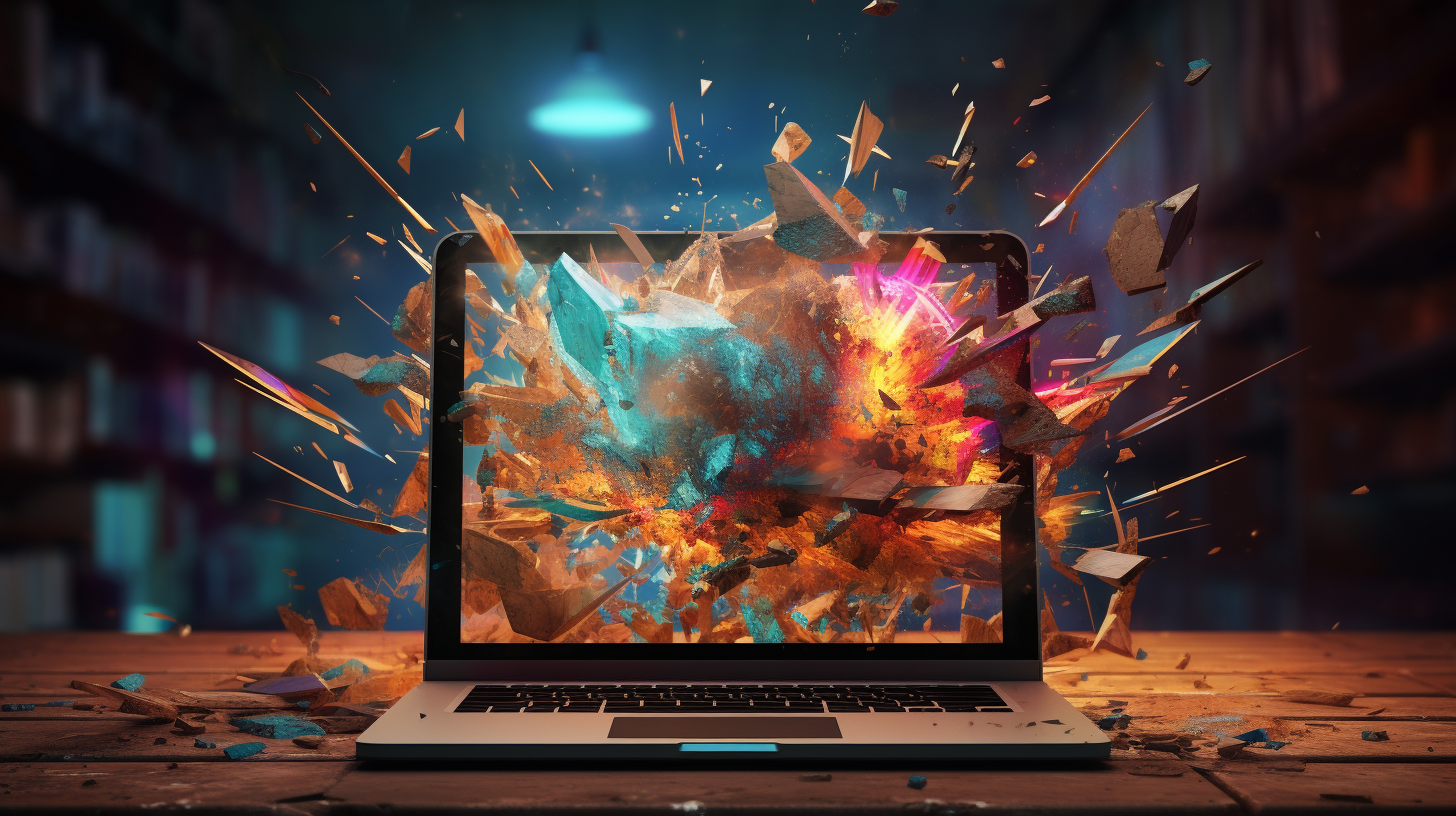
Java and Agile Development: Practices and Methodologies
Agile development is a philosophy that emphasizes flexibility, collaboration, and customer satisfaction. At its core, Agile is about adapting to change rather than strictly following a predefined plan. That is particularly relevant in the fast-paced world of software development, where requirements can shift dramatically between the initial concept and final delivery. Agile principles encourage teams to work iteratively and incrementally, allowing for frequent reassessment and adaptation of plans.
One of the foundational documents of Agile is the Agile Manifesto, which outlines four fundamental values:
- Individuals and interactions over processes and tools – This value emphasizes the importance of teamwork and communication. Developers, testers, and stakeholders are encouraged to collaborate closely to ensure that everyone is aligned and that any issues are addressed promptly.
- Working software over comprehensive documentation – Agile prioritizes delivering functional software. While documentation is important, the focus is on creating a product that meets user needs rather than getting bogged down in excessive documentation.
