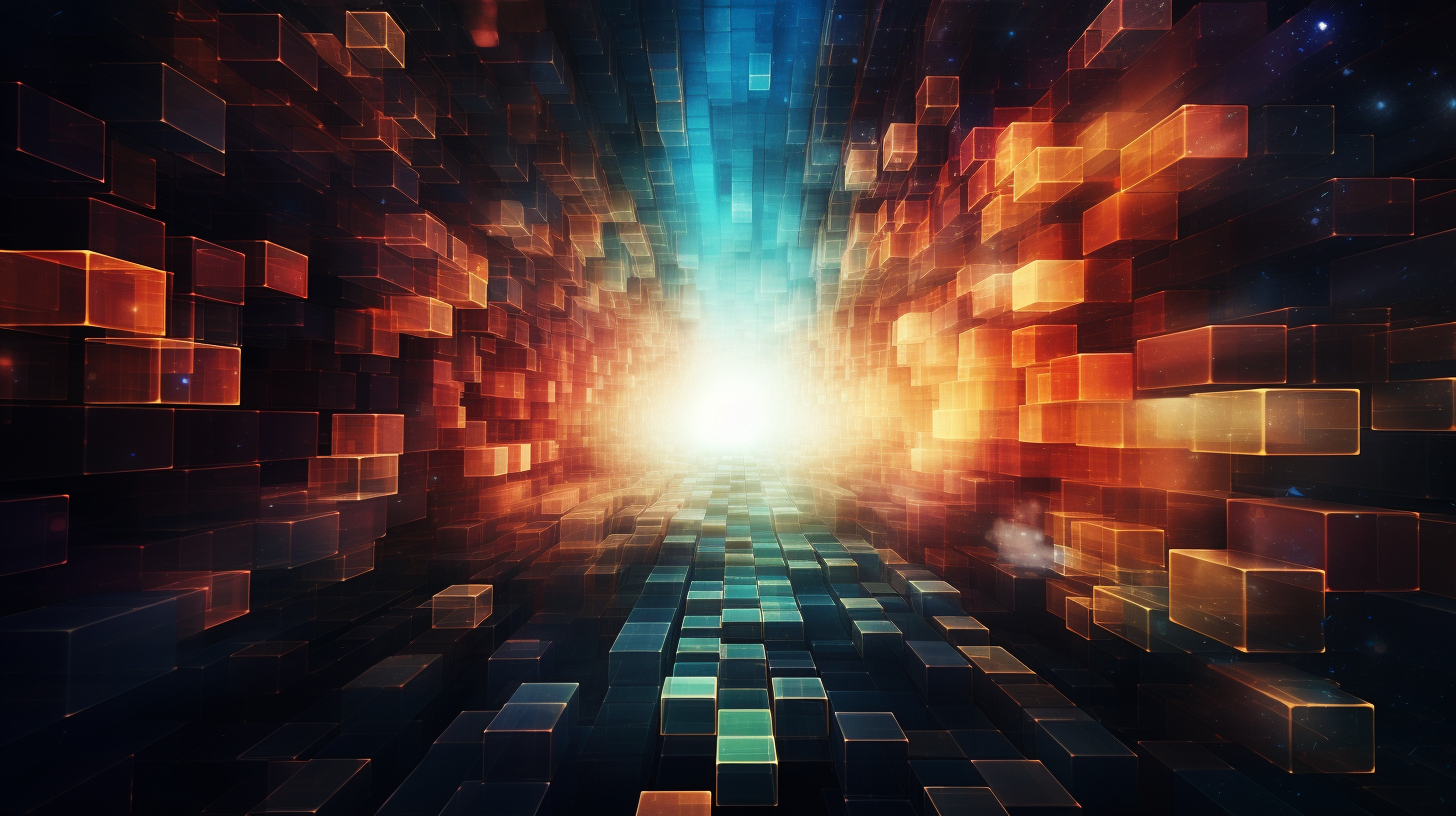
Bash for Data Encryption and Security
Data encryption is a fundamental aspect of modern computing, serving as a line of defense against unauthorized access to sensitive information. At its core, encryption transforms readable data, known as plaintext, into an unreadable format, referred to as ciphertext. The beauty of this transformation lies in its reversibility; only those who possess the correct decryption key can revert the ciphertext back to its original plaintext form.
