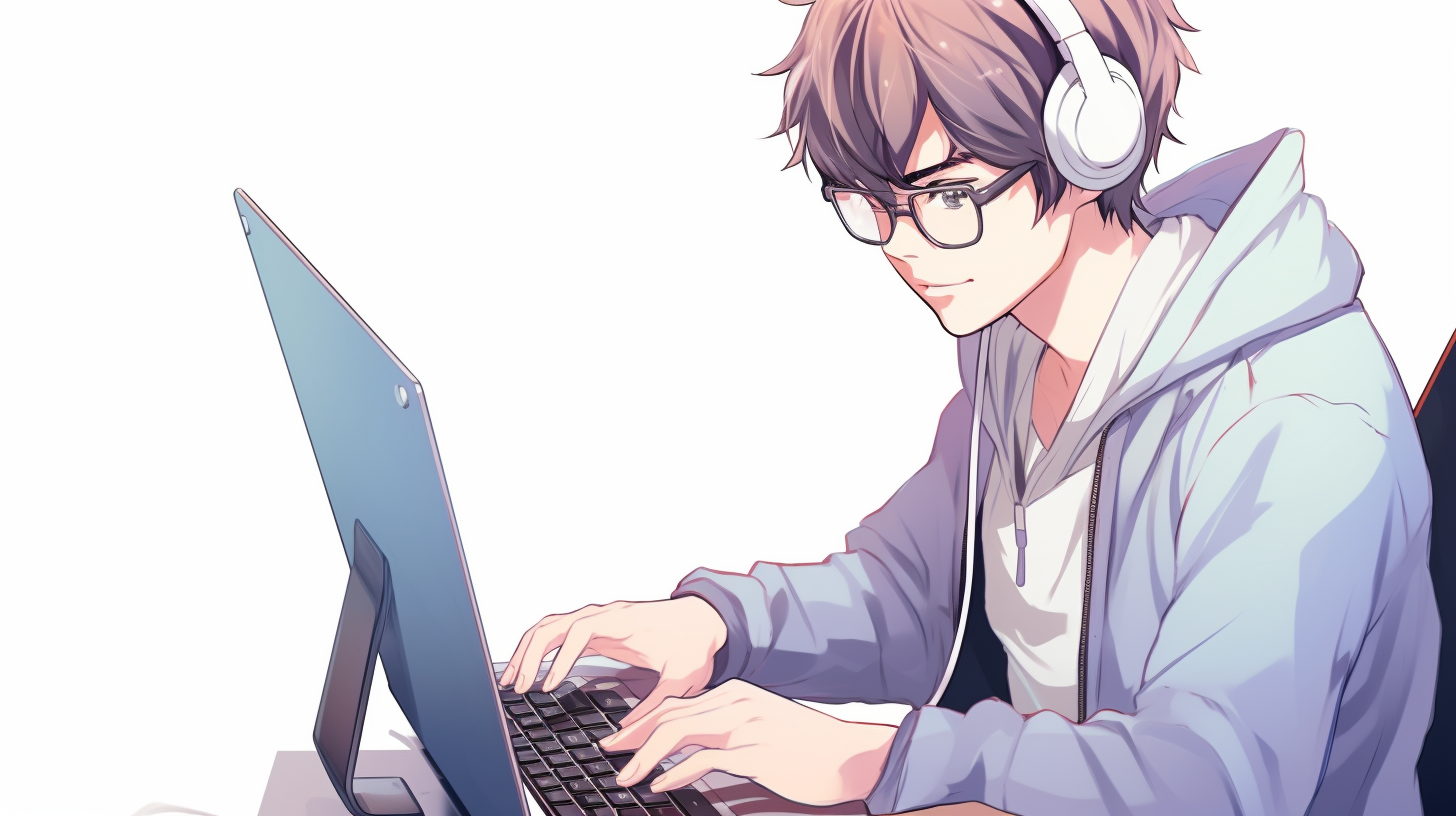
Java and Elasticsearch: Search and Analytics
Elasticsearch is a distributed, RESTful search and analytics engine that allows applications to store, search, and analyze large volumes of data quickly and in near real-time. Understanding its architecture is essential for using its full potential, especially when integrating it with Java applications.
