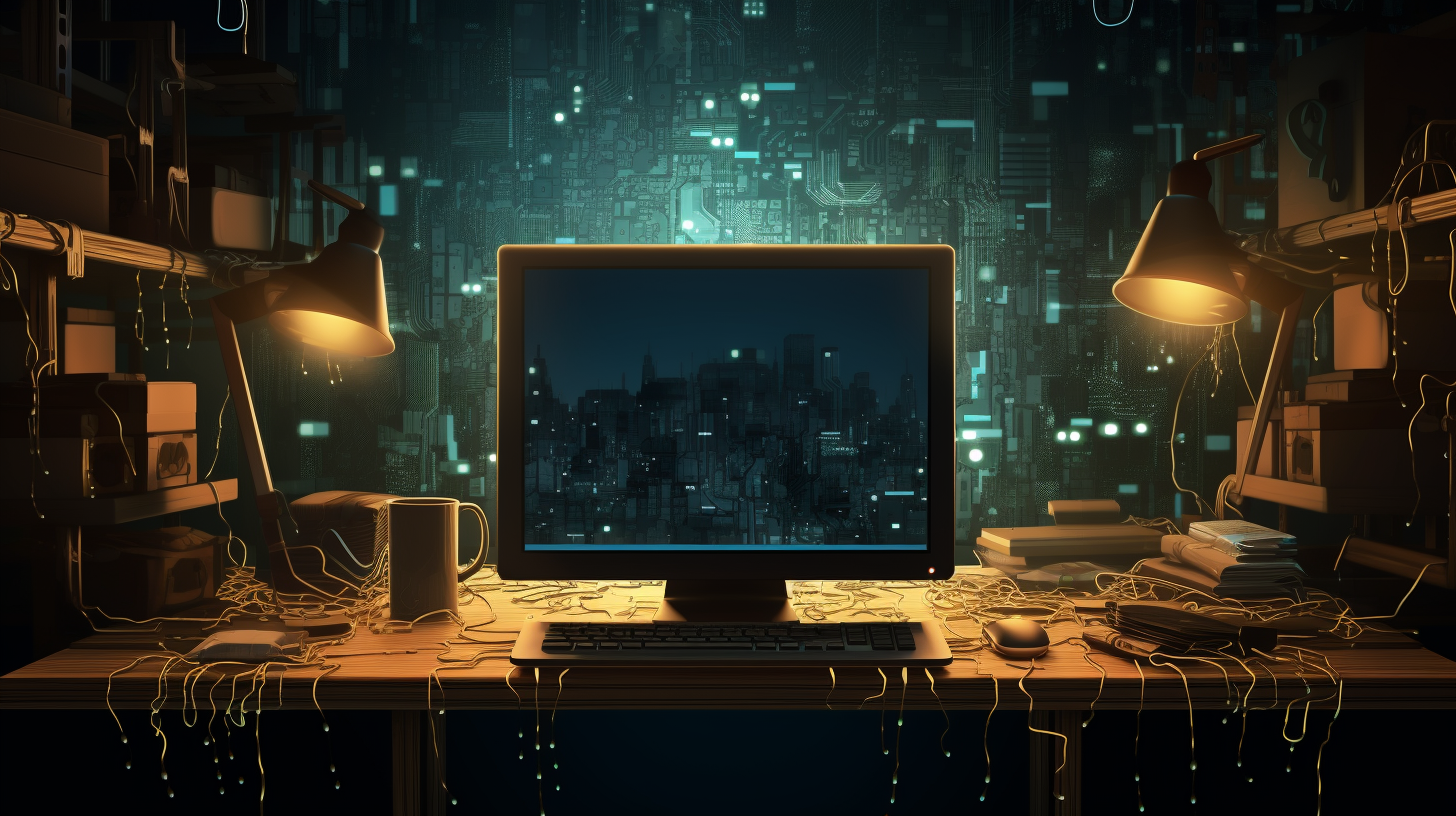
SQL for Data Lifecycle Management
Data Lifecycle Management (DLM) in SQL refers to the processes and strategies involved in managing data from its creation and initial storage to its eventual disposal. Understanding these stages is important for maintaining data integrity, optimizing storage, and ensuring compliance with regulations. At its core, DLM encompasses several key phases, including data creation, storage, usage, archiving, and deletion.
