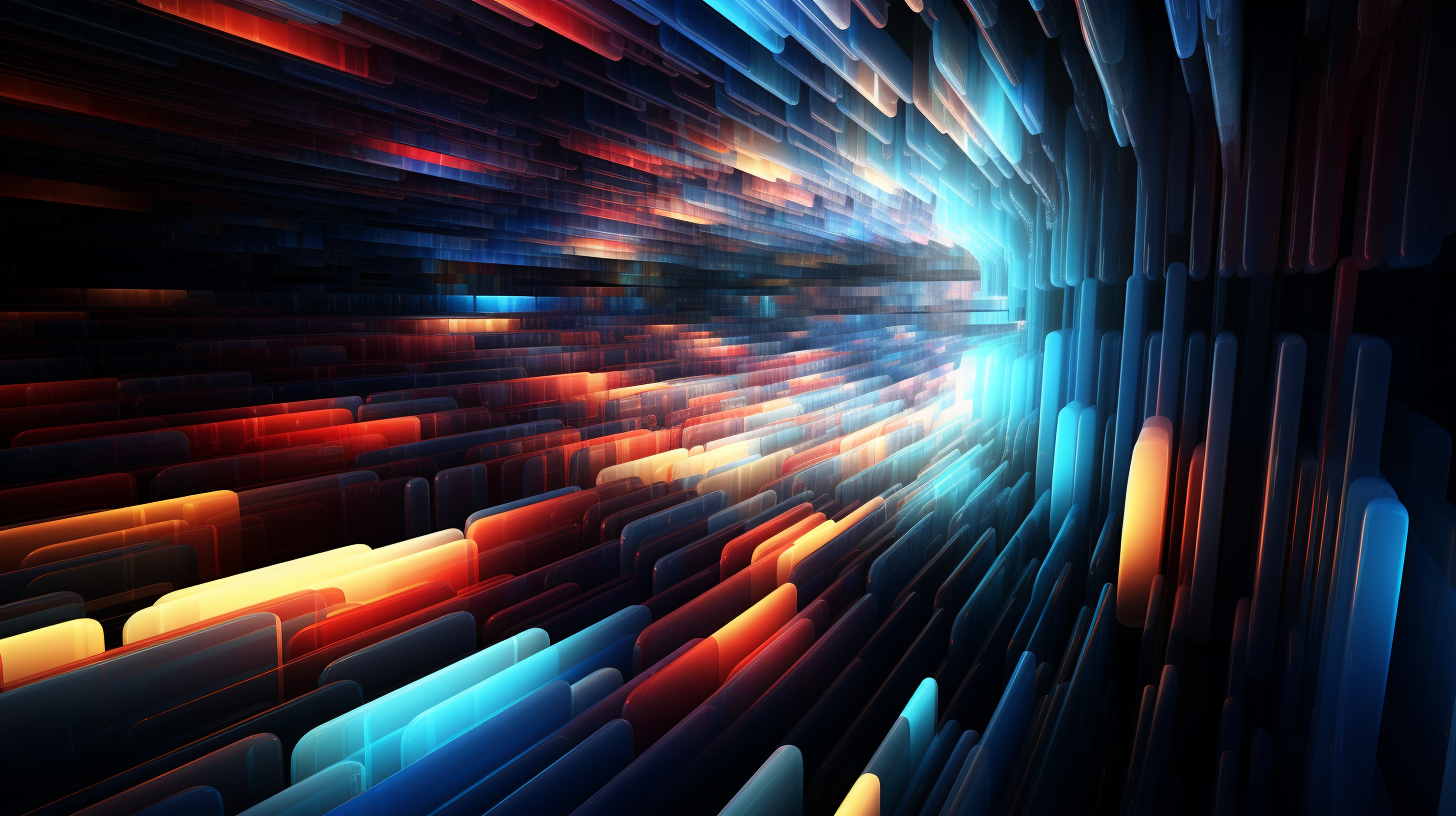
SQL for Spatial Data Analysis
In SQL, spatial data types are specialized data types designed to store geometric and geographic information. These data types enable databases to efficiently handle spatial queries and analyses, which are essential for applications involving maps, locations, and other spatial phenomena.
There are primarily two categories of spatial data types:
- These represent simple geometric shapes, such as points, lines, and polygons. They’re defined in a Cartesian coordinate system.
- These types are used for data that involves real-world locations on the Earth’s surface, represented in latitude and longitude coordinates. They account for the earth’s curvature.
Depending on the SQL database system you’re using, the specific spatial data types may vary. Here are some common spatial data types across popular SQL systems:
- PostGIS (PostgreSQL):
POINT
– Represents a single location in 2D space.LINESTRING
– Represents a sequence of points connected by straight lines.POLYGON
– Defines a polygon with a series of points (vertices).GEOMETRY
– A generic type that can represent any shape.GEOGRAPHY
– Stores geodetic data that accounts for the Earth’s curvature.