At its core, Elasticsearch is built on a cluster architecture consisting of nodes, indices, and shards. A cluster is a collection of one or more nodes that together hold your entire data and provide indexing and search capabilities across all nodes.
Nodes are essentially servers that store data and participate in the cluster’s indexing and search capabilities. Each node can serve multiple roles, including master, data, or ingest nodes. The master node is responsible for cluster-wide actions such as creating or deleting indices, tracking which nodes are part of the cluster, and allocating shards. Data nodes store the actual data and execute search and aggregation operations, while ingest nodes are responsible for pre-processing documents before they are indexed.
Each index in Elasticsearch is a logical namespace that maps to one or more physical shards. An index is analogous to a database in relational database systems, and it contains documents. Documents are JSON objects that represent your data and consist of fields (key-value pairs). Each document is identified uniquely within an index by its ID.
Shards are the basic unit of storage in Elasticsearch. Each index is divided into shards, and a shard can be hosted on any node in the cluster. This division allows Elasticsearch to scale horizontally, enabling it to handle large datasets by distributing the data and search requests across multiple nodes. The number of primary shards is defined at index creation time, while replicas are copies of those primary shards that provide redundancy and increase search throughput.
When a query is executed, it’s distributed across the shards, which allows for concurrent search execution and reduces response time. Each shard independently processes the request and returns the results, which are then aggregated at the coordinating node, which is responsible for returning the final response to the client.
In Java applications, interacting with Elasticsearch is often done through the official Elasticsearch Java Client. This client abstracts many of the complexities of interacting with the REST API, allowing developers to focus on building features rather than managing connections and requests.
import org.elasticsearch.client.RestHighLevelClient; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.client.RestClient; import org.elasticsearch.client.RestClientBuilder; public class ElasticsearchClient { private RestHighLevelClient client; public ElasticsearchClient(String hostname, int port) { RestClientBuilder builder = RestClient.builder(new HttpHost(hostname, port, "http")); client = new RestHighLevelClient(builder); } public void close() throws IOException { client.close(); } // Example method to index a document public void indexDocument(String index, String id, String json) throws IOException { IndexRequest request = new IndexRequest(index).id(id).source(json, XContentType.JSON); client.index(request, RequestOptions.DEFAULT); } }
This code snippet demonstrates how to create a simple Elasticsearch client in Java, which can be used to index documents. The client connects to the Elasticsearch cluster, and the indexDocument
method allows you to add JSON documents to a specified index.
Grasping the architecture of Elasticsearch and the interaction model within a Java application lays the groundwork for developing powerful search and analytics features. Understanding how nodes, indices, shards, and documents interrelate will empower developers to optimize their applications and fully utilize Elasticsearch’s capabilities.
Integrating Java with Elasticsearch
Integrating Java with Elasticsearch involves using the Elasticsearch Java Client, which simplifies the complexities of making REST API calls. The client provides a fluent interface that allows developers to construct requests and handle responses with ease. To begin, ensure that you have the Elasticsearch Java Client library included in your project. If you’re using Maven, you can add the following dependency to your pom.xml
:
org.elasticsearch.client elasticsearch-rest-high-level-client 7.13.0
Once the dependency is set, you can start building your integration. Here’s an example of how to connect to an Elasticsearch cluster, perform basic indexing, and execute a search query:
import org.elasticsearch.action.index.IndexRequest; import org.elasticsearch.action.search.SearchRequest; import org.elasticsearch.action.search.SearchResponse; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.client.RestHighLevelClient; import org.elasticsearch.index.query.QueryBuilders; import org.elasticsearch.search.builder.SearchSourceBuilder; import org.elasticsearch.client.RestClient; import org.elasticsearch.client.RestClientBuilder; import java.io.IOException; public class ElasticsearchIntegration { private RestHighLevelClient client; public ElasticsearchIntegration(String hostname, int port) { RestClientBuilder builder = RestClient.builder(new HttpHost(hostname, port, "http")); client = new RestHighLevelClient(builder); } public void indexDocument(String index, String id, String json) throws IOException { IndexRequest request = new IndexRequest(index).id(id).source(json, XContentType.JSON); client.index(request, RequestOptions.DEFAULT); } public SearchResponse searchDocuments(String index, String field, String value) throws IOException { SearchRequest searchRequest = new SearchRequest(index); SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); searchSourceBuilder.query(QueryBuilders.matchQuery(field, value)); searchRequest.source(searchSourceBuilder); return client.search(searchRequest, RequestOptions.DEFAULT); } public void close() throws IOException { client.close(); } }
In this example, the ElasticsearchIntegration
class provides methods to index a document and to search for documents based on a specific field and value. The indexDocument
method sends a JSON document to a specified index, while the searchDocuments
method constructs a search request and executes it against Elasticsearch.
