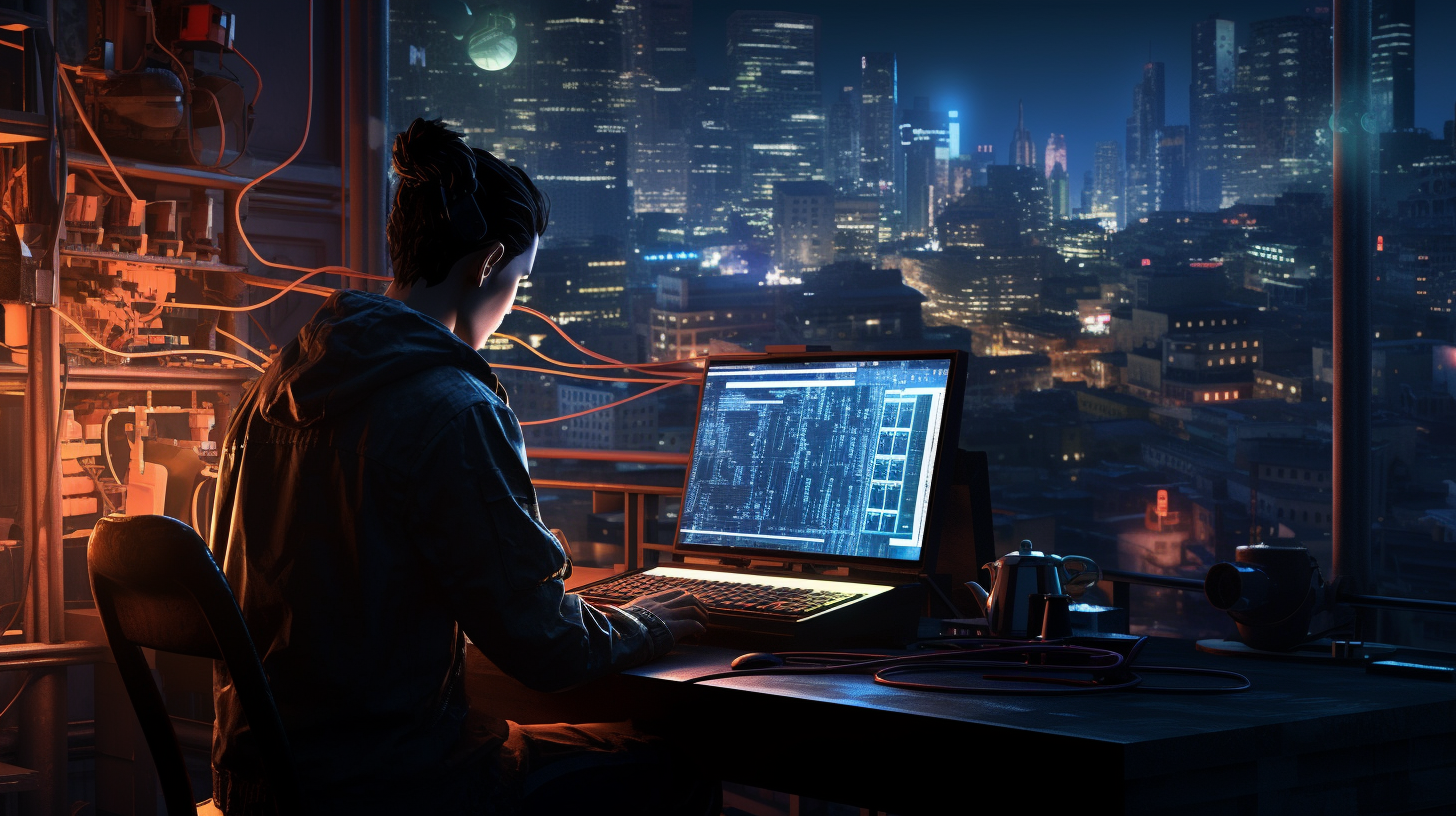
Swift and Accessibility Features
Accessibility in Swift is an essential aspect of application development that ensures all users, including those with disabilities, can interact with your app effectively. Swift provides robust tools and frameworks that allow developers to create applications that are not only functional but also inclusive. Understanding these capabilities very important for building applications with a wide user base.
