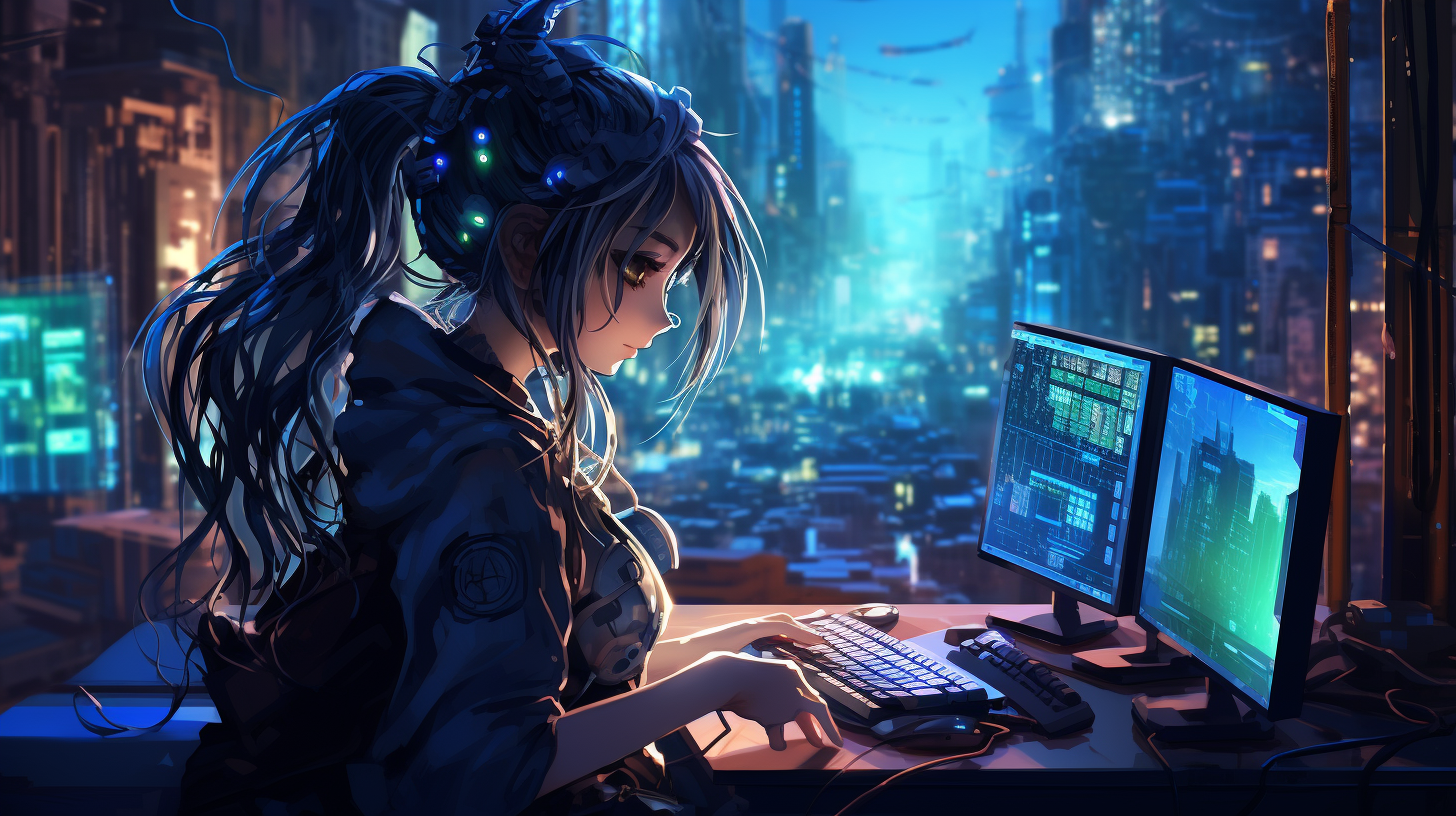
Push Notifications in Swift
Push notifications serve as a powerful means of communication between your app and its users, enabling real-time updates and engagement. In Swift, understanding how these notifications work is key to using their full potential. At their core, push notifications are messages sent from a server to a client device, which can include a variety of content such as alerts, sounds, and badges.
