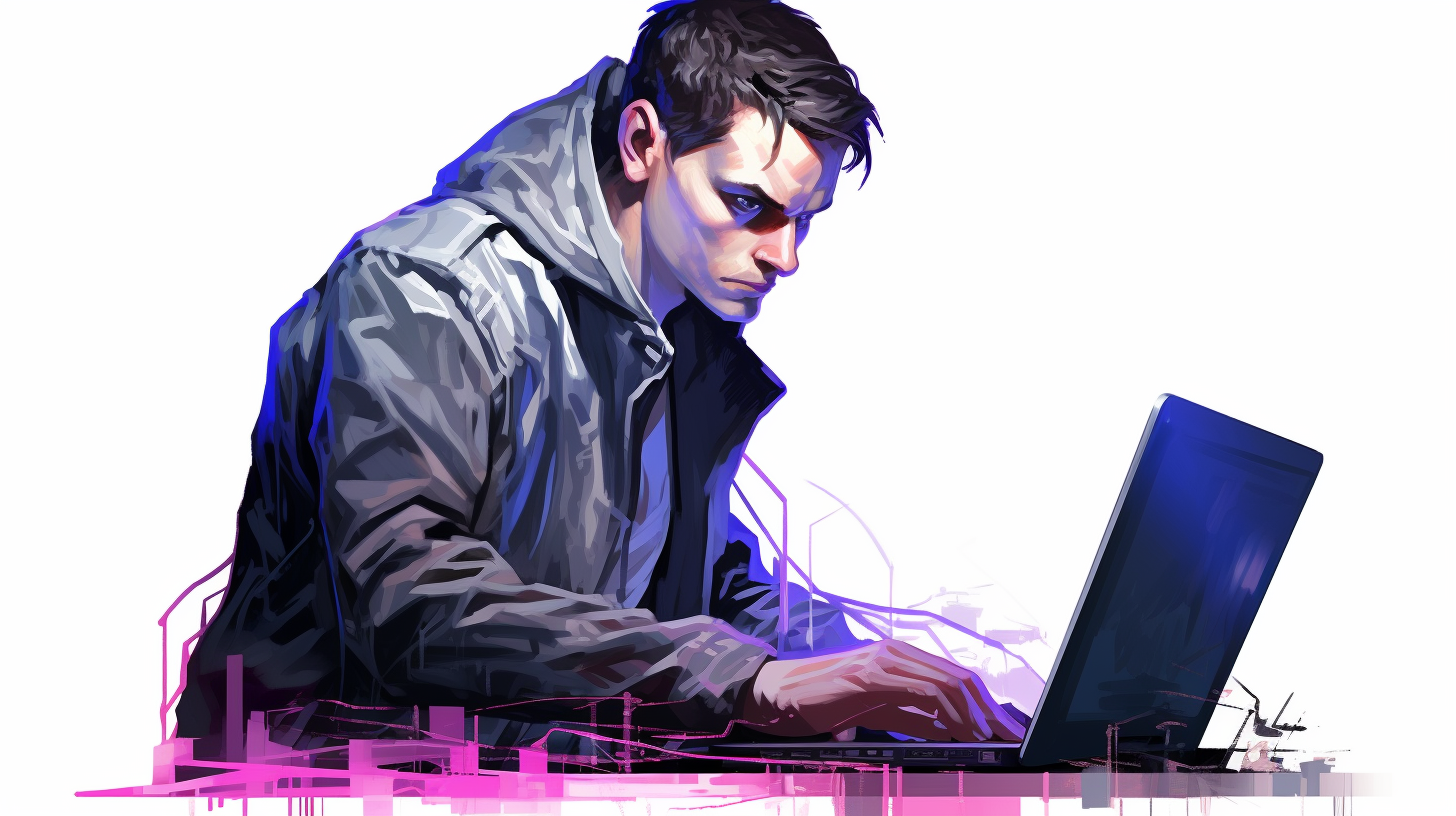
Swift and App Store Submission
When it comes to submitting your Swift application to the App Store, understanding the App Store Guidelines is pivotal. These guidelines are not merely rules; they’re a framework designed to ensure a seamless experience for users and developers alike. Apple meticulously curates the app ecosystem, which means that any app submitted must comply with specific standards and requirements.
