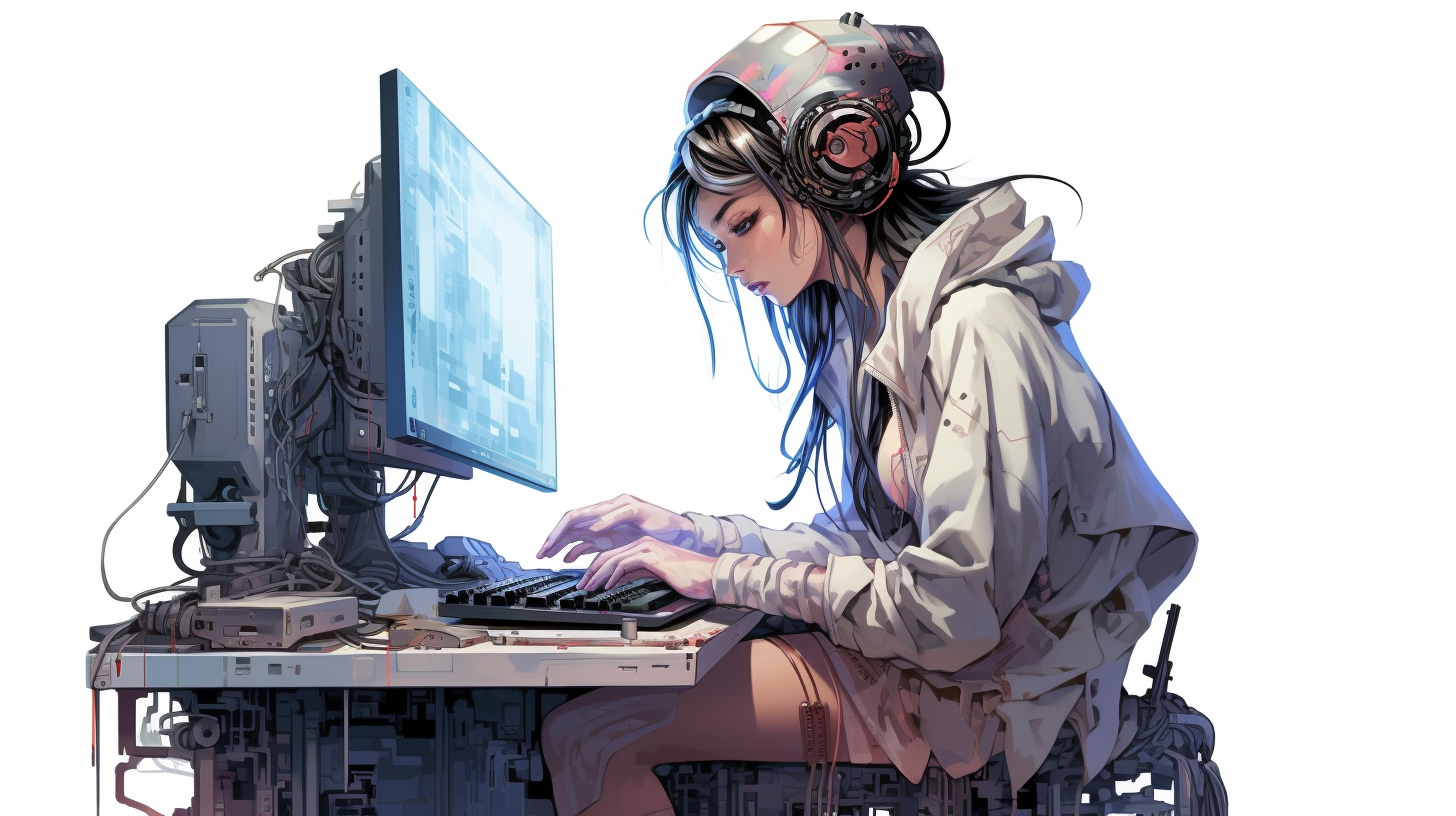
Advanced Bash Command Line Tricks
When it comes to wielding the power of the command line, mastering command substitution and process substitution in Bash is like learning to harness the very essence of your operating system’s capabilities. These features allow you to capture the output of commands and use it in your scripts or commands seamlessly.
Command substitution can be performed using two different syntaxes: the older backticks `command`
and the preferred $(command)
. The latter not only enhances readability but also allows for nesting commands more elegantly. For instance, if you need to count the number of files in a directory, you could write:
file_count=$(ls | wc -l) echo "There are $file_count files in the directory."