When a push notification is sent, it triggers a process that begins with the Apple Push Notification service (APNs), which acts as the intermediary. APNs securely delivers the message to the target device, ensuring that the notification is handled appropriately by the operating system.
In iOS, push notifications can be categorized into two types: remote notifications and local notifications. Remote notifications are delivered via APNs, while local notifications are triggered by the app itself without the need for a server. Understanding this distinction is essential for implementing the desired functionality in your app.
To utilize push notifications in your Swift application, you must first register your app with APNs and obtain a device token. This token uniquely identifies the device and especially important for sending notifications. Below is an example of how to register for push notifications in Swift:
import UIKit import UserNotifications @main class AppDelegate: UIResponder, UIApplicationDelegate { func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { // Request permission for notifications UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { granted, error in if granted { DispatchQueue.main.async { UIApplication.shared.registerForRemoteNotifications() } } } return true } func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) { let tokenString = deviceToken.map { String(format: "%02.2hhx", $0) }.joined() print("Device Token: (tokenString)") } func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) { print("Failed to register: (error.localizedDescription)") } }
Once you have registered for notifications and received the device token, the next step is to implement the mechanism to send notifications from your server. This requires a solid understanding of how APNs operates, including managing certificates and authentication. With this foundation laid, you can create a seamless flow of information from your server to the app.
Understanding push notifications in Swift is about grasping the lifecycle of a notification—from registration, through the APNs, to delivery and handling. Mastering this flow allows you to create dynamic and responsive applications that keep users engaged.
Setting Up APNs (Apple Push Notification service)
To set up APNs effectively, you must begin by configuring your app through the Apple Developer portal. This involves creating an App ID that has the push notification capability enabled. Once you’ve set up your App ID, the next step is to generate an APNs Key or Certificate. The key is a more modern approach and allows for easier management as you can use it across multiple apps.
After generating your APNs Key, you must download the .p8 file, which contains your key ID and team ID. Keep this file secure, as it is essential for authenticating your push notifications with APNs. You will also need to note down your Key ID, which is used in conjunction with your team ID for server-side authentication.
Once your APNs Key is ready, you can move on to your server-side implementation. The process of sending push notifications involves creating a JSON payload that contains the notification details, such as title, body, and any additional data you want to attach. Below is an example of how to create a simple payload in Swift:
struct NotificationPayload: Codable { let aps: Aps } struct Aps: Codable { let alert: Alert let sound: String } struct Alert: Codable { let title: String let body: String } func createPayload(title: String, body: String) -> Data? { let alert = Alert(title: title, body: body) let aps = Aps(alert: alert, sound: "default") let payload = NotificationPayload(aps: aps) let encoder = JSONEncoder() encoder.outputFormatting = .prettyPrinted do { let jsonData = try encoder.encode(payload) return jsonData } catch { print("Error encoding payload: (error)") return nil } }
Once you have the payload ready, the notification must be sent to APNs via an HTTPS request. It’s crucial to ensure you handle errors appropriately and follow the best practices for making these calls, including retrying on failures. Below is an example of how to send the notification using URLSession:
func sendPushNotification(to deviceToken: String, payload: Data) { let url = URL(string: "https://api.push.apple.com/3/device/(deviceToken)")! var request = URLRequest(url: url) request.httpMethod = "POST" request.httpBody = payload request.setValue("application/json", forHTTPHeaderField: "Content-Type") request.setValue("Bearer YOUR_JWT_TOKEN", forHTTPHeaderField: "Authorization") // Use your JWT here let task = URLSession.shared.dataTask(with: request) { data, response, error in if let error = error { print("Error sending push notification: (error.localizedDescription)") return } if let httpResponse = response as? HTTPURLResponse, httpResponse.statusCode != 200 { print("Failed with HTTP status code: (httpResponse.statusCode)") } else { print("Push notification sent successfully!") } } task.resume() }
With the configuration complete and the ability to send notifications established, you can now focus on the integration of these notifications within your app. Understanding how APNs communicates with your server and how to manage the lifecycle of your push notifications forms the backbone of a robust notification system.