- SQL Server:
GEOMETRY
– A data type for flat geometries.GEOGRAPHY
– Used for spatial data on the Earth’s surface.
- MySQL:
POINT
– Represents a point in 2D space.LINESTRING
– Represents a series of connected points.POLYGON
– Represents a polygonal area.GEOMETRY
– A generic type for various shapes.
To create a table with spatial data types in a PostgreSQL database using PostGIS, you might use the following SQL code:
CREATE TABLE locations ( id SERIAL PRIMARY KEY, name VARCHAR(100), geom GEOGRAPHY(Point, 4326) -- Using a geographic point with WGS 84 );
In SQL Server, you can define a spatial table like this:
CREATE TABLE Locations ( Id INT PRIMARY KEY, Name NVARCHAR(100), Location GEOGRAPHY -- Using the geography type for location data );
Spatial Functions and Operators
Spatial functions and operators are essential tools in SQL that allow for the manipulation and analysis of spatial data types. These functions enable users to perform a variety of spatial operations, such as measuring distances, determining intersections, and performing spatial relationships. Mastery of these functions is critical for using the potential of spatial databases.
Different SQL systems have their own sets of spatial functions, often built around their specific spatial data types. Here, we’ll explore some common spatial functions and operators available in PostGIS, SQL Server, and MySQL.
PostGIS Functions
In PostGIS, a wide range of spatial functions can be utilized to perform operations on geometric and geographic types. Some fundamental functions include:
SELECT ST_Distance( ST_MakePoint(1, 1)::geography, ST_MakePoint(2, 2)::geography );
This function calculates the distance between two points on the Earth’s surface, considering the Earth’s curvature due to the use of the geography type.
SELECT ST_Intersects( ST_MakePolygon(ST_MakeLine(ARRAY[ST_MakePoint(0,0), ST_MakePoint(0,1), ST_MakePoint(1,1), ST_MakePoint(1,0), ST_MakePoint(0,0)])), ST_MakePoint(0.5, 0.5) );
The ST_Intersects
function checks if a geometric object intersects with another; in this case, it determines whether a point lies within a polygon.
SQL Server Functions
SQL Server offers similar capabilities through its spatial functions. Here are two essential examples:
DECLARE @point1 GEOGRAPHY = GEOGRAPHY::Point(47.6097, -122.3331, 4326); DECLARE @point2 GEOGRAPHY = GEOGRAPHY::Point(34.0522, -118.2437, 4326); SELECT @point1.STDistance(@point2) AS DistanceInMeters;
This example uses the STDistance
method to calculate the distance between two geographical points in meters.
DECLARE @polygon GEOMETRY = GEOMETRY::STGeomFromText('POLYGON((0 0, 0 1, 1 1, 1 0, 0 0))', 0); DECLARE @point GEOMETRY = GEOMETRY::STGeomFromText('POINT(0.5 0.5)', 0); SELECT @polygon.STContains(@point) AS Contains;
In this case, the STContains
method checks whether a point is contained within a specified polygon.
MySQL Functions
MySQL also provides spatial functions that can be used to work with geometric data. Here are a couple of examples:
SELECT ST_Distance( ST_GeomFromText('POINT(1 1)'), ST_GeomFromText('POINT(2 2)') );
This function call calculates the distance between two points, similar to the PostGIS example.
SELECT ST_Contains( ST_GeomFromText('POLYGON((0 0, 0 1, 1 1, 1 0, 0 0))'), ST_GeomFromText('POINT(0.5 0.5)') );
Here, the ST_Contains
function is used to check if the polygon contains the specified point.
Indexing Spatial Data for Performance
Indexing spatial data especially important for optimizing the performance of spatial queries in SQL databases. Spatial indices enhance query speed, especially when dealing with large datasets or performing complex spatial analyses. Without proper indexing, spatial queries can lead to significant performance bottlenecks, as they may require scanning entire tables to retrieve relevant results.
Different SQL databases implement spatial indexing in unique ways, but the underlying principles remain consistent. Spatial indices typically rely on data structures like R-trees or Quad-trees. These structures allow for efficient querying by spatial partitioning of the data, which minimizes the number of comparisons needed during spatial operations.
In PostgreSQL with PostGIS, creating a spatial index is simpler. You can create a GiST (Generalized Search Tree) index on a spatial column like so:
CREATE INDEX locations_geom_gist ON locations USING GIST (geom);
This command creates a GiST index on the `geom` column of the `locations` table, significantly improving the performance of queries that involve spatial operations.
Similarly, in SQL Server, you can create a spatial index on a geography or geometry column using the following T-SQL command:
CREATE SPATIAL INDEX SIDX_Location ON Locations(Location);
This spatial index, named `SIDX_Location`, will optimize queries that utilize the `Location` column for spatial operations.
