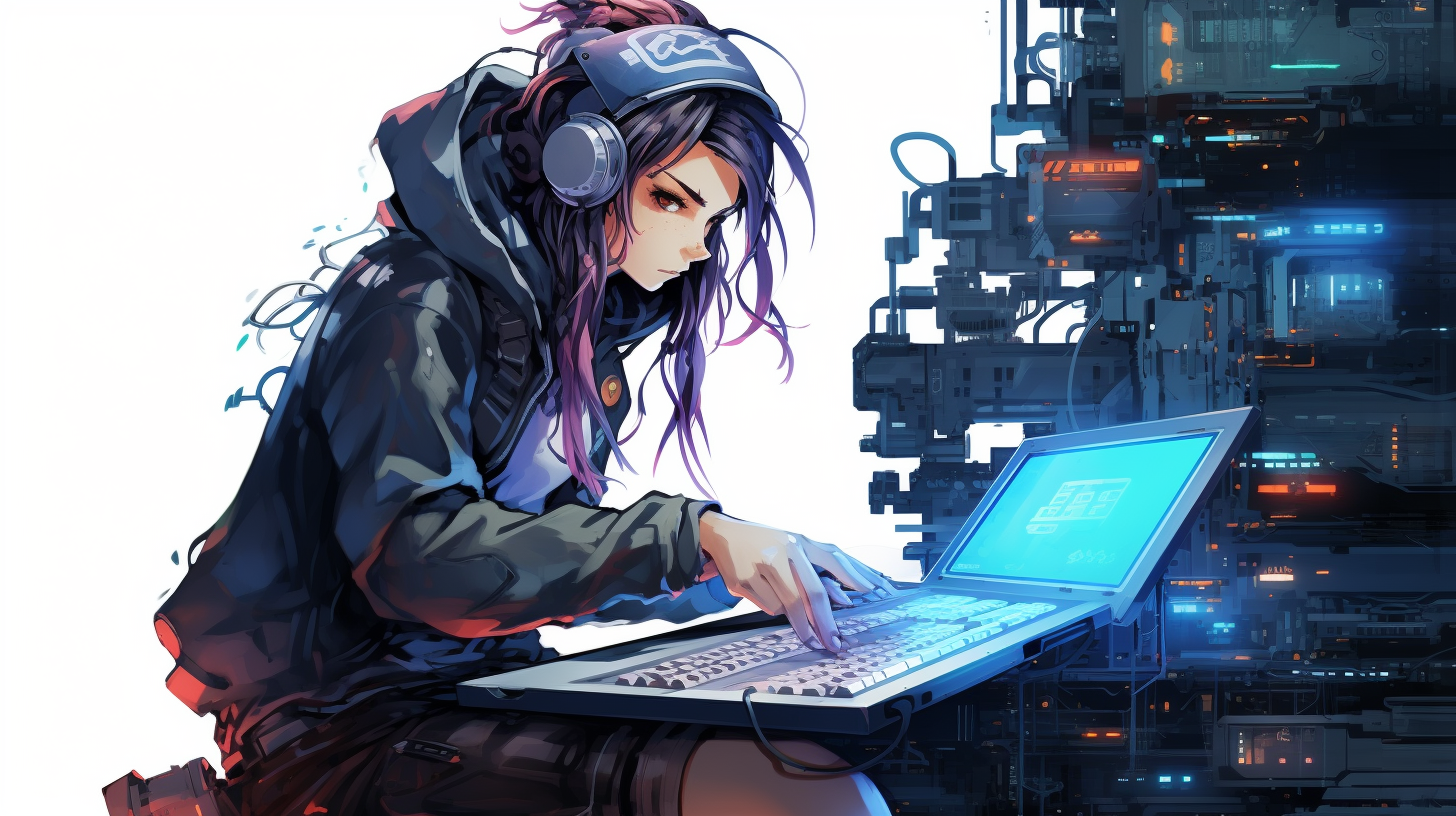
Swift and Core Data Advanced Techniques
Configuring and managing the Core Data stack is a pivotal step in using the full power of the framework. A well-structured Core Data stack forms the backbone of your data management strategy and directly influences the performance and efficiency of your app. The stack primarily consists of three components: the managed object model, the persistent store coordinator, and the managed object context.