This snippet captures the output of the ls
command, pipes it into wc -l
to count the lines, and stores the result in the variable file_count
.
On the other hand, process substitution allows you to treat the output of a command as a file. This is particularly useful when you need to compare or manipulate multiple streams of data. The syntax for process substitution uses /dev/fd/
. For example, if you want to compare the outputs of two commands, you could do something like this:
diff <(sort file1.txt) <(sort file2.txt)
Here, sort file1.txt
and sort file2.txt
are executed in parallel, and their outputs are passed to the diff
command without the need to create temporary files.
Both command substitution and process substitution are invaluable for building more complex Bash scripts that are efficient and easy to read. By mastering these techniques, you can literally transform how you interact with the command line, making your workflows smoother and more powerful.
Using Bash Aliases and Functions for Efficiency
diff <(ls dir1) <(ls dir2)
In this command, the directories dir1 and dir2 are compared by creating temporary files representing their contents, allowing diff to directly operate on these outputs without needing intermediate files. This can save both time and effort, especially when dealing with large data sets or numerous files.
When you’ve grasped the power of command and process substitution, the next step in enhancing your efficiency lies in using Bash aliases and functions. These tools allow you to create shortcuts for your most frequently used commands, transforming complex command sequences into simple, memorable words or phrases.
Creating an alias is simpler. The syntax is as simple as:
alias ll='ls -la'
This command sets up an alias ll that will execute ls -la whenever you type ll. It’s a small change, but it can save you precious keystrokes and help reduce the chance of typos in frequently used commands.
However, aliases have their limits. They can’t handle parameters or complex logic. That’s where functions come into play. A Bash function can be defined with the following syntax:
my_function() { # your commands here }
For example, if you often need to search for a specific string in a file and want to make it more flexible, you could define a function like this:
search_in_file() { grep "$1" "$2" }
Now, whenever you need to search for a term in a file, you can simply call:
search_in_file "search_term" myfile.txt
This function takes two parameters: the search term and the file name. The flexibility allows you to streamline your workflow significantly, reducing repetitive typing and making your command line experience more fluid.
To make your aliases and functions persist across sessions, add them to your .bashrc or .bash_profile files. This way, each new terminal session will automatically have access to your personalized shortcuts, ensuring that you can jump into your workflow without missing a beat.
As your command line skills evolve, you’ll find that these techniques not only improve your efficiency but also help you develop a deeper understanding of Bash scripting. The less you have to ponder about typing out complex commands repeatedly, the more mental bandwidth you’ll have to tackle larger problems, creating a virtuous cycle of productivity and learning.
Advanced Text Processing with awk and sed
diff <(ls dir1) <(ls dir2)
Here, the outputs of the `ls` command for both directories are treated as files by `diff`, so that you can see the differences between the contents of `dir1` and `dir2` in a single, clear comparison.
Moving on to text processing, `awk` and `sed` are two powerful tools in the Bash arsenal. While both are capable of performing text manipulation, each has its own strengths tailored to different tasks. With `awk`, you can easily parse and analyze text files, making it an excellent choice for columnar data. For instance, if you have a CSV file and you want to extract the second column, you can utilize `awk` like this:
awk -F, '{print $2}' file.csv
The `-F,` option specifies that the delimiter is a comma, and `{print $2}` directs `awk` to output the second column. This simple command encapsulates the essence of `awk` — powerful column-based processing with minimal syntax.
On the other hand, `sed` excels in stream editing and can perform complex text manipulations in a single pass. If you need to substitute all occurrences of a word in a file, `sed` makes this straightforward:
sed 's/oldword/newword/g' file.txt
In this command, `s/oldword/newword/g` stands for substitute the word “oldword” with “newword” globally across the file. Its ability to handle regular expressions makes `sed` particularly suited for intricate text processing tasks.
Combining `awk` and `sed` can significantly increase your productivity. For example, suppose you want to modify a log file to extract specific information while also formatting it. You could employ both tools in a pipeline:
cat log.txt | awk '{print $1, $3}' | sed 's/ERROR/WARNING/'
In this pipeline, `cat` outputs the contents of `log.txt`, `awk` extracts the first and third columns, and `sed` replaces instances of “ERROR” with “WARNING”. Such combinations allow for dynamic and efficient data manipulation directly from the command line.