MySQL also supports spatial indexing, and you can create a spatial index on a geometry column like this:
ALTER TABLE locations ADD SPATIAL INDEX (geom);
In this example, a spatial index is added to the `geom` column of the `locations` table, improving the efficiency of spatial queries.
It is important to note that while spatial indexing can greatly enhance query performance, it also comes with trade-offs. Indexes consume additional storage space and can impact the performance of data modification operations (INSERT, UPDATE, DELETE) because the index must be maintained. Therefore, it’s essential to analyze your specific use cases and workloads to determine the appropriate indexing strategy.
Spatial Queries: Techniques and Examples
Spatial queries enable the extraction of meaningful information from spatial data types in SQL. These queries utilize a combination of spatial functions, operators, and conditions to analyze data based on spatial relationships. In this section, we will delve into various techniques and examples of spatial queries applicable across different SQL systems. Understanding these techniques is essential for efficiently analyzing geographic and geometric data.
One fundamental principle in spatial querying is using spatial relationships. Common spatial relationships include containment, intersection, proximity, and distance. Depending on the SQL system, the syntax and functions might vary slightly, but the core concepts remain consistent.
In PostGIS, for instance, you can perform a query to find all points within a given distance from a specific location. This can be particularly useful for applications like finding nearby restaurants or services. Here’s an example:
SELECT name FROM locations WHERE ST_DWithin(geom, ST_MakePoint(1, 1)::geography, 500);
This query retrieves all locations whose geometry is within 500 meters of the point (1, 1). The ST_DWithin function is essential here, as it efficiently checks the distance between geometries without calculating the exact distance for every row, using the spatial index if available.
In SQL Server, you might want to check which polygons contain a certain point. For example:
DECLARE @point GEOGRAPHY = GEOGRAPHY::Point(47.6097, -122.3331, 4326); SELECT Id, Name FROM Locations WHERE Location.STContains(@point) = 1;
This query defines a point and checks which locations contain that point using the STContains method, providing a simpler way to filter results based on spatial relationships.
For MySQL users, performing a spatial query to find the nearest geometries can be similarly effective. Here’s a query that retrieves points sorted by distance from a specified location:
SELECT name, ST_Distance(geom, ST_GeomFromText('POINT(1 1)')) AS distance FROM locations ORDER BY distance LIMIT 5;
In this case, the query selects the five nearest locations to the point (1, 1), calculating the distance on-the-fly using ST_Distance, and ordering the results accordingly.
Moreover, spatial queries can also involve aggregations. For example, finding the total area covered by multiple polygons can provide insights into land use and planning:
SELECT SUM(ST_Area(geom)) AS total_area FROM locations WHERE ST_Intersects(geom, ST_MakePolygon(ST_MakeLine(ARRAY[ST_MakePoint(0,0), ST_MakePoint(0,1), ST_MakePoint(1,1), ST_MakePoint(1,0), ST_MakePoint(0,0)])));
This query sums the area of all geometries that intersect with a defined polygon, using the ST_Area function to calculate the area of each geometry. This capability especially important in applications like environmental analysis or urban planning.
Additionally, you can combine spatial data queries with other SQL functionalities, such as grouping and filtering. For instance, in a scenario where you want to categorize points by their proximity to a feature, you can use:
SELECT COUNT(*) AS count, category FROM locations WHERE ST_DWithin(geom, ST_MakePoint(1, 1)::geography, 1000) GROUP BY category;
This query counts how many points from the locations table fall within a 1000-meter radius of the specified point, grouped by their categories. Such queries are instrumental in spatial data analytics, allowing for aggregated insights across spatial datasets.
Integrating SQL with Geographic Information Systems (GIS)
Integrating SQL with Geographic Information Systems (GIS) enhances the capabilities of spatial data analysis by allowing users to leverage SQL’s powerful querying abilities alongside GIS’s rich visualization and mapping features. This integration enables organizations to analyze, visualize, and interact with spatial data more effectively, facilitating informed decision-making in fields such as urban planning, environmental management, and logistics.
Many SQL database systems, such as PostgreSQL with PostGIS, SQL Server, and MySQL, provide built-in functions and support for geospatial data, making it easier to interface with GIS applications. GIS software, like QGIS or ArcGIS, can connect to these databases, enabling users to perform spatial queries directly from their GIS environment.
One of the primary advantages of this integration is the ability to execute complex spatial queries and analyses directly from SQL, allowing GIS users to manipulate large datasets without needing to export data to other formats. Here’s an example of how you can use SQL to select and analyze spatial data in a GIS context:
SELECT name, ST_AsText(geom) AS geometry FROM locations WHERE ST_Intersects(geom, ST_MakePolygon(ST_MakeLine(ARRAY[ ST_MakePoint(-100, 40), ST_MakePoint(-100, 50), ST_MakePoint(-90, 50), ST_MakePoint(-90, 40), ST_MakePoint(-100, 40)]))) = TRUE;
This query retrieves the names and geometries of locations that intersect with a specified polygon. By using the ST_Intersects function, you can quickly filter spatial data based on geographical relationships, which can then be visualized in your GIS application.