The managed object model is where you define your data structure. Using the NSManagedObjectModel class, you can create entities, attributes, and relationships visually using Xcode’s data model editor or programmatically through code.
let modelURL = Bundle.main.url(forResource: "MyDataModel", withExtension: "momd")! let managedObjectModel = NSManagedObjectModel(contentsOf: modelURL)
The persistent store coordinator manages the persistent stores and coordinates access to them. It acts as a bridge between the managed object context and the underlying data storage. Here’s how you can initialize the persistent store coordinator:
let persistentStoreCoordinator = NSPersistentStoreCoordinator(managedObjectModel: managedObjectModel) let storeURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!.appendingPathComponent("MyData.sqlite") do { try persistentStoreCoordinator.addPersistentStore(ofType: NSSQLiteStoreType, configurationName: nil, at: storeURL, options: nil) } catch { fatalError("Persistent store error: (error)") }
The managed object context is where you interact with your data. It’s essential for creating, deleting, and managing the lifecycle of your managed objects. You can establish a main context, typically used on the main thread for UI updates, as follows:
let managedObjectContext = NSManagedObjectContext(concurrencyType: .mainQueueConcurrencyType) managedObjectContext.persistentStoreCoordinator = persistentStoreCoordinator
For background tasks, you might want to create private contexts. This allows you to perform operations without blocking the main thread, enhancing the responsiveness of your app:
let backgroundContext = NSManagedObjectContext(concurrencyType: .privateQueueConcurrencyType) backgroundContext.persistentStoreCoordinator = persistentStoreCoordinator
When you modify objects in Core Data, you must save your context to persist changes. Always remember to handle potential errors during saving:
do { try managedObjectContext.save() } catch { // Handle error print("Failed to save context: (error)") }
Configuring your Core Data stack requires careful attention to each of its components. Proper initialization and management of your managed object context, persistent store coordinator, and managed object model not only ensures data integrity but also lays the groundwork for advanced data handling techniques in your Swift applications.
Optimizing Fetch Requests for Performance
When dealing with Core Data, an often overlooked but critical aspect is optimizing fetch requests to ensure efficient data retrieval. Fetch requests are the primary way to obtain data from the persistent store, and optimizing them can significantly impact your application’s performance, especially as your data grows. Here are several strategies to improve the efficiency of your fetch requests.
Limit the Data Retrieved
One of the simplest yet most effective strategies is to limit the amount of data returned by your fetch requests. Use the fetchLimit
property to restrict the number of objects fetched:
let fetchRequest = NSFetchRequest(entityName: "EntityName") fetchRequest.fetchLimit = 50 // Only fetch 50 results
This can be particularly useful in situations like implementing pagination or when you need only the most recent entries.
Use Predicates Wisely
NSPredicate is a powerful tool for filtering results, but complex predicates can slow down fetch requests. Make sure to filter your data as narrowly as possible to reduce the workload on the fetch request:
let predicate = NSPredicate(format: "attributeName == %@", "value") fetchRequest.predicate = predicate
A well-optimized predicate can minimize the number of objects retrieved and thus improve performance significantly.
Sort Results Efficiently
Sorting your results appropriately can also affect fetch performance. If your fetch request involves sorting, ensure that the sorting attributes are indexed. You can specify sort descriptors as follows:
let sortDescriptor = NSSortDescriptor(key: "attributeName", ascending: true) fetchRequest.sortDescriptors = [sortDescriptor]
Using indexed fields for sorting allows Core Data to perform faster lookups, thus accelerating your fetch requests.
Batching Fetch Requests
Batching allows you to reduce memory usage and improve performance when dealing with large datasets. By using the fetchBatchSize
property, you can specify the number of objects to be loaded into memory at a time:
fetchRequest.fetchBatchSize = 20 // Load 20 objects at a time
This approach allows you to work with large datasets without overwhelming memory, enhancing the overall user experience.
Asynchronous Fetching
To maintain a responsive UI, consider using asynchronous fetching. This can be accomplished with NSAsynchronousFetchRequest
, which fetches data in the background and returns the results in a completion handler:
let asyncFetchRequest = NSAsynchronousFetchRequest(fetchRequest: fetchRequest) { result in guard let objects = result.finalResult else { return } // Handle fetched objects } do { try managedObjectContext.execute(asyncFetchRequest) } catch { print("Fetch error: (error)") }
This technique prevents blocking the main thread, ensuring that your app remains responsive even during extensive data operations.
Fetch Caching
Lastly, using fetch caching can significantly improve performance for frequently executed fetch requests. By using the NSFetchRequestResultType
and enabling caching, you can avoid repeated database hits:
fetchRequest.returnsObjectsAsFaults = false // Cache the fetched objects
By implementing these optimization strategies, you will enhance the performance of your Core Data fetch requests. The result is not just faster data retrieval, but also a smoother experience for your users, which is the ultimate goal of any application.
Implementing Complex Relationships and Data Modeling
Implementing complex relationships and data modeling in Core Data is essential for creating a robust data architecture that reflects the real-world relationships within your application’s domain. Core Data allows for the establishment of various relationship types, such as one-to-one, one-to-many, and many-to-many, allowing you to model your data accurately and efficiently. To illustrate these concepts, let’s explore how to define these relationships, manage them within your data model, and utilize them effectively in your Swift code.
