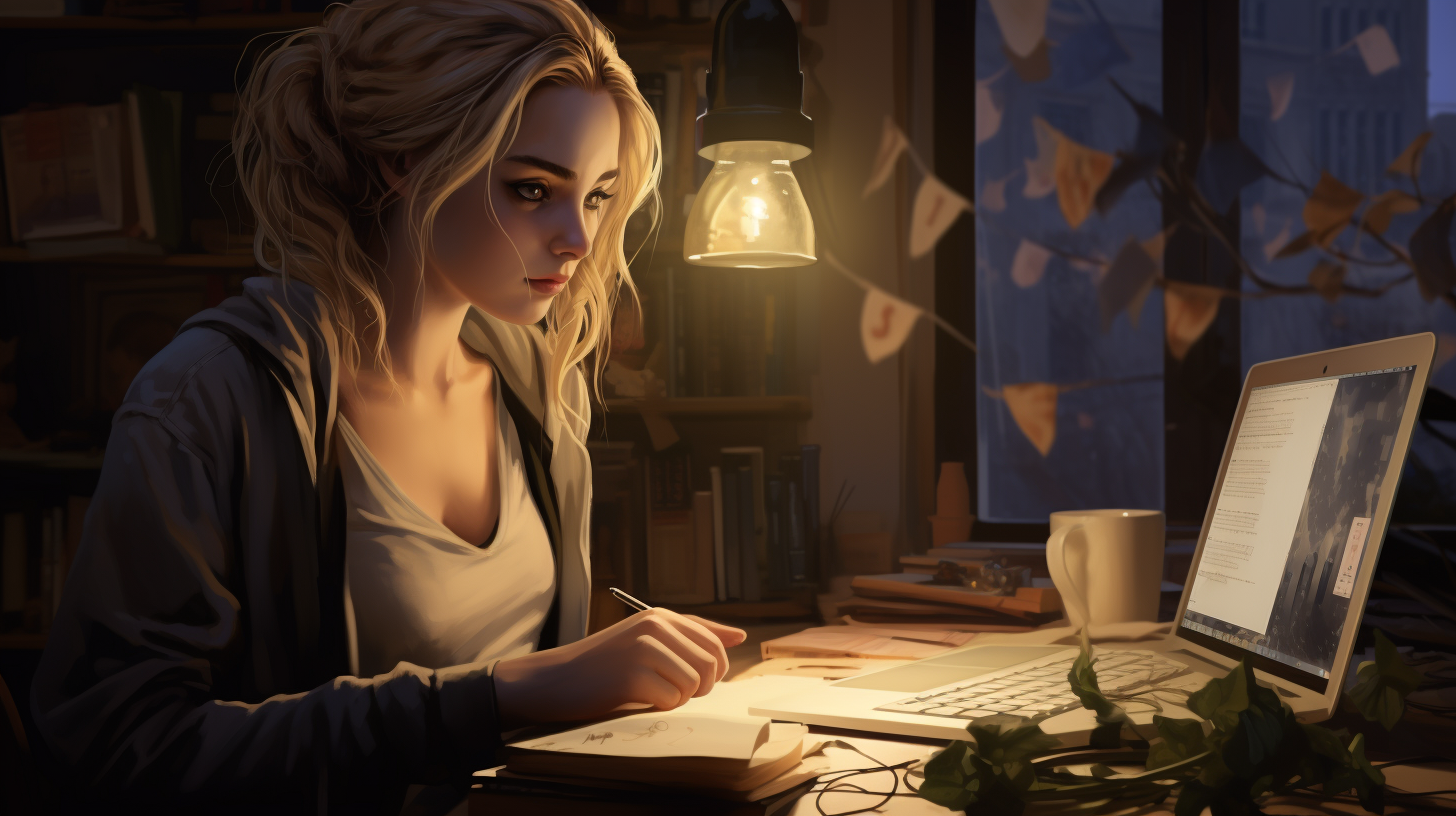
Bash Error Handling Strategies
When working with Bash scripts, it’s crucial to understand the common types of errors that can arise. These errors generally stem from various sources, including syntax mistakes, runtime issues, and command failures. Recognizing these errors is the first step in effective error handling.
