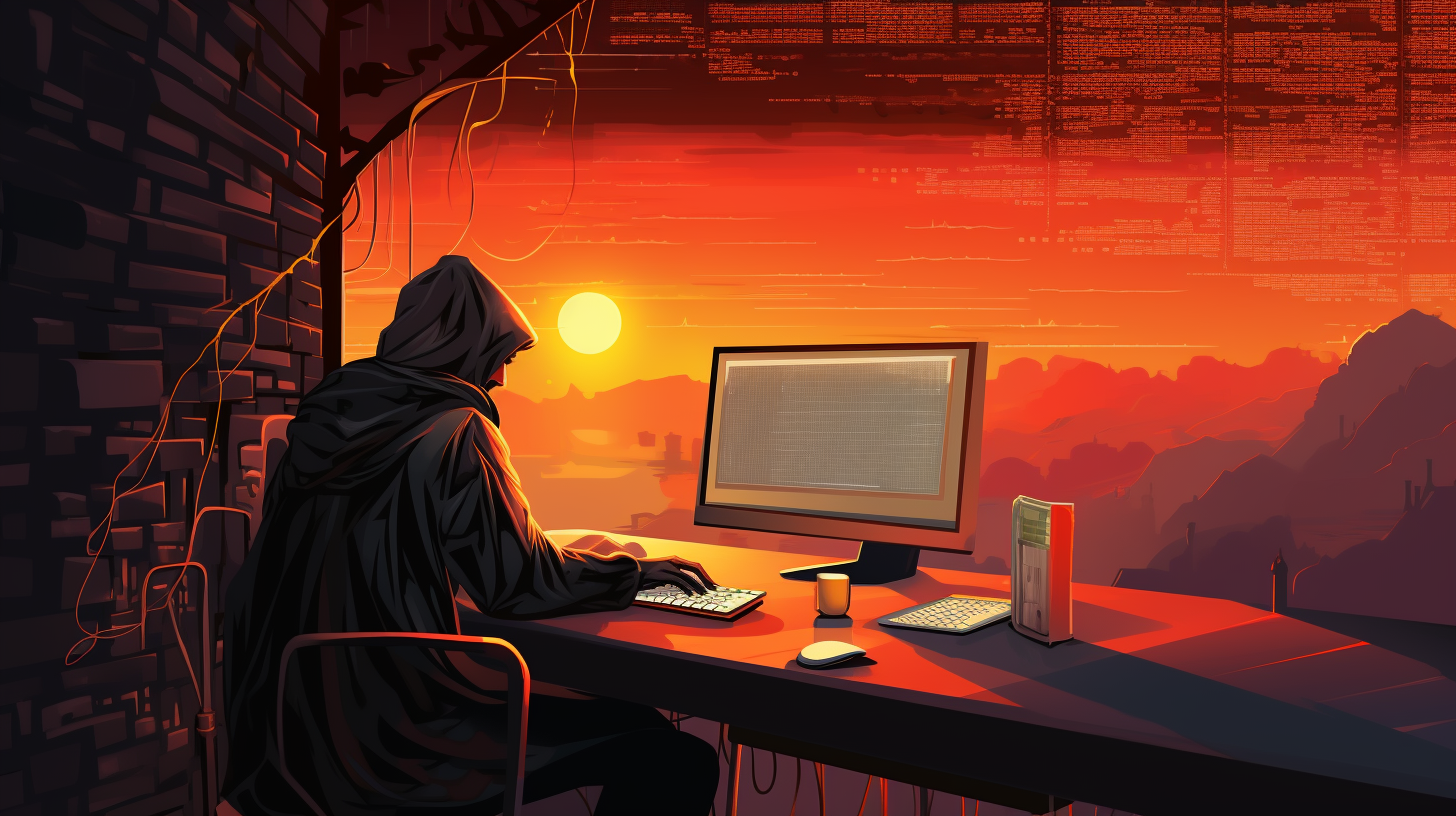
JavaScript and Browser Compatibility
JavaScript is governed by a series of standards and specifications that shape its behavior and capabilities across different environments. The primary body responsible for these standards is the ECMA International, particularly through its ECMAScript specification, which defines the core syntax, semantics, and features of the language. Each version of ECMAScript introduces new features, and browsers strive to implement these features consistently, albeit with varying degrees of success.
Understanding these standards very important when developing applications that need to run seamlessly across different browsers. The ECMAScript specification evolves over time, and the current version as of this writing is ECMAScript 2023 (ES14), which includes features such as weak references and improvements to asynchronous programming.
