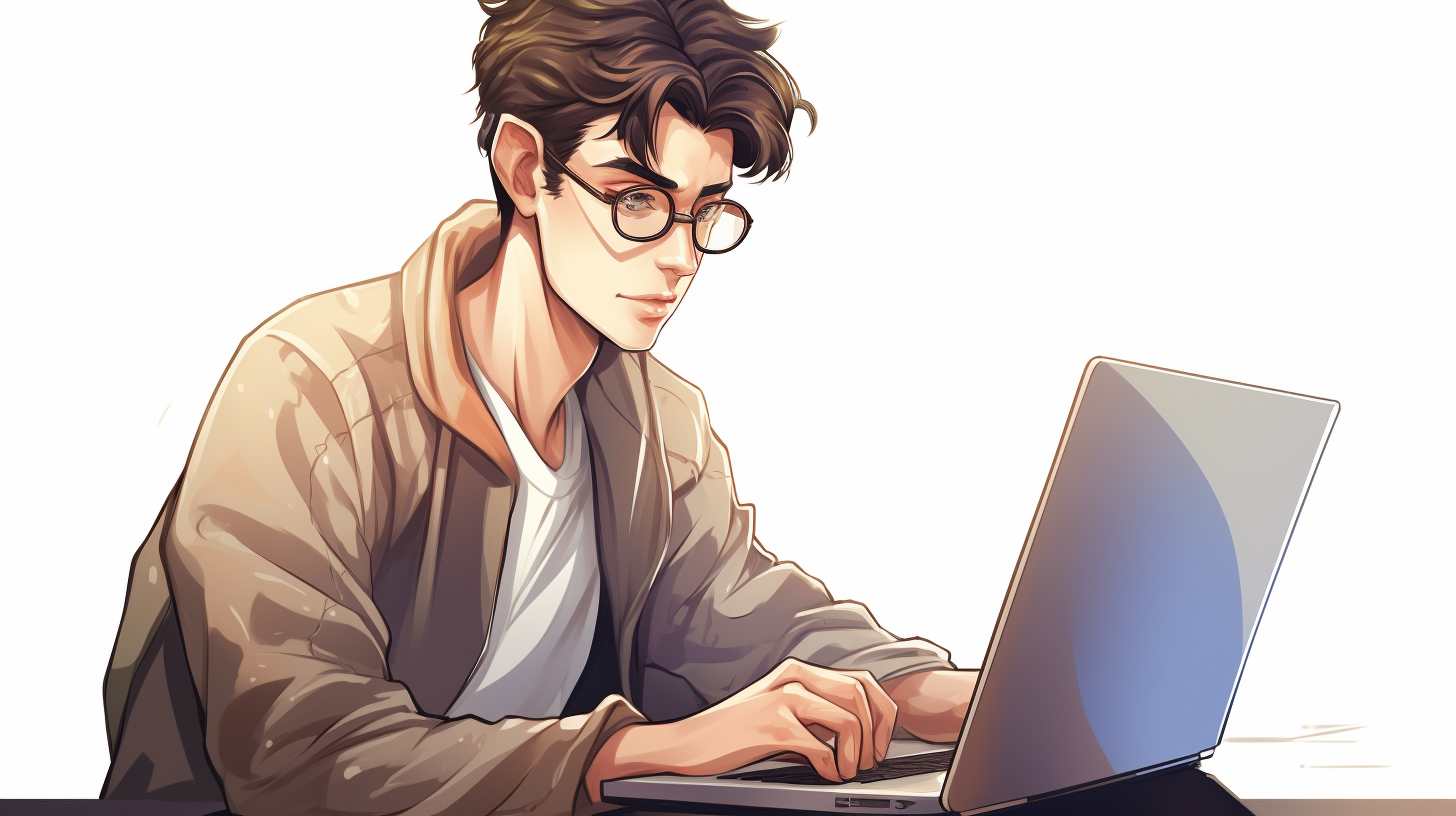
Java and RESTful API Development
In the sphere of software development, understanding the principles of RESTful APIs very important, particularly when working with Java. REST, or Representational State Transfer, is an architectural style that defines a set of constraints for creating web services. RESTful APIs leverage these principles to enable seamless communication between client and server, often through HTTP.
A RESTful API is centered around resources, which can be any type of object, data, or service that can be accessed via a unique URI (Uniform Resource Identifier). Each resource can be manipulated using standard HTTP methods: GET for retrieving data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources.
To illustrate this, think the following example. Suppose we have a resource representing a user, which can be accessed at the URI /users/{id}
. A typical RESTful interaction with this resource might look like the following:
// Example of a RESTful API interaction in Java using HttpURLConnection import java.io.*; import java.net.HttpURLConnection; import java.net.URL; public class RestClient { private static final String USER_SERVICE_URL = "http://example.com/users/"; public static void main(String[] args) throws IOException { // Retrieve user with ID 1 String response = sendGetRequest(1); System.out.println("Response: " + response); } private static String sendGetRequest(int userId) throws IOException { URL url = new URL(USER_SERVICE_URL + userId); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream())); String inputLine; StringBuilder response = new StringBuilder(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); return response.toString(); } }
In this snippet, a simple HTTP GET request retrieves user data from a specified endpoint. Understanding the underlying mechanics of such requests is integral to working effectively with RESTful APIs. Each endpoint not only serves a purpose but also adheres to the principles of statelessness, where each request from the client contains all the information needed for the server to fulfill that request.
