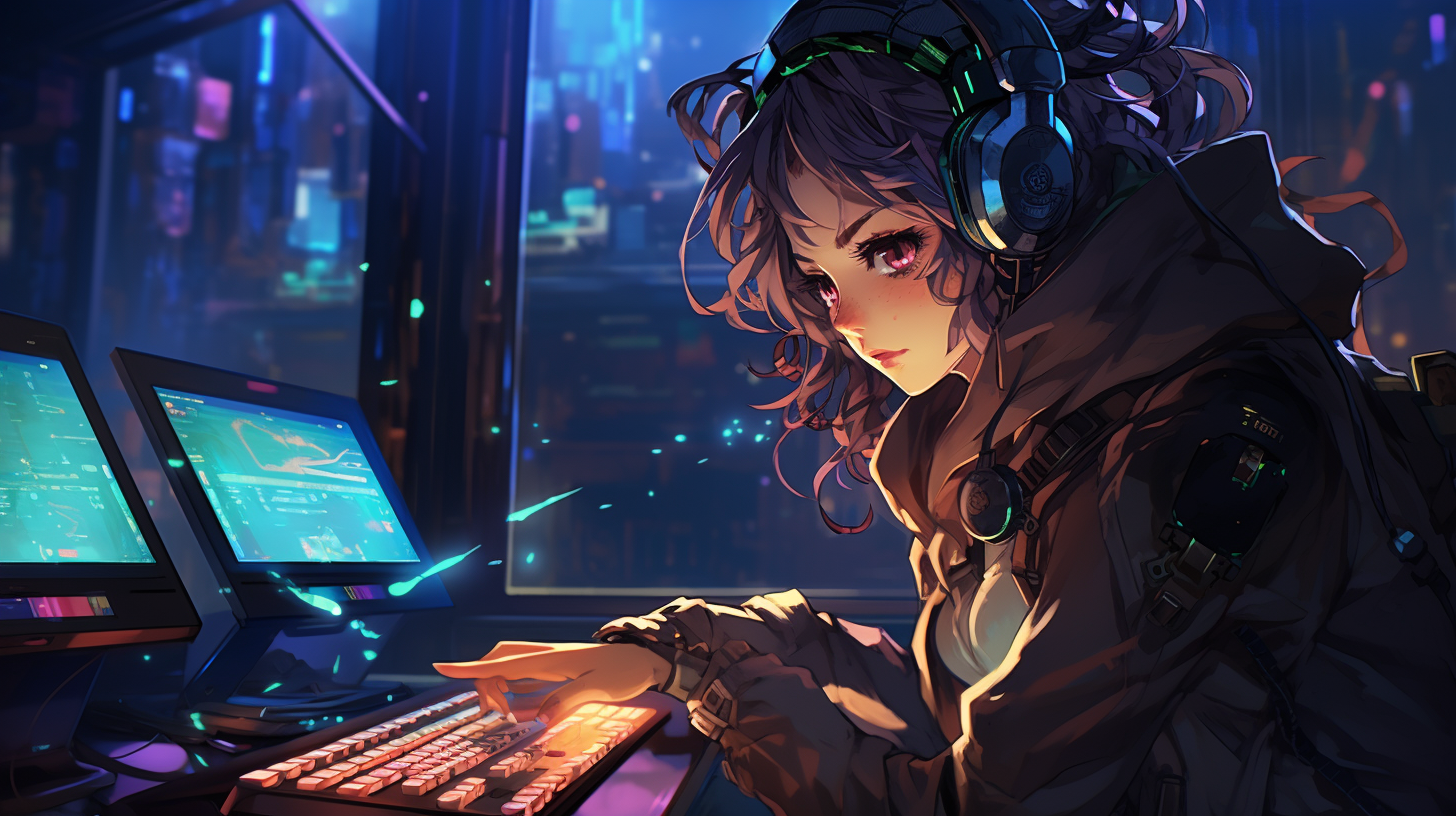
Integrating Bash with Other Languages
Integrating Bash with Python allows developers to leverage the strengths of both languages, creating a seamless workflow that enhances efficiency and productivity. Bash excels in handling system-level tasks, while Python offers powerful libraries and a rich ecosystem for data manipulation, web development, and more. This combination allows you to execute shell commands directly from Python scripts or use Python to control Bash scripts, thereby integrating the two worlds effectively.
One common approach to execute Bash commands from within a Python script is by using the subprocess
module. This module provides a simple interface for spawning new processes, connecting to their input/output/error pipes, and obtaining their return codes, offering a robust way to call shell commands.
Here’s an example of how you can use the subprocess.run
function to execute a Bash command:
import subprocess # Run a simple Bash command result = subprocess.run(['ls', '-l'], capture_output=True, text=True) # Output the result print(result.stdout)
In this example, the ls -l
command is executed, and the output is captured and printed to the console. The capture_output=True
argument ensures that both stdout and stderr are captured, while text=True
makes the output a string instead of bytes, simplifying further processing.