Configuring Your App for Push Notifications
To configure your app for push notifications in Swift, you need to set up specific settings both in your app’s Xcode project and in the Apple Developer portal. This process ensures that your application can properly handle incoming notifications and engage effectively with users.
Start by navigating to your app’s target in Xcode. Under the “Signing & Capabilities” tab, enable the “Push Notifications” capability. This allows your app to receive remote notifications through APNs. You should also ensure that your app has a unique App ID, which can be configured through the Apple Developer portal.
Once you’ve enabled the capability in Xcode, the next step is to set up the necessary entitlements. This involves creating an “App ID” that specifically has the “Push Notifications” service enabled. Within the Apple Developer portal, locate your App ID, and ensure that under the “Capabilities” section, the Push Notifications option is properly checked.
After you have your App ID configured, you will need to create an APNs Key or certificate. Using an APNs Key is generally recommended as it simplifies the process of managing push notifications across multiple applications. To create the APNs Key, go to the Certificates, Identifiers & Profiles section of your Apple Developer account, select “Keys,” and then click on the “+” icon to create a new key.
Give your key a name and enable the APNs service. Once you create the key, download the .p8 file. This file contains your key ID, which you will need for server-side communication with APNs. Make sure to store this file securely, as it is critical for your push notification implementation.
In addition to the .p8 file, note down your Key ID and your Team ID, as these will be needed to generate a JSON Web Token (JWT) used for authenticating your requests to APNs. Below is an example of how to generate a JWT in Swift:
import JWTKit func generateJWT() throws -> String { let jwtSigner = JWTSigner.hs256(key: "YOUR_SECRET_KEY_HERE") // Replace with your key let jwt = try jwtSigner.sign([ "iss": "YOUR_TEAM_ID", "iat": Date().timeIntervalSince1970, "exp": Date().addingTimeInterval(60 * 20).timeIntervalSince1970, // Token valid for 20 minutes "aud": "appstoreconnect-v1" ]) return jwt }
Once you have your push notifications configured and your JWT generation logic in place, the next step is to implement the registering process for remote notifications in your app code. That’s typically done within the AppDelegate class. As previously shown, you need to request permission to display alerts, badges, and sounds. If granted, you register for remote notifications as follows:
import UserNotifications func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { granted, error in if granted { DispatchQueue.main.async { UIApplication.shared.registerForRemoteNotifications() } } else { print("User did not grant permission: (error?.localizedDescription ?? "Unknown error")") } } return true }
By ensuring that your app is properly configured for push notifications, you set the stage for receiving and handling notifications effectively. This configuration not only involves enabling the necessary services in Xcode and the Apple Developer portal but also establishing secure authentication mechanisms for communicating with APNs. With this groundwork laid, your application will be well-prepared to engage users with timely and relevant notifications.
Handling Push Notifications in the App
Once the app is configured and registered with APNs, the next critical step is handling incoming push notifications when they arrive. This involves implementing methods to respond appropriately, depending on the state of the app at the time the notification is received—whether the app is in the foreground, background, or not running at all.
To handle push notifications, you will primarily work with the UNUserNotificationCenterDelegate
protocol, which provides methods to respond to incoming notifications. First, you need to set your AppDelegate (or another class) as the delegate for the UNUserNotificationCenter
.
import UserNotifications @main class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate { func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { UNUserNotificationCenter.current().delegate = self requestNotificationAuthorization() return true } private func requestNotificationAuthorization() { UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { granted, error in if granted { DispatchQueue.main.async { UIApplication.shared.registerForRemoteNotifications() } } } } }
With the delegate set, you can now implement methods to handle notifications. The userNotificationCenter(_:didReceive:withCompletionHandler:)
method is called when a notification is delivered while the app is in the foreground. Here, you can customize how your app responds to notifications.
extension AppDelegate { func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { let userInfo = response.notification.request.content.userInfo handleNotification(userInfo: userInfo) completionHandler() } func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) { // Show the notification alert while the app is in the foreground completionHandler([.alert, .badge, .sound]) } private func handleNotification(userInfo: [AnyHashable: Any]) { // Handle the notification content here print("Received notification with userInfo: (userInfo)") } }
When the user interacts with a notification (by tapping it), the userNotificationCenter(_:didReceive:withCompletionHandler:)
method is invoked. That’s where you can extract the information from the notification and perform any necessary actions within your app, such as navigating to a specific screen or updating the UI.