In SQL, the lifecycle of data can be effectively managed through a combination of database design, data governance practices, and specific SQL techniques. Each phase of the data lifecycle has a role to play in the efficiency and effectiveness of data management. For example, during the data creation phase, it’s essential to define data types and constraints properly to ensure data quality.
The storage phase involves choosing the right data model and indexing strategies to optimize performance. SQL provides various data types and indexing options that can significantly affect how data is stored and retrieved. Implementing appropriate normalization practices can also minimize redundancy and enhance data integrity.
As data is utilized, it’s critical to monitor its performance and adjust strategies accordingly. SQL queries should be optimized to ensure that data retrieval is fast and efficient. This can involve writing precise queries, using joins wisely, and using aggregate functions to summarize data without overwhelming the system.
When data becomes less frequently accessed, it enters the archiving phase. SQL can support this transition through partitioning tables, which can segregate active data from archived data, thus streamlining access to both types. Here’s an example of a SQL command for partitioning:
CREATE TABLE sales ( id INT, amount DECIMAL(10, 2), sale_date DATE ) PARTITION BY RANGE (YEAR(sale_date)) ( PARTITION p2019 VALUES LESS THAN (2020), PARTITION p2020 VALUES LESS THAN (2021), PARTITION p2021 VALUES LESS THAN (2022) );
Finally, the deletion phase is as significant as the others. Data this is no longer needed must be disposed of properly to free up resources and maintain compliance with data protection regulations. SQL commands like DELETE
or TRUNCATE
can be used here, but it’s vital to implement safeguards to avoid accidental data loss. Consider this example:
DELETE FROM sales WHERE sale_date < '2020-01-01';
Managing the data lifecycle effectively within SQL requires a comprehensive understanding of each phase and the tools available to facilitate these processes. Collaboration between database administrators, data architects, and end-users is essential to ensure that data is properly managed throughout its lifecycle.
Key SQL Techniques for Data Ingestion
Ingesting data into a SQL database is an important step in managing the data lifecycle effectively. This phase sets the foundation for data integrity, accessibility, and usability. Various techniques can be utilized to streamline the data ingestion process, ensuring that data is imported accurately and efficiently, aligning it with the overall DLM strategy.
One fundamental SQL technique for data ingestion is the use of the INSERT statement. This command allows for the addition of new records to a table. When performing bulk inserts, using the INSERT INTO … VALUES syntax with multiple sets of values can improve performance. Ponder the following example:
INSERT INTO employees (name, position, hire_date) VALUES ('Luke Douglas', 'Software Engineer', '2023-01-15'), ('Jane Smith', 'Data Scientist', '2023-01-16'), ('Emily Johnson', 'Project Manager', '2023-01-17');
When dealing with large datasets, using the LOAD DATA INFILE command can significantly enhance the speed of ingestion. This command allows you to import data directly from a file into a table, which is particularly useful for large volumes of data. Here’s how you might use it:
LOAD DATA INFILE '/path/to/datafile.csv' INTO TABLE sales FIELDS TERMINATED BY ',' LINES TERMINATED BY 'n' IGNORE 1 ROWS;
In addition to basic insertion techniques, SQL also offers the MERGE statement, which can be incredibly useful for changing data ingestion strategies. This command allows for conditional updates or inserts, depending on whether a record already exists in the table. This can prevent duplication and ensure data integrity. Here’s an example of how to use MERGE:
MERGE INTO target_table AS target USING source_table AS source ON target.id = source.id WHEN MATCHED THEN UPDATE SET target.value = source.value WHEN NOT MATCHED THEN INSERT (id, value) VALUES (source.id, source.value);
Another essential technique involves using staging tables. Staging tables act as temporary storage for raw data before it’s transformed and inserted into the final destination tables. This method allows for data cleaning and validation processes to be performed seamlessly. An example setup might look like this:
CREATE TABLE staging_employees ( id INT, name VARCHAR(100), position VARCHAR(50), hire_date DATE );
After validating the data in the staging table, it can then be moved to the final employees table using the previously mentioned techniques. This ensures that only clean and accurate data is ingested into the production environment.