Moreover, RESTful APIs are inherently designed to be stateless. This means that each request is independent, and the server does not store any context about the client between requests. This design principle enhances scalability and simplifies server design, allowing for an efficient handling of concurrent requests.
In addition to being stateless, RESTful APIs often return data in a format that’s easy to work with, such as JSON or XML. Java provides libraries like Jackson and Gson to facilitate the conversion between Java objects and JSON, making it simpler to serialize and deserialize API responses.
// Using Jackson to convert a Java object to JSON import com.fasterxml.jackson.databind.ObjectMapper; public class User { private int id; private String name; // Getters and Setters } public class JsonExample { public static void main(String[] args) throws IOException { User user = new User(); user.setId(1); user.setName("Neil Hamilton"); ObjectMapper objectMapper = new ObjectMapper(); String jsonString = objectMapper.writeValueAsString(user); System.out.println("JSON Output: " + jsonString); } }
This example demonstrates how to convert a Java object into a JSON string using Jackson. This capability is particularly valuable when interacting with RESTful services, as it allows developers to easily serialize Java objects for transmission over the network.
In understanding RESTful APIs, it’s also essential to think the role of status codes in HTTP responses. These codes provide insights into the outcome of requests, indicating success, failure, or redirection. For instance, a successful resource retrieval might result in a 200 OK response, while an attempt to access a non-existent resource might yield a 404 Not Found status.
In sum, a solid grasp of RESTful API concepts is foundational for any Java developer aiming to create robust and scalable web services. By using the principles of REST, alongside powerful libraries and tools available in the Java ecosystem, developers can build APIs that are both efficient and simple to operate.
Setting Up a Java Project for RESTful Development
Setting up a Java project for RESTful development involves several steps aimed at creating a structured environment that accommodates the design and implementation of RESTful services. The architecture of your project will largely depend on the frameworks and libraries you choose to use. In the Java ecosystem, there are various frameworks available, with Spring Boot being one of the most popular due to its usability and extensive support for building web applications.
To begin, it’s essential to have a development environment ready. For Java development, you will typically need the Java Development Kit (JDK) installed, along with a suitable Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse. Once your environment is set, you can initiate a new Maven or Gradle project.
Using Maven to Set Up Your Project
Maven is a widely used project management tool in the Java ecosystem. It helps manage project dependencies and provides build lifecycle management. To create a new Maven project, you can run the following command in your terminal:
mvn archetype:generate -DgroupId=com.example -DartifactId=restful-api -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command sets up a new Maven project with the specified group ID and artifact ID. The next step is to update the pom.xml file to include the dependencies necessary for RESTful development. Below is an example of how to include Spring Boot and related dependencies:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
These dependencies include the core Spring Boot web starter, which provides the necessary libraries to build web applications, and Jackson, which is used for JSON processing.
Creating the Application Class
Every Spring Boot application requires a main class annotated with @SpringBootApplication. This class serves as the entry point for your application. Here’s an example of a basic application class:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class RestApiApplication { public static void main(String[] args) { SpringApplication.run(RestApiApplication.class, args); } }
In this class, the SpringApplication.run()
method is invoked, which bootstraps the application and starts the embedded server.
Creating a Simple RESTful Controller
With the project set up and the main application class defined, the next step is to create a RESTful controller. A controller handles HTTP requests and maps them to specific endpoints. Here’s an example of a simple REST controller:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { @GetMapping("/users") public String getAllUsers() { return "[{"id":1,"name":"Luke Douglas"},{"id":2,"name":"Jane Doe"}]"; } }
This controller maps the GET request on the /users endpoint to the getAllUsers()
method, which returns a JSON array of user data. That’s the first step toward exposing your resources through a RESTful API.
Running the Application
To run your Spring Boot application, you can use the command:
mvn spring-boot:run
Once the application is running, you can access the endpoint http://localhost:8080/users
to retrieve the user data in JSON format. This simple setup illustrates how to create a foundational structure for building RESTful services in Java.
As your project grows, ensure to organize your packages logically, separating controllers, models, and services. This separation of concerns will enhance maintainability and scalability, enabling you to build more complex applications with ease.
Implementing RESTful Endpoints with Java
Implementing RESTful endpoints in Java is at the heart of creating efficient web services. With frameworks like Spring Boot, developers can swiftly map HTTP requests to Java methods, effectively bridging the gap between HTTP and Java’s object-oriented paradigms.
To begin the implementation, let’s ponder a simple user management API. We’ll create endpoints for retrieving a list of users, adding a new user, updating an existing user, and deleting a user. Each endpoint will correspond to a standard HTTP method: GET, POST, PUT, and DELETE.
