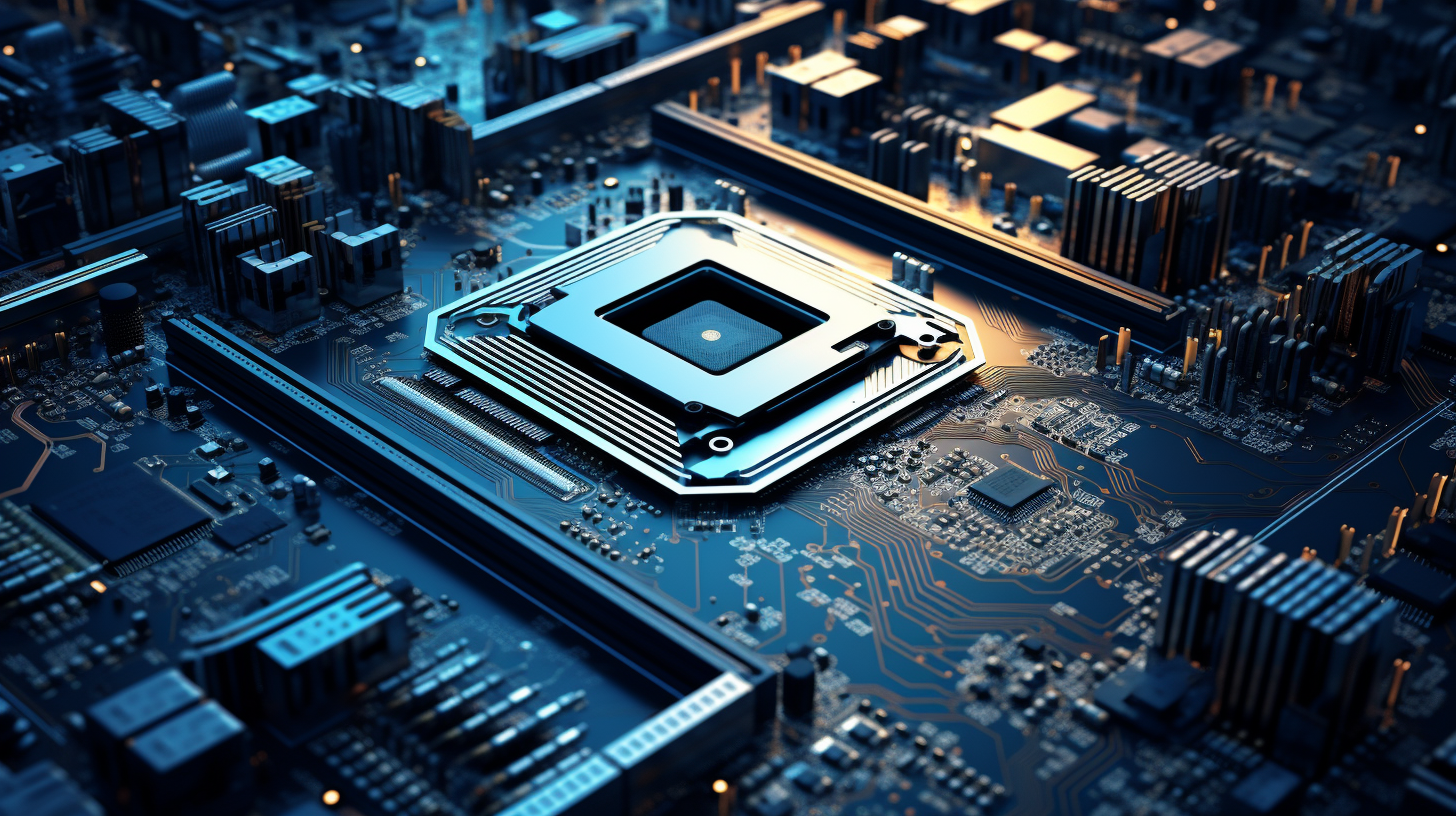
Using Window Functions for Data Analysis
Window functions in SQL are a powerful tool that allow you to perform calculations across a set of rows that are related to the current row. Unlike aggregate functions that return a single value for a group of rows, window functions maintain the detail of each row while performing calculations over a specified range or partition of the data. This unique capability allows you to generate insights and analytics directly within your SQL queries.
At their core, window functions operate with an OVER() clause that defines the window of rows the function should consider. This allows you to specify how the data should be partitioned and ordered. For instance, if you want to calculate a running total of sales for each salesperson, you can use a window function to achieve this without collapsing the individual sales records into a single total.
Here’s a basic example of a window function that calculates a running total of sales by salesperson:
SELECT salesperson_id, sale_amount, SUM(sale_amount) OVER (PARTITION BY salesperson_id ORDER BY sale_date) AS running_total FROM sales ORDER BY salesperson_id, sale_date;
In the above example, the SUM function is used as a window function. The OVER clause defines that the calculation should partition the results by salesperson_id
and order the rows by sale_date
. This allows each salesperson’s sales to be totaled up cumulatively as you move through the sales records chronologically.