To maintain data integrity during ingestion, it’s also important to implement proper error handling and logging mechanisms. Using transactions allows you to group multiple operations into a single unit of work, ensuring that either all operations succeed or none at all. For example:
BEGIN; INSERT INTO employees (name, position, hire_date) VALUES ('Alice Brown', 'Analyst', '2023-01-18'); INSERT INTO employees (name, position, hire_date) VALUES ('Tom White', 'Developer', '2023-01-19'); COMMIT;
Implementing these techniques not only enhances the efficiency of data ingestion but also helps to maintain the overall quality and reliability of the data within the SQL database. As organizations increasingly rely on data for decision-making, mastering these ingestion methodologies becomes essential for effective data lifecycle management.
Managing Data Retention and Archiving with SQL
Managing data retention and archiving in SQL is a critical aspect of data lifecycle management, focusing on the strategies and techniques used to keep data for as long as necessary while ensuring that it remains accessible and compliant with regulations. As data accumulates over time, organizations must implement robust policies for data retention and archiving to optimize performance and storage costs.
One of the primary techniques for managing data retention is to establish clear retention policies that determine how long different types of data should be kept. This can vary based on legal, regulatory, or business requirements. SQL provides various commands and functionalities that can assist in effectively implementing these policies.
For instance, to facilitate data archiving, SQL allows for the creation of archive tables. These tables can store historical data this is no longer needed for day-to-day operations but must be retained for future reference. Here’s an example of how to create an archive table:
CREATE TABLE archived_sales ( id INT, amount DECIMAL(10, 2), sale_date DATE );
Once the archive table is established, data can be moved from the main sales table to the archive table based on a defined retention policy. This can be done using the INSERT INTO … SELECT statement combined with a DELETE operation to remove the archived records from the original table:
INSERT INTO archived_sales (id, amount, sale_date) SELECT id, amount, sale_date FROM sales WHERE sale_date < DATE_SUB(CURDATE(), INTERVAL 1 YEAR); DELETE FROM sales WHERE sale_date < DATE_SUB(CURDATE(), INTERVAL 1 YEAR);
In addition to archiving, SQL Server and other database systems provide features such as partitioning, which allows you to manage large tables by dividing them into smaller, more manageable pieces. That is particularly useful for retention management, as you can easily drop entire partitions when the data therein is no longer needed. For example, you can drop an entire partition that contains data older than a certain date:
ALTER TABLE sales DROP PARTITION FOR VALUES LESS THAN (YEAR(CURDATE()) - 1);
Another essential consideration in retention management is the use of automated processes to periodically review and purge data this is no longer necessary. Implementing stored procedures or scheduled jobs can help automate these tasks, ensuring that data retention policies are adhered to consistently. A simple stored procedure can be created to handle the archiving and deletion processes:
CREATE PROCEDURE ArchiveOldSales AS BEGIN INSERT INTO archived_sales (id, amount, sale_date) SELECT id, amount, sale_date FROM sales WHERE sale_date < DATE_SUB(CURDATE(), INTERVAL 1 YEAR); DELETE FROM sales WHERE sale_date < DATE_SUB(CURDATE(), INTERVAL 1 YEAR); END;
Finally, it is crucial to ponder data retention in the context of compliance. Regulations such as GDPR or HIPAA require specific handling of data, including retention periods and methods of disposal. SQL provides the flexibility to implement such regulations within your data retention strategies, ensuring that data is stored securely and disposed of properly when it reaches the end of its useful life.