To show how these standards translate into actual code, consider the following example of using the optional chaining feature, introduced in ECMAScript 2020 (ES11). This feature allows developers to safely access deeply nested properties without having to explicitly check for the existence of each level in the hierarchy.
const user = { profile: { address: { city: "New York" } } }; const city = user.profile?.address?.city; // "New York" const country = user.profile?.address?.country; // undefined
In the above code, the ?.
operator allows the code to fail gracefully if any property in the chain does not exist, avoiding runtime errors that can arise in less robust code.
However, while ECMAScript provides a framework for JavaScript’s functionality, browser vendors have varying timelines for implementing these specifications. For example, while most modern browsers have embraced the optional chaining operator, older versions of Internet Explorer do not support it at all. This discrepancy can lead to compatibility issues that developers must navigate.
Additionally, JavaScript’s dynamic typing and prototype-based inheritance can also result in behavior that differs between browsers, particularly when it comes to how these features are interpreted. Understanding these subtleties is vital for ensuring that your scripts perform as intended across all platforms.
Common Browser Compatibility Issues
When developing JavaScript applications, one of the most pressing challenges developers face is the variation in how different browsers implement JavaScript features. This inconsistency can lead to numerous compatibility issues that impact the functionality and user experience of web applications. Understanding these issues is essential for any serious JavaScript programmer.
One common compatibility problem arises from the differences in support for newer ECMAScript features across browsers. For instance, while modern browsers such as Chrome, Firefox, and Edge have adopted features from the latest ECMAScript versions, older browsers like Internet Explorer lag significantly behind. This can lead to runtime errors or unexpected behavior when code that relies on these new features is executed in an unsupported environment. For example, think the following code that uses the `Array.prototype.includes` method, introduced in ECMAScript 2016 (ES7):
const fruits = ['apple', 'banana', 'mango']; console.log(fruits.includes('banana')); // true console.log(fruits.includes('orange')); // false
If this code is run in an environment that does not support the `includes` method, such as an older version of Internet Explorer, it will result in an error.
Another source of compatibility issues stems from the differences in how browsers handle JavaScript execution contexts, particularly with respect to asynchronous programming. The way Promises and async/await are implemented can differ, leading to discrepancies in how asynchronous code behaves across browsers. For instance, consider the following asynchronous function:
async function fetchData(url) { const response = await fetch(url); const data = await response.json(); return data; }
While most modern browsers will handle this code correctly, older browsers that do not support `async/await` will throw syntax errors. This necessitates the use of polyfills or transpilers like Babel to ensure broader compatibility.
Developers must also be wary of the variances in the implementation of the Document Object Model (DOM) and events. While most DOM methods are standardized, subtle differences can lead to bugs. For example, event handling can vary slightly between browsers, particularly with respect to the `event` object. Using the `addEventListener` method is generally safe, but developers should be cautious about relying on the `event` object’s properties, as they may not behave identically across all browsers.
Moreover, CSS and JavaScript often interact in ways that can expose compatibility issues. Certain CSS properties or values may not be recognized by all browsers, which can affect JavaScript-driven animations or element manipulations. A developer may attempt to set a CSS property using JavaScript:
document.getElementById('myElement').style.transition = 'all 0.5s ease';
If the `transition` property is not supported in a particular browser, the expected animation will not occur, potentially leading to user frustration.
Tools and Techniques for Testing Compatibility
When it comes to ensuring that JavaScript code runs smoothly across various browsers, using the right tools and techniques for testing compatibility is paramount. A well-rounded approach involves using a combination of automated testing frameworks, polyfills, and transpilers, as well as employing browser-specific testing tools. By integrating these tools into your development workflow, you can significantly reduce the risk of compatibility issues and enhance the reliability of your web applications.
One of the most effective ways to test for compatibility is through automated testing frameworks. Frameworks like Jest and Mocha allow developers to write unit tests that can be run across multiple environments. These tests can be structured to check whether specific features are supported in different browsers, providing immediate feedback when compatibility issues arise. For instance, to check for the support of the `Promise` object, you could write a simple test case:
describe('Promise support', () => { it('should support Promises', () => { expect(typeof Promise).toBe('function'); }); });
This snippet checks whether the `Promise` object exists, allowing developers to identify browsers that may not support this critical feature.
Polyfills are another essential tool for ensuring that your JavaScript code remains functional across different browsers. A polyfill is essentially a piece of code that provides missing functionality in older browsers. For example, if you are using the `Array.prototype.includes` method, which may not be supported in older environments, you can include a polyfill like so:
if (!Array.prototype.includes) { Array.prototype.includes = function(searchElement, fromIndex) { // Implementation of includes method if (this == null) { throw new TypeError('"this" is null or not defined'); } const O = Object(this); const len = O.length >>> 0; if (len === 0) return false; let n = fromIndex | 0; let k = Math.max(n >= 0 ? n : len - Math.abs(n), 0); while (k < len) { if (O[k] === searchElement) return true; k++; } return false; }; }
By including this polyfill, you ensure that the `includes` method will be available in browsers that do not natively support it, allowing your application to work seamlessly across environments.
