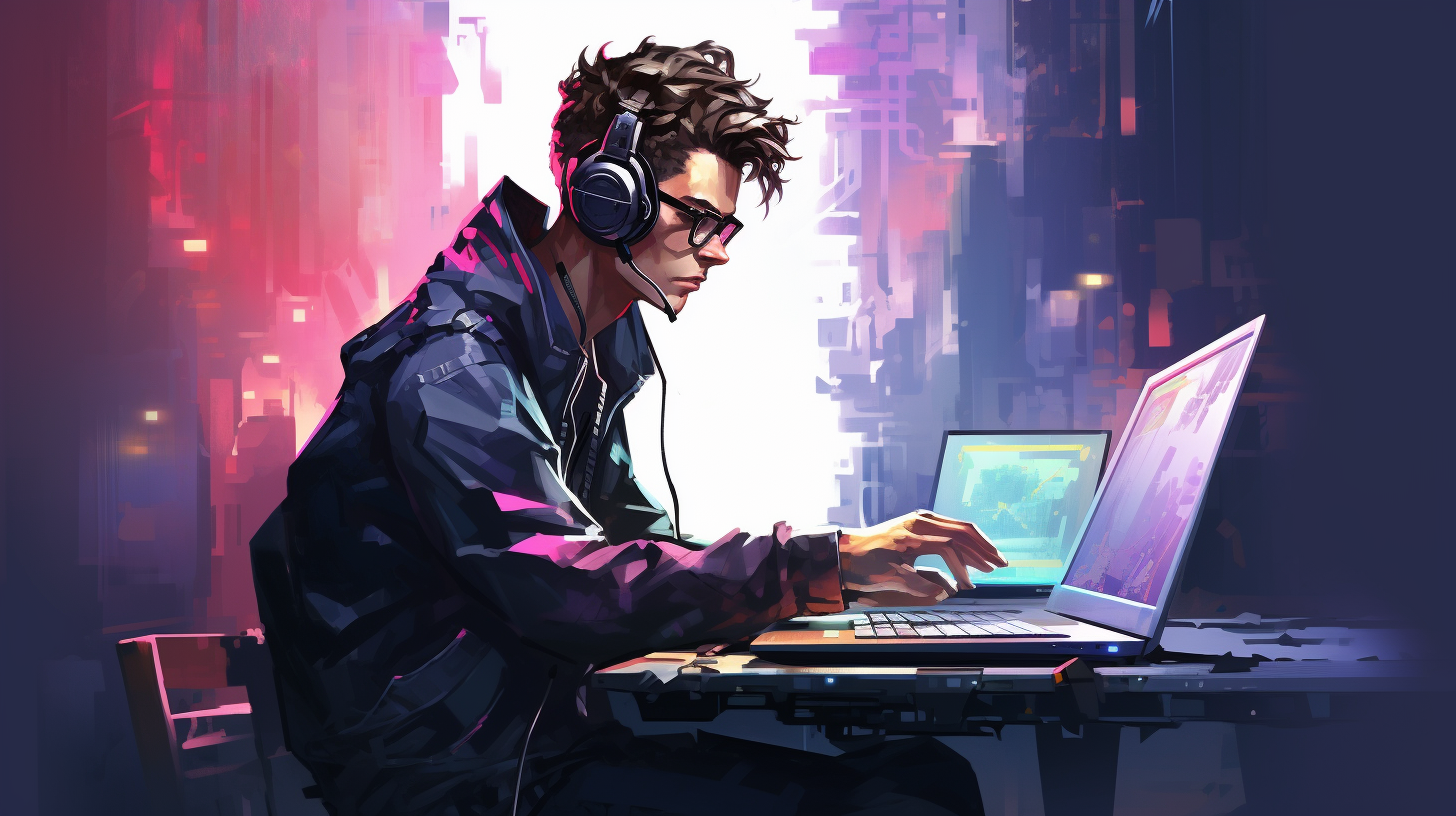
Swift and ARKit
ARKit is Apple’s powerful augmented reality (AR) framework that allows developers to create immersive experiences by blending digital content with the real world. It harnesses the capabilities of the device’s camera and motion sensors to provide a robust environment for rendering 3D objects, recognizing surfaces, and tracking movements in real-time.
