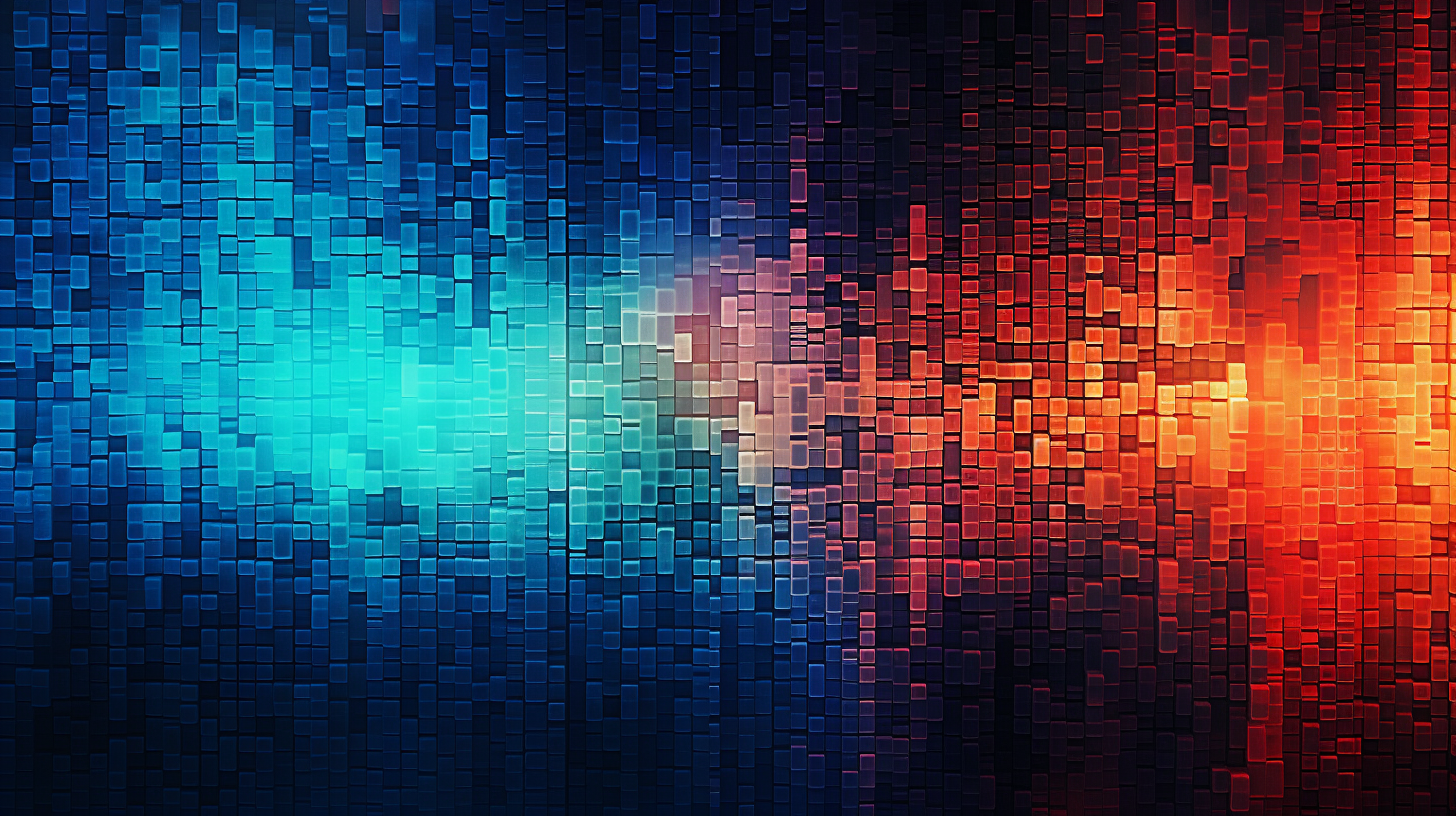
Python for Data Mining: Techniques and Tools
Data mining is an essential process in the field of data science, allowing practitioners to extract meaningful patterns and insights from large datasets. In Python, data mining leverages various libraries and techniques that facilitate the manipulation, analysis, and visualization of data, making it one of the most versatile tools in the data scientist’s toolkit.
