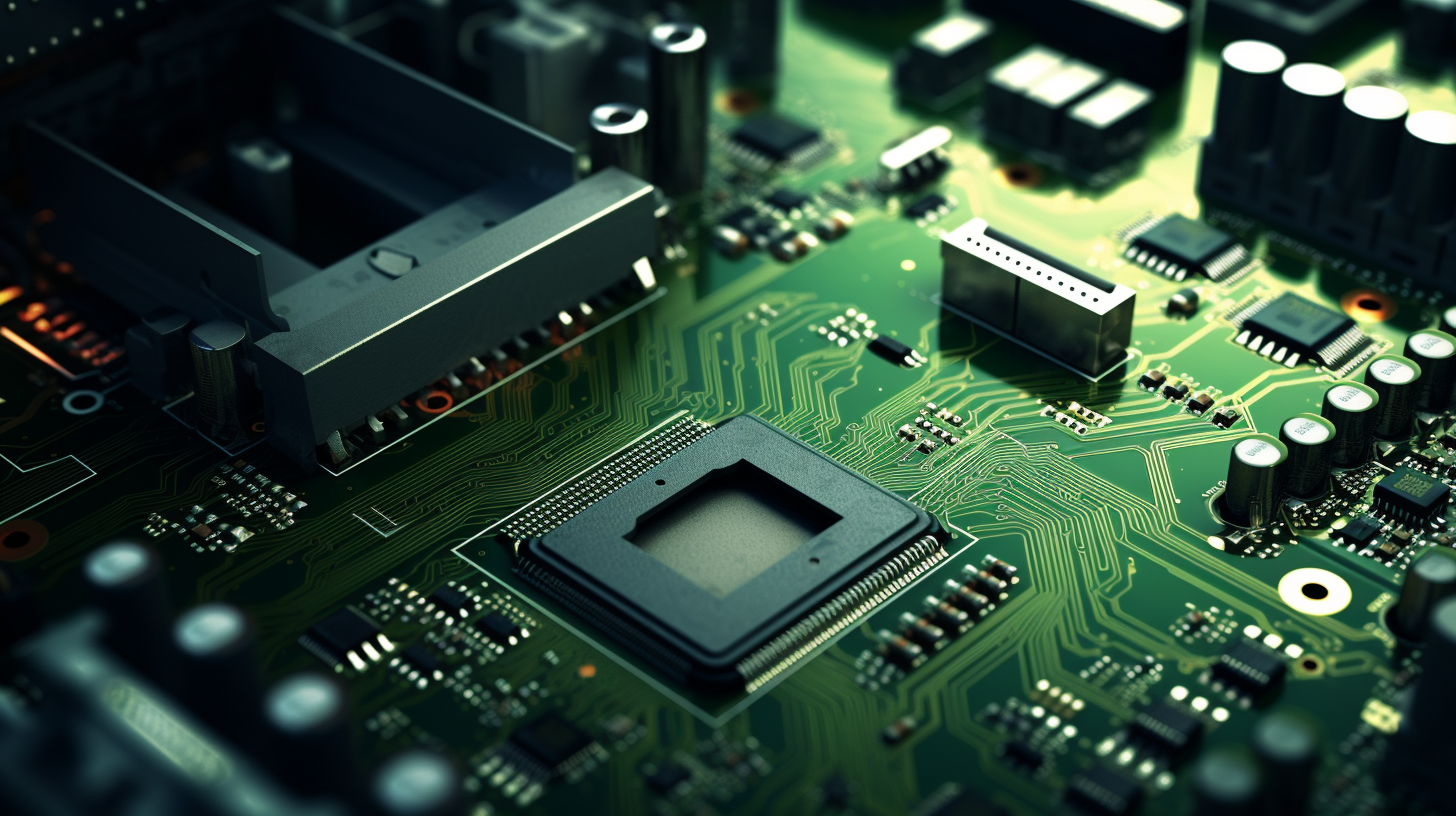
File Handling in Java: FileReader and FileWriter
File handling in Java is an important aspect that allows developers to read from and write to files seamlessly. Java provides a robust set of classes and APIs specifically designed for file operations, which are part of the java.io
package. Understanding how to manipulate files using these classes is essential for effective data management in any Java application.
At the core of file handling are the FileReader and FileWriter classes, which are used for reading and writing character files, respectively. These classes offer a simpler way to handle text files, making it easier to process data in a human-readable format.