Encryption employs algorithms that dictate how data is encoded. These algorithms work through a combination of mathematical functions and keys, which are strings of bits that serve as inputs to the encryption process. The strength of encryption largely depends on the complexity of these keys and the algorithms employed. Two primary forms of encryption exist: symmetric and asymmetric.
In symmetric encryption, the same key is used for both encryption and decryption. This method tends to be faster and is suitable for encrypting large amounts of data. However, the challenge lies in securely sharing the key between the parties involved. A common symmetric encryption algorithm is AES (Advanced Encryption Standard), known for its efficiency and strong security.
# Example of AES encryption using OpenSSL echo "My secret message" | openssl enc -aes-256-cbc -e -base64 -k "mysecretpassword"
On the other hand, asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. This method allows anyone to encrypt data using the public key, but only the holder of the private key can decrypt it. Asymmetric encryption is often employed for secure key exchange and digital signatures. The RSA (Rivest-Shamir-Adleman) algorithm is one of the most widely recognized forms of asymmetric encryption.
# Example of RSA encryption using OpenSSL openssl genpkey -algorithm RSA -out private_key.pem openssl rsa -in private_key.pem -outform PEM -pubout -out public_key.pem echo "My secret message" | openssl rsautl -encrypt -pubin -inkey public_key.pem -out encrypted_message.bin
Understanding the theoretical underpinnings of encryption is important, but practical implementation is where the real challenge lies. The choice of algorithms, key lengths, and management of keys all contribute to the overall security of the encrypted data. With the right tools and practices, Bash can facilitate robust encryption and secure data handling strategies.
Using OpenSSL for Secure Data Handling
OpenSSL is a powerful toolkit widely utilized for implementing encryption protocols, providing users with a versatile command-line interface to perform various cryptographic operations. This tool can handle both symmetric and asymmetric encryption, making it a cornerstone for secure data handling in Bash. Using OpenSSL effectively requires familiarity with its commands and options, as well as an understanding of the data flow involved in encryption and decryption processes.
At the heart of OpenSSL’s functionality is its ability to encrypt data securely using strong algorithms. To begin, let’s explore a basic example of symmetric encryption using the Advanced Encryption Standard (AES). The command structure is simple yet effective, which will allow you to encrypt plaintext directly from the shell.
echo "This is a confidential message." | openssl enc -aes-256-cbc -e -base64 -k "examplepassword"
In this command, the data “This is a confidential message.” is piped into OpenSSL, which encrypts it using AES-256 in CBC mode. The -e
flag indicates encryption, while -base64
encodes the output in Base64 format, making it readable as text. The -k
option specifies the encryption key.
On the decryption side, OpenSSL provides a complementary command that mirrors the encryption process. To decrypt the data, you would reverse the operation, using the appropriate key and flags:
echo "BASE64_ENCODED_CIPHERTEXT" | openssl enc -aes-256-cbc -d -base64 -k "examplepassword"
Replace BASE64_ENCODED_CIPHERTEXT
with the output you received from the encryption command. The -d
flag specifies decryption, and the process returns the original plaintext message, provided the correct key is used.
Asymmetric encryption, a different beast altogether, allows for encrypting data with a public key and decrypting it with a private key. This approach is particularly useful for secure communications and file transfers. OpenSSL simplifies this process as well. First, you must generate a pair of keys:
openssl genpkey -algorithm RSA -out private_key.pem
openssl rsa -in private_key.pem -outform PEM -pubout -out public_key.pem
After generating the private key, the command extracts the public key for sharing. You can now use the public key to encrypt messages:
echo "This is another confidential message." | openssl rsautl -encrypt -pubin -inkey public_key.pem -out encrypted_message.bin
To decrypt the message, use the private key with the following command:
openssl rsautl -decrypt -inkey private_key.pem -in encrypted_message.bin
With OpenSSL’s robust features at your disposal, you can implement strong encryption strategies directly from your Bash environment. The key is to understand the nuances of each command and how they fit into your overall data security strategy. This command-line flexibility allows you to automate and streamline encryption tasks, ensuring that your sensitive information remains secure against unauthorized access.
Implementing Symmetric and Asymmetric Encryption
Implementing symmetric and asymmetric encryption in Bash using OpenSSL opens up a world of possibilities for securing sensitive data. As we delve deeper into the nuances of these encryption methods, it’s essential to recognize the appropriate scenarios for their application and the specific commands that facilitate their implementation.