- Customer collaboration over contract negotiation – Agile methodologies encourage ongoing engagement with customers. This means soliciting feedback regularly and making adjustments based on user input, rather than adhering to a strict contract that may not reflect the customer’s evolving needs.
- Responding to change over following a plan – Agile teams are encouraged to be flexible. If new requirements emerge or changes are needed, teams can pivot and adjust their focus, ensuring that the final product remains relevant and useful.
In addition to these values, Agile is guided by 12 principles that further define its approach. Some notable principles include:
- Customer satisfaction through early and continuous delivery – By delivering small, functional increments of software regularly, teams can keep customers happy while minimizing the risk of delivering a product that doesn’t meet their needs.
- Emphasis on face-to-face communication – Agile values direct communication, which can lead to faster problem-solving and greater clarity among team members.
- Self-organizing teams – Agile promotes the idea that the best solutions come from teams that are empowered to make decisions rather than relying on top-down directives.
- Sustainable development – Agile encourages teams to maintain a constant pace that can be sustained indefinitely, preventing burnout and allowing for consistent productivity.
For Java developers, understanding and applying these Agile principles can significantly enhance the development process. For instance, a Java team might employ Agile practices by using frameworks like Spring and JUnit, which support iterative development and testing. Below is a simple example of how a Java class might be structured in an Agile context:
public class User { private String name; private String email; public User(String name, String email) { this.name = name; this.email = email; } // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
This simple class demonstrates a foundational piece of functionality that could be built upon iteratively. As user feedback is gathered, additional features or modifications can be implemented, showcasing the Agile principle of responding to change.
By embracing these Agile development principles, Java teams can create a dynamic environment where continuous improvement and collaboration thrive, ultimately leading to better software solutions that meet user needs effectively.
Key Agile Methodologies in Java Development
In the context of Agile development tailored for Java, several methodologies stand out, each offering unique frameworks and practices that cater to different project requirements and team dynamics. Among these, Scrum and Kanban are widely adopted, serving the dual purpose of enhancing collaboration and streamlining workflows.
Scrum is a popular Agile framework characterized by its structured approach to iterative development. In Scrum, work is divided into time-boxed iterations called sprints, typically lasting two to four weeks. During each sprint, a cross-functional team collaborates to deliver a potentially shippable product increment. The Scrum process is supported by specific roles, ceremonies, and artifacts that help maintain focus and accountability:
- Key roles include the Scrum Master, who facilitates the process and removes impediments, the product Owner, who represents the stakeholders and prioritizes this product backlog, and the Development Team, which is responsible for delivering the work.
- Scrum involves regular ceremonies such as Sprint Planning, Daily Stand-ups, Sprint Reviews, and Sprint Retrospectives, fostering transparency and continuous improvement.
- Artifacts like the product Backlog and Sprint Backlog help teams manage and visualize their work effectively.
Here’s a simple example illustrating how a Scrum team might use a Java class to represent a task in a Sprint:
public class Task { private String description; private boolean isCompleted; public Task(String description) { this.description = description; this.isCompleted = false; } public void completeTask() { this.isCompleted = true; } public boolean isCompleted() { return isCompleted; } public String getDescription() { return description; } }
This Task
class exemplifies a simpler representation of work items within a Scrum framework. The team can iteratively add tasks to their Sprint Backlog, track their progress, and manage completion states, aligning perfectly with Agile practices.
Kanban, on the other hand, offers a more fluid approach to Agile development. Unlike Scrum, Kanban does not rely on fixed iterations; instead, it emphasizes continuous delivery and flow. Key aspects of Kanban include:
- Kanban boards are used to visualize work, allowing teams to track progress and identify bottlenecks at a glance.
- By limiting the number of tasks that can be in progress at any one time, Kanban encourages teams to focus on completing tasks before starting new ones, enhancing efficiency.
- Kanban fosters a culture of continuous improvement by encouraging teams to analyze their workflow and make adjustments as needed.
Here’s an example of how a simple Kanban board might be represented using Java classes:
import java.util.ArrayList; import java.util.List; public class KanbanBoard { private List todo; private List inProgress; private List done; public KanbanBoard() { this.todo = new ArrayList(); this.inProgress = new ArrayList(); this.done = new ArrayList(); } public void addTask(Task task) { todo.add(task); } public void moveToInProgress(Task task) { todo.remove(task); inProgress.add(task); } public void moveToDone(Task task) { inProgress.remove(task); done.add(task); } }
In this KanbanBoard
class, tasks can be categorized into different states, allowing teams to monitor workflow effectively. This flexibility aligns well with Agile’s emphasis on adaptability and responsiveness.