When working with files, one of the first steps is to create a File
object that represents the file in the file system. This object acts as a bridge between your Java application and the actual file on disk. Once you have a reference to the file, you can perform various operations, such as checking if the file exists, determining its size, or deleting it.
To read data from a file, FileReader is employed. It reads the bytes from the file and converts them into characters, which can then be processed. For writing data, FileWriter serves a similar purpose, facilitating the conversion of characters back into bytes that are stored in a file.
Below is an example that illustrates how to use FileReader to read text from a file:
import java.io.FileReader; import java.io.BufferedReader; import java.io.IOException; public class FileReadExample { public static void main(String[] args) { try (BufferedReader br = new BufferedReader(new FileReader("example.txt"))) { String line; while ((line = br.readLine()) != null) { System.out.println(line); } } catch (IOException e) { e.printStackTrace(); } } }
This code snippet demonstrates how to read lines from a file named example.txt
using a BufferedReader
, which provides an efficient way to read the text line by line.
On the other hand, if you want to write text to a file, you can utilize the FileWriter class as shown in the following example:
import java.io.FileWriter; import java.io.IOException; public class FileWriteExample { public static void main(String[] args) { try (FileWriter fw = new FileWriter("output.txt")) { fw.write("Hello, World!"); fw.write("nThis is a test file."); } catch (IOException e) { e.printStackTrace(); } } }
In this example, the FileWriter
is used to create a new file called output.txt
and write two lines of text to it. The use of try-with-resources ensures that the file is closed properly, even if an exception occurs during the write operation.
Understanding these fundamental operations lays the groundwork for more complex file handling tasks, allowing developers to create applications that can interact with file systems effectively.
Using FileReader for Reading Text Files
When it comes to reading text files in Java, the FileReader class is a vital component that simplifies the process of accessing file data. This class is designed to read the character streams from a file, rendering it effortless to process text stored on disk. It can handle various character encodings, thereby accommodating different text formats without much hassle.
Typically, FileReader is used in conjunction with a BufferedReader, which provides an efficient way to read the file’s contents line by line. The BufferedReader class buffers the input, minimizing the number of I/O operations and enhancing reading performance, particularly when dealing with large files. That’s an important optimization that can significantly reduce the time taken to read data.
Let’s delve deeper into the mechanics of using FileReader. To begin with, you create an instance of FileReader by passing the desired file’s path to its constructor. This instance can then be wrapped with a BufferedReader to facilitate more efficient reading. Here’s how you can implement this:
import java.io.FileReader; import java.io.BufferedReader; import java.io.IOException; public class FileReadExample { public static void main(String[] args) { // Create a BufferedReader wrapped around FileReader try (BufferedReader br = new BufferedReader(new FileReader("example.txt"))) { String line; // Read each line until reaching the end of the file while ((line = br.readLine()) != null) { System.out.println(line); // Output the line to the console } } catch (IOException e) { e.printStackTrace(); // Handle exceptions } } }
In this code snippet, a FileReader is created for the file example.txt. The BufferedReader reads each line in a loop, and we print the content to the console. Note that we are using a try-with-resources statement, which automatically closes the BufferedReader and FileReader when the block is exited, ensuring that resources are properly released.
While the FileReader class is simpler to use, it’s important to be aware of potential issues when reading files. For instance, if the file does not exist or cannot be accessed due to permissions, an IOException will be thrown. Proper exception handling, as shown in the example, is essential to gracefully manage these scenarios.
In addition to reading text line by line, FileReader can also read data character by character. This can be useful in scenarios where you need more granular control over the reading process. Here’s a quick example:
import java.io.FileReader; import java.io.IOException; public class CharReadExample { public static void main(String[] args) { try (FileReader fr = new FileReader("example.txt")) { int ch; // Read the file character by character while ((ch = fr.read()) != -1) { System.out.print((char) ch); // Print the character to the console } } catch (IOException e) { e.printStackTrace(); // Handle exceptions } } }
In this example, we read each character from the file example.txt until the end of the file is reached. Each character is cast from an integer to a character type before being printed. This method provides a low-level way of reading, which may be beneficial depending on the application’s needs.
Using FileReader in combination with BufferedReader or on its own allows Java developers to read text files efficiently and effectively, laying the foundation for more complex file operations and data manipulations.
