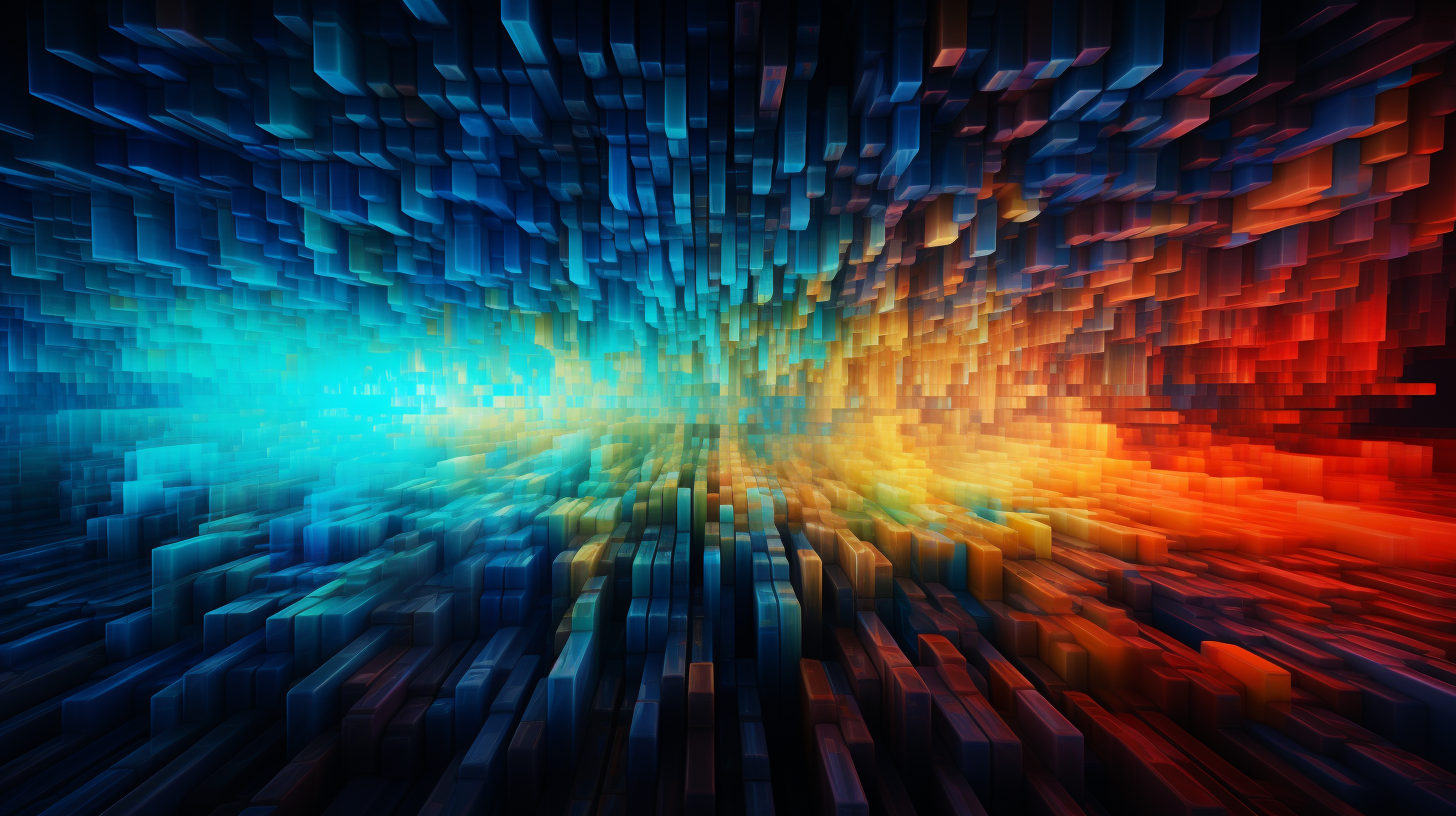
Python in Space Exploration: Data Analysis and Simulation
In the context of space exploration, Python has emerged as a cornerstone language that facilitates a wide array of applications, from mission planning to real-time operations. Its versatility and ease of integration with various tools and libraries make it particularly suited for the unique challenges encountered in space missions.