Another key feature of window functions is their ability to operate over a specified frame of rows, which can be controlled with the ROWS
or RANGE
clauses within the OVER() clause. This allows for even finer control over which rows are included in the calculation relative to the current row.
For example, to calculate a moving average of sales over the last three days for each salesperson, you would define the frame like so:
SELECT salesperson_id, sale_date, sale_amount, AVG(sale_amount) OVER (PARTITION BY salesperson_id ORDER BY sale_date ROWS BETWEEN 2 PRECEDING AND CURRENT ROW) AS moving_average FROM sales ORDER BY salesperson_id, sale_date;
In this case, the AVG
function calculates the average for the current row and the two preceding rows, giving you a dynamic view of performance over time.
Understanding how to leverage window functions effectively will significantly enhance your ability to perform complex data analysis directly within SQL, enabling you to derive insights that would otherwise require additional processing outside of your database queries.
Types of Window Functions
When discussing types of window functions, it is essential to recognize that they can be categorized into several distinct groups based on the nature of their calculations. The primary categories include aggregation functions, ranking functions, and analytic functions. Each type serves a specific purpose and utilizes the OVER() clause, allowing complex analyses without losing row-level detail.
Aggregation Functions are perhaps the most commonly used types of window functions. These functions perform a calculation across a set of rows that are related to the current row, similar to traditional aggregate functions but without collapsing the result set. Common aggregate functions used as window functions include SUM, AVG, COUNT, MIN, and MAX.
For instance, if you want to calculate the total sales for each product category while still displaying individual product sales, you could use the SUM function like this:
SELECT category_id, product_id, sale_amount, SUM(sale_amount) OVER (PARTITION BY category_id) AS total_sales_by_category FROM sales ORDER BY category_id, product_id;
In this example, the total sales for each category are calculated and displayed alongside individual product sales, providing both detail and summarized information.
Ranking Functions provide methods to assign a rank to each row within a partition of a result set. The most common ranking functions are ROW_NUMBER(), RANK(), and DENSE_RANK(). These functions are particularly useful in scenarios where you need to rank items based on specific criteria.
For example, if you want to rank products within each category based on their sales, you can use the ROW_NUMBER function as follows:
SELECT category_id, product_id, sale_amount, ROW_NUMBER() OVER (PARTITION BY category_id ORDER BY sale_amount DESC) AS rank FROM sales ORDER BY category_id, rank;
In this case, each product is assigned a unique rank within its category based on the sale_amount value, allowing for a clear comparative analysis.
Analytic Functions encompass a variety of calculations that are useful for advanced statistical analyses. Functions like LEAD() and LAG() fall under this category and enable you to access data from subsequent or preceding rows in the result set without requiring a self-join.
For example, if you need to compare each product’s sales with the sales of the previous product, you can use the LAG function like this:
SELECT product_id, sale_date, sale_amount, LAG(sale_amount) OVER (ORDER BY sale_date) AS previous_sale FROM sales ORDER BY sale_date;
This gives you the ability to directly compare each row with its predecessor, enabling rich temporal analyses of how sales are changing over time.
Understanding the different types of window functions and their respective applications is fundamental for any data analyst or SQL practitioner. Each type of window function brings its strengths, allowing for robust data manipulation and insightful analyses within your SQL queries.
Practical Applications in Data Analysis
In the sphere of data analysis, practical applications of window functions are vast and transformative, enabling analysts to derive insights that inform strategic decisions. One of the most notable applications is in financial reporting, where running totals can be essential for understanding cash flow and sales trends.
Ponder a scenario where a company wants to analyze its monthly revenue streams. By employing a window function, you can conveniently compute the cumulative revenue for each month while still displaying the individual monthly figures. This can be executed with the following SQL statement:
SELECT month, revenue, SUM(revenue) OVER (ORDER BY month) AS cumulative_revenue FROM monthly_revenue ORDER BY month;
In this example, the cumulative revenue is calculated by summing up the revenue from the start of the dataset up to the current month. This allows stakeholders to easily visualize the growth trajectory over time.
Another compelling use case for window functions is in customer analytics, particularly for tracking customer engagement over time. By using the LAG function, you can compare a customer’s current activity with their past behavior, helping to identify patterns or changes in engagement. Here’s how you might implement this:
SELECT customer_id, engagement_date, activity_level, LAG(activity_level) OVER (PARTITION BY customer_id ORDER BY engagement_date) AS previous_activity FROM customer_engagement ORDER BY customer_id, engagement_date;
This query provides a clear view of changes in each customer’s activity level compared to their previous engagement. Such insights can inform targeted marketing campaigns or personalized customer service interventions.
