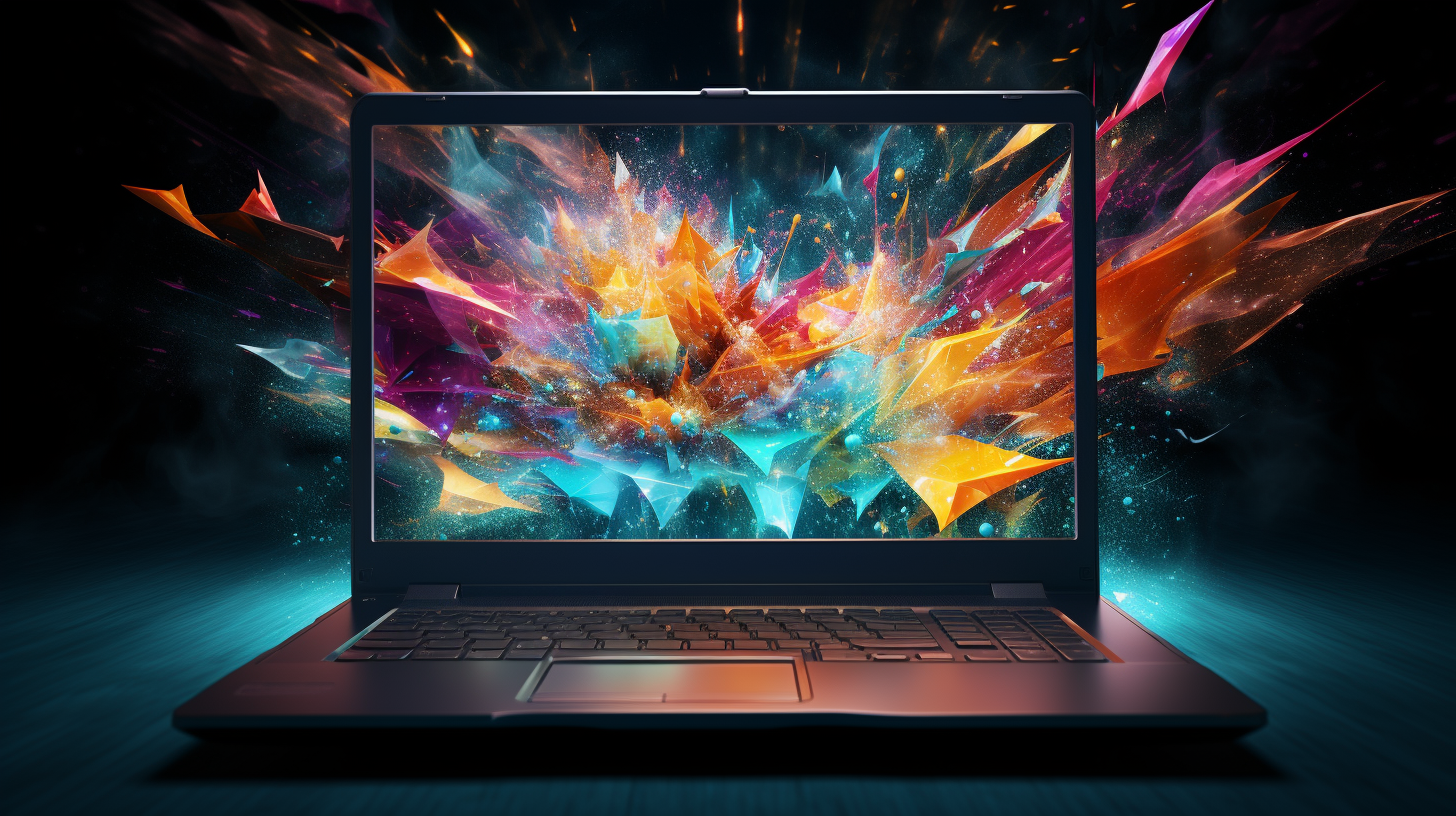
Automating File Transfers with Bash
When it comes to automating file transfers, understanding the various protocols and tools available very important. Each file transfer protocol has its own strengths and weaknesses, and the choice of which to use can greatly affect both security and efficiency. Among the most common protocols are FTP (File Transfer Protocol), SFTP (SSH File Transfer Protocol), and rsync.
FTP: That’s one of the oldest and most widely used file transfer protocols. However, it transmits data in plaintext, making it vulnerable to interception. While FTP can be useful in trusted environments, its lack of encryption is a significant drawback for sensitive data transfers.
SFTP: Unlike FTP, SFTP runs over an SSH (Secure Shell) connection, providing a secure channel for file transfers. It encrypts both the command and data channels, ensuring that your files remain protected during transmission. This makes SFTP a preferred choice for secure file transfers over untrusted networks.
rsync: This tool is not just a protocol but a powerful utility for synchronizing files and directories between two locations. It’s particularly efficient because it only transfers the differences between source and destination, minimizing bandwidth usage. Rsync can work over SSH, further adding a layer of security to the file transfer process.
It is also worth mentioning other tools such as scp (Secure Copy Protocol), which is a simpler alternative for transferring files securely over SSH. While it may lack the advanced features of rsync, it’s simpler and effective for single-file transfers.