When executing a search, you can build complex queries using the rich query DSL provided by Elasticsearch. The SearchSourceBuilder
allows you to define various types of queries, sorts, and aggregations. Understanding how to leverage these features in your Java application can significantly enhance your search capabilities.
Remember to handle exceptions appropriately when integrating with Elasticsearch, as I/O operations can lead to various exceptions. It is also a good practice to ensure that your Elasticsearch client is closed properly to free up resources.
By using Java’s powerful ecosystem along with Elasticsearch’s capabilities, developers can create scalable, efficient, and robust search solutions that meet the demands of modern applications. The seamless integration of these technologies opens up numerous possibilities for advanced data retrieval and analysis.
Building Search Features in Java Applications
Building search features within Java applications that utilize Elasticsearch requires a nuanced understanding of how to perform queries effectively and how to manipulate the data returned by these queries. The power of Elasticsearch lies in its ability to perform full-text searches, filter results, and execute complex queries in real-time, making it indispensable for applications requiring dynamic data retrieval.
Let’s dive deeper into how you can build robust search features using the Elasticsearch Java Client. One of the most essential components of any search feature is the ability to construct and execute various types of queries. Elasticsearch supports a plethora of query types, from simple match queries to more complex boolean queries that combine multiple conditions.
Here is an example of how to implement a more sophisticated search feature that uses a boolean query to filter results based on multiple criteria:
import org.elasticsearch.action.search.SearchRequest; import org.elasticsearch.action.search.SearchResponse; import org.elasticsearch.client.RequestOptions; import org.elasticsearch.index.query.BoolQueryBuilder; import org.elasticsearch.index.query.QueryBuilders; import org.elasticsearch.search.builder.SearchSourceBuilder; public class AdvancedSearch { private RestHighLevelClient client; public AdvancedSearch(RestHighLevelClient client) { this.client = client; } public SearchResponse searchWithMultipleCriteria(String index, String field1, String value1, String field2, String value2) throws IOException { SearchRequest searchRequest = new SearchRequest(index); SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); BoolQueryBuilder boolQuery = QueryBuilders.boolQuery() .must(QueryBuilders.matchQuery(field1, value1)) .filter(QueryBuilders.rangeQuery(field2).gte(value2)); searchSourceBuilder.query(boolQuery); searchRequest.source(searchSourceBuilder); return client.search(searchRequest, RequestOptions.DEFAULT); } }
In this example, the searchWithMultipleCriteria
method constructs a boolean query that requires documents to match a specific condition on field1
and also filters results based on a range condition for field2
. This approach allows you to refine the search results significantly, ensuring that only the most relevant data is returned.
To improve user experience further, implementing pagination is important. Elasticsearch supports pagination through its from
and size
parameters in the search request. Here’s how you can modify the previous search method to include pagination:
public SearchResponse searchWithPagination(String index, String field1, String value1, String field2, String value2, int from, int size) throws IOException { SearchRequest searchRequest = new SearchRequest(index); SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); BoolQueryBuilder boolQuery = QueryBuilders.boolQuery() .must(QueryBuilders.matchQuery(field1, value1)) .filter(QueryBuilders.rangeQuery(field2).gte(value2)); searchSourceBuilder.query(boolQuery).from(from).size(size); searchRequest.source(searchSourceBuilder); return client.search(searchRequest, RequestOptions.DEFAULT); }
With this modification, you can specify which page of results to return and how many results to include per page. That’s particularly useful for applications that return large datasets, as it allows users to navigate through the results efficiently.