One of the most significant applications of Python in space missions is its role in data processing. Spacecraft generate massive amounts of telemetry data that must be analyzed and interpreted promptly. Python’s rich ecosystem of data analysis libraries, such as Pandas and Numpy, empowers scientists and engineers to efficiently manipulate and visualize this data. For instance, consider the following code snippet that demonstrates how to load and analyze telemetry data:
import pandas as pd # Load telemetry data from a CSV file data = pd.read_csv('telemetry_data.csv') # Display the first few rows print(data.head()) # Perform basic statistics print(data.describe())
Furthermore, Python’s capabilities extend to simulation environments where mission scenarios are modeled. Libraries like Matplotlib and SimPy enable teams to create simulations of spacecraft operations under various conditions, allowing for better preparation and risk assessment. A simple example of simulating spacecraft trajectories might look like this:
import numpy as np import matplotlib.pyplot as plt # Define time variables time = np.linspace(0, 10, 100) # 10 seconds # Simulate a simple trajectory x = 5 * np.cos(time) # X position y = 5 * np.sin(time) # Y position # Plotting the trajectory plt.plot(x, y) plt.title('Spacecraft Trajectory Simulation') plt.xlabel('X Position') plt.ylabel('Y Position') plt.axis('equal') plt.grid() plt.show()
Moreover, Python’s integration with hardware and real-time systems is noteworthy. Libraries such as PySerial allow for communication with spacecraft hardware, enabling on-board systems to be monitored and controlled effectively. Here’s a brief example of how one might establish a connection to a satellite’s serial interface:
import serial # Establishing a serial connection ser = serial.Serial('/dev/ttyUSB0', 9600, timeout=1) # Reading data from the satellite data = ser.readline() print(data) ser.close()
Python is also utilized in the automation of satellite operations. The ability to script complex sequences of commands ensures that operations can be executed with precision and speed, minimizing the chance of human error during critical mission phases. The following example illustrates how one might automate a series of command transmissions to a satellite:
commands = ['START', 'STATUS', 'STOP'] for command in commands: ser.write(command.encode()) response = ser.readline() print(f"Response to {command}: {response}")
Data Analysis Techniques for Spaceborne Instruments
When it comes to analyzing data collected by spaceborne instruments, Python’s robust set of libraries and tools plays an important role in transforming raw data into actionable insights. The data collected during space missions can range from telemetry to scientific measurements, often requiring sophisticated processing techniques to extract meaningful information. In this context, several data analysis techniques become indispensable.
One of the primary techniques employed in the analysis of spaceborne data is time-series analysis, given the temporal nature of the data collected. Libraries such as Pandas and Scipy provide the necessary functionality to manipulate and analyze time-series data efficiently. Here’s an example of how to perform a simple moving average on telemetry data:
import pandas as pd # Load telemetry data with a time index data = pd.read_csv('telemetry_data.csv', parse_dates=['timestamp'], index_col='timestamp') # Calculate the moving average data['moving_average'] = data['sensor_value'].rolling(window=5).mean() # Display the result print(data[['sensor_value', 'moving_average']].head(10))
Another important aspect of data analysis involves the identification of anomalies or outliers in the datasets. This is particularly crucial in space missions where the detection of unexpected sensor readings could indicate potential issues. Python’s Numpy and Scikit-learn libraries can be leveraged for this purpose. The following code demonstrates how to use Z-scores to identify outliers:
import numpy as np from scipy import stats # Assume sensor_values contains the telemetry data for a specific sensor sensor_values = data['sensor_value'].values # Calculate Z-scores z_scores = stats.zscore(sensor_values) # Identify outliers (Z-score > 3 or 3)[0] print("Outlier indices:", outliers)
Additionally, visualization is a paramount technique in data analysis, allowing scientists to interpret the data visually for patterns and anomalies. Python’s Matplotlib and Seaborn libraries provide comprehensive tools for data visualization. Here’s an example of plotting the telemetry data along with the moving average:
import matplotlib.pyplot as plt # Plotting the telemetry data and its moving average plt.figure(figsize=(12, 6)) plt.plot(data.index, data['sensor_value'], label='Sensor Value', alpha=0.5) plt.plot(data.index, data['moving_average'], label='Moving Average', color='orange') plt.title('Telemetry Data and Moving Average') plt.xlabel('Time') plt.ylabel('Sensor Value') plt.legend() plt.grid() plt.show()
Furthermore, multivariate analysis techniques are often employed to understand the relationships between different sensors and their readings. The Seaborn library can be instrumental in creating pair plots and correlation matrices, facilitating the discovery of these relationships:
import seaborn as sns # Assume df contains multiple sensor readings df = pd.read_csv('multiple_sensor_data.csv') # Create a pair plot to analyze relationships sns.pairplot(df) plt.title('Pair Plot of Sensor Readings') plt.show()
Simulation of Spacecraft Dynamics and Trajectories
When simulating spacecraft dynamics and trajectories, Python provides a rich set of tools that allow engineers and scientists to model the complex behaviors of spacecraft under various conditions. The ability to perform accurate simulations is important for mission planning, as it helps teams understand how spacecraft will interact with gravitational fields, maneuver through space, and respond to control inputs.