When defining relationships in your data model, you can use Xcode’s visual data model editor or configure them programmatically using the NSManagedObjectModel
class. Relationships can be unidirectional or bidirectional. For instance, if you have two entities, Author and Book, you might define a one-to-many relationship where one author can write multiple books:
let authorEntity = NSEntityDescription.entity(forEntityName: "Author", in: managedObjectContext)! let bookEntity = NSEntityDescription.entity(forEntityName: "Book", in: managedObjectContext)! let authorToBooksRelationship = NSRelationshipDescription() authorToBooksRelationship.name = "books" authorToBooksRelationship.destinationEntity = bookEntity authorToBooksRelationship.isToMany = true authorToBooksRelationship.minCount = 0 authorToBooksRelationship.maxCount = 0 // No limit authorToBooksRelationship.inverseRelationship = NSRelationshipDescription() // Define inverse if needed
Once your relationships are defined, you can create instances of these entities and establish the relationships in your Swift code seamlessly. To create an author and a book while linking them, you would proceed as follows:
let newAuthor = Author(context: managedObjectContext) newAuthor.name = "Mitch Carter" let newBook1 = Book(context: managedObjectContext) newBook1.title = "First Book" newBook1.author = newAuthor let newBook2 = Book(context: managedObjectContext) newBook2.title = "Second Book" newBook2.author = newAuthor // Save the context to persist changes do { try managedObjectContext.save() } catch { print("Failed to save context: (error)") }
In this example, newAuthor is associated with two books: newBook1 and newBook2. Notice how the author property of each book is set to link back to the Author instance. This showcases the bidirectional nature of relationships in Core Data.
When dealing with many-to-many relationships, the approach is similar but requires an intermediary entity to manage the relationships. For instance, if both Authors and Books can have multiple connections, you might define a CoAuthorship entity that links the two:
let coAuthorshipEntity = NSEntityDescription.entity(forEntityName: "CoAuthorship", in: managedObjectContext)! let coAuthorshipRelationship = NSRelationshipDescription() coAuthorshipRelationship.name = "coAuthors" coAuthorshipRelationship.destinationEntity = authorEntity coAuthorshipRelationship.isToMany = true
By managing relationships effectively, you can retrieve related data simply and intuitively. For example, to fetch all books written by a specific author, you might leverage a fetch request along with a predicate:
let fetchRequest = NSFetchRequest(entityName: "Book") fetchRequest.predicate = NSPredicate(format: "author.name == %@", "Frank McKinnon") do { let booksByJohnDoe = try managedObjectContext.fetch(fetchRequest) print("Books by Luke Douglas: (booksByJohnDoe.map { $0.title })") } catch { print("Fetch error: (error)") }
Complex data modeling in Core Data also requires careful consideration of performance implications. Be mindful of circular references in relationships, as they can complicate data fetching and lead to performance bottlenecks. Using weak references in the context of relationships can help alleviate these issues.
Implementing complex relationships and data modeling in Core Data not only provides a foundation for representing your data accurately but also improves the efficiency of data management and retrieval in your applications. Understanding these relationships is essential for any Swift developer looking to harness the power of Core Data effectively.
Using NSPredicate and NSFetchedResultsController Effectively
NSPredicate and NSFetchedResultsController are two powerful tools in Core Data that help you manage and retrieve data efficiently while providing a responsive user experience. By understanding how to use these components effectively, you can optimize your app’s data handling capabilities significantly.
NSPredicate is primarily used to filter and specify conditions for fetching data from your persistent store. It provides a flexible way to define search criteria that can be easily modified at runtime, allowing for dynamic queries based on user input or other factors. Creating an NSPredicate can be as simpler as using a format string. Here’s a simple example:
let predicate = NSPredicate(format: "age >= %d", 18)
This predicate filters results to include only those with an age of 18 or older. You can combine predicates using logical operators to create more complex queries:
let combinedPredicate = NSCompoundPredicate(orPredicateWithSubpredicates: [NSPredicate(format: "firstName == %@", "John"), NSPredicate(format: "lastName == %@", "Doe")])
Using predicates effectively allows you to narrow down your fetch results, reducing the amount of data processed and speeding up your queries.