Transpilers, such as Babel, provide another layer of protection against compatibility issues by converting modern JavaScript syntax into a version that’s compatible with older browsers. For example, if you are using modern ES6+ features like arrow functions or `let`/`const`, Babel can transpile this code into ES5 syntax, which is widely supported. An example configuration for Babel might look like this:
{ "presets": ["@babel/preset-env"] }
By setting up Babel with this configuration, you can develop using the latest JavaScript features while ensuring that the resulting code will operate in older browsers.
In addition to these tools, employing browser-specific testing tools can streamline your compatibility testing process. Services like BrowserStack and Sauce Labs allow developers to run their code in real-time across a myriad of browser and operating system combinations. By using these platforms, you can quickly identify any issues related to browser compatibility, enabling rapid iteration and fixes. For example, testing tools can show if a specific feature behaves differently in Chrome compared to Firefox, allowing developers to address discrepancies directly.
Best Practices for Writing Cross-Browser JavaScript Code
Writing cross-browser JavaScript code requires a keen understanding of the discrepancies between different environments. Developers must adopt best practices that not only account for these differences but also promote code simplicity and maintainability. Here are several strategies to improve the compatibility and robustness of JavaScript code across various browsers.
1. Feature Detection Over Browser Detection
One of the cardinal rules of cross-browser development is to employ feature detection rather than relying on browser detection. Relying on the user agent string to determine browser capabilities can lead to unreliable results since user agent strings can be spoofed easily. Instead, use feature detection libraries like Modernizr or write your own checks. For instance, to check for the existence of the `fetch` API, you can do the following:
if (window.fetch) { // Fetch is supported fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Fetch error:', error)); } else { console.error('Fetch API is not supported in this browser.'); }
This approach ensures that you only use features supported by the user’s browser, thus avoiding potential runtime errors.
2. Avoid Using Deprecated or Non-Standard Features
While it may be tempting to utilize certain non-standard or deprecated JavaScript features for quick solutions, this often leads to longer-term problems. Browsers may not support these features consistently, and they may be removed in future versions. Always refer to up-to-date documentation and avoid methods or properties that have been marked as obsolete. For example, the `document.all` collection was once widely used but is now considered a relic of the past:
if (document.all) { console.log('This browser supports document.all'); } else { console.log('document.all is deprecated and not supported'); }
Instead, opt for standard methods that are widely supported and part of the ECMAScript specification.
3. Use Polyfills Wisely
Polyfills can be a lifesaver for ensuring compatibility with older browsers. However, they should be used judiciously. Adding unnecessary polyfills can bloat your codebase and lead to performance issues. Only include polyfills for features that you’re actually using in your application. Here’s how you might include a polyfill for the `Array.prototype.includes` method:
if (!Array.prototype.includes) { Array.prototype.includes = function(searchElement, fromIndex) { // Implementation here }; }
By checking if the method already exists before defining it, you prevent multiple definitions and keep your code cleaner.
4. Write Modular Code
Modular code promotes maintainability and allows you to isolate browser-specific code. Using modules, whether through ES6 imports or using tools like CommonJS or AMD, helps in organizing code into independent sections that can be tested and updated separately. Here’s an example of creating a simple module:
export function add(a, b) { return a + b; }
This modular approach makes it easier to adapt or replace specific features without disrupting the entire codebase.
5. Graceful Degradation and Progressive Enhancement
Implementing graceful degradation and progressive enhancement ensures that your application remains functional across various environments. Start with a basic version of your application that works on all browsers, then enhance it with advanced features for modern browsers. For example, you can utilize CSS animations for modern browsers while providing a fallback for older browsers:
function animateElement(element) { if (element.animate) { element.animate([{ transform: 'scale(1)' }, { transform: 'scale(1.1)' }], { duration: 500, iterations: 1 }); } else { // Fallback for older browsers element.style.transition = 'transform 0.5s'; element.style.transform = 'scale(1.1)'; } }
By prioritizing functionality, you cater to all users, regardless of their browser choice.
6. Testing Across Different Browsers and Devices
Finally, rigorous testing is paramount. Regularly evaluate your application across multiple browsers and devices, including mobile platforms. Emulators and virtual testing environments can facilitate this process, making it easier to identify and address compatibility issues. Utilize tools such as Selenium or Cypress for automated testing to streamline this process. Moreover, think using A/B testing frameworks to gather real user data on performance across different platforms.