Let’s elaborate on symmetric encryption first, for which the Advanced Encryption Standard (AES) is a popular choice. The beauty of symmetric encryption lies in its simplicity: the same key is used for both encryption and decryption. However, this simplicity can lead to challenges in key management, especially when sharing the key over potentially insecure channels.
To encrypt data using AES in a Bash script, the following command can be employed:
echo "This is a confidential message." | openssl enc -aes-256-cbc -e -base64 -k "examplepassword"
In this command, the plaintext message is piped directly into OpenSSL, which processes the data and returns the ciphertext encoded in Base64. The use of the -e flag explicitly indicates that we are encrypting, while -base64 ensures that the output is in a text-friendly format, suitable for display or transmission.
Decrypting the message is just as simpler. Replace the output from the previous command with BASE64_ENCODED_CIPHERTEXT in the following command:
echo "BASE64_ENCODED_CIPHERTEXT" | openssl enc -aes-256-cbc -d -base64 -k "examplepassword"
The -d flag signals OpenSSL to perform decryption, effectively reverting the ciphertext back to its original plaintext, provided that the correct key is used.
Now, shifting our focus to asymmetric encryption, this method employs a pair of keys, which adds complexity but greatly enhances security. The public key is used for encryption, while the private key is reserved for decryption. This architecture eliminates the need for key sharing, as the public key can be distributed freely, while the private key remains confidential.
To set up RSA asymmetric encryption, begin by generating your key pair:
openssl genpkey -algorithm RSA -out private_key.pem openssl rsa -in private_key.pem -outform PEM -pubout -out public_key.pem
With your keys in place, you can encrypt a message using the public key:
echo "This is another confidential message." | openssl rsautl -encrypt -pubin -inkey public_key.pem -out encrypted_message.bin
When the need arises to decrypt the message, utilize the private key with the following command:
openssl rsautl -decrypt -inkey private_key.pem -in encrypted_message.bin
These commands illustrate the practical usage of both symmetric and asymmetric encryption in a Bash environment. Understanding the subtleties of each method not only enhances your ability to secure data but also equips you with the knowledge to choose the right approach based on your specific needs. As you navigate the landscape of data encryption, OpenSSL serves as a powerful ally, so that you can confidently implement encryption strategies that guard against unauthorized access.
Automating Encryption Processes with Bash Scripts
Automating encryption processes using Bash scripts can significantly enhance efficiency and security when managing sensitive data. By using the power of Bash, you can create scripts that not only perform encryption and decryption tasks but also manage keys, handle multiple files, and integrate with other systems seamlessly. This automation can save considerable time and reduce the likelihood of human error, which very important in a security-sensitive context.
To get started, ponder a simple Bash script that performs AES symmetric encryption on a file. This script will take a plaintext file as input, encrypt it, and then save the ciphertext to a specified output file.
#!/bin/bash # Check for the correct number of arguments if [ "$#" -ne 3 ]; then echo "Usage: $0 " exit 1 fi INPUT_FILE="$1" OUTPUT_FILE="$2" PASSWORD="$3" # Encrypt the file using OpenSSL openssl enc -aes-256-cbc -salt -in "$INPUT_FILE" -out "$OUTPUT_FILE" -k "$PASSWORD" echo "Encryption complete. Encrypted file saved as $OUTPUT_FILE."
This script begins by checking that three arguments are provided: the input file, the output file, and the password for encryption. It then uses the OpenSSL command to encrypt the input file with AES-256-CBC while applying a salt to improve security. The result is saved to the specified output file, ensuring that the process is user-friendly and simpler.
Decryption can also be automated using a similar approach. Here’s an example of a Bash script designed to decrypt an AES-encrypted file:
#!/bin/bash # Check for the correct number of arguments if [ "$#" -ne 3 ]; then echo "Usage: $0 " exit 1 fi INPUT_FILE="$1" OUTPUT_FILE="$2" PASSWORD="$3" # Decrypt the file using OpenSSL openssl enc -aes-256-cbc -d -in "$INPUT_FILE" -out "$OUTPUT_FILE" -k "$PASSWORD" echo "Decryption complete. Decrypted file saved as $OUTPUT_FILE."
In this decryption script, the process mirrors that of encryption. The key difference lies in the use of the -d flag, which instructs OpenSSL to decrypt the input file. The decrypted data is then written to the specified output file. Again, the user is prompted to provide the necessary arguments, making it accessible for non-technical users as well.