First, we would define a User class that models our user resource:
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; // Constructors, Getters, and Setters public User() {} public User(String name) { this.name = name; } public Long getId() { return id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
This class uses JPA annotations to indicate that it is an entity and includes an autogenerated ID field. Next, we’ll create a repository interface that provides CRUD operations:
import org.springframework.data.jpa.repository.JpaRepository; public interface UserRepository extends JpaRepository { }
It is time to implement a controller to handle the requests. The UserController class will define our RESTful endpoints:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @RequestMapping("/users") public class UserController { @Autowired private UserRepository userRepository; @GetMapping public List getAllUsers() { return userRepository.findAll(); } @PostMapping public User createUser(@RequestBody User user) { return userRepository.save(user); } @PutMapping("/{id}") public ResponseEntity updateUser(@PathVariable Long id, @RequestBody User userDetails) { User user = userRepository.findById(id) .orElseThrow(() -> new ResourceNotFoundException("User not found with id " + id)); user.setName(userDetails.getName()); final User updatedUser = userRepository.save(user); return ResponseEntity.ok(updatedUser); } @DeleteMapping("/{id}") public ResponseEntity deleteUser(@PathVariable Long id) { userRepository.deleteById(id); return ResponseEntity.noContent().build(); } }
In this controller:
- The GET method retrieves all users from the database.
- The POST method allows for adding a new user using the request body.
- The PUT method updates the user information for a given ID.
- The DELETE method removes a user based on the provided ID.
Handling errors is also crucial. For example, when trying to update or delete a user that doesn’t exist, we can throw a custom exception. Here’s a simple implementation of the ResourceNotFoundException:
import org.springframework.http.HttpStatus; import org.springframework.web.bind.annotation.ResponseStatus; @ResponseStatus(value = HttpStatus.NOT_FOUND) public class ResourceNotFoundException extends RuntimeException { public ResourceNotFoundException(String message) { super(message); } }
This exception will return a 404 status code when a resource is not found, ensuring that clients receive appropriate feedback.
Finally, to handle JSON serialization and deserialization, we rely on the capabilities of Spring Boot, which uses Jackson by default. This integration allows for seamless conversion between Java objects and JSON, enabling the API to communicate effectively with clients.
Implementing RESTful endpoints in Java using Spring Boot simplifies the process of creating robust APIs. With clear structuring and proper error handling, you can build services that are both efficient and simple to operate, ultimately enhancing the client-server communication experience.
Testing and Securing Java RESTful APIs
Testing and securing Java RESTful APIs is a critical aspect of the development lifecycle. It ensures that APIs not only function correctly but also adhere to security best practices. In this section, we will explore various techniques to effectively test and secure your Java RESTful APIs.
Testing Java RESTful APIs
Testing is essential for verifying that your RESTful services behave as expected. A common approach to testing APIs in Java is using the Spring Test framework combined with JUnit. This allows you to write integration tests that simulate HTTP requests to your endpoints.
Here’s a simple example of how to write a test case for the UserController
we implemented earlier:
import static org.springframework.test.web.servlet.MockMvc.*; import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc; import org.springframework.boot.test.context.SpringBootTest; @SpringBootTest @AutoConfigureMockMvc public class UserControllerTest { @Autowired private MockMvc mockMvc; @BeforeEach public void setUp() { // Setup code can go here, if necessary } @Test public void getAllUsers_ShouldReturnAllUsers() throws Exception { mockMvc.perform(get("/users")) .andExpect(status().isOk()) .andExpect(content().contentType("application/json")) .andExpect(jsonPath("$[0].name").value("Alex Stein")); } }
In this test, we use the MockMvc
class to simulate an HTTP GET request to the /users
endpoint. The assertions verify that the response status is OK and that the content type is JSON. You can expand your tests to cover other endpoints and HTTP methods as needed.
Securing Java RESTful APIs
Security is paramount when exposing APIs to the internet. Java provides several ways to secure RESTful APIs, with Spring Security being one of the most robust frameworks available. It helps protect your applications from common vulnerabilities like CSRF, XSS, and unauthorized access.
A basic approach to securing your API is to use HTTP Basic Authentication or JWT (JSON Web Tokens). Below is an example of how to set up basic authentication using Spring Security:
import org.springframework.context.annotation.Bean; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.web.authentication.www.BasicAuthenticationFilter; @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/users/**").authenticated() .and() .httpBasic(); } }
This configuration disables CSRF protection (which is fine for stateless REST APIs), requires authentication for all endpoints under /users/**
, and enables HTTP Basic Authentication. This is just a starting point, and you can further customize the security settings as needed.
For more advanced security measures, ponder implementing JWTs. JWT allows you to create a token upon user authentication that can be sent with subsequent requests, enabling stateless session management. Implementing JWT involves generating a token after a successful login and validating it with each request.
Effective testing and security practices are essential components of developing resilient Java RESTful APIs. By employing the right tools and strategies, you can ensure that your APIs perform reliably while safeguarding sensitive data and operations from unauthorized access.