On the other hand, NSFetchedResultsController is designed to manage the results returned from a fetch request. It not only helps in organizing your data but also provides automatic updates to your user interface when the underlying data changes. This is particularly useful in table views, where you want to display data that may change over time. Here’s how to set up an NSFetchedResultsController:
let fetchRequest: NSFetchRequest = NSFetchRequest(entityName: "EntityName") fetchRequest.predicate = predicate fetchRequest.sortDescriptors = [NSSortDescriptor(key: "attributeName", ascending: true)] let fetchedResultsController = NSFetchedResultsController(fetchRequest: fetchRequest, managedObjectContext: managedObjectContext, sectionNameKeyPath: nil, cacheName: nil)
After initializing the fetched results controller, you must perform the fetch operation to retrieve the data:
do { try fetchedResultsController.performFetch() } catch { print("Failed to perform fetch: (error)") }
To stay informed of changes to the data, implement the NSFetchedResultsControllerDelegate protocol. This allows your UI to react to updates in the underlying data:
extension YourViewController: NSFetchedResultsControllerDelegate { func controllerWillChangeContent(_ controller: NSFetchedResultsController) { tableView.beginUpdates() } func controllerDidChangeContent(_ controller: NSFetchedResultsController) { tableView.endUpdates() } func controller(_ controller: NSFetchedResultsController, didChange anObject: Any, at indexPath: IndexPath?, for type: NSFetchedResultsChangeType, newIndexPath: IndexPath?) { switch type { case .insert: if let newIndexPath = newIndexPath { tableView.insertRows(at: [newIndexPath], with: .fade) } case .delete: if let indexPath = indexPath { tableView.deleteRows(at: [indexPath], with: .fade) } case .update: if let indexPath = indexPath { tableView.reloadRows(at: [indexPath], with: .fade) } case .move: if let indexPath = indexPath, let newIndexPath = newIndexPath { tableView.moveRow(at: indexPath, to: newIndexPath) } @unknown default: fatalError("Unknown change type") } } }
This setup ensures that your table view reflects the latest state of the data without requiring explicit reloads. The combination of NSPredicate for targeted fetching and NSFetchedResultsController for automatic updates allows for a clean, efficient data management strategy in your Swift applications.
By mastering NSPredicate and NSFetchedResultsController, you can create responsive, data-driven interfaces that enhance user engagement and streamline the data handling process in your Core Data-based applications.
Error Handling and Data Migration Strategies in Core Data
Error handling and data migration are critical aspects of working with Core Data that ensure your applications remain robust and functional amid changes to the data model or unexpected runtime issues. Handling errors gracefully can significantly enhance user experience, while effective migration strategies prevent data loss during updates.
When working with Core Data, it’s imperative to manage errors that may occur during operations such as fetching, saving, or deleting managed objects. Core Data operations can throw various errors, and being prepared to handle them appropriately is essential. For instance, when saving a context, it’s vital to anticipate problems that could arise, such as validation errors or issues with data integrity. Here’s how you can implement error handling when attempting to save a managed object context:
do { try managedObjectContext.save() } catch let error as NSError { // Handle specific error codes switch error.code { case NSManagedObjectSaveError: print("Failed to save context: (error.localizedDescription)") default: print("An unexpected error occurred: (error.localizedDescription)") } }
This example demonstrates how to catch errors and provide meaningful feedback, which can be beneficial during development and in production environments. Logging errors or showing alerts can inform users of issues while maintaining application stability.
Data migration strategies become essential when you modify your Core Data model, such as adding new attributes or changing the data types of existing ones. Core Data provides several migration options, including lightweight and heavyweight migration. Lightweight migration is suitable for simple changes and can be enabled with minimal configuration:
let options = [NSMigratePersistentStoresAutomaticallyOption: true, NSInferMappingModelAutomaticallyOption: true] do { try persistentStoreCoordinator.addPersistentStore(ofType: NSSQLiteStoreType, configurationName: nil, at: storeURL, options: options) } catch { fatalError("Failed to add persistent store: (error)") }
In the above code, enabling the options for automatic migration allows Core Data to infer changes in the model and apply necessary migration steps seamlessly. However, if your model changes are more complex, you may need to create a mapping model that explicitly defines how data should be transformed during migration.
When implementing a custom mapping model, you can specify how to handle attributes that may have changed. It involves creating an instance of NSMappingModel
and defining source and destination entities. For instance, if you changed an attribute type from String to Integer, you would outline this transformation in your mapping model. Here’s a simplified example of defining a mapping model programmatically:
let mappingModel = NSMappingModel(from: [sourceModel, destinationModel]) mappingModel.addEntityMapping(entityMapping) do { try NSMigrationManager(sourceModel: sourceModel, destinationModel: destinationModel) .migrateStore(from: sourceStoreURL, destinationURL: destinationStoreURL, sourceType: NSSQLiteStoreType, destinationType: NSSQLiteStoreType, options: nil) } catch { fatalError("Migration failed: (error)") }
This more advanced migration process allows you to customize how data transitions from one model version to another, ensuring that your application retains data integrity even as your data schema evolves.
Effectively handling errors and implementing robust migration strategies are vital parts of Core Data management in any Swift application. By ensuring that your app can gracefully deal with errors and smoothly transition through model changes, you significantly enhance its reliability and user satisfaction.