At its core, ARKit utilizes computer vision and device motion tracking to deliver a seamless AR experience. The framework enables the detection of horizontal and vertical surfaces, making it simpler to place virtual objects in a realistic manner. By understanding the environment, ARKit can anchor objects to surfaces, track their positions, and maintain their relative orientations as the user moves.
One of the standout features of ARKit is its ability to achieve high levels of performance with relatively low overhead. That’s largely due to its integration with Metal, Apple’s low-level graphics API, which allows developers to render graphics efficiently. Additionally, ARKit supports a variety of rendering techniques, making it possible to create visually stunning applications with relative ease.
To help bridge the gap between the virtual and real worlds, ARKit also provides advanced capabilities such as light estimation, which helps in adjusting the virtual object’s appearance to match the real-world lighting, and image detection, allowing the framework to recognize and track images as they are presented in the environment.
Using ARKit is simpler for developers familiar with Swift. The framework is designed to work seamlessly with existing UIKit and SceneKit technologies, ensuring that developers can leverage their existing knowledge while expanding their skill set into the realm of augmented reality.
Here’s a simple example to show how to set up an AR session using ARKit:
import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() // Set the view's delegate sceneView.delegate = self // Show statistics such as fps and timing information sceneView.showsStatistics = true // Create a new scene let scene = SCNScene() // Set the scene to the view sceneView.scene = scene } override func viewWillAppear(_ animated: Bool) { super.viewWillAppear(animated) // Create a session configuration let configuration = ARWorldTrackingConfiguration() // Run the view's session sceneView.session.run(configuration) } override func viewWillDisappear(_ animated: Bool) { super.viewWillDisappear(animated) // Pause the view's session sceneView.session.pause() } }
This code snippet illustrates the basic setup required to initialize an AR session. By creating an instance of `ARWorldTrackingConfiguration`, the app is ready to interact with the physical world, providing a solid foundation for further development. As developers delve deeper into ARKit, they will find a wealth of features and capabilities at their disposal, allowing for the creation of rich, engaging user experiences.
Setting Up Your First ARKit Project
To get started with ARKit, you first need to set up your iOS project correctly. This entails configuring the project settings, adding the necessary frameworks, and ensuring that your app has the right permissions to access the camera, which very important for AR experiences.
Open Xcode and create a new project. Choose the “Augmented Reality App” template which is specifically designed for AR development. This template includes the essential components for ARKit, such as a basic scene setup and configuration files.
Next, you will need to ensure that your project is configured to use ARKit. In the project settings, navigate to the “General” tab and check that “ARKit” is listed under the “Linked Frameworks and Libraries” section. If not, add it manually by clicking the “+” button and selecting “ARKit.framework”.
Another crucial step is to request permission to use the device’s camera. You can do this by adding a key to your Info.plist file. Open the Info.plist file and add the key NSCameraUsageDescription with a value that explains why your app needs access to the camera. This message will be displayed to users when the app requests permission.
Here is how the Info.plist entry looks:
NSCameraUsageDescription This app requires access to the camera for augmented reality experiences.
After setting up the project configurations, you can begin to build your AR experience. An essential step is to add the ARSCNView to your storyboard or create it programmatically. The ARSCNView is the primary view used for displaying AR content, and it combines the functionalities of ARKit and SceneKit.
Here’s how to add an ARSCNView programmatically in your view controller:
import UIKit import ARKit class ViewController: UIViewController, ARSCNViewDelegate { var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() // Initialize and set up the ARSCNView sceneView = ARSCNView(frame: self.view.frame) sceneView.delegate = self sceneView.showsStatistics = true self.view.addSubview(sceneView) // Create a new scene let scene = SCNScene() sceneView.scene = scene } }
With this setup, you’ll have a basic AR view ready to go. Remember to run your AR session using the appropriate configuration. The most common choice is ARWorldTrackingConfiguration, which provides a comprehensive solution for tracking the device’s position and orientation in real time.
Incorporating AR into your app requires careful attention to detail and understanding the workflows involved in ARKit. As you continue to develop your project, you will find that ARKit provides extensive tools and resources to create highly interactive and engaging augmented reality experiences, ensuring that the barriers between the real and virtual worlds blur seamlessly.
Key Features of ARKit
ARKit comes equipped with an array of powerful features that make it a formidable tool for developers aiming to create immersive augmented reality experiences. Understanding these key features is essential for using the full potential of the framework.