Furthermore, GIS tools often allow for the visualization of SQL query results in map form, making spatial patterns and trends more apparent. For instance, once you have retrieved a set of locations based on specific criteria, you can plot these results directly onto a map interface in your GIS application, facilitating a visual analysis of the spatial distribution.
Another key aspect of integrating SQL with GIS is enabling real-time updates and analyses. For example, if you have a table that tracks the movement of assets (like delivery trucks), you can continuously query the database to analyze their locations and assess their proximity to various geographic features or customers:
SELECT truck_id, ST_Distance(geom, ST_MakePoint(customer_long, customer_lat)::geography) AS distance FROM delivery_trucks WHERE ST_DWithin(geom, ST_MakePoint(customer_long, customer_lat)::geography, 5000;
This query calculates the distance of delivery trucks from a specified customer location, filtering those within 5 kilometers. Such analyses can be critical for optimizing delivery routes and improving service efficiency.
In addition to querying, SQL’s ability to handle large datasets complements GIS’s visualization capabilities. When dealing with big data, SQL can preprocess and aggregate spatial information, making it more manageable before feeding it into GIS software for visualization. That is particularly useful in scenarios where data is collected in real-time, such as environmental sensors or traffic monitoring systems.
Best Practices for Spatial Data Management in SQL
Best practices for spatial data management in SQL are essential to ensure that your spatial analyses are efficient, accurate, and scalable. Managing spatial data effectively involves several key considerations that can help optimize performance and maintain data integrity. Below are some of the best practices you should implement when working with spatial data in SQL.
1. Choose the Right Spatial Data Types:
When designing your database schema, it is critical to select appropriate spatial data types that align with your application’s needs. For instance, if you’re dealing with geographic data that requires accounting for the Earth’s curvature, opting for the GEOGRAPHY
type in PostgreSQL or SQL Server is advisable. Conversely, if your data operates in a flat Cartesian plane, the GEOMETRY
type may be more suitable.
CREATE TABLE spatial_data ( id SERIAL PRIMARY KEY, location GEOGRAPHY(Point, 4326) );
2. Implement Spatial Indexing:
As previously discussed, spatial indexing significantly improves query performance. Always create spatial indices on columns that will be involved in spatial queries. This minimizes the amount of data scanned and speeds up query execution. Remember to periodically analyze and update your indices as your data changes, particularly if you have a high volume of updates.
CREATE INDEX spatial_index ON spatial_data USING GIST (location);
3. Normalize Spatial Data:
Normalization is a critical practice in general database management, and it holds true for spatial data as well. Break your data into logical tables to reduce redundancy and maintain data integrity. For example, ponder separating attributes of locations into a different table, linking it with a foreign key to your spatial table.
CREATE TABLE locations ( id SERIAL PRIMARY KEY, name VARCHAR(100) ); CREATE TABLE spatial_locations ( location_id INT REFERENCES locations(id), geom GEOGRAPHY(Point, 4326) );
4. Maintain Data Quality:
Spatial data must be accurate and reliable. Implement validation rules to ensure that the spatial data being inserted meets specific criteria, such as ensuring that geometries are valid or that coordinates fall within expected ranges. Utilize functions like ST_IsValid
in PostGIS to check for valid geometries.
SELECT id, ST_IsValid(geom) AS is_valid FROM spatial_data WHERE NOT ST_IsValid(geom);
5. Optimize Spatial Queries:
When writing spatial queries, aim to limit the processed dataset as early as possible. Use filtering criteria to narrow down your query results before applying spatial functions. This approach minimizes the workload on your database and accelerates query response times. Also, ponder using ST_DWithin
instead of ST_Distance
for proximity checks, as it’s often more efficient.
SELECT name FROM locations WHERE ST_DWithin(location, ST_MakePoint(1, 1)::geography, 500);
6. Monitor Performance:
Regularly monitor the performance of your spatial queries and indexing strategies. Use database profiling tools to analyze query execution plans and identify bottlenecks. Pay attention to the execution time of spatial queries, especially as your dataset grows. Adjust your indexing strategies and query structures accordingly to maintain efficiency.
7. Leverage Batch Processing:
For operations that affect large datasets, such as updates or data migrations, utilize batch processing techniques to minimize database locks and improve performance. This can be particularly useful in high-traffic applications where downtime or slow performance could affect user experience.
BEGIN; UPDATE spatial_data SET geom = ST_SetSRID(ST_MakePoint(new_x, new_y), 4326) WHERE conditions RETURNING id; COMMIT;