Moreover, it is important to handle the response from your search queries. The SearchResponse
object contains metadata about the search operation as well as the documents that matched the query. Parsing this response is essential for displaying results effectively in your application:
public void printSearchResults(SearchResponse response) { Arrays.stream(response.getHits().getHits()).forEach(hit -> { System.out.println("Document ID: " + hit.getId()); System.out.println("Source: " + hit.getSourceAsString()); }); }
The printSearchResults
method iterates over the search hits and prints out the document ID and its source. This allows you to see the raw data retrieved from Elasticsearch, which can then be formatted or processed further according to the needs of your application.
By combining these techniques—advanced querying, pagination, and response handling—you can build sophisticated search features into your Java applications that leverage the full power of Elasticsearch. The flexibility and performance of Elasticsearch, paired with Java’s robust ecosystem, empower developers to create seamless and responsive search experiences that cater to user needs effectively.
Optimizing Performance and Query Strategies
Optimizing performance and query strategies in Elasticsearch when integrated with Java applications very important for achieving high efficiency in search and analytics operations. As the volume of data grows and user expectations for speed and relevance increase, understanding how to fine-tune your Elasticsearch queries and configurations becomes paramount.
At the most fundamental level, one of the key strategies for optimization is to ensure that your queries are as efficient as possible. This involves not only selecting the appropriate query type but also structuring queries to minimize resource consumption. For instance, using filters instead of queries wherever possible can drastically improve performance, as filters are cached and do not score documents, which speeds up retrieval.
BoolQueryBuilder boolQuery = QueryBuilders.boolQuery() .must(QueryBuilders.matchQuery("field1", "value1")) .filter(QueryBuilders.rangeQuery("field2").gte(10).lte(100));
In the snippet above, the filter
clause ensures that the range query is executed more efficiently because it benefits from caching, thereby reducing the need for repeated computations on the same data. Additionally, remember that complex queries should be tested and monitored, as they can lead to longer execution times if not optimized properly.
Another important consideration is the use of pagination and limiting the number of returned results. When displaying search results, it’s essential to limit the data being returned to only what is needed for the user experience. This not only reduces load on the Elasticsearch server but also speeds up response times. Here’s how you might implement pagination:
public SearchResponse searchWithPagination(String index, String queryField, String queryValue, int from, int size) throws IOException { SearchRequest searchRequest = new SearchRequest(index); SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); // Construct the query searchSourceBuilder.query(QueryBuilders.matchQuery(queryField, queryValue)) .from(from) .size(size); // Limit number of results searchRequest.source(searchSourceBuilder); return client.search(searchRequest, RequestOptions.DEFAULT); }
In this example, the method searchWithPagination
takes parameters for pagination, which will allow you to fetch a specific subset of results. This is particularly useful for displaying results across multiple pages in a user interface.
Furthermore, optimizing the index itself is just as important as optimizing the queries. You should think the following aspects:
- Ensure you define correct mappings for your data types. This allows Elasticsearch to optimize the storage and retrieval of data.
- Analyze your data and decide on an optimal number of primary shards and replicas. More shards can improve parallel processing but can also increase overhead, so balance is essential.
- Adjust the refresh interval for indices depending on your use case. For write-heavy applications, increasing the refresh interval can reduce the number of refresh operations and improve indexing performance.
Another powerful feature of Elasticsearch is its ability to perform aggregations. However, aggregations can be resource-intensive, so it’s vital to use them judiciously. Think the complexity of your aggregation queries and avoid running them on large datasets without appropriate filters. Here’s an example of a simple aggregation query:
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); searchSourceBuilder.aggregation(AggregationBuilders.terms("by_field").field("field_name")); searchRequest.source(searchSourceBuilder);
By strategically applying filters and carefully structuring your aggregations, you can extract meaningful insights without incurring significant performance penalties.
Lastly, always monitor your Elasticsearch cluster’s health and performance metrics. Tools like Kibana provide visualizations that can help you identify bottlenecks in your queries or resource usage. Optimize iteratively by making adjustments based on observed performance metrics.
Implementing effective performance optimization and query strategies in Elasticsearch, especially when integrated with Java applications, requires a balanced approach that considers both the queries and the underlying architecture. By refining query structures, using Elasticsearch features, optimizing indices, and monitoring your cluster, you can build fast, responsive applications that handle complex searches with agility and precision.