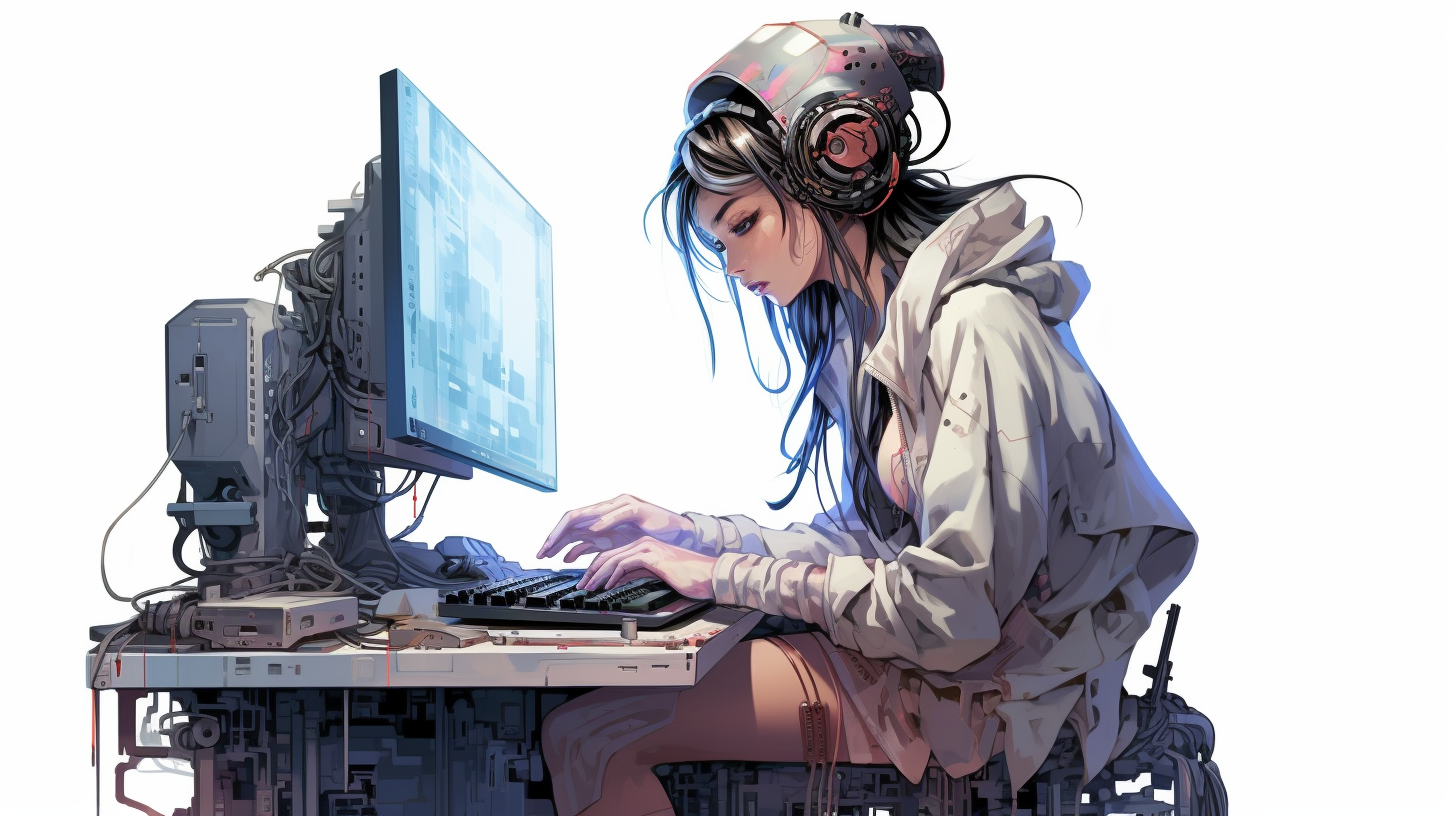
JavaScript in Robotics and Hardware
When delving into the realm of robotics development, JavaScript emerges as a surprisingly versatile language, thanks to an array of frameworks specifically designed to facilitate robotic applications. These frameworks not only streamline the development process but also open up opportunities for innovative solutions in controlling and programming robotic systems.
One prominent framework is Johnny-Five, a JavaScript Robotics and IoT platform that provides a simple API for controlling hardware through JavaScript. It abstracts the complexities of various hardware interactions, allowing developers to focus on building intelligent behaviors. Here’s a basic example of how to use Johnny-Five to control an LED connected to an Arduino:
const { Board, Led } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const led = new Led(13); led.blink(500); // Blink the LED every 500ms });
Another notable framework, Node-RED, offers a visual programming interface that integrates JavaScript for wiring together hardware devices, APIs, and online services. This framework is particularly useful for those who prefer a more graphical approach to programming, allowing developers to create complex workflows without deep diving into the code. With Node-RED, one can easily combine various nodes to interact with a robot’s sensors and actuators.