One of the foundational aspects of spacecraft dynamics is understanding Newton’s laws of motion, particularly as they apply to the three-dimensional motion of a spacecraft. By using libraries such as NumPy for numerical calculations and Matplotlib for visualization, we can create simulations that illustrate these principles. Below is an example demonstrating a simple simulation of a spacecraft’s motion in a two-dimensional space under the influence of gravitational forces.
import numpy as np import matplotlib.pyplot as plt # Constants G = 6.67430e-11 # gravitational constant mass_earth = 5.972e24 # mass of the Earth in kg r_earth = 6.371e6 # radius of the Earth in meters # Initial conditions pos = np.array([r_earth + 500e3, 0]) # 500 km above the Earth's surface vel = np.array([0, 7800]) # initial velocity in m/s (low Earth orbit) # Simulation parameters dt = 1 # time step in seconds num_steps = 3600 # number of simulation steps # Lists to store positions for plotting positions = [] # Simulation loop for _ in range(num_steps): r = np.linalg.norm(pos) # distance from the center of the Earth force_gravity = -G * mass_earth / r**2 # gravitational force magnitude acceleration = force_gravity * pos / r # acceleration vector vel += acceleration * dt # update velocity pos += vel * dt # update position positions.append(pos.copy()) # Convert positions to a NumPy array for plotting positions = np.array(positions) # Plotting the trajectory plt.figure(figsize=(8, 8)) plt.plot(positions[:, 0], positions[:, 1], label='Trajectory', color='b') plt.plot(0, 0, 'ro', markersize=10, label='Earth') # Earth position at origin plt.title('Simulated Spacecraft Trajectory') plt.xlabel('X Position (m)') plt.ylabel('Y Position (m)') plt.axis('equal') plt.grid() plt.legend() plt.show()
This simulation begins with a spacecraft positioned 500 kilometers above the Earth’s surface, moving at a velocity typical for low Earth orbit. By applying Newton’s laws iteratively, we can observe the effects of gravitational acceleration on the spacecraft’s path. The result is a visual representation of the trajectory, showcasing how the spacecraft would behave in the gravitational field of the Earth.
In more complex modeling scenarios, engineers often utilize specialized libraries like Poliastro for astrodynamics or pysim for simulating spacecraft dynamics under various control laws. These libraries provide built-in functions to handle orbital mechanics, making it easier to simulate maneuvers such as orbital insertions and transfers.
from poliastro.maneuver import Maneuver from poliastro.bodies import Earth from poliastro.twobody import Orbit from poliastro import plotting # Define an initial orbit initial_orbit = Orbit.from_classical(Earth, 7000 * u.km, 0.01, 0 * u.deg, 0 * u.deg, 0 * u.deg, 0 * u.deg) # Define a maneuver (for example, a Hohmann transfer) maneuver = Maneuver.hohmann(initial_orbit, target_orbit) # Apply the maneuver and calculate the new orbit final_orbit = initial_orbit.apply_maneuver(maneuver) # Plotting the orbits fig, ax = plt.subplots(figsize=(10, 10)) plotting.static_orbit(initial_orbit, label='Initial Orbit', ax=ax) plotting.static_orbit(final_orbit, label='Final Orbit', ax=ax) plt.title('Orbit Transfer Simulation') plt.xlabel('Distance (km)') plt.ylabel('Distance (km)') plt.grid() plt.legend() plt.show()
In this example, we create an initial orbit around the Earth and use a Hohmann transfer maneuver to simulate a transfer to a different orbit. The Poliastro library simplifies the handling of orbital mechanics, allowing for simpler adjustments and visualizations of orbital paths.
Machine Learning in Astrophysics Research
Machine learning has rapidly transformed the landscape of astrophysics research, offering powerful techniques to analyze vast datasets and uncover hidden patterns within the cosmic noise. Python, with its extensive libraries and frameworks, has become the go-to language for implementing machine learning algorithms in this domain. From classification of celestial objects to predicting the behavior of astrophysical phenomena, Python is at the forefront, driving innovative solutions.