For more robust automation, ponder implementing logging and error handling in your scripts. Adding logs can help track successful operations and diagnose any issues that may arise during the encryption or decryption processes.
#!/bin/bash # Set log file path LOG_FILE="encryption_log.txt" # Function to log messages log_message() { echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" >> "$LOG_FILE" } # Check for the correct number of arguments if [ "$#" -ne 3 ]; then log_message "Error: Invalid number of arguments." echo "Usage: $0 " exit 1 fi INPUT_FILE="$1" OUTPUT_FILE="$2" PASSWORD="$3" # Encrypt the file using OpenSSL if openssl enc -aes-256-cbc -salt -in "$INPUT_FILE" -out "$OUTPUT_FILE" -k "$PASSWORD"; then log_message "Encryption of '$INPUT_FILE' to '$OUTPUT_FILE' successful." echo "Encryption complete. Encrypted file saved as $OUTPUT_FILE." else log_message "Error: Encryption failed for '$INPUT_FILE'." echo "Encryption failed." exit 1 fi
In this enhanced script, a logging function captures timestamps and messages, providing a clear record of operations. This inclusion allows for better tracking and troubleshooting, ensuring that you have a complete audit trail of encryption activities.
Automating encryption processes with Bash scripts not only streamlines tasks but also reinforces security protocols, making it easier to consistently apply encryption across various types of data. As you design your scripts, think your specific requirements and how you can leverage Bash to create efficient, secure, and simple to operate solutions for data protection.
Best Practices for Data Security in Bash
When discussing best practices for data security in Bash, it is essential to consider a multifaceted approach that encompasses secure coding techniques, environment hardening, and proper key management. Security is not merely about encryption algorithms; it is about building a comprehensive framework that safeguards sensitive information throughout its lifecycle.
1. Use Strong Passwords and Keys: The strength of your encryption largely hinges on the complexity of the passwords or keys you use. Ensure that your keys are sufficiently long and randomly generated. For example, using a password manager can help create and store complex passwords securely, mitigating the risk associated with weak or reused passwords.
openssl rand -base64 32
This command generates a strong, random key that can be used for encryption, significantly enhancing security.
2. Secure Key Storage: The storage of encryption keys is a critical component of data security. Avoid hardcoding keys directly into your scripts. Instead, think using environment variables or secure vaults to handle your keys dynamically. For instance, you could export your password using:
export ENCRYPTION_KEY="your_secure_key"
And then reference it in your script without revealing it in the source code:
openssl enc -aes-256-cbc -salt -in "$INPUT_FILE" -out "$OUTPUT_FILE" -k "$ENCRYPTION_KEY"
3. Implement Logging and Auditing: Keeping track of who accessed what data and when is important. Implement logging for encryption and decryption processes. This not only helps in audits but also allows for quick identification of potentially malicious activities. Use a logging function in your scripts to capture relevant events:
log_message() { echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" >> "security_log.txt" }
Incorporate calls to this function at crucial points in your scripts to maintain detailed records of operations, including successes and failures.
4. Limit File Permissions: Be mindful of file permissions on scripts and sensitive data. Use the chmod
command to restrict access, ensuring that only authorized users can read or execute your scripts:
chmod 700 my_script.sh
This command makes the script executable only by the owner, reducing the risk of unauthorized access.
5. Keep Software Up to Date: Regularly update your Bash environment and any cryptographic tools you use to protect against vulnerabilities. Ensure that your OpenSSL version is current, as updates often include important security fixes.
sudo apt-get update && sudo apt-get upgrade openssl
This command updates the OpenSSL package on a Debian-based system, helping to maintain security integrity.
6. Validate Input and Output: Always validate the input before processing it, especially when dealing with sensitive data. Sanitize any user input to prevent injection attacks or unintended command execution. Additionally, confirm the success of encryption or decryption operations by checking exit statuses:
if openssl enc -aes-256-cbc -salt -in "$INPUT_FILE" -out "$OUTPUT_FILE" -k "$ENCRYPTION_KEY"; then echo "Encryption successful" else echo "Encryption failed" fi
Implementing these best practices enhances your Bash scripts’ security posture, ensuring that your encryption processes are resilient against various threats. Data security is not a one-time setup; it requires ongoing vigilance, regular updates, and a commitment to following established protocols. By adhering to these principles, you can effectively mitigate risks and protect your sensitive information.