At its core, data mining involves several key steps: data collection, data preprocessing, exploratory data analysis (EDA), model building, and model evaluation. Python’s rich ecosystem provides robust support for each of these phases, enabling users to efficiently handle, analyze, and draw conclusions from complex data.
Python’s simplicity and readability make it particularly appealing for data mining tasks. The language allows for rapid prototyping, meaning analysts can quickly iterate on their findings. This aligns well with the exploratory nature of data mining, where hypotheses are tested and refined in an agile manner.
Beyond its syntactic advantages, Python boasts a variety of powerful libraries designed specifically for data mining. These libraries offer functionalities that simplify the implementation of algorithms and the execution of complex data transformations.
The integration of tools like Pandas for data manipulation, Matplotlib and Seaborn for visualization, and Scikit-learn for machine learning provides a comprehensive environment for executing data mining projects. The following Python code snippet demonstrates how to load a dataset using Pandas and display its basic characteristics:
import pandas as pd # Load dataset data = pd.read_csv('data.csv') # Show the first few rows of the dataset print(data.head()) # Display summary statistics print(data.describe())
Through this simple interaction with Python, users can readily engage with their data, observing initial trends and distributions. This lays the foundation for deeper analysis.
As we delve deeper into specific techniques and tools, it becomes clear that the combination of Python’s flexibility and rich library support is what makes it a primary choice for data mining practitioners. The language not only meets the technical demands of data mining but also fosters an intuitive workflow that aligns with the analytical mindset.
Essential Libraries for Data Mining
To fully harness the capabilities of Python in data mining, it very important to understand the essential libraries that serve as the backbone for data manipulation, processing, and analysis. Each library brings unique features to the table, catering to different aspects of the data mining process, ensuring that analysts can work efficiently and effectively.
Pandas is arguably the most important library for data manipulation in Python. It provides data structures like DataFrames that allow for easy handling of structured data, making it simple to perform operations such as filtering, grouping, and aggregating data. The following example demonstrates how to use Pandas to filter data based on specific conditions:
import pandas as pd # Load dataset data = pd.read_csv('data.csv') # Filter rows where a specific condition is met filtered_data = data[data['column_name'] > threshold_value] # Show the filtered results print(filtered_data)
In addition to data manipulation, visualization is a critical component of data mining, helping to uncover patterns and insights visually. Libraries such as Matplotlib and Seaborn are widely used for this purpose. Matplotlib provides a low-level interface for creating static, interactive, and animated visualizations, while Seaborn builds on Matplotlib and offers more advanced statistical graphics.
Here’s an example of how to create a simple scatter plot using Seaborn:
import seaborn as sns import matplotlib.pyplot as plt # Load dataset data = pd.read_csv('data.csv') # Create a scatter plot sns.scatterplot(data=data, x='feature1', y='feature2', hue='category') plt.title('Scatter Plot of Feature1 vs Feature2') plt.show()
For machine learning tasks, Scikit-learn is the go-to library, providing a wide array of algorithms for classification, regression, clustering, and more. Its consistent API makes it easy to switch between different models and evaluate their performance. Here’s how you might implement a simple linear regression model using Scikit-learn:
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Load dataset data = pd.read_csv('data.csv') # Define features and target variable X = data[['feature1', 'feature2']] y = data['target'] # Split the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = LinearRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, predictions) print(f'Mean Squared Error: {mse}')
Each of these libraries—Pandas, Matplotlib, Seaborn, and Scikit-learn—plays a vital role in the data mining workflow, bridging the gap between data manipulation and analytical insights. By mastering these tools, practitioners can elevate their data mining capabilities, enabling them to extract deeper insights and drive informed decision-making.
Data Preprocessing Techniques
Data preprocessing is an important step in the data mining process, as the quality of the data directly impacts the performance of any analytical model. In Python, preprocessing involves a series of techniques aimed at cleaning and transforming raw data into a format that is more suitable for analysis. This step is essential for ensuring that the data is accurate, consistent, and relevant.
Common data preprocessing techniques include handling missing values, encoding categorical variables, normalizing or standardizing numerical features, and removing duplicates. Each of these steps can significantly influence the outcome of data mining tasks. Let’s explore these techniques in more detail, accompanied by Python code examples using Pandas.