One of the most compelling applications of machine learning in astrophysics is the classification of astronomical objects. Traditionally, this task relied on manual inspection by astronomers, which was both time-consuming and subject to human bias. However, with the advent of machine learning, we can now automate this process using classification algorithms like support vector machines (SVM) and neural networks. Below is an example of how to use the Scikit-learn library to classify galaxies based on their features:
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.svm import SVC from sklearn.metrics import classification_report # Load galaxy data data = pd.read_csv('galaxy_data.csv') # Split features and labels X = data.drop('class', axis=1) # features y = data['class'] # labels # Split the dataset into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the SVM classifier clf = SVC(kernel='linear') clf.fit(X_train, y_train) # Make predictions y_pred = clf.predict(X_test) # Print the classification report print(classification_report(y_test, y_pred))
This snippet demonstrates how to load galaxy data, prepare it for analysis, and apply an SVM classifier to classify galaxies. The use of machine learning not only streamlines the classification process but also enhances accuracy by using complex patterns that may not be immediately visible to human observers.
Another area where machine learning proves invaluable is in the detection of transient events, such as supernovae or gravitational waves. These events generate signals that can be faint and easily lost in the background noise of cosmic radiation. Machine learning algorithms, especially deep learning models, can sift through massive datasets to identify these rare occurrences. Here’s how one might implement a simple convolutional neural network (CNN) using Keras for this purpose:
import numpy as np from keras.models import Sequential from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Load and preprocess the data # Assume X_train and y_train are prepared with images of transient events and labels X_train = np.load('transient_event_images.npy') y_train = np.load('transient_event_labels.npy') # Build the CNN model model = Sequential() model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(64, 64, 1))) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dense(1, activation='sigmoid')) # binary classification # Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Train the model model.fit(X_train, y_train, epochs=10, batch_size=32)
In this example, we define a CNN to classify images of transient events. By training on a large dataset of labeled images, the model learns to identify patterns that may indicate a transient event, significantly improving detection capabilities in astrophysical research.
Furthermore, machine learning techniques are applied in the sphere of simulation and modeling of complex astrophysical phenomena. For instance, generative models can produce synthetic astrophysical observations that can be used to train classifiers or to test theories. The following code demonstrates how to use a simple Generative Adversarial Network (GAN) to create synthetic data:
import numpy as np from keras.models import Sequential from keras.layers import Dense # Define the generator model def create_generator(): model = Sequential() model.add(Dense(128, activation='relu', input_dim=100)) model.add(Dense(256, activation='relu')) model.add(Dense(512, activation='relu')) model.add(Dense(784, activation='sigmoid')) # For example, generating 28x28 images return model # Train the GAN generator = create_generator() # Assume we have a function to generate random noise and real data # train_gan(generator, noise, real_data) # Generate synthetic data noise = np.random.normal(0, 1, (10, 100)) # 10 random noise vectors synthetic_data = generator.predict(noise)
This GAN example outlines the fundamental structure for generating artificial data, which can be beneficial for simulating astrophysical observations or even for augmenting datasets used in training classifiers.
Real-time Data Processing for Space Exploration
Real-time data processing is an essential component of space exploration, enabling mission teams to respond promptly to telemetry from spacecraft and satellite systems. With the vast amounts of data generated during space missions, Python’s capabilities in real-time processing have become invaluable. By using libraries designed for high performance and effective data handling, engineers can ensure that critical information is not only captured but also analyzed rapidly to inform decision-making.
One of the key libraries employed for real-time data processing in Python is PyData, which includes Pandas for data manipulation and Numpy for numerical operations. These libraries allow teams to create pipelines that can efficiently process incoming data streams, making it feasible to monitor systems continuously. Below is an example illustrating how to set up a real-time data processing loop that reads telemetry data from a socket connection:
import socket import pandas as pd # Set up a socket connection to receive telemetry data sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.connect(('localhost', 5000)) # Function to process incoming telemetry data def process_data(data): # Convert the incoming data to a pandas DataFrame df = pd.DataFrame(data) # Perform any necessary data processing here return df # Continuous data reading loop while True: data = sock.recv(1024) # Receive data from the socket if not data: break # Process the received data processed_data = process_data(data) print(processed_data) sock.close()
This snippet creates a TCP socket that listens for incoming telemetry data. Once data is received, it’s processed and can be analyzed or visualized in real time. The flexibility of Python allows for easy modifications to the process_data function, enabling the addition of specific data analysis tasks like filtering or summarizing telemetry information.