Both Scrum and Kanban provide robust frameworks that facilitate Agile practices within Java development teams. By understanding and implementing these methodologies, teams can enhance their collaboration, improve productivity, and ultimately deliver software that meets the dynamic needs of users.
Best Practices for Java Agile Teams
Best practices for Java Agile teams revolve around fostering an environment that enhances collaboration, promotes sustainable development, and ensures the delivery of high-quality software. These practices aim to maximize the efficiency of Agile methodologies while adapting them to the unique demands of Java development.
Coding Standards and Best Practices
Maintaining high coding standards is paramount in Agile environments. Java teams should adopt conventions such as the Google Java Style Guide or the Oracle Code Conventions to ensure consistent coding practices across the board. This consistency not only facilitates better collaboration among team members but also simplifies code reviews and enhances maintainability.
public class User { private String name; private String email; // Constructor public User(String name, String email) { this.name = name; this.email = email; } // Getters public String getName() { return name; } public String getEmail() { return email; } // Setters public void setName(String name) { this.name = name; } public void setEmail(String email) { this.email = email; } }
This class example showcases adherence to standard coding practices with clear getters and setters, a well-defined constructor, and appropriate naming conventions, which contribute to clear and maintainable code.
Test-Driven Development (TDD)
Incorporating Test-Driven Development (TDD) into the Agile process can significantly enhance code quality. TDD encourages developers to write tests before coding the functionality, which leads to better-designed, more reliable software. Java developers can utilize frameworks such as JUnit to facilitate TDD practices.
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; public class UserTest { @Test public void testUserCreation() { User user = new User("Alice", "[email protected]"); assertEquals("Alice", user.getName()); assertEquals("[email protected]", user.getEmail()); } @Test public void testSetName() { User user = new User("Alice", "[email protected]"); user.setName("Bob"); assertEquals("Bob", user.getName()); } }
These test cases demonstrate how TDD can help catch issues early, ensuring that the code behaves as expected right from the start. This proactive approach saves time and resources in the long run.
Continuous Integration and Continuous Deployment (CI/CD)
Implementing CI/CD practices is another key best practice for Agile teams. By setting up automated build and deployment pipelines, Java teams can ensure that code changes are integrated frequently and that software is deployed rapidly and reliably. Tools like Jenkins, GitLab CI, and CircleCI can be employed to automate these processes, allowing teams to focus on writing code instead of managing deployments.
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } stage('Deploy') { steps { sh 'docker build -t myapp .' sh 'docker run -d -p 8080:8080 myapp' } } } }
This example defines a simple CI/CD pipeline using a declarative syntax, illustrating how to automate the build, testing, and deployment processes effectively.
Regular Retrospectives
Conducting regular retrospectives at the end of each sprint very important for continuous improvement. These meetings provide a platform for team members to reflect on what went well, what could be improved, and how to implement changes moving forward. This practice ensures that the team remains adaptive and continuously enhances their processes.
By embracing these best practices, Java Agile teams can create a robust framework that fosters collaboration, enhances code quality, and promotes a culture of continuous improvement. Through the effective application of these methodologies, teams can navigate the complexities of software development while delivering high-quality products that meet user needs.
Tools and Frameworks Supporting Agile Java Development
Within the scope of Agile development, selecting appropriate tools and frameworks can significantly enhance a Java team’s efficiency and capability to deliver high-quality software. These tools not only facilitate the Agile processes but also streamline collaboration and integration between team members. Below, we explore essential tools and frameworks that support Agile Java development.
Version Control Systems
One of the cornerstones of Agile practices is effective version control. Tools like Git, along with platforms such as GitHub, GitLab, and Bitbucket, provide teams with the ability to collaborate on code seamlessly. These platforms support branching strategies, allowing multiple team members to work on features or bug fixes simultaneously without stepping on each other’s toes.
git checkout -b new-feature git add . git commit -m "Implement new feature" git push origin new-feature
Using pull requests in these platforms fosters code reviews, ensuring quality before integration into the main codebase. This Agile practice helps maintain high standards while also encouraging peer feedback and collaboration.
Project Management Tools
Managing tasks and tracking progress is critical in Agile environments. Tools like Jira, Trello, and Asana facilitate the organization of tasks through simple to operate interfaces. These tools support the Scrum framework by allowing teams to create product backlogs, manage sprint planning, and visualize workflows through Kanban boards.
// Simple representation of a Kanban task public class KanbanTask { private String title; private String status; // TODO, IN_PROGRESS, DONE public KanbanTask(String title) { this.title = title; this.status = "TODO"; } public void startTask() { this.status = "IN_PROGRESS"; } public void completeTask() { this.status = "DONE"; } // Getters public String getTitle() { return title; } public String getStatus() { return status; } }
This simple KanbanTask class exemplifies how tasks can be represented programmatically, allowing teams to create, update, and track the status of their work items efficiently.