Window functions shine particularly when dealing with time-series data, allowing for advanced analyses like calculating year-over-year growth rates. By combining window functions with arithmetic operations, you can derive actionable insights from historical data. For example, to assess the percentage growth in revenue compared to the same month in the previous year, you could use the following query:
SELECT month, revenue, LAG(revenue) OVER (ORDER BY month) AS last_year_revenue, (revenue - LAG(revenue) OVER (ORDER BY month)) / LAG(revenue) OVER (ORDER BY month) * 100 AS growth_rate FROM monthly_revenue ORDER BY month;
This SQL statement calculates the growth rate as a percentage, providing vital information for forecasting and budget planning. By using window functions, analysts can create a richer narrative around their data that is both insightful and actionable.
Furthermore, window functions can also be instrumental in detecting outliers or anomalies within datasets. By calculating the average or standard deviation across a dataset and comparing individual records against these metrics, analysts can flag values that deviate significantly from the norm. For instance:
SELECT product_id, sale_amount, AVG(sale_amount) OVER () AS average_sales, STDDEV(sale_amount) OVER () AS sales_std_dev, CASE WHEN sale_amount > (AVG(sale_amount) OVER () + 2 * STDDEV(sale_amount) OVER ()) THEN 'Outlier' ELSE 'Normal' END AS sale_status FROM product_sales ORDER BY sale_amount DESC;
In this query, we calculate the average and standard deviation of sales across all products to categorize sales amounts as either ‘Outlier’ or ‘Normal’. This type of analysis can significantly enhance data quality assessments and ensure more reliable decision-making.
Ultimately, the practical applications of window functions in data analysis are limited only by your creativity. Whether you are aiming for deeper customer insights, performance tracking, financial reporting, or anomaly detection, window functions provide a robust framework for achieving complex analytical goals directly within your SQL environment. Embracing these functions will undoubtedly refine your analytical prowess and empower your data-driven decisions.
Best Practices for Using Window Functions
When working with window functions, it’s essential to adhere to several best practices to maximize their effectiveness and maintain optimal performance. These practices ensure not only the accuracy of your queries but also their efficiency, especially when dealing with large datasets.
1. Understand the Partitioning and Ordering
Properly defining the partitioning and ordering within the OVER() clause very important. Partitioning allows you to break your dataset into logical segments where window functions will operate independently. Ordering determines the sequence in which rows are processed within each partition. A well-structured partition and order can significantly affect the results and the performance of your queries. For example:
SELECT employee_id, department_id, salary, RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank FROM employees;
In this case, ensuring that the partition is set by department_id allows each department’s salaries to be ranked independently.
2. Limit the Number of Rows Processed
Window functions can be resource-intensive, especially on large datasets. To enhance performance, it’s advisable to limit the number of rows that the window function processes. This can be achieved using filtering conditions before applying window functions. For instance, if you are only interested in recent records, you might do something like:
WITH recent_sales AS ( SELECT * FROM sales WHERE sale_date >= '2023-01-01' ) SELECT salesperson_id, sale_date, sale_amount, SUM(sale_amount) OVER (PARTITION BY salesperson_id ORDER BY sale_date) AS running_total FROM recent_sales;
This approach narrows down the dataset to only those records that are relevant, improving query efficiency.
3. Use Appropriate Frame Specifications
When using window functions that require a frame specification, it’s important to choose the right frame based on your analytical needs. For instance, while calculating a moving average, you could specify a frame to limit calculations to a certain number of preceding rows, as shown:
SELECT salesperson_id, sale_date, sale_amount, AVG(sale_amount) OVER (PARTITION BY salesperson_id ORDER BY sale_date ROWS BETWEEN 4 PRECEDING AND CURRENT ROW) AS moving_average FROM sales;
This frame specification allows you to calculate the moving average over the last five sales, providing a smooth view of performance that balances detail with trend analysis.
4. Avoid Excessive Nesting of Window Functions
Nesting window functions can lead to increased complexity and decreased readability in your SQL queries. While SQL allows for this, it can often be better to break complex queries into smaller, more manageable components. For instance, instead of nesting multiple window functions, ponder using common table expressions (CTEs) to simplify the logic:
WITH ranked_sales AS ( SELECT salesperson_id, sale_date, sale_amount, RANK() OVER (PARTITION BY salesperson_id ORDER BY sale_date) AS rank FROM sales ) SELECT salesperson_id, sale_date, sale_amount, rank, SUM(sale_amount) OVER (PARTITION BY salesperson_id) AS total_sales FROM ranked_sales;
By separating the ranking and summation logic, you enhance the clarity of your SQL code.
5. Test Performance with Realistic Data
It’s crucial to test your queries using realistic datasets to understand how window functions will behave in production. Performance can vary significantly based on data volume, structure, and distribution. Use EXPLAIN plans to analyze query performance and identify potential bottlenecks.
EXPLAIN SELECT salesperson_id, sale_date, sale_amount, SUM(sale_amount) OVER (PARTITION BY salesperson_id ORDER BY sale_date) AS running_total FROM sales;
This will give you insights into how the database engine plans to execute your query, which will allow you to make informed optimizations.
By adhering to these best practices, you can harness the full power of window functions while ensuring your SQL queries remain efficient, clear, and maintainable. The ability to perform complex analyses directly within your database opens up a world of possibilities for data-driven decision-making.