In addition to socket programming, Python’s AsyncIO library is another powerful tool for managing real-time data processing. It facilitates asynchronous programming, allowing the handling of multiple data streams at the same time without blocking operations. That is particularly useful when dealing with multiple sources of telemetry data, such as several sensors on a spacecraft. Here’s an example of how one might implement asynchronous data reading:
import asyncio import websockets import pandas as pd async def read_telemetry(websocket, path): async for message in websocket: # Assume message is a JSON string representing telemetry data data = pd.read_json(message) print(data) async def main(): async with websockets.serve(read_telemetry, 'localhost', 5001): await asyncio.Future() # Run forever # Start the async event loop asyncio.run(main())
In this example, a WebSocket server is created to handle incoming telemetry messages. Each message is processed in real time as it arrives, allowing mission control to react to changes in sensor data immediately. The ability to handle numerous connections and manage data flows asynchronously is a significant advantage for space missions where responsiveness is critical.
Moreover, Python’s integration with data visualization libraries such as Matplotlib and Plotly allows engineers to create real-time visual representations of data. This capability is important for quickly assessing system statuses and making timely adjustments. Below is a simple example of how to update a plot in real time based on incoming telemetry data:
import matplotlib.pyplot as plt import numpy as np # Initialize the plot plt.ion() # Turn on interactive mode fig, ax = plt.subplots() x_data, y_data = [], [] line, = ax.plot(x_data, y_data) # Function to update the plot def update_plot(x, y): x_data.append(x) y_data.append(y) line.set_xdata(x_data) line.set_ydata(y_data) ax.relim() ax.autoscale_view() plt.draw() plt.pause(0.01) # Pause to allow the plot to update # Simulation loop for incoming data for i in range(100): incoming_data = np.random.random() # Simulate incoming telemetry data update_plot(i, incoming_data) # Update the plot with new data
This code initializes a real-time plotting window that updates dynamically with incoming data. Such visualizations are invaluable during mission operations, allowing teams to detect anomalies or trends at a glance.
Case Studies: Python in Recent Space Missions
Python has successfully been employed in various prominent space missions, showcasing its versatility and capability in handling complex tasks across multiple domains of space exploration. One notable example is the Mars Rover missions, particularly the Perseverance rover. The mission team utilized Python for several aspects, including data analysis and control algorithms for autonomous navigation. By using libraries such as NumPy and Matplotlib, they effectively processed the vast amounts of scientific data generated by the rover’s instruments. Below is a simplified example of how data from the rover’s spectrometer can be analyzed using Python:
import pandas as pd import matplotlib.pyplot as plt # Load spectrometer data from a CSV file spectrometer_data = pd.read_csv('spectrometer_data.csv') # Plot the spectral data plt.plot(spectrometer_data['wavelength'], spectrometer_data['intensity']) plt.title('Spectrometer Data from Perseverance Rover') plt.xlabel('Wavelength (nm)') plt.ylabel('Intensity') plt.grid() plt.show()
Another significant application of Python in recent space missions is seen in the European Space Agency’s (ESA) Gaia mission, which aims to map the positions and motions of over a billion stars in our galaxy. The mission scientists used Python extensively for data processing and analysis tasks, given the sheer volume of data involved. Techniques such as parallel processing using the Dask library were employed to handle data efficiently. Here’s an example demonstrating how to use Dask for parallel computation:
import dask.dataframe as dd # Load data using Dask df = dd.read_csv('gaia_stars_data.csv') # Perform a computation, such as filtering and aggregating data filtered_data = df[df['parallax'] > 0.1].groupby('source_id').agg({'brightness': 'mean'}).compute() print(filtered_data.head())
Furthermore, during the Hubble Space Telescope servicing missions, Python played an important role in telemetry data analysis. The mission teams utilized Python scripts to monitor the health of various systems in real time, analyzing data generated during the various stages of the mission. The following code snippet illustrates how to automate the extraction of telemetry data for monitoring:
import requests # Function to fetch telemetry data from a URL def fetch_telemetry(url): response = requests.get(url) telemetry_data = response.json() # Assume the data is in JSON format return telemetry_data # Fetch and display telemetry data url = 'http://hubbletelemetrydata.org/latest' telemetry = fetch_telemetry(url) print(telemetry)
Finally, NASA’s TESS (Transiting Exoplanet Survey Satellite) mission also harnesses Python for processing the light curves of stars. The mission requires efficient handling of time-series data to detect exoplanets through the transit method. Python’s SciPy and AstroPy libraries have been instrumental in this regard. The following code demonstrates a basic approach to analyzing light curves:
import numpy as np from astropy.io import fits import matplotlib.pyplot as plt # Load light curve data from a FITS file with fits.open('tess_light_curve.fits') as hdul: light_curve_data = hdul[1].data time = light_curve_data['TIME'] flux = light_curve_data['FLUX'] # Plotting the light curve plt.plot(time, flux) plt.title('TESS Light Curve Data') plt.xlabel('Time (days)') plt.ylabel('Flux') plt.grid() plt.show()