For developers looking to harness the power of WebSocket and real-time communication, Socket.io is an essential library. This allows for real-time, bidirectional communication between clients and servers, which is pivotal in robotics for responsive control and monitoring. Ponder the following snippet that establishes a connection and sends data from a robot:
const socket = require("socket.io-client")("http://localhost:3000"); socket.on("connect", () => { console.log("Connected to the server"); // Send robot's position const position = { x: 100, y: 200 }; socket.emit("robotPosition", position); });
These frameworks exemplify how JavaScript can be utilized in robotics development. By using existing libraries and tools, developers can innovate rapidly and efficiently. As the community continues to grow, so too will the capabilities and support for JavaScript in the field of robotics.
Integrating JavaScript with Microcontrollers
Integrating JavaScript with microcontrollers presents a unique opportunity to harness the flexibility and power of a web-centric language while working directly with hardware. This integration enables developers to create dynamic and responsive robotic systems that can interact with both the physical world and online resources seamlessly.
Microcontrollers like the Arduino have become staples in the robotics community due to their affordability and ease of use. JavaScript provides the means to program these devices effectively through frameworks like Johnny-Five, which allows developers to interact with the hardware in a simple and intuitive manner.
To begin integrating JavaScript with a microcontroller, one typically starts by establishing a connection. For instance, when using an Arduino, the board must be connected to a host computer via USB. The following example demonstrates how to initiate this connection and read data from a potentiometer:
const { Board, Sensor } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const potentiometer = new Sensor("A0"); potentiometer.on("change", () => { console.log(`Potentiometer value: ${potentiometer.value}`); }); });
This code initializes a connection to the Arduino board and listens for changes in the value of a potentiometer connected to the analog pin A0. Every time the potentiometer is adjusted, the new value is logged to the console, showcasing the real-time interaction capabilities of JavaScript.
Furthermore, microcontrollers can be expanded with various sensors and actuators, allowing for intricate control mechanisms. For example, integrating a temperature sensor to control a fan based on ambient temperature can be accomplished elegantly:
const { Board, Sensor, Led } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const temperature = new Sensor("A1"); const fan = new Led(9); // Assume a fan is controlled via PWM on pin 9 temperature.on("change", () => { if (temperature.value > 30) { // Threshold temperature fan.on(); // Turn on fan console.log("Fan turned on"); } else { fan.off(); // Turn off fan console.log("Fan turned off"); } }); });
This code snippet illustrates a scenario where the fan operates based on temperature readings. It showcases a fundamental application of control logic in robotics using JavaScript, allowing developers to create intelligent systems that respond to environmental changes.
Moreover, the integration of JavaScript with microcontrollers isn’t limited to local applications. By employing frameworks like Node-RED, developers can create complex flows that incorporate web services, allowing microcontrollers to send and receive data over the internet. This opens the door for remote monitoring and control, which are essential features in modern robotics.
The integration of JavaScript with microcontrollers not only simplifies the development process but also enhances the potential of robotic systems. By using frameworks like Johnny-Five and Node-RED, developers can create sophisticated applications that bridge the gap between hardware and the web, driving innovation in the field of robotics.
Real-Time Data Processing in Robotics Using JavaScript
Real-time data processing is a critical aspect of robotics that ensures systems can respond to dynamic environments promptly and efficiently. JavaScript, with its event-driven architecture and support for asynchronous programming, is well-suited for handling real-time data in robotic applications. When robots interact with their surroundings, they must process sensor input and execute commands in real-time to maintain functionality and safety.
One of the foundational tools for real-time data processing in JavaScript is the concept of event listeners. These listeners monitor sensor inputs and trigger actions based on data changes. For instance, when working with a distance sensor to avoid obstacles, we can use JavaScript to continuously read the sensor data and adjust the robot’s movements accordingly. Below is an example that showcases how to implement this using Johnny-Five:
const { Board, Sensor, Motor } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const distance = new Sensor("A0"); const motor = new Motor(9); // Motor connected to pin 9 distance.on("change", () => { const distanceValue = distance.value; console.log(`Distance: ${distanceValue} cm`); if (distanceValue < 20) { // If an obstacle is detected within 20 cm motor.stop(); // Stop the motor console.log("Obstacle detected! Stopping motor."); } else { motor.start(); // Continue moving } }); });
In this code, we set up a distance sensor that continuously checks for obstacles. When the distance falls below a certain threshold, the motor stops, preventing potential collisions. This example highlights JavaScript’s capability to handle real-time sensor data and control actuators based on that data.