In addition to handling notifications, you may also want to implement additional customizations for notification appearance. This includes setting notification categories and actions, which allow users to respond directly to notifications. Categories can be configured within your app and registered with the notification center.
func setupNotificationCategories() { let replyAction = UNTextInputNotificationAction(identifier: "REPLY_ACTION", title: "Reply", options: [.foreground], textInputButtonTitle: "Send", textInputPlaceholder: "Type your message") let category = UNNotificationCategory(identifier: "MESSAGE_CATEGORY", actions: [replyAction], intentIdentifiers: [], options: []) UNUserNotificationCenter.current().setNotificationCategories([category]) }
Make sure to call setupNotificationCategories()
during the app’s launch phase to ensure that your categories are registered. Then, when you create your notification payload on the server, you can specify the appropriate category identifier.
By effectively managing push notifications in your app, you can not only deliver informative messages to users but also create a more engaging and interactive experience. Handling notifications appropriately based on the app state allows for a seamless flow of information, maintaining user attention and enhancing overall app usability.
User Notifications Framework
The User Notifications Framework (UserNotifications) in iOS provides a powerful interface for managing the delivery and handling of push notifications. It allows developers to handle notifications in a way that reflects the rich interactions users expect from modern applications. To leverage this framework effectively, you must understand its architecture and how it modifies the standard notification behavior.
At the heart of the User Notifications Framework are the UNUserNotificationCenter and UNNotificationRequest classes. The UNUserNotificationCenter is the central point for managing notifications in your app, and it acts as the bridge between your app and the system notification services. You can use this class to schedule notifications, check notification settings, and manage notification categories.
To begin using the User Notifications Framework, you first need to import the UserNotifications module and ensure that your app is properly configured to send and receive notifications. After you’ve done that, you can create and schedule notifications. Here’s how you can create a basic notification request:
import UserNotifications func scheduleNotification() { let content = UNMutableNotificationContent() content.title = "Hello!" content.body = "This is a test notification." content.sound = UNNotificationSound.default // Create a trigger to fire the notification after 5 seconds let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 5, repeats: false) // Create the request let request = UNNotificationRequest(identifier: UUID().uuidString, content: content, trigger: trigger) // Schedule the request with the user notification center UNUserNotificationCenter.current().add(request) { error in if let error = error { print("Error scheduling notification: (error.localizedDescription)") } } }
This function constructs a notification with a title, body, and sound, then schedules it to trigger after a specified interval. The identifier is a unique string that allows you to reference this notification later if needed, for example, to cancel it or update its content.
Additionally, you can customize notifications by defining categories and actions that can be presented to the user. Categories help group related notifications and can include actions that users can perform directly from the notification interface. For instance, you might want to allow users to reply to a message directly via a notification. Below is an example of how to set up a notification category with an action:
func setupNotificationActions() { let replyAction = UNTextInputNotificationAction(identifier: "REPLY_ACTION", title: "Reply", options: [.foreground], textInputButtonTitle: "Send", textInputPlaceholder: "Type your reply") let category = UNNotificationCategory(identifier: "MESSAGE_CATEGORY", actions: [replyAction], intentIdentifiers: [], options: []) UNUserNotificationCenter.current().setNotificationCategories([category]) }
Once you have defined your notification categories, ensure you set them up early in your app’s lifecycle, typically in the AppDelegate’s didFinishLaunchingWithOptions method. Then, when you create your notification content, you can assign the category identifier:
content.categoryIdentifier = "MESSAGE_CATEGORY"
As users interact with notifications, your app can handle those interactions through the delegate methods of UNUserNotificationCenterDelegate. This allows you to respond to user actions based on how they interact with the notification, whether by tapping it or performing a custom action. For example, you can handle the reply action like so:
extension AppDelegate: UNUserNotificationCenterDelegate { func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { let userInfo = response.notification.request.content.userInfo if response.actionIdentifier == "REPLY_ACTION", let textResponse = response as? UNTextInputNotificationResponse { let userReply = textResponse.userText // Handle the reply text print("User replied with: (userReply)") } completionHandler() } }
This method checks if the user interacted with the reply action and captures the reply text. You can then process this information in your app, perhaps sending it back to a server or displaying it in the UI.