At the heart of accessibility in iOS is the UIKit framework, which offers various APIs to help developers implement accessibility features. Elements such as buttons, labels, and images can be easily modified to provide a better experience for users with visual, auditory, or physical impairments.
One of the primary components of accessibility in Swift is the use of accessibility traits. These traits provide context to assistive technologies like VoiceOver, allowing them to convey the purpose and functionality of UI elements effectively. For instance, a button can be identified as a UIAccessibilityTraitButton
, indicating its clickable nature.
Here’s a simple example of how to set accessibility traits in a Swift button:
let myButton = UIButton() myButton.setTitle("Submit", for: .normal) myButton.accessibilityTraits = .button
In addition to traits, developers can enhance accessibility by providing meaningful accessibility labels and hints. An accessibility label is a brief description of the element, while a hint explains its purpose or provides additional context. That’s particularly useful for users who rely on screen readers.
Here’s how you can set an accessibility label and hint for a button:
myButton.accessibilityLabel = "Submit your form" myButton.accessibilityHint = "Double tap to submit the data"
Moreover, accessibility values can be used for sliders, progress indicators, or other components that have a measurable state. Providing an accurate value helps users understand the current status of these components, making navigation intuitive.
Lastly, incorporating accessibility in your Swift applications isn’t merely about adding features; it’s about fostering an inclusive environment where every user can have a fulfilling experience. By understanding and using the accessibility tools provided by Swift, developers can create applications that cater to a diverse audience, ensuring that accessibility is a cornerstone of their design philosophy.
Implementing VoiceOver Support
Implementing VoiceOver support in your Swift applications is a critical step toward making them more accessible. VoiceOver is a screen reader feature that allows users with visual impairments to interact with their devices through auditory feedback. When VoiceOver is enabled, it reads aloud the text and describes the UI elements on the screen, giving users a rich understanding of the app’s content and functionality.
To ensure that your app works seamlessly with VoiceOver, you need to provide appropriate accessibility properties for UI elements. The first step in this process is to confirm that your UI components are properly identified. By doing this, you can give VoiceOver the information it needs to describe the elements accurately.
Let’s ponder a basic example where we have a UIImageView that displays a profile picture. It’s crucial to give this image view an accessibility label so that VoiceOver can describe it to users.
let profileImageView = UIImageView() profileImageView.image = UIImage(named: "profile_picture") profileImageView.accessibilityLabel = "Profile picture of Frank McKinnon"
In the code snippet above, we set the accessibilityLabel
property of the image view to provide context. Now, when VoiceOver users swipe to this image, they will hear “Profile picture of Albert Lee,” which gives them a clear understanding of what they’re viewing.
Another important consideration is the use of accessibility actions. These actions enable users to perform specific tasks beyond just tapping. For instance, if you have a custom view that plays a video, you might want to allow users to play or pause the video through specific VoiceOver gestures. You can achieve this by adding custom accessibility actions:
class VideoPlayerView: UIView { override init(frame: CGRect) { super.init(frame: frame) self.isAccessibilityElement = true self.accessibilityLabel = "Video player" self.accessibilityCustomActions = [ UIAccessibilityCustomAction(name: "Play", target: self, selector: #selector(playVideo)), UIAccessibilityCustomAction(name: "Pause", target: self, selector: #selector(pauseVideo)) ] } @objc func playVideo() { // Logic to play video } @objc func pauseVideo() { // Logic to pause video } required init?(coder aDecoder: NSCoder) { fatalError("init(coder:) has not been implemented") } }
With the above implementation, users can now activate the “Play” or “Pause” actions using VoiceOver gestures, enhancing their interaction with the video player.
Testing your app with VoiceOver is essential to ensure everything works as intended. Enable VoiceOver in the device settings and navigate through your application. Pay attention to how elements are read aloud and whether the descriptions make sense. Listen for any missing labels or hints, and adjust your accessibility properties accordingly for a smoother experience.
By diligently implementing VoiceOver support and fine-tuning your accessibility properties, you create an environment where users with visual impairments can enjoy your app’s functionality just as much as any other user. This commitment to inclusivity not only broadens your app’s audience but also enhances its overall user experience.
Enhancing User Interface with Dynamic Type
When it comes to enhancing user interfaces in Swift applications, one of the key features to consider is Dynamic Type. Dynamic Type allows users to adjust the font size of text displayed in your app, making it easier for those with visual impairments to read content comfortably. By incorporating Dynamic Type into your application, you ensure that your users have a personalized and accessible experience that caters to their individual needs.