Another useful approach is to call a Bash script directly from Python. This allows for more complex command sequences to be encapsulated in a script, enabling better code organization and reuse. Here’s how you can run a Bash script named script.sh
:
import subprocess # Run a Bash script result = subprocess.run(['bash', 'script.sh'], capture_output=True, text=True) # Check for errors and print the output if result.returncode == 0: print("Script output:", result.stdout) else: print("Error:", result.stderr)
In this snippet, the script.sh
script is executed, and both the output and error streams are handled appropriately. The return code is checked to ensure that the script executed successfully, allowing for effective error handling.
For scenarios where you want to pass arguments from Python to a Bash script, simply include the arguments in the list passed to subprocess.run
:
import subprocess # Arguments to pass to the script arg1 = "Hello" arg2 = "World" # Run the Bash script with arguments result = subprocess.run(['bash', 'script.sh', arg1, arg2], capture_output=True, text=True) print("Script output:", result.stdout)
In this case, script.sh
can access $1
and $2
as the first and second arguments, respectively, allowing for dynamic behavior based on the inputs provided from the Python script.
Lastly, if you need to run a lengthy Bash operation without blocking your Python script, you can use the subprocess.Popen
method. This allows the script to continue executing while the Bash command runs asynchronously:
import subprocess import time # Start a long-running Bash command process = subprocess.Popen(['sleep', '10']) # Continue running Python code while the command executes print("The Bash command is running in the background...") # Wait for the command to complete process.wait() print("Bash command completed.")
This example demonstrates running a long-running command, such as sleep
, which simulates a delay without blocking the main thread of execution in Python.
Through these methods, integrating Bash with Python not only enhances the capabilities of your applications but also allows for a more streamlined and efficient development process, tapping into the strengths of both languages effectively.
Using Bash Scripts in Ruby Applications
Incorporating Bash scripts within Ruby applications presents an intriguing way to harness the power of both languages, allowing developers to utilize Ruby’s object-oriented features alongside Bash’s robust command-line capabilities. Ruby, known for its elegance and readability, can call Bash scripts to execute system-level tasks, manage files, and perform operations that are often cumbersome to handle purely within Ruby. This synergy enables a richer and more versatile development experience.
To execute a Bash script from Ruby, the `system` method is a simpler choice. This method runs the specified command in a subshell, returning true if the command is successful and false otherwise. Here’s an example of how to call a Bash script named `script.sh`:
# Run a Bash script result = system("bash script.sh") if result puts "Script executed successfully." else puts "Script failed to execute." end
In this example, the result of the `system` call indicates whether the script executed successfully, enabling simple error handling.
For scenarios where you need to capture the output of a Bash command directly within your Ruby application, the backticks or the `%x` syntax are excellent alternatives. Both methods execute a command and return its standard output as a string. Here’s how you can use backticks to capture the output of the `ls -l` command:
# Capture output from a Bash command output = `ls -l` puts "Command output:n#{output}"
This approach allows you to work with the output like any other Ruby string, making it easy to manipulate or display as needed.
Passing arguments from Ruby to a Bash script is also simpler. By including arguments in the command string, you can dynamically alter the behavior of your scripts. Here’s an example:
# Arguments to pass to the script arg1 = "Hello" arg2 = "World" # Run the Bash script with arguments output = `bash script.sh #{arg1} #{arg2}` puts "Script output:n#{output}"
In this case, `script.sh` can access `$1` and `$2` as the first and second arguments, respectively, enabling it to behave differently based on the inputs provided from Ruby.
If you have a long-running Bash process that you want to run without blocking the Ruby application, you can use the `Process.spawn` method. This allows the Ruby script to continue executing while the Bash command runs asynchronously:
# Start a long-running Bash command pid = Process.spawn("sleep 10") puts "The Bash command is running in the background..." # Wait for the command to complete Process.wait(pid) puts "Bash command completed."
This example showcases how to initiate a long-running command such as `sleep`, allowing the Ruby application to maintain responsiveness during the execution of the external command.
Integrating Bash scripts into Ruby applications not only enhances functionality but also enriches the development process. By using Ruby’s expressive syntax alongside Bash’s command-line prowess, developers can create more efficient and powerful applications that elegantly blend the best features of both worlds.
Calling Bash from Java: Bridging Two Worlds
Calling Bash from Java opens up a world of possibilities, allowing developers to harness the power of the command line while using Java’s robust architecture. Java, known for its portability and scalability, can seamlessly integrate with Bash scripts to perform system-level tasks, manage files, and execute complex command sequences. This integration is particularly useful in scenarios where tasks require quick execution or when using existing Bash scripts is more efficient than rewriting logic in Java.