One of the foundational capabilities of ARKit is its world tracking. This feature utilizes the device’s camera and motion sensors to map the environment and track the user’s position and orientation in space. World tracking ensures that the virtual objects remain anchored to the real world, providing a cohesive experience as users move through their environment. The underlying technology involves simultaneous localization and mapping (SLAM), which allows for real-time tracking of the device’s movement while simultaneously mapping the surrounding space.
Another significant feature is plane detection. ARKit can automatically detect flat surfaces—like tables and floors—using computer vision algorithms. After detecting a plane, developers can place virtual objects on it, creating a more realistic interaction. This can be achieved through the ARPlaneAnchor
, which represents a detected horizontal or vertical surface in the user’s environment. Here’s a snippet demonstrating how to implement plane detection:
import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView.delegate = self sceneView.showsStatistics = true let configuration = ARWorldTrackingConfiguration() configuration.planeDetection = [.horizontal, .vertical] sceneView.session.run(configuration) } func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) { guard let planeAnchor = anchor as? ARPlaneAnchor else { return } let planeNode = createPlaneNode(with: planeAnchor) node.addChildNode(planeNode) } private func createPlaneNode(with planeAnchor: ARPlaneAnchor) -> SCNNode { let plane = SCNPlane(width: CGFloat(planeAnchor.extent.x), height: CGFloat(planeAnchor.extent.z)) plane.materials.first?.diffuse.contents = UIColor(white: 1.0, alpha: 0.5) let planeNode = SCNNode(geometry: plane) planeNode.position = SCNVector3(planeAnchor.center.x, 0, planeAnchor.center.z) planeNode.eulerAngles.x = -.pi / 2 return planeNode } }
Next, ARKit includes light estimation, which significantly enhances realism by matching the lighting conditions of virtual objects to those of the real environment. This feature analyzes the ambient light in the user’s surroundings and adjusts the virtual object’s properties, such as color and intensity, dynamically. By enabling light estimation, developers can ensure that their augmented objects integrate seamlessly into various lighting conditions, thus improving the overall user experience.
In addition to these features, ARKit supports image tracking. This functionality allows the framework to recognize and track 2D images in the environment, anchoring virtual content to those images. Developers can create interactive experiences where virtual elements respond to printed materials, such as posters or QR codes. To use image tracking, you must define reference images in your asset catalog and configure the session to use them. Here’s a brief example:
import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() let configuration = ARWorldTrackingConfiguration() if let referenceImages = ARReferenceImage.referenceImages(inGroupNamed: "AR Resources", bundle: nil) { configuration.detectionImages = referenceImages configuration.maximumNumberOfTrackedImages = 1 } sceneView.session.run(configuration) } func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) { guard let imageAnchor = anchor as? ARImageAnchor else { return } let node = createContentNode(for: imageAnchor) node.anchor = imageAnchor self.sceneView.session.add(anchor: node) } private func createContentNode(for imageAnchor: ARImageAnchor) -> SCNNode { let contentNode = SCNNode() // Add virtual objects to the contentNode based on the imageAnchor return contentNode } }
Overall, ARKit’s feature set is expansive and versatile, catering to a wide range of augmented reality applications. From world tracking and plane detection to light estimation and image tracking, the possibilities for creating engaging and interactive AR experiences are virtually limitless. As developers explore these features, they will discover new ways to enhance their applications, pushing the boundaries of what augmented reality can achieve.
Integrating ARKit with SwiftUI
Integrating ARKit with SwiftUI opens up new dimensions for building augmented reality applications with a more declarative approach. While ARKit traditionally relied on UIKit, SwiftUI’s modern syntax and reactivity make it an appealing option for AR development. To leverage the power of both frameworks, developers can create a bridge between SwiftUI and ARKit, enabling them to build visually appealing interfaces that respond to changes in the augmented reality environment.