Using FileWriter for Writing Text Files
When it comes to writing text files in Java, the FileWriter class is your best friend, providing a simpler interface to convert character data into bytes and store it in files. It’s a simple yet powerful tool that allows developers to create new files or append to existing ones, making it indispensable in many applications where data persistence is required.
At its core, FileWriter works by writing characters directly to the output stream of the file, enabling you to manage text-based data efficiently. When you create an instance of FileWriter, you can specify whether you want to overwrite the file or append to it, thereby giving you flexibility in how you manage your file data.
To utilize FileWriter, you need to first instantiate it with the file’s path, similar to FileReader. Moreover, it’s advisable to wrap FileWriter in a BufferedWriter, which can buffer the output, enhancing performance by minimizing the number of write operations. That’s particularly useful when writing large amounts of data.
Here’s a simple example demonstrating how to use FileWriter to create a new file and write data to it:
import java.io.FileWriter; import java.io.BufferedWriter; import java.io.IOException; public class FileWriteExample { public static void main(String[] args) { // Use try-with-resources to ensure the BufferedWriter is closed properly try (BufferedWriter bw = new BufferedWriter(new FileWriter("output.txt"))) { bw.write("Hello, World!"); // Write the first line bw.newLine(); // Move to the next line bw.write("This is a test file."); // Write the second line } catch (IOException e) { e.printStackTrace(); // Handle any exceptions } } }
In this example, we create a file named output.txt and write two lines of text to it. The BufferedWriter not only facilitates writing strings but also offers methods like newLine() to handle line breaks more cleanly.
Additionally, if you intend to append data to an existing file rather than overwrite it, you can achieve this by passing a second parameter true to the FileWriter constructor:
import java.io.FileWriter; import java.io.BufferedWriter; import java.io.IOException; public class FileAppendExample { public static void main(String[] args) { // Append to the existing file instead of overwriting try (BufferedWriter bw = new BufferedWriter(new FileWriter("output.txt", true))) { bw.newLine(); // Start a new line bw.write("Appending a new line to the file."); // Write the appended text } catch (IOException e) { e.printStackTrace(); // Handle exceptions } } }
In this snippet, we append a new line to output.txt, showcasing how easy it is to add information without losing any previously written data. That’s particularly useful for log files or any scenarios where preserving prior content is essential.
It’s important to remember that writing to a file can also lead to exceptions, particularly if the file path is incorrect, if there are permission issues, or if the disk is full. Consequently, proper exception handling is critical when working with file operations to ensure your application can gracefully recover from such errors.
With the FileWriter class, Java developers can efficiently handle text file creation and writing, paving the way for more complex data manipulation and storage solutions in their applications.
Handling Exceptions in File Operations
When dealing with file operations in Java, it is essential to anticipate that things may not always go as planned. File handling is fraught with potential pitfalls, which is why managing exceptions effectively is a quintessential skill for any Java developer. The Java I/O framework uses the IOException
class to signal various issues that could arise during file operations, such as when a file is not found, when access is denied, or when there are issues with reading or writing data.
In Java, the handling of exceptions is typically performed using try-catch blocks. This structure allows developers to attempt a block of code that could potentially throw an exception and then catch that exception to handle it gracefully without crashing the application. When it comes to file operations, this approach becomes especially relevant, as you may need to manage different types of file-related exceptions.
Here’s an example that demonstrates how to properly handle exceptions while reading from a file:
import java.io.FileReader; import java.io.BufferedReader; import java.io.IOException; public class FileReadWithExceptionHandling { public static void main(String[] args) { String filePath = "example.txt"; try (BufferedReader br = new BufferedReader(new FileReader(filePath))) { String line; while ((line = br.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.err.println("An error occurred while reading the file: " + e.getMessage()); } } }
In this example, the program attempts to read lines from a file called example.txt
. If the file does not exist or there is an issue accessing it, the catch
block captures the IOException
and outputs an error message to the console. This not only informs the user about the issue but also prevents the application from crashing unexpectedly.