1. Syntax Errors: These occur when the Bash interpreter encounters a command that it cannot parse. Syntax errors can be as simple as a missing quotation mark or an incorrectly placed semicolon. These errors will typically prevent the script from running altogether.
# Example of a syntax error echo "Hello World
In this case, the missing closing quotation mark will prompt an error message, indicating that the command was not understood.
2. Command Not Found: This type of error arises when a script tries to execute a command that is not available in the current environment. This could occur due to a typo or an uninstalled package.
# Example of command not found non_existent_command
When this happens, Bash will return an error stating that the command could not be found, causing the script to halt.
3. File Not Found: This common error appears when a script attempts to access a file or directory that does not exist. This can happen for several reasons, such as incorrect paths or missing files.
# Example of file not found error cat nonexistent_file.txt
In this scenario, Bash will output an error message indicating that the specified file could not be accessed.
4. Permission Denied: Attempting to execute a file or access a directory without the necessary permissions triggers this error. It can occur when trying to run a script without executable permissions or accessing a file without read permissions.
# Example of permission denied error ./protected_script.sh
Bash will respond with a message stating that permission was denied, preventing the action from taking place.
5. Runtime Errors: These occur during the execution of a script, often due to logical errors or conditions that were not anticipated. An example would be attempting to divide by zero.
# Example of a runtime error result=$((10 / 0))
This code will lead to a runtime error, as division by zero is mathematically undefined.
Understanding these common error types is essential for developing more resilient scripts. By anticipating potential issues and crafting appropriate error handling strategies, you can significantly enhance the reliability and robustness of your Bash scripts.
Exit Status Codes and Their Interpretation
In Bash, every command returns an exit status upon completion, which provides valuable information about its execution. This exit status is an integer value, where a status of `0` indicates success and any non-zero value indicates an error. The interpretation of these exit codes is fundamental to building scripts that can handle failures gracefully.
The exit status can be accessed immediately after a command is executed using the special variable $?
. This allows you to check whether the preceding command ran successfully or if it encountered an error.
command if [ $? -eq 0 ]; then echo "Command executed successfully." else echo "Command failed with exit status: $?" fi
Understanding common exit codes can help in diagnosing issues within your scripts. For instance, an exit status of `1` typically indicates a general error, while `2` denotes a misuse of shell builtins. Other common exit codes are:
- Command not found, indicating that the specified command couldn’t be located in the PATH.
- Command invoked cannot execute, which occurs when a command is found but is not executable.
- Script terminated by Ctrl+C, which comes into play when a user interrupts a running script.
- Exit status out of range, used to indicate that an exit status has exceeded the allowable range (0-255).
By checking the exit status after executing commands, you can implement conditional logic to handle errors effectively. This technique allows for the creation of more reliable scripts that can adapt to various operational contexts.
# Example script demonstrating exit status checks #!/bin/bash command1 if [ $? -ne 0 ]; then echo "Command1 failed. Exiting." exit 1 fi command2 if [ $? -ne 0 ]; then echo "Command2 failed. Exiting." exit 2 fi echo "Both commands executed successfully."
In this example, if either command1
or command2
fails, the script will output an error message and exit with a specific exit code. This practice not only helps in identifying issues but also aids in debugging scripts efficiently.
Incorporating these exit status checks into your Bash scripts is a best practice that can transform a simple script into a professional-grade program capable of handling errors in a controlled manner. This way, you can ensure that issues are promptly addressed without causing unintended consequences in larger systems.
Using Trap for Error Handling
In Bash, the trap command serves as a powerful tool for managing errors and improving script reliability. When you want to handle signals or specific exit conditions, trap allows you to define custom actions that should be executed when a script exits or when specific signals are received. This can be invaluable for cleaning up resources, logging errors, or performing specific tasks in response to failures.
To utilize trap, you first specify the command or commands you want Bash to execute upon receiving a particular signal or when exiting a script. The syntax is straightforward:
trap 'commands' SIGNAL
For instance, if you want to ensure that a temporary file is deleted when your script exits, you can set up a trap for the EXIT signal:
tempfile=$(mktemp) trap 'rm -f "$tempfile"' EXIT
In this example, the trap command tells Bash to execute rm -f "$tempfile"
when the script exits, regardless of whether it exits normally or due to an error. This practice ensures that temporary files do not persist after the script has run, maintaining a clean environment.