By using the User Notifications Framework effectively, you create a rich user experience that enhances interaction with your app through timely and relevant notifications. Customizing the appearance and actions of notifications not only keeps users informed but also encourages engagement by allowing them to respond directly from the notification interface, promoting a seamless flow of interaction between your app and its users.
Best Practices for Push Notifications
When implementing push notifications in a Swift application, adhering to best practices especially important for ensuring a positive user experience and maximizing engagement. Here are several recommendations to keep in mind:
1. Be Selective with Notifications: It’s essential to avoid bombarding users with notifications. Instead, be strategic about when and how often you send them. Send notifications that provide real value, such as important updates or reminders that enhance the user experience. Analyze user behavior and preferences to determine the most effective moments for engagement.
2. Personalization: Tailoring notifications to individual users can significantly improve engagement rates. Utilize data such as user preferences, behavior patterns, and interactions within the app to craft personalized messages. This could involve sending location-based notifications or reminders about features the user has shown interest in.
3. Use Rich Notifications: Enhance notifications by incorporating multimedia elements such as images, videos, or action buttons. Rich notifications capture users’ attention more effectively than standard text-only notifications. This can be achieved using the UNNotificationContent
class to include attachments or custom actions.
import UserNotifications func createRichNotification() { let content = UNMutableNotificationContent() content.title = "New Message!" content.body = "You've received a new message." content.sound = UNNotificationSound.default // Adding an image attachment if let attachment = try? UNNotificationAttachment(identifier: "image", url: imageURL, options: nil) { content.attachments = [attachment] } let request = UNNotificationRequest(identifier: UUID().uuidString, content: content, trigger: nil) UNUserNotificationCenter.current().add(request) { error in if let error = error { print("Error scheduling rich notification: (error.localizedDescription)") } } }
4. Implement User Controls: Allow users to manage their notification preferences easily. Provide settings within your app where users can specify what types of notifications they want to receive and how they want to be notified (e.g., sound, badge, alerts). This transparency builds trust and encourages users to stay engaged.
5. A/B Testing: Just as critical as selecting when and what to notify users about, testing different messages, timings, and formats can provide valuable insights. Use A/B testing to compare engagement rates between different notification strategies, adjusting your approach based on real user feedback.
6. Handle User Interaction Gracefully: When users interact with notifications, ensure that the app responds in a relevant manner. For example, if a user taps on a notification to view a message, the app should navigate them directly to that message instead of a generic home screen. This facilitates a smoother user experience.
extension AppDelegate: UNUserNotificationCenterDelegate { func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { // Navigate to the appropriate screen based on notification type if response.notification.request.identifier == "MESSAGE_NOTIFICATION" { navigateToMessageScreen() } completionHandler() } private func navigateToMessageScreen() { // Implement app navigation logic here print("Navigating to message screen.") } }
7. Respect User Privacy: Ensure that you respect users’ privacy and adhere to regulations such as GDPR. Make it clear what data you collect and how it will be used in relation to notifications. Give users the option to opt out of non-essential notifications.
8. Monitor Performance: Keep tabs on the performance of your push notifications through analytics. Evaluate metrics such as open rates, click-through rates, and user retention to assess the effectiveness of your notification strategies. Continuous monitoring allows you to refine your approach over time.
By following these best practices, you can enhance the effectiveness of your push notifications in Swift, fostering a more meaningful connection with users and driving engagement within your application.