Firstly, the guidelines cover a range of topics including safety, performance, business, design, and legal considerations. The Safety section mandates that your app should not harm users or their devices. This includes ensuring that your app does not contain offensive content or violate user privacy.
The Performance guidelines emphasize that your app must be fully functional, stable, and free of bugs. Users expect a smooth experience, so make sure you rigorously test your app on various devices and iOS versions prior to submission.
In terms of Design, Apple has a distinctive aesthetic and user interface philosophy. Your app should align with these principles, ensuring a cohesive look and feel that resonates with Apple’s design standards. This means using native components and adhering to the Human Interface Guidelines provided by Apple.
import UIKit class MyCustomViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Customize your UI elements here view.backgroundColor = .white let titleLabel = UILabel() titleLabel.text = "Welcome to My App" titleLabel.font = UIFont.boldSystemFont(ofSize: 24) titleLabel.textColor = .black titleLabel.textAlignment = .center titleLabel.frame = CGRect(x: 0, y: 100, width: view.frame.width, height: 50) view.addSubview(titleLabel) } }
From a Business perspective, your app must comply with all market regulations. This includes any in-app purchases, subscriptions, and advertising policies. Make sure to familiarize yourself with Apple’s payment processing guidelines to avoid rejections.
Lastly, the Legal section addresses copyright issues, intellectual property rights, and compliance with local laws. This includes obtaining the necessary permissions if your app uses third-party content or services.
Preparing Your Swift App for Submission
Once you’re armed with a proper understanding of the App Store Guidelines, the next crucial step is preparing your Swift app for submission. This involves multiple stages, each vital for ensuring that your app not only meets Apple’s requirements but also resonates with users.
First and foremost, ensure that your app is thoroughly tested across an array of devices and iOS versions. Using XCTest, Apple’s testing framework, allows you to automate the testing process, ensuring that your app remains stable and functional. Think writing unit tests to cover critical functions and UI tests to validate the user interface.
import XCTest @testable import MyApp class MyAppTests: XCTestCase { func testExampleFunction() { let result = exampleFunction() XCTAssertEqual(result, expectedValue, "The example function did not return the expected value.") } }
Next, focus on the app’s metadata and assets. Properly prepare your app’s icons, screenshots, and description before submission. Apple requires specific resolutions for app icons, so ensure you follow the guidelines to avoid any last-minute hassles. Screenshots should reflect the key functionalities of your app, showcasing its best features to entice potential users.
func prepareScreenshots() { let screenshots = [ "screenshot1.png", // Home Screen "screenshot2.png", // Feature Overview "screenshot3.png" // User Experience ] // Code to upload screenshots to App Store Connect }
Another aspect to think is the app’s localization. If your app targets multiple regions, ensure that it supports localization for various languages. Use the NSLocalizedString function to facilitate text localization within your app.
let welcomeMessage = NSLocalizedString("welcome_message", comment: "Welcome message for users")
Additionally, it’s essential to establish a robust privacy policy. This document should clearly outline how your app collects, uses, and shares user data. Apple mandates that all apps transparently communicate their data practices, so including a privacy policy URL in your app’s metadata is necessary.
Common Issues During Submission
Submitting your Swift application to the App Store can sometimes feel like navigating a minefield, especially when it comes to addressing common issues that may arise during the submission process. Even the most meticulously prepared app can encounter hurdles that might delay its journey to the App Store. Understanding these potential challenges is paramount for a smooth submission experience.