To begin integrating ARKit with SwiftUI, you can create a custom UIViewControllerRepresentable that wraps ARSCNView, the core view for displaying AR content. This allows you to manage the AR session and present augmented reality experiences within a SwiftUI view hierarchy. Here’s a simple implementation that showcases how to set this up:
import SwiftUI import ARKit struct ARView: UIViewControllerRepresentable { func makeUIViewController(context: Context) -> ARViewController { return ARViewController() } func updateUIViewController(_ uiViewController: ARViewController, context: Context) { // Update the view controller if needed } } class ARViewController: UIViewController, ARSCNViewDelegate { var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView = ARSCNView(frame: self.view.frame) sceneView.delegate = self sceneView.showsStatistics = true self.view.addSubview(sceneView) let configuration = ARWorldTrackingConfiguration() sceneView.session.run(configuration) } override func viewWillDisappear(_ animated: Bool) { super.viewWillDisappear(animated) sceneView.session.pause() } }
With this setup, you now have a reusable AR view component that you can incorporate into your SwiftUI views. To display this AR view, simply use it like any other SwiftUI view:
struct ContentView: View { var body: some View { ARView() .edgesIgnoringSafeArea(.all) // Allow the AR view to fill the entire screen } }
This simpler integration allows developers to encapsulate AR functionality while using SwiftUI’s state-driven design. As a result, you can create dynamic user interfaces that can respond to changes in the AR experience without the overhead of managing UIKit views directly.
Moreover, using SwiftUI’s features, such as bindings and state management, can enhance user interactions within AR environments. For instance, you can create buttons or sliders in SwiftUI that influence the AR scene. To demonstrate, ponder a scenario where tapping a button adds a virtual object to the scene:
struct ContentView: View { @State private var showCube = false var body: some View { ZStack { ARView(showCube: $showCube) .edgesIgnoringSafeArea(.all) VStack { Spacer() Button("Add Cube") { showCube.toggle() } .padding() .background(Color.white) .cornerRadius(10) } } } } class ARViewController: UIViewController, ARSCNViewDelegate { var sceneView: ARSCNView! @Binding var showCube: Bool override func viewDidLoad() { super.viewDidLoad() sceneView = ARSCNView(frame: self.view.frame) sceneView.delegate = self self.view.addSubview(sceneView) let configuration = ARWorldTrackingConfiguration() sceneView.session.run(configuration) // Add cube if showCube is true if showCube { addCube() } } func addCube() { let cube = SCNBox(width: 0.1, height: 0.1, length: 0.1, chamferRadius: 0) let cubeNode = SCNNode(geometry: cube) cubeNode.position = SCNVector3(0, 0, -0.5) // Position in front of the camera sceneView.scene.rootNode.addChildNode(cubeNode) } override func viewWillDisappear(_ animated: Bool) { super.viewWillDisappear(animated) sceneView.session.pause() } }
This pattern allows for a high degree of interactivity, as users can modify the scene with simple button taps. The AR experience remains fluid and responsive to user input, maximizing both engagement and ease of use.
As you continue to explore the integration of ARKit with SwiftUI, you’ll discover that the combination of these frameworks provides rich opportunities to create engaging augmented reality experiences. With SwiftUI’s declarative syntax and efficient state management, developers can focus on crafting compelling user experiences while ARKit handles the underlying complexities of augmented reality.
Best Practices for AR Development
When developing augmented reality (AR) applications using ARKit, adhering to best practices very important for ensuring a seamless and engaging user experience. These practices can greatly enhance performance, usability, and overall application quality. Below are some key considerations that developers should keep in mind.
1. Optimize Performance
AR applications can be resource-intensive, so optimizing performance is essential. Make use of ARKit’s built-in capabilities, such as occlusion and light estimation. Occlusion allows virtual objects to be blocked by real-world objects, creating a more realistic experience. Implementing light estimation helps virtual objects blend better with the environment by adjusting their appearance based on ambient lighting conditions.
Moreover, ponder minimizing the number of active nodes in the scene. Keeping the node hierarchy simple can lead to better performance. Use SCNNode
efficiently and remove any nodes that are no longer needed.
func removeUnnecessaryNodes() { for node in sceneView.scene.rootNode.childNodes { if !node.isVisible { node.removeFromParentNode() } } }
2. Handle User Interactions Thoughtfully
AR applications should offer intuitive and responsive interactions. Ensure that any gestures or touch events are handled gracefully. Consider using gestures like tap, pinch, and pan to interact with virtual objects. Swift’s UIGestureRecognizer
provides an easy way to add these interactions.
let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap(_:))) sceneView.addGestureRecognizer(tapGesture) @objc func handleTap(_ gesture: UITapGestureRecognizer) { let location = gesture.location(in: sceneView) let hitTestResults = sceneView.hitTest(location, options: nil) if let result = hitTestResults.first { // Interact with the tapped node handleTappedNode(result.node) } }
3. Provide User Feedback
Feedback is vital in AR applications. Users should receive clear cues about their interactions or when they need to take actions, such as moving closer to a detected object. Visual indicators or haptic feedback can enhance user experience significantly.