Setting Up SSH for Secure Transfers
To set up SSH for secure file transfers, you’ll first need to ensure that SSH is installed on both the local and remote machines. Most Linux distributions come with SSH pre-installed, but it can be easily installed if it’s not available. You can check if SSH is installed by running the following command:
ssh -V
This command will output the version of SSH installed, verifying its presence. If SSH is not installed, you can install it using your package manager. For Debian-based systems, use:
sudo apt-get install openssh-client openssh-server
For Red Hat-based systems, use:
sudo yum install openssh-clients openssh-server
Once SSH is installed, the next step is to configure it for secure access. This involves generating SSH key pairs that will allow secure, password-less authentication. You can generate these keys using the following command:
ssh-keygen -t rsa -b 4096
When prompted, you can accept the default file location and choose whether to set a passphrase. The passphrase adds an extra layer of security, but for automation purposes, it might be more convenient to leave it empty.
After generating your SSH keys, the next step is to copy the public key to the remote server. This can be done efficiently with the following command:
ssh-copy-id user@remote-server
Replace “user” with your username on the remote machine and “remote-server” with the hostname or IP address of the remote machine. This command will prompt you for your password on the remote server, after which it will copy your public key into the appropriate file on the remote server.
Now you can test the SSH connection to ensure everything is working correctly:
ssh user@remote-server
If everything is set up correctly, you should be logged in without being prompted for a password. This process is the foundation for secure file transfers, so that you can automate tasks without compromising security.
For even more security, think disabling password authentication altogether in your SSH configuration. Edit the SSH configuration file on the remote server located at /etc/ssh/sshd_config
and set the following parameters:
PasswordAuthentication no ChallengeResponseAuthentication no
After making these changes, restart the SSH service to apply them:
sudo systemctl restart sshd
Using rsync for Efficient File Synchronization
When it comes to file synchronization, rsync stands out as an exceptional tool due to its efficiency and flexibility. Unlike other transfer methods that may send entire files regardless of changes, rsync employs a unique algorithm that identifies differences between the source and target files, transferring only the modified portions. This not only saves bandwidth but also significantly speeds up the transfer process, making it an ideal choice for regular backups and updates.
To begin using rsync, ensure it’s installed on your system. Most Linux distributions include rsync by default, but you can verify its presence with the following command:
rsync --version
If rsync is not installed, you can easily add it using your package manager. For Debian-based systems, run:
sudo apt-get install rsync
For Red Hat-based systems, use:
sudo yum install rsync
Once installed, you can start using rsync to synchronize files. The basic syntax for rsync is as follows:
rsync [options] source destination
For instance, to synchronize a local directory with a remote server, you would use:
rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
In this command:
- This option stands for “archive” and preserves permissions, timestamps, symbolic links, and other file attributes.
- This enables “verbose” mode, giving you detailed output about the transfer process.
- This compresses file data during the transfer, which can considerably speed up the synchronization process over slower connections.
To exclude certain files or directories from synchronization, use the –exclude option. For example, to exclude all .tmp files, you could modify the command as follows:
rsync -avz --exclude='*.tmp' /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
Rsync can also be utilized for local synchronization between directories on the same machine. The syntax remains largely unchanged:
rsync -av /path/to/source/ /path/to/destination/
Additionally, using rsync over SSH adds a vital layer of security. You can specify the SSH option within your rsync command:
rsync -avz -e "ssh" /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
To automate your rsync tasks, consider incorporating them into a cron job. This can be done by editing your crontab:
crontab -e
Then, you can add a line to schedule your rsync operation. For example, to run the synchronization every day at 2 AM, the line would look like this:
0 2 * * * rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
Automating Transfers with Cron Jobs
# Open the crontab editor crontab -e # Add the following line to schedule rsync for daily execution at 2 AM 0 2 * * * rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
Automating file transfers using cron jobs allows you to schedule tasks to run at specified intervals without manual intervention. This is particularly useful for regular backups, data synchronization, and other repetitive tasks. The cron service in Unix-like operating systems manages these scheduled tasks through a configuration file known as crontab.
To get started with cron jobs, you first need to access your crontab file, where you can define your scheduled tasks. Begin by executing the command:
crontab -e
This command opens your user’s crontab file in the default text editor. Each line in this file represents a scheduled task (or cron job), constructed with a specific format that dictates when and how often the command should run.
The structure of a cron job consists of five time and date fields followed by the command to be executed:
* * * * * command_to_execute - - - - - | | | | | | | | | +---- Day of the week (0 - 7) (Sunday is both 0 and 7) | | | +------ Month (1 - 12) | | +-------- Day of the month (1 - 31) | +---------- Hour (0 - 23) +------------ Minute (0 - 59)
For example, to run an rsync command every day at 2 AM, you would write:
0 2 * * * rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
In this line, `0 2 * * *` specifies that the task should be executed at 2:00 AM each day. The `rsync` command that follows will synchronize the specified local directory with the remote directory on the server.
You can also customize the frequency of the job according to your needs. For instance, if you want to run the task every hour, you would modify the crontab entry to:
0 * * * * rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/
This entry runs the rsync command at the start of every hour.
Once you’ve added your cron jobs, save and exit the editor. The cron daemon automatically reads and applies your changes without requiring a restart. You can verify that your cron job has been scheduled successfully by listing your current cron jobs with:
crontab -l
Additionally, it’s essential to handle output and potential errors from your cron jobs. By default, any output generated by the command is sent to the email address associated with your user account. To redirect this output to a log file or to suppress it entirely, you can append the command with `>> /path/to/logfile.log 2>&1` (to log output and errors) or `>/dev/null 2>&1` (to discard it).
For instance:
0 2 * * * rsync -avz /path/to/local/dir/ user@remote-server:/path/to/remote/dir/ >> /path/to/logfile.log 2>&1