To enable Dynamic Type, you must configure your text elements to respond to the user’s preferred text size settings. This is achieved by using the UIFontMetrics class in conjunction with the standard font styles provided by UIKit. These font styles are designed to scale appropriately, maintaining readability and layout integrity across different text sizes.
Here’s an example of how to implement Dynamic Type for a UILabel:
let myLabel = UILabel() myLabel.text = "Welcome to our accessible app!" myLabel.font = UIFont.preferredFont(forTextStyle: .body) myLabel.adjustsFontForContentSizeCategory = true
In this snippet, we first set the text for the label. Then, we assign a font that corresponds to the body text style, which is part of the standard text styles in iOS. The key line here is adjustsFontForContentSizeCategory, which, when set to true, allows the label to automatically adjust its font size based on the user’s preferences.
Beyond labels, you can apply Dynamic Type to other UI components as well—like buttons and text fields. For instance, here’s how you can do this for a UIButton:
let myButton = UIButton(type: .system) myButton.setTitle("Tap Me!", for: .normal) myButton.titleLabel?.font = UIFont.preferredFont(forTextStyle: .headline) myButton.titleLabel?.adjustsFontForContentSizeCategory = true
This implementation ensures that the button text adapts to different text size settings, providing a consistent experience across your app.
It’s also highly recommended to test your application using different text sizes to see how it affects your layout. You can adjust the font size in the Device Settings under Display & Brightness > Text Size. By doing so, you can observe firsthand how your UI elements respond to size changes and make necessary adjustments to your constraints and layouts to accommodate larger text sizes.
Furthermore, you can enhance the experience by observing the contentSizeCategoryDidChangeNotification notification, which lets you react programmatically when the user changes their preferred text size. This allows for a dynamic adjustment of your interface, ensuring that all elements remain visually appealing and usable:
NotificationCenter.default.addObserver(self, selector: #selector(adjustFonts), name: UIContentSizeCategory.didChangeNotification, object: nil) @objc func adjustFonts() { myLabel.font = UIFont.preferredFont(forTextStyle: .body) myButton.titleLabel?.font = UIFont.preferredFont(forTextStyle: .headline) }
Implementing Dynamic Type not only contributes to accessibility but also improves the overall usability of your app. By adopting this approach, you demonstrate a commitment to inclusivity, enabling users of all abilities to interact with your application in a way that suits them best. Amid increasing focus on where technology is meant to enhance lives, ensuring that your app is adaptable to various needs is not just a best practice, but a necessity.
Customizing Accessibility Properties
Customizing accessibility properties in Swift applications involves a systematic approach to ensure that all UI elements are not only functional but also easily interpretable by users relying on assistive technologies. It’s essential to provide a clear and concise description of each UI element’s purpose and functionality, so users can navigate your app efficiently. Swift provides developers with the tools to achieve this through various accessibility properties.
One of the first steps in customizing accessibility properties is to define the accessibilityLabel. This label serves as a verbal description of the UI element. It should succinctly convey the element’s purpose. For instance, if you have a button that submits a form, you should ensure its accessibility label reflects this action.
let submitButton = UIButton() submitButton.setTitle("Submit", for: .normal) submitButton.accessibilityLabel = "Submit your form"
In this example, when users encounter the submitButton, they will hear “Submit your form” when they navigate to it using VoiceOver, providing them with a clear understanding of its function.
Another critical aspect of accessibility is the accessibilityHint. This property offers additional context or instructions about how to interact with the UI element. It can guide users on the expected action or outcome when they activate the element.
submitButton.accessibilityHint = "Double tap to submit the data"
Adding an accessibility hint like this informs users that they need to double-tap the button to perform the submission, enhancing their understanding and interaction experience.
For elements that convey a range of values, such as sliders or progress indicators, the accessibilityValue property becomes invaluable. It provides users with real-time information about the current status of these components. For instance, if you have a slider to adjust volume, you can set its value as follows:
let volumeSlider = UISlider() volumeSlider.value = 0.5 // Represents 50% volume volumeSlider.accessibilityValue = "Volume at 50%"
This accessibility value allows users to understand exactly where the slider is positioned, thereby making navigation more intuitive.