Handling Missing Values
Missing values can arise from various sources, such as incomplete data collection or errors during data entry. In Python, the Pandas library provides simpler methods to identify and handle missing data. You can choose to either drop rows with missing values or fill them with a statistic, such as the mean or median of the column. Here’s an example of how to handle missing values:
import pandas as pd # Load dataset data = pd.read_csv('data.csv') # Display the count of missing values per column print(data.isnull().sum()) # Fill missing values with the mean of each column data.fillna(data.mean(), inplace=True) # Alternatively, drop rows with any missing values # data.dropna(inplace=True)
Encoding Categorical Variables
Categorical features often need to be converted into a numerical format so that they can be used in machine learning algorithms. This can be achieved through techniques such as one-hot encoding or label encoding. Here’s how to perform one-hot encoding using Pandas:
# Load dataset data = pd.read_csv('data.csv') # One-hot encode categorical variables data_encoded = pd.get_dummies(data, columns=['categorical_column'], drop_first=True) print(data_encoded.head())
Normalizing and Standardizing Numerical Features
Normalization and standardization are techniques used to scale numerical features. Normalization adjusts the values within a range, typically between 0 and 1, while standardization transforms the data to have a mean of 0 and a standard deviation of 1. This ensures that all features contribute equally during model training. Below is an example of standardizing data:
from sklearn.preprocessing import StandardScaler # Load dataset data = pd.read_csv('data.csv') # Initialize the scaler scaler = StandardScaler() # Select numerical features numerical_features = data[['feature1', 'feature2']] # Fit and transform the features data[numerical_features.columns] = scaler.fit_transform(numerical_features) print(data.head())
Removing Duplicates
Data duplication can occur due to various reasons, such as merging multiple datasets or errors in data collection. It is important to identify and remove duplicate entries to maintain the integrity of the analysis. Here’s an example of how to remove duplicates with Pandas:
# Load dataset data = pd.read_csv('data.csv') # Remove duplicate rows data.drop_duplicates(inplace=True) print(f'Duplicates removed: {data.duplicated().sum()}')
These preprocessing techniques are foundational steps that prepare the data for more advanced analysis, ensuring that the data mining process is both effective and efficient. Mastery of these techniques allows analysts to work confidently with data, paving the way for deeper exploratory analysis and model building.
Exploratory Data Analysis with Python
Exploratory Data Analysis (EDA) is a fundamental step in the data mining process that allows practitioners to gain insights into the underlying structure and relationships within their data. By employing various visualization and statistical techniques, EDA helps in identifying patterns, detecting anomalies, and formulating hypotheses that can guide subsequent analysis. In Python, EDA is facilitated by powerful libraries such as Pandas, Matplotlib, and Seaborn, which provide the tools necessary to explore data effectively.
The first step in EDA typically involves summarizing the dataset to understand its dimensions, types of variables, and the presence of any missing values. Using Pandas, this can be achieved easily. Here’s an example of how to obtain a brief overview of a dataset:
import pandas as pd # Load the dataset data = pd.read_csv('data.csv') # Display basic information about the dataset print(data.info()) # Show descriptive statistics for numerical features print(data.describe())
Once you have a basic understanding of the dataset, visualizations can be employed to further explore the relationships between variables. Scatter plots, histograms, box plots, and heatmaps are common visualization techniques that can reveal insights into the data’s distribution and correlations.
For instance, scatter plots can be used to examine the relationship between two numerical variables. Below is an example using Seaborn:
import seaborn as sns import matplotlib.pyplot as plt # Load the dataset data = pd.read_csv('data.csv') # Create a scatter plot to visualize the relationship between two features sns.scatterplot(data=data, x='feature1', y='feature2', hue='category') plt.title('Scatter Plot of Feature1 vs Feature2') plt.xlabel('Feature 1') plt.ylabel('Feature 2') plt.show()
In addition to scatter plots, histograms can provide insights into the distribution of a single variable. The following code illustrates how to create a histogram to visualize the distribution of a specific feature:
# Create a histogram to visualize the distribution of a feature plt.figure(figsize=(10, 6)) sns.histplot(data['feature1'], bins=30, kde=True) plt.title('Distribution of Feature1') plt.xlabel('Feature 1') plt.ylabel('Frequency') plt.show()
Box plots offer another powerful visualization for identifying outliers and understanding the spread of the data. The example below demonstrates how to create a box plot to compare the distributions of a numerical variable across different categories:
# Create a box plot to compare distributions across categories plt.figure(figsize=(10, 6)) sns.boxplot(data=data, x='category', y='feature1') plt.title('Box Plot of Feature1 by Category') plt.xlabel('Category') plt.ylabel('Feature 1') plt.show()
Correlation heatmaps are particularly useful for visualizing the strength and direction of relationships between multiple numerical variables. The following example shows how to create a heatmap using Seaborn:
# Calculate the correlation matrix correlation_matrix = data.corr() # Create a heatmap to visualize correlations plt.figure(figsize=(12, 8)) sns.heatmap(correlation_matrix, annot=True, fmt='.2f', cmap='coolwarm', square=True) plt.title('Correlation Heatmap') plt.show()
EDA is not just about generating visualizations; it also involves conducting statistical tests to validate hypotheses about the data. By using libraries like SciPy alongside Pandas, analysts can perform tests such as t-tests, chi-squared tests, and ANOVA to draw more rigorous conclusions from the data.
