly initializing it with values. Here’s how to initialize a two-dimensional array with specific dimensions:
matrix = new int[3][4]; // A 3x4 matrix
In this instance, `matrix` is a two-dimensional array with 3 rows and 4 columns. All elements are initially set to zero. For immediate initialization with predefined values, you can utilize the following syntax:
int[][] predefinedMatrix = { {1, 2, 3}, {4, 5, 6} }; // A 2x3 matrix
The `predefinedMatrix` in this example contains two rows and three columns. Each inner array represents a row in the matrix. Accessing elements in a multidimensional array involves specifying both the row and column indices:
System.out.println("Element at row 1, column 2: " + predefinedMatrix[1][2]); // Outputs 6
Working with multidimensional arrays can also extend to iterating through the elements. A nested loop is typically employed for this purpose—one loop for the rows and another for the columns. Here’s how you can iterate through all elements of a two-dimensional array:
for (int i = 0; i < predefinedMatrix.length; i++) { for (int j = 0; j < predefinedMatrix[i].length; j++) { System.out.print(predefinedMatrix[i][j] + " "); } System.out.println(); // Move to the next line after each row }
This code snippet will print out all the elements of the `predefinedMatrix`, row by row. The outer loop iterates over each row, while the inner loop iterates over each column within that row.
It’s also possible to create arrays with more than two dimensions, although the syntax can become cumbersome. A three-dimensional array, for instance, can be declared as follows:
int[][][] threeDArray = new int[2][3][4]; // A 2x3x4 array
Here, `threeDArray` consists of 2 blocks, each containing 3 rows and 4 columns. Accessing an element in a three-dimensional array would similarly require an additional index:
threeDArray[1][2][3] = 10; // Setting a specific value
However, it’s crucial to manage the complexity associated with higher-dimensional arrays judiciously, as they can lead to code that’s difficult to read and maintain. Multidimensional arrays open up a world of possibilities for data representation, allowing developers to tackle intricate programming challenges with elegance and efficiency.
Common Operations and Methods for Arrays
When working with arrays in Java, several common operations and methods can enhance your ability to manage and manipulate data efficiently. The Arrays class in the java.util package provides a wealth of static methods that facilitate various operations, such as sorting, searching, and comparing arrays. Understanding these methods can significantly streamline your coding practices and improve your overall productivity.
One of the most frequently used methods is Arrays.sort(), which organizes the elements of an array in ascending order. This method can be applied to both single-dimensional and multi-dimensional arrays. Here’s an example of how to sort an array of integers:
int[] numbers = {5, 3, 8, 1, 4}; Arrays.sort(numbers); System.out.println("Sorted array: " + Arrays.toString(numbers));
In this snippet, the Arrays.sort(numbers)
method processes the numbers
array and arranges its elements, while Arrays.toString(numbers)
converts the array to a readable string format for display.
Another vital operation you can perform on arrays is searching for an element using the Arrays.binarySearch() method. This method requires the array to be sorted prior to searching and returns the index of the specified element, or a negative value if the element is not found:
int target = 3; int index = Arrays.binarySearch(numbers, target); if (index >= 0) { System.out.println("Element " + target + " found at index: " + index); } else { System.out.println("Element " + target + " not found."); }
In this case, we search for the number 3
in the sorted numbers
array. If found, the index is printed; otherwise, a message indicates that the element is absent.
Moreover, you can use Arrays.copyOf() to create a new array this is a copy of an existing array. That’s particularly useful when you want to resize an array or create a duplicate without altering the original:
int[] copiedArray = Arrays.copyOf(numbers, numbers.length); System.out.println("Copied array: " + Arrays.toString(copiedArray));
This example demonstrates how to create a new array called copiedArray
, which contains the same elements as the original numbers
array.
Comparing arrays is also simpler with the Arrays.equals() method, which checks if two arrays are equal in terms of length and content:
int[] anotherArray = {1, 2, 3, 4, 5}; boolean areEqual = Arrays.equals(numbers, anotherArray); System.out.println("Are the two arrays equal? " + areEqual);
In this example, the areEqual
variable will be set to false
since the contents of numbers
and anotherArray
differ. This functionality is essential when verifying data integrity or ensuring consistency across different datasets.
Additionally, the Arrays.fill() method allows you to fill an entire array or a specific portion of an array with a designated value. This can be invaluable when initializing or resetting an array:
int[] resetArray = new int[5]; Arrays.fill(resetArray, 0); System.out.println("Reset array: " + Arrays.toString(resetArray));
Here, the resetArray
is initialized with the value 0
for all its elements, demonstrating a quick way to set a default state for an array.
In summary, the operations and methods provided by the Arrays class empower developers to perform essential data manipulation tasks with ease. Whether sorting, searching, copying, comparing, or filling arrays, these built-in functionalities greatly enhance your programming capabilities and make array handling in Java not only convenient but also efficient.