Moreover, JavaScript’s ability to handle asynchronous operations through Promises and async/await syntax enhances its effectiveness in real-time applications. For example, when integrating multiple sensors, it’s important to handle data from each sensor without blocking the program execution. The following snippet demonstrates how to read data from multiple sensors asynchronously:
const { Board, Sensor } = require("johnny-five"); const board = new Board(); board.on("ready", async () => { const temperatureSensor = new Sensor("A1"); const lightSensor = new Sensor("A2"); const readSensors = async () => { while (true) { const temperatureValue = await new Promise(resolve => { temperatureSensor.once("change", () => resolve(temperatureSensor.value)); }); const lightValue = await new Promise(resolve => { lightSensor.once("change", () => resolve(lightSensor.value)); }); console.log(`Temperature: ${temperatureValue} °C, Light: ${lightValue} lux`); await new Promise(resolve => setTimeout(resolve, 1000)); // Delay for 1 second } }; readSensors(); });
This snippet sets up two sensors: one for temperature and one for light. The `readSensors` function continuously retrieves data from both sensors every second without blocking the main thread. This method allows for efficient and responsive data handling, crucial for modern robotics applications.
In applications requiring complex data analysis, such as image processing from cameras, libraries like TensorFlow.js can be utilized. This library allows for the deployment of machine learning models directly in the browser or Node.js, enabling robots to make intelligent decisions based on processed data. Here’s a brief illustration of how to integrate TensorFlow.js for real-time image classification:
const tf = require("@tensorflow/tfjs-node"); const { Board, Camera } = require("johnny-five"); board.on("ready", async () => { const camera = new Camera(); // Assume we've set up a camera const model = await tf.loadLayersModel('file://path/to/model.json'); camera.on("frame", async (image) => { const inputTensor = tf.browser.fromPixels(image).expandDims(0); const predictions = model.predict(inputTensor); predictions.print(); }); });
This code snippet exemplifies how a robot can use a camera to capture images and a pre-trained model to classify what it sees in real-time. Using the computational power of TensorFlow.js in conjunction with JavaScript brings machine learning capabilities to robotics, allowing for sophisticated interaction with the environment.
Ultimately, using JavaScript for real-time data processing in robotics not only simplifies the development workflow but also enhances the system’s responsiveness and adaptability. By embracing tools and frameworks tailored for real-time applications, developers can create advanced robotic systems that interact intelligently with their surroundings.
Case Studies: Successful Robotics Projects Using JavaScript
Exploring the practical applications of JavaScript in robotics reveals a myriad of successful projects that exemplify the language’s capabilities and flexibility. The following case studies highlight how developers have harnessed JavaScript to create innovative robotic solutions that address various challenges and demonstrate the potential of integrating this web-centric language into hardware development.
One striking example is the RoboCup Soccer project, which leverages the Johnny-Five framework to control robotic soccer players. These robots are designed to recognize and respond to their environment, making decisions based on sensor inputs to navigate the playing field. The project employs a combination of computer vision for ball detection and the coordination of multiple motors for movement. Here’s a simplified version of the code used to identify the ball and maneuver towards it:
const { Board, Sensor, Motor } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const ballSensor = new Sensor("A0"); // Assume a sensor detects the ball const leftMotor = new Motor(9); const rightMotor = new Motor(10); ballSensor.on("change", () => { const distanceValue = ballSensor.value; if (distanceValue < 30) { // If ball is within 30 cm leftMotor.start(); rightMotor.start(); console.log("Moving towards the ball!"); } else { leftMotor.stop(); rightMotor.stop(); console.log("Stopping."); } }); });
In another project, Home Automation Robots utilize Node-RED and JavaScript to create intelligent home environments. These robots can control various appliances, respond to user commands via a mobile application, and even learn from user behavior. By combining Node-RED’s visual programming interface with JavaScript, developers can wire up sensors, actuators, and web services seamlessly. The following snippet showcases how a developer might create a flow to control a robotic arm based on voice commands:
const { Board, Servo } = require("johnny-five"); const board = new Board(); board.on("ready", () => { const arm = new Servo(10); // Assume servo on pin 10 // Simulated voice command input const commands = ["move up", "move down"]; commands.forEach(command => { if (command === "move up") { arm.to(90); // Move arm to 90 degrees console.log("Arm moved up."); } else if (command === "move down") { arm.to(0); // Move arm to 0 degrees console.log("Arm moved down."); } }); });
Moreover, the Drone Navigation System is a cutting-edge application that utilizes JavaScript for controlling drones equipped with cameras and sensors. The system processes video feeds in real-time, enabling the drone to navigate autonomously via obstacle avoidance algorithms. Using TensorFlow.js for image classification, the drone can identify objects in its path and adjust its flight accordingly. Below is a conceptual example of how this might be implemented:
const tf = require("@tensorflow/tfjs-node"); const { Drone } = require("drone-library"); // Placeholder for actual drone library const drone = new Drone(); async function startDrone() { const model = await tf.loadLayersModel('file://path/to/model.json'); drone.on("cameraFrame", async (image) => { const inputTensor = tf.browser.fromPixels(image).expandDims(0); const predictions = await model.predict(inputTensor); // Logic to navigate based on predictions if (predictions.class === "obstacle") { drone.moveBackward(); console.log("Obstacle detected! Moving backward."); } else { drone.moveForward(); console.log("Path clear. Moving forward."); } }); } startDrone();
These case studies illustrate the diverse applications of JavaScript in robotics, showcasing how developers are pushing the boundaries of what is possible with this language. From playing soccer to automating homes and navigating drones, JavaScript has proven to be a powerful tool that fosters innovation in robotic systems. As these projects continue to evolve, they will undoubtedly inspire new ideas and implementations within the field, paving the way for more sophisticated robotic applications in the future.