Furthermore, it’s crucial to consider the accessibilityTraits. These traits give assistive technologies insight into how to treat the UI element. For example, if a view is meant to be a button, you would designate it with the button trait:
submitButton.accessibilityTraits = .button
This trait informs VoiceOver that the element is actionable, prompting it to guide users accordingly when they swipe to it.
In addition to these properties, developers can create custom accessibility actions to improve user engagement further. Custom actions allow you to define specific gestures or interactions that can be performed on your UI elements.
let customAction = UIAccessibilityCustomAction(name: "Reset", target: self, selector: #selector(resetAction)) myButton.accessibilityCustomActions = [customAction] @objc func resetAction() { // Logic to reset a form }
By adding a custom action, users can use VoiceOver gestures to access the “Reset” functionality directly, streamlining their interaction with the app.
Customizing accessibility properties is a vital practice in Swift application development. By thoughtfully applying accessibility labels, hints, values, traits, and custom actions, developers can create a more inclusive and simple to operate experience that resonates with all users, regardless of their abilities. This commitment to accessibility not only broadens the reach of your application but also fosters a culture of inclusivity and awareness within the tech community.
Testing and Debugging Accessibility Features
Testing and debugging accessibility features in Swift applications is a critical process that ensures all users, especially those with disabilities, can interact with your app seamlessly. The goal is to identify any accessibility issues early in the development process, allowing you to create an inclusive experience. As you develop your app, it’s important to adopt best practices for testing these features systematically.
One of the most effective ways to test accessibility features is to utilize VoiceOver, Apple’s built-in screen reader. By enabling VoiceOver on your device, you can experience your app as users with visual impairments would. Navigate through your UI components using gestures, and listen carefully to how VoiceOver describes each element. Ensure that accessibility labels, hints, and values are being read correctly and meaningfully.
For example, if a button is supposed to allow users to submit a form, ensure that its accessibility label reads something like “Submit your form” and not just “Button.” Here’s a quick reminder of how to set an accessibility label for a button:
let submitButton = UIButton() submitButton.setTitle("Submit", for: .normal) submitButton.accessibilityLabel = "Submit your form"
Once you’ve set your accessibility attributes, use VoiceOver gestures to navigate to the button. If you hear a vague or incorrect description, revisit the code to refine your accessibility properties.
In addition to manual testing with VoiceOver, automated testing can complement your accessibility checks. XCTest, Apple’s testing framework, can be extended to include accessibility checks. You can create UI tests that simulate user interactions and check for the presence of accessibility properties. For instance, you might use assertions to verify that specific elements have the expected accessibility labels:
func testSubmitButtonAccessibility() { let app = XCUIApplication() app.launch() let submitButton = app.buttons["Submit your form"] XCTAssertTrue(submitButton.exists) XCTAssertEqual(submitButton.label, "Submit your form") }
This simple test checks that the submit button exists and has the correct label, helping you catch issues early in the development cycle.
Another important aspect of testing is checking for dynamic content updates in your app. For instance, if your app receives notifications or updates the UI based on user interactions, ensure that VoiceOver announces these changes appropriately. You can use the UIAccessibility.post(notification:argument:) method to inform VoiceOver of updates. Here’s an example:
func notifyUserOfUpdate() { // Update content UIAccessibility.post(notification: .announcement, argument: "New message received") }
By calling this method, VoiceOver will read out the announcement, informing users of the update. This functionality is essential for keeping users informed of changes in real-time.
Don’t forget to involve users with disabilities in your testing process when possible. Getting feedback from real users can uncover issues that automated tests or even manual checks may overlook. Their insights will be invaluable in making your app more usable and inclusive.
Lastly, ensure that your app’s navigation is logical and intuitive. Test the flow of your app using different accessibility features. For instance, check how interactive elements are grouped and whether users can navigate seamlessly between them using VoiceOver gestures. If you identify any navigation issues, think modifying the order of accessibility elements or grouping related elements for a better experience.
Diligent testing and debugging of accessibility features in Swift applications significantly enhance the usability of your app for all users. By using both manual and automated testing techniques, you can identify and rectify accessibility issues, thereby fostering a more inclusive digital environment. This commitment to accessibility not only benefits users with disabilities but also improves the overall user experience, making your app approachable and enjoyable for everyone.