Moreover, trap can also capture signals like SIGINT
(triggered by Ctrl+C) or SIGTERM
(sent by the kill command). By capturing these signals, you can gracefully handle interruptions. For example, you can provide users with a message and perform cleanup actions before the script terminates:
trap 'echo "Script interrupted. Cleaning up..."; exit' SIGINT
Using this trap, if a user interrupts the script, it will output a friendly message and perform any necessary cleanup operations before exiting.
Another common application of trap is to log errors when a script fails. By capturing the EXIT signal, you can log the exit status of the script and any relevant information:
trap 'echo "Script exited with status $?" >> error.log' EXIT
This way, if your script fails at any point, you’ll have a record of the exit status saved in error.log
, which can be incredibly useful for debugging.
Combining the use of trap with exit status checks creates a robust error handling mechanism. Here’s how you might implement it in a script:
#!/bin/bash tempfile=$(mktemp) trap 'rm -f "$tempfile"; echo "Script exited with status $?"; exit' EXIT SIGINT echo "Creating a temporary file at $tempfile" # Simulating some work sleep 2 # Simulating a command that could fail if ! cp some_file.txt "$tempfile"; then echo "Error: Failed to copy file." exit 1 fi echo "File copied successfully!"
In this script, the trap command ensures that the temporary file is deleted and the exit status is logged whether the script completes successfully, encounters an error, or is interrupted.
By using the trap command, you significantly enhance your script’s ability to handle unexpected situations gracefully. This not only aids in maintaining system cleanliness but also provides valuable feedback during troubleshooting, making it an essential tool for any Bash programmer aiming to build reliable scripts.
Best Practices for Robust Bash Scripts
When it comes to writing robust Bash scripts, adhering to best practices is paramount. These practices not only enhance the reliability of your scripts but also make them easier to debug and maintain. Here are several key strategies to consider:
1. Use Clear and Descriptive Variable Names:
Choosing meaningful variable names can vastly improve the readability of your scripts. Instead of using ambiguous names like `a` or `var1`, opt for descriptive names that indicate the purpose of the variable.
filename="user_data.txt" user_count=0
In this example, `filename` and `user_count` make it immediately clear what these variables represent, which aids future maintainability.
2. Validate Input:
Always check for valid input before proceeding with script execution. This can help prevent runtime errors and ensure the script behaves as expected.
if [[ ! -f $filename ]]; then echo "Error: File '$filename' not found." exit 1 fi
In this snippet, the script checks whether the specified file exists before attempting to access it, promptly terminating if it does not.
3. Use Functions for Modularity:
Breaking your script into functions can significantly enhance its organization and reusability. Functions can encapsulate related commands, making it easier to manage your code.
function process_file { local file=$1 echo "Processing file: $file" # Additional processing commands go here } process_file "user_data.txt"
By defining a function for processing files, you can easily reuse this code without duplicating logic throughout your script.
4. Implement Error Handling:
As discussed previously, implementing robust error handling is essential. Using exit status checks and the trap command can help you respond to errors effectively.
command1 || { echo "command1 failed"; exit 1; } command2 || { echo "command2 failed"; exit 2; }
This method ensures that your script gracefully exits whenever a command fails, providing meaningful feedback to the user.
5. Comment Your Code:
Adding comments throughout your script can provide context and explanations for complex logic. That’s particularly useful for anyone who may read or modify your script in the future, including your future self.
# Check if the specified file exists if [[ ! -f $filename ]]; then echo "Error: File '$filename' not found." exit 1 fi
6. Test Your Scripts:
Before deploying scripts in a production environment, it’s prudent to test them in different scenarios. This helps you identify potential bugs and ensures that your error handling works as intended.
bash -n your_script.sh # Syntax check bash your_script.sh # Run the script
7. Use ShellCheck:
Ponder using tools like ShellCheck, a static analysis tool for shell scripts. It helps identify common mistakes and provides warnings about potential issues in your scripts.
shellcheck your_script.sh
By adopting these best practices, you can transform your Bash scripts into reliable, maintainable, and efficient programs. As you progress in your Bash programming journey, keep refining your skills and approaches to script development.