Testing Frameworks
Ensuring code quality is paramount in Agile development. Testing frameworks such as JUnit and Mockito are invaluable for Java developers, enabling them to write unit tests and perform mocking easily. This practice aligns with Test-Driven Development (TDD), promoting writing tests first before the actual implementation.
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; public class KanbanTaskTest { @Test public void testTaskInitialState() { KanbanTask task = new KanbanTask("Implement feature"); assertEquals("TODO", task.getStatus()); } @Test public void testTaskCompletion() { KanbanTask task = new KanbanTask("Implement feature"); task.startTask(); assertEquals("IN_PROGRESS", task.getStatus()); task.completeTask(); assertEquals("DONE", task.getStatus()); } }
These tests ensure that the KanbanTask class operates as intended, providing confidence in the code’s reliability as it evolves.
Continuous Integration/Continuous Deployment (CI/CD) Tools
CI/CD practices are integral to Agile development, enabling teams to automate their build and deployment processes. Tools like Jenkins, CircleCI, and Travis CI allow Java developers to automatically build their applications, run tests, and deploy them to production environments. This not only speeds up delivery but also enhances code quality through automated testing and verification.
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } stage('Deploy') { steps { sh 'docker-compose up -d' } } } }
This Jenkins pipeline example illustrates how to automate the build, test, and deployment processes, allowing teams to deploy new features rapidly while maintaining high standards for quality assurance.
Collaboration and Communication Tools
Effective communication is vital in Agile teams. Tools like Slack, Microsoft Teams, and Zoom provide platforms for real-time chat and video conferencing, fostering interaction among team members, especially in distributed environments. These tools enable quick discussions, allowing teams to make decisions and solve problems swiftly, which is aligned with Agile principles of frequent communication.
Using the right tools and frameworks in Agile Java development can drastically improve efficiency, collaboration, and code quality. By integrating these technologies into their workflow, teams can create a robust environment that supports iterative development and rapid delivery, ultimately enhancing their ability to meet user needs effectively.
Case Studies: Successful Java Agile Implementations
Real-world implementations of Agile methodologies in Java development showcase the flexibility and adaptability of Agile practices, leading to successful project outcomes across various industries. These case studies highlight how teams effectively integrated Agile principles into their Java projects, illustrating the tangible benefits of Agile development.
One compelling case is that of a global financial services company that sought to modernize its legacy applications. The organization had been facing challenges related to slow release cycles and difficulty in responding to market changes. By transitioning to Agile methodologies, the Java development team adopted Scrum as their framework, allowing them to work in iterative sprints.
During the initial phase, the team established a dedicated cross-functional group consisting of developers, testers, and business analysts. This group conducted sprint planning sessions to define deliverables, ensuring that they aligned with stakeholder expectations. A key aspect was their focus on frequent iterations, delivering working software every two weeks. This approach enabled them to gather user feedback early and often, significantly improving their product’s relevance and user satisfaction.
They utilized Java technologies such as Spring Boot for rapid application development and JUnit for test-driven development, which ensured a high level of code quality. The team maintained a robust suite of automated tests that ran during the Continuous Integration (CI) process, catching issues before they reached production. This led to a reduction in defects and increased confidence among team members when deploying new features.
public class PaymentService { public String processPayment(Payment payment) { // Business logic to process payment return "Payment processed for " + payment.getAmount(); } }
This PaymentService class exemplifies Agile’s focus on functional delivery, where each feature is developed to meet specific user needs. Each sprint focused on adding new payment methods, enhancing security, and improving transaction speed based on user feedback.
Another inspiring case is from a tech startup that aimed to create a mobile application for managing personal finances. The small team adopted Kanban principles, visually managing their workflow with a Kanban board. This allowed them to prioritize tasks based on user stories and continuously deliver valuable features.
Using Java for back-end development and integrating it with a responsive front-end framework, the team was able to quickly implement changes as they received user feedback. They faced challenges with feature prioritization, so they held regular stand-up meetings to facilitate communication and make real-time adjustments to their development focus.
public class Budget { private String category; private double limit; // Constructor, getters, and setters public Budget(String category, double limit) { this.category = category; this.limit = limit; } }
The Budget class represents a simple yet effective way to manage user financial data, allowing for easy enhancements as the application evolved. The team leveraged feedback from early adopters to refine their user interface and enhance the application’s usability.
Both case studies show how Agile practices can be tailored to meet the specific needs of a project. By employing iterative development, continuous feedback, and a focus on user satisfaction, these Java teams successfully navigated challenges, delivered high-quality software, and achieved significant improvements in productivity. The key takeaway is that Agile methodologies, when correctly implemented, can transform the software development landscape, making teams more responsive and capable of delivering exceptional products.