To execute a Bash command from a Java application, the ProcessBuilder
class is a reliable choice. This class allows you to create operating system processes and manage their input and output streams efficiently. Here’s an example of using ProcessBuilder
to run a simple Bash command:
ProcessBuilder processBuilder = new ProcessBuilder("bash", "-c", "ls -l"); processBuilder.redirectErrorStream(true); Process process = processBuilder.start(); BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream())); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } int exitCode = process.waitFor(); System.out.println("Exited with code: " + exitCode);
In this code snippet, we create a new ProcessBuilder
instance to run the ls -l
command. The output of the command is read from the input stream, and each line is printed to the console. The redirectErrorStream(true
) method call very important as it merges standard error with standard output, making it easier to handle any errors encountered during execution.
For scenarios where you need to execute a Bash script, the process is similar. You simply need to provide the script name and any arguments directly to the ProcessBuilder
:
ProcessBuilder processBuilder = new ProcessBuilder("bash", "script.sh", "arg1", "arg2"); processBuilder.redirectErrorStream(true); Process process = processBuilder.start(); BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream())); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } int exitCode = process.waitFor(); System.out.println("Exited with code: " + exitCode);
In this example, we run a script called script.sh
and pass two arguments to it. This allows the script to behave dynamically based on the input provided, using the flexibility of both Bash and Java.
When working with long-running Bash processes, you might want your Java application to remain responsive. This can be achieved using the ProcessBuilder
to spawn the process and continuing execution without waiting for it to finish:
ProcessBuilder processBuilder = new ProcessBuilder("bash", "-c", "sleep 10"); Process process = processBuilder.start(); System.out.println("The Bash command is running in the background..."); // Continue executing other code // Optionally wait for the process to finish later int exitCode = process.waitFor(); System.out.println("Bash command completed with code: " + exitCode);
This demonstration highlights how you can initiate a long-running command such as sleep
, allowing the Java application to execute further logic without being blocked by the running Bash command.
Integrating Bash into Java applications not only augments functionality but also enriches the development experience. By bridging the gap between these two powerful languages, developers can enhance their productivity, streamline workflows, and tap into the strengths of each language effectively.
Interoperability: Bash and JavaScript in Web Development
Integrating Bash with JavaScript in web development opens up exciting possibilities for developers who want to harness the robustness of Bash while using the flexibility and interactivity of JavaScript. This integration is particularly beneficial when dealing with server-side scripting, automating deployment processes, or managing system-level tasks that JavaScript alone may struggle to perform. Node.js, a popular JavaScript runtime built on Chrome’s V8 engine, offers excellent support for executing Bash commands, allowing developers to create rich, dynamic applications that can interact seamlessly with the underlying operating system.
To execute Bash commands from a Node.js application, the child_process module provides a simpler interface. This module allows you to spawn new processes, send commands to the shell, and obtain their return results. Here’s a basic example of how to use the exec function from the child_process module to run a simple Bash command:
const { exec } = require('child_process'); // Run a simple Bash command exec('ls -l', (error, stdout, stderr) => { if (error) { console.error(`Error executing command: ${error}`); return; } console.log(`Command output:n${stdout}`); });
In this example, the ls -l command is executed, and its output is captured and printed to the console. If any error occurs during execution, it’s logged to the console as well, providing an effective way to handle potential issues.
When it comes to executing a Bash script, the process remains largely the same. You can run a script by providing its path and any necessary arguments. Here’s how you might execute a script named script.sh:
const { exec } = require('child_process'); // Run a Bash script with arguments const arg1 = 'Hello'; const arg2 = 'World'; exec(`bash script.sh ${arg1} ${arg2}`, (error, stdout, stderr) => { if (error) { console.error(`Error executing script: ${error}`); return; } console.log(`Script output:n${stdout}`); });
In this snippet, script.sh is executed with two arguments passed in from the Node.js application. This enables the script to access these arguments within its execution context, allowing for dynamic behavior based on the inputs.
For scenarios where you want to execute a long-running Bash command without blocking your Node.js application, the spawn function from the child_process module is a great choice. Unlike exec, which buffers the output, spawn handles the command as a stream, allowing for more control over the input/output:
const { spawn } = require('child_process'); // Start a long-running Bash command const process = spawn('bash', ['-c', 'sleep 10']); console.log('The Bash command is running in the background...'); // Listen for data from stdout process.stdout.on('data', (data) => { console.log(`Output: ${data}`); }); // Listen for errors process.stderr.on('data', (data) => { console.error(`Error: ${data}`); }); // When the process completes process.on('exit', (code) => { console.log(`Bash command completed with exit code: ${code}`); });
This example demonstrates how to run a long-running command such as sleep, allowing the Node.js application to remain responsive while the command executes in the background. The use of event listeners for stdout and stderr provides real-time feedback on the execution process.
Integrating Bash with JavaScript through Node.js not only enhances the capabilities of your applications but also facilitates a more efficient and powerful development environment. By merging the strengths of both languages, developers can create applications that are both versatile and robust, tapping into the rich features provided by each language effectively.