One of the frequent issues developers face is the dreaded “App Rejected” notification. This can stem from various reasons, including non-compliance with Apple’s guidelines, bugs in the app, or poor user experience. For example, if your app crashes on startup or contains broken links, it will likely be flagged during the review process. Therefore, it’s paramount to conduct extensive testing prior to submission.
import UIKit class AppLaunchTest: XCTestCase { func testAppLaunch() { let app = XCUIApplication() app.launch() XCTAssertTrue(app.isRunning, "The app did not launch successfully.") } }
Another significant issue that developers often encounter is related to app metadata. Poorly crafted app descriptions or using misleading screenshots can lead to rejection. Each app must clearly represent its functionality and provide accurate information to users. Take time to ensure that your app’s description is not only enticing but also simpler and honest about what users can expect.
In addition to these concerns, developers should also pay attention to the app’s pricing model and in-app purchases. If your app includes in-app purchases, they must be properly configured within App Store Connect. It’s critical to ensure that all pricing tiers are correctly set up and that the app does not contain any direct links for external purchases, as this violates Apple’s policies.
let purchase = SKPayment(product: myProduct) SKPaymentQueue.default().add(purchase)
Sometimes, issues arise from the app’s use of third-party libraries or frameworks. If any of these components have licensing restrictions or violate Apple’s guidelines, your app may face rejection. It’s wise to review the licenses of all third-party dependencies and ensure they comply with Apple’s requirements. Additionally, avoid using private APIs, as that’s a surefire way to get your app rejected.
Another common area of contention is app performance. Apple prioritizes user experience, which means that if your app drains battery life excessively, uses too much data, or is slow to respond, it could be flagged. Optimize your app to ensure it runs efficiently across all devices.
func optimizeMemoryUsage() { let largeArray = Array(1...1_000_000) // Use memory-efficient methods to handle large data sets }
Lastly, let’s not forget the importance of responding to reviewer feedback. If your app is rejected, Apple typically provides a reason. Addressing these points thoroughly and promptly can help expedite the re-review process. Clear communication with Apple through Resolution Center can also help clarify any misunderstandings regarding your app’s features or compliance with guidelines.
Post-Submission: What to Expect
Once your Swift app is submitted to the App Store, the next phase is to understand what to expect during the review process and beyond. Apple’s review team meticulously examines every app, focusing on compliance with their guidelines, performance, and overall user experience. What happens next can determine the fate of your app, so it’s crucial to be prepared.
Typically, the review process can take anywhere from a few hours to several days. During this time, you can check the status of your app in App Store Connect. If your app passes the review, you will receive a notification, and it will be made available on the App Store. However, if there are issues, you’ll receive feedback from Apple detailing the reasons for rejection.
It’s important to read this feedback carefully. Often, the reasons for rejection can be addressed by making necessary changes or fixes to your app. For instance, if Apple identifies a bug that causes a crash, you’ll need to debug and resolve that issue before resubmitting. Here’s a simple Swift example of how to log errors which can assist in tracing issues before submission:
func logError(_ error: Error) { print("Error occurred: (error.localizedDescription)") }
Upon receiving the rejection, it’s essential to act promptly. Address the issues outlined by the review team and provide a clear, concise explanation of the changes made when resubmitting your app. This communication can facilitate a smoother process and increase the chances of a successful approval on the next submission.
Beyond the review process, once your app is live, another phase begins: monitoring its performance. Using analytics tools such as Firebase or Mixpanel can help track user engagement, crashes, and other vital metrics. This data is invaluable for making informed decisions about future updates and improvements to your app.
Moreover, user feedback will start to pour in. Pay close attention to reviews and ratings on the App Store. Positive feedback can affirm your development choices, while constructive criticism can highlight areas needing attention. Responding to users, especially those who report issues or provide feedback, can enhance customer satisfaction and build a loyal user base.
Lastly, keep an eye on Apple’s evolving guidelines and policies. The App Store is a dynamic environment, and staying informed about any changes ensures that your app remains compliant and continues to thrive. Regular updates may also be necessary to address new features or requirements set forth by Apple, as well as to improve your app based on user input.