Thus, by mastering the usage of `awk` and `sed`, you can perform advanced text processing tasks with relative ease, enhancing your command line efficiency and opening new avenues for automation and data analysis.
Streamlining Workflow with Job Control and Background Processes
diff <(ls dir1) <(ls dir2)
In this example, the diff
command compares the outputs of two separate ls
commands that list the contents of dir1
and dir2
. By using process substitution, you can effectively treat the outputs as if they were files, allowing for powerful comparisons without the need to create temporary files.
Bash also provides robust job control features that enable you to manage multiple processes efficiently. Understanding how to use jobs and the associated commands can significantly streamline your workflow. When you run a command, it typically runs in the foreground, blocking further input until it completes. However, you can easily run commands in the background by appending an ampersand (&
) to the command:
sleep 30 &
This will initiate the sleep
command in the background, which will allow you to continue working in the same terminal session. To manage these background jobs, you can use the jobs
command to list them, and the fg
or bg
commands to bring them to the foreground or continue them in the background, respectively.
For example, after starting a job in the background, you might want to bring it back to the foreground:
fg %1
Here, %1
refers to the first job listed by the jobs
command. Using these job control features allows you to multitask efficiently right from your terminal.
Another useful feature of Bash is the ability to manage processes through their job IDs. You can suspend a foreground job using Ctrl+Z
, which pauses the process and places it in the background. To resume it later, you can use the bg
command, allowing it to continue running in the background without requiring any further input.
bg %1
Additionally, if you find yourself overburdened with numerous background processes, you can terminate them gracefully using the kill
command followed by the job ID or process ID:
kill %1
This command sends a termination signal to the specified job, so that you can maintain control over your terminal environment.
By mastering job control and background processes in Bash, you not only enhance your productivity but also cultivate a more efficient workflow, making it possible to juggle multiple tasks with ease. Understanding these concepts is akin to wielding a powerful tool that can cut through the chaos of multitasking, so that you can focus on what matters most—solving problems and executing your commands with precision.
Enhancing Scripts with Error Handling and Debugging Techniques
diff <(ls /path/to/dir1) <(ls /path/to/dir2)
In this command, the output of the ls commands for each directory is treated as if it were coming from a file, allowing diff to compare them directly. This can be incredibly useful in situations where you are dealing with data streams that you’d like to analyze or compare without creating intermediate files.
When you’re writing scripts, especially as they grow in complexity, it’s essential to integrate robust error handling and debugging techniques to ensure your code behaves as expected and to facilitate troubleshooting when issues arise. Bash provides several mechanisms for this purpose.
First, using the `set` command can significantly improve your script’s reliability. By including `set -e` at the top of your script, you instruct Bash to exit immediately if any command exits with a non-zero status, effectively halting execution on errors.
#!/bin/bash set -e cp /source/file /destination/file echo "File copied successfully!"
In this script, if the `cp` command fails, the script exits without executing any further commands, preventing potential cascading errors.
For more granular control, you can use conditional constructs to handle errors more gracefully. Ponder this example:
#!/bin/bash cp /source/file /destination/file if [ $? -ne 0 ]; then echo "Error: File copy failed!" >&2 exit 1 fi echo "File copied successfully!"
Here, `$?` captures the exit status of the `cp` command. If it fails, you output an error message to standard error (`>&2`) and exit the script with a non-zero status to indicate failure.
Debugging is another critical aspect of script development. Bash offers a handy debugging feature that can be activated with `set -x`, which prints each command before executing it. This can be invaluable in tracing through your script to see exactly what is happening at each step.
#!/bin/bash set -x cp /source/file /destination/file echo "File copied successfully!"
With `set -x` enabled, every command and its arguments will be displayed in the terminal, so that you can identify where things might be going awry with your script.
Additionally, you can create custom debug functions that enhance your debugging experience. For example, a simple function to log messages could look like this:
log() { echo "$(date +'%Y-%m-%d %H:%M:%S') - $1" } log "Starting file copy process..." cp /source/file /destination/file log "File copy completed!"
In this code, `log` adds timestamps to your messages, which can be very helpful when you’re trying to track the execution flow.
By employing these error handling and debugging techniques, you’ll not only enhance the resilience of your Bash scripts but also cultivate a more efficient workflow, enabling you to address issues with precision and confidence.