Future Trends: JavaScript’s Role in the Evolution of Robotics
As we look toward the future, JavaScript’s role in the evolution of robotics becomes increasingly prominent, driven by its adaptability, ease of use, and the ongoing development of libraries and frameworks tailored for robotic applications. The convergence of JavaScript with emerging technologies such as artificial intelligence (AI), the Internet of Things (IoT), and cloud computing is set to redefine how we approach robotics.
One significant trend is the growing incorporation of AI into robotic systems. JavaScript, particularly through the use of libraries like TensorFlow.js, enables developers to implement machine learning models directly in their applications. This capability allows robots to analyze data in real-time and make intelligent decisions based on learned behaviors. For instance, consider a robot designed for warehouse automation that uses JavaScript to process visual data and optimize its navigation routes. Below is a conceptual implementation of a robot that utilizes TensorFlow.js for real-time object detection:
const tf = require("@tensorflow/tfjs-node"); const { Board, Camera } = require("johnny-five"); const board = new Board(); board.on("ready", async () => { const camera = new Camera(); // Assume we've set up a camera const model = await tf.loadLayersModel('file://path/to/model.json'); camera.on("frame", async (image) => { const inputTensor = tf.browser.fromPixels(image).expandDims(0); const predictions = model.predict(inputTensor); console.log(predictions.dataSync()); // Output predictions }); });
This integration of JavaScript and AI enhances the capability of robots to perform complex tasks with minimal human intervention, paving the way for a future where robots can adapt to unpredictable environments.
Additionally, the rise of IoT technologies is facilitating the seamless integration of JavaScript with cloud services. Cloud-based solutions provide a platform for IoT devices to communicate, share data, and perform analytics at scale. JavaScript excels in this realm, allowing developers to create applications that connect multiple robotic units, enabling collaboration and data sharing among them. For example, a fleet of delivery drones could communicate through a cloud service to optimize delivery routes and avoid obstacles based on real-time data. Here’s a simple example of how a drone might send its status updates to a cloud endpoint using JavaScript:
const axios = require("axios"); async function sendStatusUpdate(droneId, status) { try { await axios.post("https://api.example.com/drone/status", { droneId, status, }); console.log("Status update sent: ", status); } catch (error) { console.error("Error sending status update: ", error); } } // Example usage sendStatusUpdate("drone123", { battery: "80%", location: "45.123,-93.456" });
This capability to communicate over the cloud empowers robots to operate more intelligently, using data from various sources to improve their performance and efficiency.
Moreover, JavaScript’s inherent support for asynchronous programming and event-driven architectures aligns well with the demands of real-time robotic systems. As robots become increasingly complex, the ability to process multiple inputs and outputs concurrently very important. Tools like Node.js allow for the creation of non-blocking applications that can handle various tasks concurrently, ensuring that robots remain responsive to their environments.
Looking ahead, the integration of JavaScript into robotic systems is likely to accelerate, driven by community innovation and contributions to open-source projects. As frameworks evolve and new libraries emerge, developers will have access to even more powerful tools, making it easier to create sophisticated robotics applications. The future may see the proliferation of autonomous systems powered by JavaScript, capable of performing a wide range of tasks, from personal assistants to industrial automation, all while learning and adapting to their surroundings.
Ultimately, the role of JavaScript in robotics is set to expand dramatically, shaping the next generation of intelligent machines that not only coexist with humans but also enhance our capabilities in unprecedented ways.