Managing data retention and archiving with SQL is not merely about removing old data; it involves a thoughtful approach that balances accessibility, compliance, and efficient resource management. By employing the various techniques and SQL functionalities discussed, organizations can ensure that their data lifecycle management is robust and effective. This proactive approach not only preserves the integrity of the data but also optimizes performance and storage use, setting a solid foundation for using data-driven insights.
Optimizing Data Retrieval and Cleanup Strategies
When it comes to optimizing data retrieval and cleanup strategies within SQL, the key is to focus on performance enhancement and ensuring that the database remains uncluttered and efficient. As data grows and becomes more complex, the necessity for effective retrieval methods and cleanup processes becomes critical. This section addresses various SQL techniques that can be employed to achieve these goals, ensuring that data management practices are both proactive and responsive.
One of the foundational strategies for optimizing data retrieval lies in the use of proper indexing. Indexes are data structures that improve the speed of data retrieval operations on a database table. They function similarly to an index in a book, allowing the database engine to locate data without having to scan every row in a table. Here’s an example of creating an index on a commonly queried column:
CREATE INDEX idx_sale_date ON sales (sale_date);
With this index in place, queries that filter or sort by the sale_date column will execute more quickly, leading to improved performance. However, it’s essential to balance the number of indexes, as excessive indexing can slow down data modification operations such as INSERT, UPDATE, and DELETE.
Additionally, optimizing SQL queries themselves plays a critical role in improving data retrieval. This includes avoiding unnecessary columns in SELECT statements, using WHERE clauses to filter data effectively, and making use of JOIN operations rather than subqueries where appropriate. For example, ponder the following query that retrieves sales data for a specific year:
SELECT id, amount, sale_date FROM sales WHERE YEAR(sale_date) = 2022;
While this query is simpler, it can be optimized further by ensuring that the sale_date column is indexed, allowing for quicker access to the relevant records.
Another technique for optimizing data retrieval is to utilize query caching. Many SQL database systems offer caching mechanisms that store the results of frequently executed queries, allowing subsequent queries to fetch results directly from the cache rather than re-executing the query. This can significantly reduce the load on the database and improve response times for end-users.
Moving on to cleanup strategies, regular maintenance of the database very important to ensure that it remains efficient and performant. One effective approach involves the use of the DELETE command to remove obsolete records. However, it’s essential to execute these operations wisely to avoid locking issues and performance degradation. For instance, instead of deleting a large number of records in a single transaction, which can cause locking and performance bottlenecks, it’s often better to batch the DELETE operations:
DELETE FROM sales WHERE sale_date < '2020-01-01' LIMIT 1000;
This command deletes a batch of records at a time, thereby reducing the strain on the database and allowing other operations to proceed simultaneously.
Furthermore, implementing the TRUNCATE command can be a highly efficient way to remove all records from a table when the data is no longer needed. TRUNCATE operates differently from DELETE in that it does not log individual row deletions, making it faster for large datasets:
TRUNCATE TABLE sales;
While TRUNCATE is powerful, it should be used with caution as it cannot be rolled back in most systems once executed.
Regular maintenance tasks such as reorganizing and rebuilding indexes can also contribute to optimized data retrieval and cleanup. Over time, as data is inserted, updated, and deleted, indexes can become fragmented, leading to performance degradation. Running maintenance commands can help realign these indexes:
ALTER INDEX idx_sale_date REBUILD;
This command rebuilds the specified index, which can restore its efficiency and improve retrieval times.
Implementing comprehensive logging and monitoring mechanisms is equally crucial. By tracking query performance and identifying slow-running queries, database administrators can proactively address potential issues before they escalate. Tools and SQL commands that gather query statistics can provide valuable insights into how to optimize both data retrieval and cleanup strategies effectively.
Optimizing data retrieval and cleanup strategies involves a multifaceted approach that includes indexing, efficient query writing, regular maintenance, and effective logging practices. By adopting these SQL techniques, organizations can ensure that their databases remain responsive, reliable, and ready to meet the demands of their users.