When writing to files, similar exception handling is necessary. For instance, when using FileWriter
, you may encounter exceptions related to file permissions or disk space. Here’s how you might handle exceptions while writing to a file:
import java.io.FileWriter; import java.io.BufferedWriter; import java.io.IOException; public class FileWriteWithExceptionHandling { public static void main(String[] args) { String filePath = "output.txt"; try (BufferedWriter bw = new BufferedWriter(new FileWriter(filePath))) { bw.write("This is a sample text."); bw.newLine(); bw.write("Writing to a file can throw exceptions!"); } catch (IOException e) { System.err.println("An error occurred while writing to the file: " + e.getMessage()); } } }
In this example, the writing process is wrapped within a try block. Should any errors occur during the writing operation, the catch block captures the IOException
and reports it. This practice is important for maintaining the robustness of your application, allowing it to handle errors smoothly without affecting user experience.
Moreover, it’s also advisable to avoid excessive use of catch blocks for specific exceptions unless necessary. In many cases, generic exception handling can simplify your code. However, in scenarios where specific exceptions require tailored responses, using multiple catch blocks can be beneficial.
Another best practice is to log exceptions rather than simply printing them out. Logging provides a way to track issues in production environments more effectively. Java provides several logging frameworks, such as java.util.logging
and third-party libraries like Log4j
and SLF4J
, which can be employed to log exception details for further analysis.
Handling exceptions effectively in file operations is a critical aspect that enhances the reliability of your Java applications. By anticipating errors and managing them gracefully, you not only improve user experience but also create a more maintainable codebase. Always remember to keep your exception handling strategies clear and concise, ensuring that they serve the application’s needs without adding unnecessary complexity.
Best Practices for File Handling in Java
When it comes to best practices for file handling in Java, a solid understanding of efficient resource management and error handling is paramount. The intricacies of file operations can lead to various issues if not approached correctly. Here are some key practices to keep in mind when dealing with files in your Java applications.
1. Use Try-With-Resources: In Java, the try-with-resources statement is an invaluable feature that ensures that each resource is closed at the end of the statement. That is especially important for file streams, as failing to close them can lead to resource leaks. For example:
try (BufferedReader br = new BufferedReader(new FileReader("example.txt"))) { // Your file reading logic here } catch (IOException e) { e.printStackTrace(); }
In this snippet, both the BufferedReader and FileReader are closed automatically, even if an exception occurs. This practice helps maintain resource integrity and avoids potential memory overflow issues.
2. Handle Exceptions Gracefully: As previously discussed, handling exceptions is critical. Always catch specific exceptions before generic ones to provide tailored error messages. For instance, if a file is not found, it’s helpful to inform the user explicitly rather than using a generic IOException message.
try { // Your file operations } catch (FileNotFoundException fnfe) { System.err.println("File not found: " + fnfe.getMessage()); } catch (IOException ioe) { System.err.println("I/O error: " + ioe.getMessage()); }
3. Validate File Paths: Always verify the file paths before attempting to read from or write to files. This prevents unnecessary exceptions. You can use the File class to check if the file exists and if it’s writable/readable:
File file = new File("example.txt"); if (!file.exists()) { System.out.println("File does not exist."); } else if (!file.canRead()) { System.out.println("File is not readable."); }
4. Use Buffered Streams: When performing file I/O operations, wrapping your FileReader and FileWriter in BufferedReader or BufferedWriter can greatly enhance performance. Buffered streams reduce the number of I/O operations by using an internal buffer, which is particularly beneficial when reading or writing large files.
try (BufferedWriter bw = new BufferedWriter(new FileWriter("output.txt"))) { bw.write("Efficient file writing practice."); }
5. Avoid Hardcoding File Paths: Hardcoding file paths can lead to portability issues. Instead, consider using relative paths or allowing users to specify their own file paths, which increases the flexibility of your application. You can also utilize Java’s built-in properties for user directories:
String userHome = System.getProperty("user.home"); File file = new File(userHome, "myfolder/example.txt"); // Creates path based on user home
6. Clean Up Resources: Even though try-with-resources handles cleanup, it’s worth mentioning that when managing multiple resources, you should ensure all are closed properly. This is particularly crucial in complex applications where file handling errors can cascade.
7. Log Errors: Instead of merely printing exception details to the console, ponder logging errors using a logging framework. This practice allows you to track application behavior and diagnose issues without cluttering the console output.
import java.util.logging.Logger; Logger logger = Logger.getLogger("FileLogger"); try { // Your file operations } catch (IOException e) { logger.severe("File operation failed: " + e.getMessage()); }
By adhering to these best practices, Java developers can enhance the reliability, maintainability, and performance of their file handling code. Emphasizing resource management and proper error handling not only leads to cleaner code but also fosters a robust application environment.