func provideFeedback(for node: SCNNode) { // Example of feedback: change color of the node node.geometry?.firstMaterial?.diffuse.contents = UIColor.red // Optional: Add haptic feedback let impactFeedbackGenerator = UIImpactFeedbackGenerator(style: .medium) impactFeedbackGenerator.impactOccurred() }
4. Test in Various Environments
Testing your AR application in diverse environments allows you to identify issues related to lighting, surfaces, and tracking. Different conditions can greatly affect AR performance. Be prepared to iterate based on feedback from real-world usage, ensuring the app performs well in varied conditions.
5. Keep Usability in Mind
Be mindful of the user experience. Excessive AR content or overly complex interactions can overwhelm users. Instead, aim for clarity and simplicity. Regularly check that the app is enjoyable and simple to operate, focusing on the primary use case you intend to solve.
Ensure your application provides a clear onboarding experience to guide users through the AR functionalities. A simple tutorial or pop-up messages can help familiarize users with interactions and settings.
6. Stay Updated with ARKit Enhancements
ARKit is continuously evolving, with Apple regularly releasing updates that introduce new features and improvements. Stay informed about the latest changes to leverage new capabilities and enhance your applications. Regularly check the official documentation and engage with the developer community to learn best practices and innovative techniques.
By implementing these best practices in your ARKit development, you will create more optimized, engaging, and user-friendly experiences. Emphasizing performance, usability, and user feedback will be instrumental in the success of your augmented reality applications.
Future Trends in ARKit and Swift Development
The future of ARKit and Swift development is poised for exciting advancements, driven by the rapid evolution of technology and increasing consumer interest in augmented reality applications. As developers continue to explore the capabilities of ARKit, several emerging trends and enhancements are likely to shape the landscape of AR development.
One notable trend is the integration of machine learning and AI technologies into AR applications. Apple’s Core ML framework allows developers to leverage pre-trained models to improve AR experiences. By combining ARKit with machine learning, developers can create applications that recognize objects, understand the user’s context, and personalize experiences in real-time. For example, imagine an AR app that not only places virtual furniture in a user’s home but also analyzes the room layout to suggest optimal arrangements, adapting to user preferences as they interact.
Additionally, as AR devices become more sophisticated, we can expect improvements in hardware capabilities that will enhance AR experiences. With the introduction of mixed reality headsets and advanced mobile devices, ARKit is likely to expand its functionality to support features like hand tracking, eye tracking, and spatial audio. This will enable developers to create even more immersive environments where users can interact with virtual objects in a more natural and intuitive way.
Another area of growth is collaborative AR experiences. As more users adopt AR technologies, the demand for shared augmented reality experiences will increase. ARKit is already laying the groundwork for this through features such as ARKit’s collaboration session, which allows multiple users to share an AR environment and interact with the same virtual content. Developers will need to think creatively about how to design shared experiences that promote interaction and engagement among users. For example, implementing multiplayer games or collaborative design tools could become increasingly popular.
Moreover, the rise of 5G technology is set to revolutionize the way AR applications function. With faster download speeds and lower latency, developers can build richer, more complex AR experiences that rely on cloud computing for processing and rendering. This opens the door for AR applications that can stream high-quality graphics and real-time data, allowing for seamless interactions without the limitations of local device processing power. Imagine an AR navigation app that overlays real-time traffic data on the user’s surroundings, adapting the route dynamically based on current conditions.
As ARKit evolves, it will also benefit from improved tools and resources that make development easier and more efficient. New features, such as improved environmental understanding and enhanced support for 3D content creation, will allow developers to create more intricate and engaging AR applications. Enhanced debugging and performance tools will also empower developers to optimize their applications, ensuring a smoother user experience.
Lastly, as AR technology becomes more mainstream, ethical considerations will come to the forefront of AR development. Developers will need to address concerns related to privacy, data security, and user consent. Transparent data handling practices and user education about AR functionalities will be critical to gaining user trust and ensuring that augmented reality remains a positive addition to everyday life.
The future of ARKit and Swift development is bright, marked by the integration of emerging technologies, enhanced hardware capabilities, and collaborative experiences. By staying on the cutting edge of these trends and adhering to best practices, developers can create innovative applications that push the boundaries of what augmented reality can achieve, ultimately transforming the way users interact with the world around them.