Ultimately, the aim of EDA is to transform raw data into a comprehensible form that can inform the next steps in the data mining workflow. With Python’s versatile libraries, analysts can engage with their data interactively, uncovering insights that drive informed decision-making and model development.
Building and Evaluating Predictive Models
Building predictive models is a pivotal stage in the data mining process, where the goal is to leverage historical data to make informed predictions about future outcomes. In Python, this process is streamlined through the use of various libraries that provide pre-built algorithms, enabling users to focus on model selection, training, and evaluation rather than the intricacies of algorithm implementation.
The journey of building a predictive model begins with data preparation, where the dataset is split into training and testing subsets. This is an important step, as the model learns patterns from the training data and is subsequently evaluated on the unseen testing data to assess its performance. Python’s Scikit-learn library excels in this area, providing simple functions to accomplish this task efficiently. Below is an example of how to split a dataset:
from sklearn.model_selection import train_test_split import pandas as pd # Load dataset data = pd.read_csv('data.csv') # Define features and target variable X = data[['feature1', 'feature2']] y = data['target'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
With the data split, the next step involves selecting a suitable model based on the nature of the problem—whether it’s a classification task, regression, or clustering. Scikit-learn provides an extensive library of algorithms ranging from linear regression to decision trees, support vector machines, and ensemble methods. For illustrative purposes, let’s implement a decision tree classifier:
from sklearn.tree import DecisionTreeClassifier # Initialize the model model = DecisionTreeClassifier(random_state=42) # Train the model model.fit(X_train, y_train)
Once the model is trained, the next logical step is to make predictions on the testing set and evaluate the model’s performance. Evaluation metrics vary depending on the type of predictive task at hand. For classification tasks, accuracy, precision, recall, and F1-score are commonly used metrics, while for regression tasks, metrics like Mean Squared Error (MSE) or R² score are more applicable. Here is how you can evaluate the decision tree classifier:
from sklearn.metrics import accuracy_score, classification_report # Make predictions predictions = model.predict(X_test) # Evaluate the model accuracy = accuracy_score(y_test, predictions) print(f'Accuracy: {accuracy:.2f}') # Display a detailed classification report print(classification_report(y_test, predictions))
For regression tasks, the evaluation process is similar, but we will focus on MSE to determine how close the predicted values are to the actual values:
from sklearn.metrics import mean_squared_error # Make predictions for regression model predictions = model.predict(X_test) # Calculate Mean Squared Error mse = mean_squared_error(y_test, predictions) print(f'Mean Squared Error: {mse:.2f}')
In addition to building and evaluating models, hyperparameter tuning is another critical step that can significantly enhance model performance. Scikit-learn offers techniques such as Grid Search and Random Search to optimize these parameters. For example, using Grid Search to find the best parameters for a decision tree can be done as follows:
from sklearn.model_selection import GridSearchCV # Define the model and parameters to tune model = DecisionTreeClassifier(random_state=42) param_grid = {'max_depth': [None, 10, 20, 30], 'min_samples_split': [2, 5, 10]} # Setup the grid search grid_search = GridSearchCV(model, param_grid, cv=5) # Fit the grid search to the data grid_search.fit(X_train, y_train) # Retrieve the best parameters print(f'Best parameters: {grid_search.best_params_}')
Finally, once a satisfactory model is achieved, it can be deployed for real-world applications, transforming insights into actionable outcomes. Whether predicting customer behaviors, forecasting sales, or identifying anomalies, well-constructed predictive models serve as critical tools in the data mining arsenal.